KCalendarCore::ICalFormat
#include <icalformat.h>
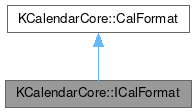
Additional Inherited Members | |
![]() | |
static const QString & | application () |
static QString | createUniqueId () |
static const QString & | productId () |
static void | setApplication (const QString &application, const QString &productID) |
![]() | |
void | setLoadedProductId (const QString &id) |
Detailed Description
iCalendar format implementation.
This class implements the iCalendar format. It provides methods for loading/saving/converting iCalendar format data into the internal representation as Calendar and Incidences.
- Warning
- When importing/loading to a Calendar, there is only duplicate check if those Incidences are loaded into the Calendar. If they are not loaded it will create duplicates.
Definition at line 44 of file icalformat.h.
Constructor & Destructor Documentation
◆ ICalFormat()
ICalFormat::ICalFormat | ( | ) |
Constructor a new iCalendar Format object.
Definition at line 54 of file icalformat.cpp.
◆ ~ICalFormat()
|
override |
Destructor.
Definition at line 59 of file icalformat.cpp.
Member Function Documentation
◆ createScheduleMessage()
QString ICalFormat::createScheduleMessage | ( | const IncidenceBase::Ptr & | incidence, |
iTIPMethod | method ) |
Creates a scheduling message string for an Incidence.
- Parameters
-
incidence is a pointer to an IncidenceBase object to be scheduled. method is a Scheduler::Method
- Returns
- a QString containing the message if successful; 0 otherwise.
Definition at line 402 of file icalformat.cpp.
◆ durationFromString()
Parses a string representation of a duration.
- Parameters
-
duration iCal representation of a duration.
- Since
- 5.95
Definition at line 391 of file icalformat.cpp.
◆ fromRawString()
|
overridevirtual |
Implements KCalendarCore::CalFormat.
Definition at line 174 of file icalformat.cpp.
◆ fromString() [1/3]
bool CalFormat::fromString | ( | const Calendar::Ptr & | calendar, |
const QString & | string ) |
Loads a calendar from a string.
- Returns
- true if successful; false otherwise.
- See also
- fromRawString(), toString().
- Since
- 5.97
Definition at line 77 of file calformat.cpp.
◆ fromString() [2/3]
Incidence::Ptr ICalFormat::fromString | ( | const QString & | string | ) |
Parses a string, returning the first iCal component as an Incidence.
- Parameters
-
string is a QString containing the data to be parsed.
- Returns
- non-zero pointer if the parsing was successful; 0 otherwise.
Definition at line 230 of file icalformat.cpp.
◆ fromString() [3/3]
bool ICalFormat::fromString | ( | RecurrenceRule * | rule, |
const QString & | string ) |
Parses a string and fills a RecurrenceRule object with the information.
- Parameters
-
rule is a pointer to a RecurrenceRule object. string is a QString containing the data to be parsed.
- Returns
- true if successful; false otherwise.
Definition at line 371 of file icalformat.cpp.
◆ load()
|
overridevirtual |
Implements KCalendarCore::CalFormat.
Definition at line 64 of file icalformat.cpp.
◆ parseFreeBusy()
FreeBusy::Ptr ICalFormat::parseFreeBusy | ( | const QString & | string | ) |
◆ parseScheduleMessage()
ScheduleMessage::Ptr ICalFormat::parseScheduleMessage | ( | const Calendar::Ptr & | calendar, |
const QString & | string ) |
Parses a Calendar scheduling message string into ScheduleMessage object.
- Parameters
-
calendar is a pointer to a Calendar object associated with the scheduling message. string is a QString containing the data to be parsed.
- Returns
- a pointer to a ScheduleMessage object if successful; 0 otherwise. The calling routine may later free the return memory.
Definition at line 482 of file icalformat.cpp.
◆ readIncidence()
Incidence::Ptr ICalFormat::readIncidence | ( | const QByteArray & | string | ) |
Parses a bytearray, returning the first iCal component as an Incidence, ignoring timezone information.
This function is significantly faster than fromString by avoiding the overhead of parsing timezone information. Timezones are instead solely interpreted by using system-timezones.
- Parameters
-
string is a utf8 QByteArray containing the data to be parsed.
- Returns
- non-zero pointer if the parsing was successful; 0 otherwise.
Definition at line 137 of file icalformat.cpp.
◆ save()
|
overridevirtual |
Implements KCalendarCore::CalFormat.
Definition at line 92 of file icalformat.cpp.
◆ setTimeZone()
void ICalFormat::setTimeZone | ( | const QTimeZone & | timeZone | ) |
Sets the iCalendar time zone.
- Parameters
-
timeZone is the time zone to set.
- See also
- timeZone().
Definition at line 608 of file icalformat.cpp.
◆ timeZone()
QTimeZone ICalFormat::timeZone | ( | ) | const |
Returns the iCalendar time zone.
- See also
- setTimeZone().
Definition at line 614 of file icalformat.cpp.
◆ timeZoneId()
QByteArray ICalFormat::timeZoneId | ( | ) | const |
Returns the timezone id string used by the iCalendar; an empty string if the iCalendar does not have a timezone.
Definition at line 620 of file icalformat.cpp.
◆ toICalString()
QString ICalFormat::toICalString | ( | const Incidence::Ptr & | incidence | ) |
Converts an Incidence to iCalendar formatted text.
- Parameters
-
incidence is a pointer to an Incidence object to be converted into iCal formatted text.
- Returns
- the QString will be Null if the conversion was unsuccessful.
Definition at line 307 of file icalformat.cpp.
◆ toRawString()
QByteArray ICalFormat::toRawString | ( | const Incidence::Ptr & | incidence | ) |
Converts an Incidence to a QByteArray.
- Parameters
-
incidence is a pointer to an Incidence object to be converted into a QByteArray.
- Returns
- the QString will be Null if the conversion was unsuccessful.
- Since
- 4.7
Definition at line 321 of file icalformat.cpp.
◆ toString() [1/4]
|
overridevirtual |
Implements KCalendarCore::CalFormat.
Definition at line 241 of file icalformat.cpp.
◆ toString() [2/4]
Converts a Duration to an iCal string.
- Parameters
-
duration a Duration object.
- Returns
- iCal formatted duration
- Since
- 5.95
Definition at line 362 of file icalformat.cpp.
◆ toString() [3/4]
QString ICalFormat::toString | ( | const Incidence::Ptr & | incidence | ) |
◆ toString() [4/4]
QString ICalFormat::toString | ( | RecurrenceRule * | rule | ) |
Converts a RecurrenceRule to a QString.
- Parameters
-
rule is a pointer to a RecurrenceRule object to be converted into a QString.
- Returns
- the QString will be Null if the conversion was unsuccessful.
Definition at line 353 of file icalformat.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 27 2024 11:52:22 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.