KBookmark::List
#include <kbookmark.h>
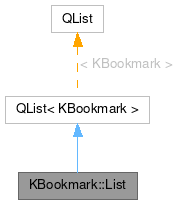
Public Member Functions | |
void | populateMimeData (QMimeData *mimeData) const |
![]() | |
QList (const QList< T > &other) | |
QList (const QList< T > &other) | |
QList (InputIterator first, InputIterator last) | |
QList (InputIterator first, InputIterator last) | |
QList (QList< T > &&other) | |
QList (QList< T > &&other) | |
QList (qsizetype size) | |
QList (qsizetype size) | |
QList (qsizetype size, parameter_type value) | |
QList (qsizetype size, parameter_type value) | |
QList (std::initializer_list< T > args) | |
QList (std::initializer_list< T > args) | |
void | append (const QList< T > &value) |
void | append (const QList< T > &value) |
void | append (parameter_type value) |
void | append (parameter_type value) |
void | append (QList< T > &&value) |
void | append (QList< T > &&value) |
void | append (rvalue_ref value) |
void | append (rvalue_ref value) |
const_reference | at (qsizetype i) const const |
const_reference | at (qsizetype i) const const |
reference | back () |
reference | back () |
const_reference | back () const const |
const_reference | back () const const |
iterator | begin () |
iterator | begin () |
const_iterator | begin () const const |
const_iterator | begin () const const |
qsizetype | capacity () const const |
qsizetype | capacity () const const |
const_iterator | cbegin () const const |
const_iterator | cbegin () const const |
const_iterator | cend () const const |
const_iterator | cend () const const |
void | clear () |
void | clear () |
const_iterator | constBegin () const const |
const_iterator | constBegin () const const |
const_pointer | constData () const const |
const_pointer | constData () const const |
const_iterator | constEnd () const const |
const_iterator | constEnd () const const |
const T & | constFirst () const const |
const T & | constFirst () const const |
const T & | constLast () const const |
const T & | constLast () const const |
bool | contains (const AT &value) const const |
bool | contains (const AT &value) const const |
qsizetype | count () const const |
qsizetype | count () const const |
qsizetype | count (const AT &value) const const |
qsizetype | count (const AT &value) const const |
const_reverse_iterator | crbegin () const const |
const_reverse_iterator | crbegin () const const |
const_reverse_iterator | crend () const const |
const_reverse_iterator | crend () const const |
pointer | data () |
pointer | data () |
const_pointer | data () const const |
const_pointer | data () const const |
iterator | emplace (const_iterator before, Args &&... args) |
iterator | emplace (const_iterator before, Args &&... args) |
iterator | emplace (qsizetype i, Args &&... args) |
iterator | emplace (qsizetype i, Args &&... args) |
reference | emplace_back (Args &&... args) |
reference | emplace_back (Args &&... args) |
reference | emplaceBack (Args &&... args) |
reference | emplaceBack (Args &&... args) |
bool | empty () const const |
bool | empty () const const |
iterator | end () |
iterator | end () |
const_iterator | end () const const |
const_iterator | end () const const |
bool | endsWith (parameter_type value) const const |
bool | endsWith (parameter_type value) const const |
iterator | erase (const_iterator begin, const_iterator end) |
iterator | erase (const_iterator begin, const_iterator end) |
iterator | erase (const_iterator pos) |
iterator | erase (const_iterator pos) |
qsizetype | erase (QList< T > &list, const AT &t) |
qsizetype | erase (QList< T > &list, const AT &t) |
qsizetype | erase_if (QList< T > &list, Predicate pred) |
qsizetype | erase_if (QList< T > &list, Predicate pred) |
QList< T > & | fill (parameter_type value, qsizetype size) |
QList< T > & | fill (parameter_type value, qsizetype size) |
T & | first () |
T & | first () |
const T & | first () const const |
const T & | first () const const |
QList< T > | first (qsizetype n) const const |
QList< T > | first (qsizetype n) const const |
reference | front () |
reference | front () |
const_reference | front () const const |
const_reference | front () const const |
qsizetype | indexOf (const AT &value, qsizetype from) const const |
qsizetype | indexOf (const AT &value, qsizetype from) const const |
iterator | insert (const_iterator before, parameter_type value) |
iterator | insert (const_iterator before, parameter_type value) |
iterator | insert (const_iterator before, qsizetype count, parameter_type value) |
iterator | insert (const_iterator before, qsizetype count, parameter_type value) |
iterator | insert (const_iterator before, rvalue_ref value) |
iterator | insert (const_iterator before, rvalue_ref value) |
iterator | insert (qsizetype i, parameter_type value) |
iterator | insert (qsizetype i, parameter_type value) |
iterator | insert (qsizetype i, qsizetype count, parameter_type value) |
iterator | insert (qsizetype i, qsizetype count, parameter_type value) |
iterator | insert (qsizetype i, rvalue_ref value) |
iterator | insert (qsizetype i, rvalue_ref value) |
bool | isEmpty () const const |
bool | isEmpty () const const |
T & | last () |
T & | last () |
const T & | last () const const |
const T & | last () const const |
QList< T > | last (qsizetype n) const const |
QList< T > | last (qsizetype n) const const |
qsizetype | lastIndexOf (const AT &value, qsizetype from) const const |
qsizetype | lastIndexOf (const AT &value, qsizetype from) const const |
qsizetype | length () const const |
qsizetype | length () const const |
QList< T > | mid (qsizetype pos, qsizetype length) const const |
QList< T > | mid (qsizetype pos, qsizetype length) const const |
void | move (qsizetype from, qsizetype to) |
void | move (qsizetype from, qsizetype to) |
bool | operator!= (const QList< T > &other) const const |
bool | operator!= (const QList< T > &other) const const |
QList< T > | operator+ (const QList< T > &other) && |
QList< T > | operator+ (const QList< T > &other) && |
QList< T > | operator+ (const QList< T > &other) const &const |
QList< T > | operator+ (const QList< T > &other) const &const |
QList< T > | operator+ (QList< T > &&other) && |
QList< T > | operator+ (QList< T > &&other) && |
QList< T > | operator+ (QList< T > &&other) const &const |
QList< T > | operator+ (QList< T > &&other) const &const |
QList< T > & | operator+= (const QList< T > &other) |
QList< T > & | operator+= (const QList< T > &other) |
QList< T > & | operator+= (parameter_type value) |
QList< T > & | operator+= (parameter_type value) |
QList< T > & | operator+= (QList< T > &&other) |
QList< T > & | operator+= (QList< T > &&other) |
QList< T > & | operator+= (rvalue_ref value) |
QList< T > & | operator+= (rvalue_ref value) |
bool | operator< (const QList< T > &other) const const |
bool | operator< (const QList< T > &other) const const |
QList< T > & | operator<< (const QList< T > &other) |
QList< T > & | operator<< (const QList< T > &other) |
QList< T > & | operator<< (parameter_type value) |
QList< T > & | operator<< (parameter_type value) |
QDataStream & | operator<< (QDataStream &out, const QList< T > &list) |
QDataStream & | operator<< (QDataStream &out, const QList< T > &list) |
QList< T > & | operator<< (QList< T > &&other) |
QList< T > & | operator<< (QList< T > &&other) |
QList< T > & | operator<< (rvalue_ref value) |
QList< T > & | operator<< (rvalue_ref value) |
bool | operator<= (const QList< T > &other) const const |
bool | operator<= (const QList< T > &other) const const |
QList< T > & | operator= (const QList< T > &other) |
QList< T > & | operator= (const QList< T > &other) |
QList< T > & | operator= (QList< T > &&other) |
QList< T > & | operator= (QList< T > &&other) |
QList< T > & | operator= (std::initializer_list< T > args) |
QList< T > & | operator= (std::initializer_list< T > args) |
bool | operator== (const QList< T > &other) const const |
bool | operator== (const QList< T > &other) const const |
bool | operator> (const QList< T > &other) const const |
bool | operator> (const QList< T > &other) const const |
bool | operator>= (const QList< T > &other) const const |
bool | operator>= (const QList< T > &other) const const |
QDataStream & | operator>> (QDataStream &in, QList< T > &list) |
QDataStream & | operator>> (QDataStream &in, QList< T > &list) |
reference | operator[] (qsizetype i) |
reference | operator[] (qsizetype i) |
const_reference | operator[] (qsizetype i) const const |
const_reference | operator[] (qsizetype i) const const |
void | pop_back () |
void | pop_back () |
void | pop_front () |
void | pop_front () |
void | prepend (parameter_type value) |
void | prepend (parameter_type value) |
void | prepend (rvalue_ref value) |
void | prepend (rvalue_ref value) |
void | push_back (parameter_type value) |
void | push_back (parameter_type value) |
void | push_back (rvalue_ref value) |
void | push_back (rvalue_ref value) |
void | push_front (parameter_type value) |
void | push_front (parameter_type value) |
void | push_front (rvalue_ref value) |
void | push_front (rvalue_ref value) |
size_t | qHash (const QList< T > &key, size_t seed) |
size_t | qHash (const QList< T > &key, size_t seed) |
reverse_iterator | rbegin () |
reverse_iterator | rbegin () |
const_reverse_iterator | rbegin () const const |
const_reverse_iterator | rbegin () const const |
void | remove (qsizetype i, qsizetype n) |
void | remove (qsizetype i, qsizetype n) |
qsizetype | removeAll (const AT &t) |
qsizetype | removeAll (const AT &t) |
void | removeAt (qsizetype i) |
void | removeAt (qsizetype i) |
void | removeFirst () |
void | removeFirst () |
qsizetype | removeIf (Predicate pred) |
qsizetype | removeIf (Predicate pred) |
void | removeLast () |
void | removeLast () |
bool | removeOne (const AT &t) |
bool | removeOne (const AT &t) |
reverse_iterator | rend () |
reverse_iterator | rend () |
const_reverse_iterator | rend () const const |
const_reverse_iterator | rend () const const |
void | replace (qsizetype i, parameter_type value) |
void | replace (qsizetype i, parameter_type value) |
void | replace (qsizetype i, rvalue_ref value) |
void | replace (qsizetype i, rvalue_ref value) |
void | reserve (qsizetype size) |
void | reserve (qsizetype size) |
void | resize (qsizetype size) |
void | resize (qsizetype size) |
void | resize (qsizetype size, parameter_type c) |
void | resize (qsizetype size, parameter_type c) |
void | shrink_to_fit () |
void | shrink_to_fit () |
qsizetype | size () const const |
qsizetype | size () const const |
QList< T > | sliced (qsizetype pos) const const |
QList< T > | sliced (qsizetype pos) const const |
QList< T > | sliced (qsizetype pos, qsizetype n) const const |
QList< T > | sliced (qsizetype pos, qsizetype n) const const |
void | squeeze () |
void | squeeze () |
bool | startsWith (parameter_type value) const const |
bool | startsWith (parameter_type value) const const |
void | swap (QList< T > &other) |
void | swap (QList< T > &other) |
void | swapItemsAt (qsizetype i, qsizetype j) |
void | swapItemsAt (qsizetype i, qsizetype j) |
T | takeAt (qsizetype i) |
T | takeAt (qsizetype i) |
value_type | takeFirst () |
value_type | takeFirst () |
value_type | takeLast () |
value_type | takeLast () |
QList< T > | toList () const const |
QList< T > | toList () const const |
QList< T > | toVector () const const |
QList< T > | toVector () const const |
T | value (qsizetype i) const const |
T | value (qsizetype i) const const |
T | value (qsizetype i, parameter_type defaultValue) const const |
T | value (qsizetype i, parameter_type defaultValue) const const |
Static Public Member Functions | |
static bool | canDecode (const QMimeData *mimeData) |
static KBookmark::List | fromMimeData (const QMimeData *mimeData, QDomDocument &parentDocument) |
static QStringList | mimeDataTypes () |
![]() | |
QList< T > | fromList (const QList< T > &list) |
QList< T > | fromList (const QList< T > &list) |
QList< T > | fromVector (const QList< T > &list) |
QList< T > | fromVector (const QList< T > &list) |
Additional Inherited Members | |
![]() | |
typedef | const_pointer |
typedef | const_reference |
typedef | const_reverse_iterator |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | parameter_type |
typedef | pointer |
typedef | reference |
typedef | reverse_iterator |
typedef | rvalue_ref |
typedef | size_type |
typedef | value_type |
Detailed Description
KBookmark::List is a QList that contains bookmarks with a few convenience methods.
Definition at line 42 of file kbookmark.h.
Constructor & Destructor Documentation
◆ List()
KBookmark::List::List | ( | ) |
Definition at line 670 of file kbookmark.cpp.
Member Function Documentation
◆ canDecode()
|
static |
Return true if mimeData
contains bookmarks.
Definition at line 694 of file kbookmark.cpp.
◆ fromMimeData()
|
static |
Extract a list of bookmarks from the contents of mimeData
.
Decoding will fail if mimeData
does not contain any bookmarks.
- Parameters
-
mimeData the mime data to extract from; cannot be 0 parentDocument pass an empty QDomDocument here, it will be used as container for the bookmarks. You just need to make sure it stays alive longer (or just as long) as the returned bookmarks.
- Returns
- the list of bookmarks
- Since
- 4.3.2
Definition at line 704 of file kbookmark.cpp.
◆ mimeDataTypes()
|
static |
Return the list of mimeTypes that can be decoded by fromMimeData.
Definition at line 699 of file kbookmark.cpp.
◆ populateMimeData()
void KBookmark::List::populateMimeData | ( | QMimeData * | mimeData | ) | const |
Adds this list of bookmark into the given QMimeData.
- Parameters
-
mimeData the QMimeData instance used to drag or copy this bookmark
Definition at line 675 of file kbookmark.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:55:16 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.