KCalendarCore::IncidenceBase
#include <incidencebase.h>
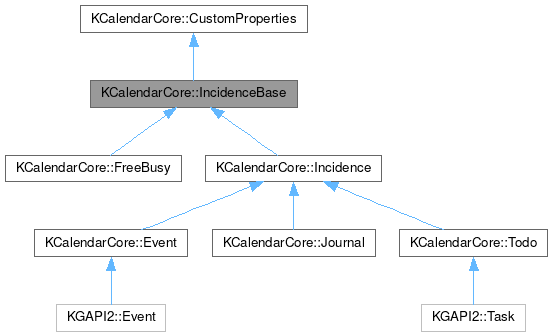
Classes | |
class | IncidenceObserver |
Public Types | |
enum | DateTimeRole { RoleAlarmStartOffset = 0 , RoleAlarmEndOffset , RoleSort , RoleCalendarHashing , RoleStartTimeZone , RoleEndTimeZone , RoleEndRecurrenceBase , RoleEnd , RoleDisplayEnd , RoleAlarm , RoleRecurrenceStart , RoleDisplayStart , RoleDnD } |
enum | Field { FieldDtStart , FieldDtEnd , FieldLastModified , FieldDescription , FieldSummary , FieldLocation , FieldCompleted , FieldPercentComplete , FieldDtDue , FieldCategories , FieldRelatedTo , FieldRecurrence , FieldAttachment , FieldSecrecy , FieldStatus , FieldTransparency , FieldResources , FieldPriority , FieldGeoLatitude , FieldGeoLongitude , FieldRecurrenceId , FieldAlarms , FieldSchedulingId , FieldAttendees , FieldOrganizer , FieldCreated , FieldRevision , FieldDuration , FieldContact , FieldComment , FieldUid , FieldUnknown , FieldUrl , FieldConferences , FieldColor } |
enum | IncidenceType { TypeEvent = 0 , TypeTodo , TypeJournal , TypeFreeBusy , TypeUnknown } |
typedef QSharedPointer< IncidenceBase > | Ptr |
Properties | |
bool | allDay |
QList< KCalendarCore::Attendee > | attendees |
QDateTime | dtStart |
QDateTime | lastModified |
KCalendarCore::Person | organizer |
QString | uid |
QUrl | url |
Static Public Member Functions | |
static quint32 | magicSerializationIdentifier () |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
Protected Types | |
enum | VirtualHook |
Protected Member Functions | |
IncidenceBase (const IncidenceBase &)=delete | |
KCALENDARCORE_NO_EXPORT | IncidenceBase (const IncidenceBase &ib, IncidenceBasePrivate *p) |
virtual IncidenceBase & | assign (const IncidenceBase &other) |
void | customPropertyUpdate () override |
void | customPropertyUpdated () override |
virtual void | deserialize (QDataStream &in) |
virtual bool | equals (const IncidenceBase &incidenceBase) const |
virtual void | serialize (QDataStream &out) const |
void | setFieldDirty (IncidenceBase::Field field) |
virtual void | virtual_hook (VirtualHook id, void *data)=0 |
![]() |
Protected Attributes | |
IncidenceBasePrivate *const | d_ptr |
bool | mReadOnly |
Detailed Description
An abstract class that provides a common base for all calendar incidence classes.
define: organizer (person) define: uid (same as the attendee uid?)
Several properties are not allowed for VFREEBUSY objects (see rfc:2445), so they are not in IncidenceBase. The hierarchy is:
So IncidenceBase contains all properties that are common to all classes, and Incidence contains all additional properties that are common to Events, Todos and Journals, but are not allowed for FreeBusy entries.
Definition at line 98 of file incidencebase.h.
Member Typedef Documentation
◆ Ptr
A shared pointer to an IncidenceBase.
Definition at line 113 of file incidencebase.h.
Member Enumeration Documentation
◆ DateTimeRole
The different types of incidence date/times roles.
- See also
- dateTime()
Enumerator | |
---|---|
RoleAlarmStartOffset | Role for an incidence alarm's starting offset date/time. |
RoleAlarmEndOffset | Role for an incidence alarm's ending offset date/time. |
RoleSort | Role for an incidence's date/time used when sorting. |
RoleCalendarHashing | Role for looking up an incidence in a Calendar. |
RoleStartTimeZone | Role for determining an incidence's starting timezone. |
RoleEndTimeZone | Role for determining an incidence's ending timezone. |
RoleEnd | Role for determining an incidence's dtEnd, will return an invalid QDateTime if the incidence does not support dtEnd. |
RoleDisplayEnd | Role used for display purposes, represents the end boundary if an incidence supports dtEnd. |
RoleAlarm | Role for determining the date/time of the first alarm. Returns invalid time if the incidence doesn't have any alarm |
RoleRecurrenceStart | Role for determining the start of the recurrence. Currently that's DTSTART for an event and DTDUE for a to-do. (NOTE: If the incidence is a to-do, recurrence should be calculated having DTSTART for a reference, not DT-DUE. This is one place KCalendarCore isn't compliant with RFC2445) |
RoleDisplayStart | Role for display purposes, represents the start boundary of an incidence. To-dos return dtDue here, for historical reasons |
RoleDnD | Role for determining new start and end dates after a DnD. |
Definition at line 131 of file incidencebase.h.
◆ Field
The different types of incidence fields.
Definition at line 158 of file incidencebase.h.
◆ IncidenceType
The different types of incidences, per RFC2445.
Enumerator | |
---|---|
TypeEvent | Type is an event. |
TypeTodo | Type is a to-do. |
TypeJournal | Type is a journal. |
TypeFreeBusy | Type is a free/busy. |
TypeUnknown | Type unknown. |
Definition at line 119 of file incidencebase.h.
◆ VirtualHook
|
protected |
Definition at line 735 of file incidencebase.h.
Property Documentation
◆ allDay
|
readwrite |
Definition at line 104 of file incidencebase.h.
◆ attendees
|
read |
Definition at line 106 of file incidencebase.h.
◆ dtStart
|
readwrite |
Definition at line 103 of file incidencebase.h.
◆ lastModified
|
readwrite |
Definition at line 102 of file incidencebase.h.
◆ organizer
|
readwrite |
Definition at line 105 of file incidencebase.h.
◆ uid
|
readwrite |
Definition at line 101 of file incidencebase.h.
◆ url
|
readwrite |
Definition at line 107 of file incidencebase.h.
Constructor & Destructor Documentation
◆ IncidenceBase() [1/2]
|
explicit |
Constructs an empty IncidenceBase.
- Parameters
-
p (non-null) a Private data object provided by the instantiated class (Event, Todo, Journal, FreeBusy). It takes ownership of the object.
Definition at line 61 of file incidencebase.cpp.
◆ ~IncidenceBase()
|
override |
Destroys the IncidenceBase.
Definition at line 75 of file incidencebase.cpp.
◆ IncidenceBase() [2/2]
|
protected |
Constructs an IncidenceBase as a copy of another IncidenceBase object.
- Parameters
-
ib is the IncidenceBase to copy. p (non-null) a Private data object provided by the instantiated class (Event, Todo, Journal, FreeBusy). It takes ownership of the object.
Definition at line 68 of file incidencebase.cpp.
Member Function Documentation
◆ accept()
|
virtual |
Accept IncidenceVisitor.
A class taking part in the visitor mechanism has to provide this implementation:
bool accept(Visitor &v) { return v.visit(this); }
- Parameters
-
v is a reference to a Visitor object. incidence is a valid IncidenceBase object for visiting.
Definition at line 163 of file incidencebase.cpp.
◆ addAttendee()
void IncidenceBase::addAttendee | ( | const Attendee & | attendee, |
bool | doUpdate = true ) |
Add Attendee to this incidence.
- Parameters
-
attendee the attendee to add doUpdate If true the Observers are notified, if false they are not.
Definition at line 364 of file incidencebase.cpp.
◆ addComment()
void IncidenceBase::addComment | ( | const QString & | comment | ) |
Adds a comment to the incidence.
Does not add a linefeed character; simply appends the text as specified.
- Parameters
-
comment is the QString containing the comment to add.
- See also
- removeComment().
Definition at line 294 of file incidencebase.cpp.
◆ addContact()
void IncidenceBase::addContact | ( | const QString & | contact | ) |
Adds a contact to thieincidence.
Does not add a linefeed character; simply appends the text as specified.
- Parameters
-
contact is the QString containing the contact to add.
- See also
- removeContact().
Definition at line 328 of file incidencebase.cpp.
◆ allDay()
bool IncidenceBase::allDay | ( | ) | const |
Returns true or false depending on whether the incidence is all-day.
i.e. has a date but no time attached to it.
- See also
- setAllDay()
Definition at line 267 of file incidencebase.cpp.
◆ assign()
|
protectedvirtual |
Provides polymorfic assignment.
- Parameters
-
other is the IncidenceBase to assign.
Reimplemented in KCalendarCore::Event, KCalendarCore::FreeBusy, KCalendarCore::Incidence, KCalendarCore::Journal, and KCalendarCore::Todo.
Definition at line 92 of file incidencebase.cpp.
◆ attendeeByMail()
Returns the attendee with the specified email address.
- Parameters
-
email is a QString containing an email address of the form "FirstName LastName <emailaddress>".
- See also
- attendeeByMails(), attendeesByUid().
Definition at line 427 of file incidencebase.cpp.
◆ attendeeByMails()
Attendee IncidenceBase::attendeeByMails | ( | const QStringList & | emails, |
const QString & | email = QString() ) const |
Returns the first incidence attendee with one of the specified email addresses.
- Parameters
-
emails is a list of QStrings containing email addresses of the form "FirstName LastName <emailaddress>". email is a QString containing a single email address to search in addition to the list specified in emails
.
- See also
- attendeeByMail(), attendeesByUid().
Definition at line 436 of file incidencebase.cpp.
◆ attendeeByUid()
Returns the incidence attendee with the specified attendee UID.
- Parameters
-
uid is a QString containing an attendee UID.
- See also
- attendeeByMail(), attendeeByMails().
Definition at line 450 of file incidencebase.cpp.
◆ attendeeCount()
int IncidenceBase::attendeeCount | ( | ) | const |
Returns the number of incidence attendees.
Definition at line 387 of file incidencebase.cpp.
◆ attendees()
Attendee::List IncidenceBase::attendees | ( | ) | const |
Returns a list of incidence attendees.
All pointers in the list are valid.
Definition at line 382 of file incidencebase.cpp.
◆ clearAttendees()
void IncidenceBase::clearAttendees | ( | ) |
Removes all attendees from the incidence.
Definition at line 416 of file incidencebase.cpp.
◆ clearComments()
void IncidenceBase::clearComments | ( | ) |
Deletes all incidence comments.
Definition at line 315 of file incidencebase.cpp.
◆ clearContacts()
void IncidenceBase::clearContacts | ( | ) |
Deletes all incidence contacts.
Definition at line 351 of file incidencebase.cpp.
◆ comments()
QStringList IncidenceBase::comments | ( | ) | const |
Returns all incidence comments as a list of strings.
Definition at line 323 of file incidencebase.cpp.
◆ contacts()
QStringList IncidenceBase::contacts | ( | ) | const |
Returns all incidence contacts as a list of strings.
Definition at line 359 of file incidencebase.cpp.
◆ customPropertyUpdate()
|
overrideprotectedvirtual |
CustomProperties::customPropertyUpdate()
Reimplemented from KCalendarCore::CustomProperties.
Definition at line 546 of file incidencebase.cpp.
◆ customPropertyUpdated()
|
overrideprotectedvirtual |
CustomProperties::customPropertyUpdated()
Reimplemented from KCalendarCore::CustomProperties.
Definition at line 551 of file incidencebase.cpp.
◆ dateTime()
|
pure virtual |
Returns a date/time corresponding to the specified DateTimeRole.
- Parameters
-
role is a DateTimeRole.
Implemented in KCalendarCore::Event, KCalendarCore::FreeBusy, KCalendarCore::Journal, and KCalendarCore::Todo.
◆ deserialize()
|
protectedvirtual |
Sub-type specific deserialization.
Reimplemented in KCalendarCore::Incidence.
Definition at line 591 of file incidencebase.cpp.
◆ dirtyFields()
QSet< IncidenceBase::Field > IncidenceBase::dirtyFields | ( | ) | const |
Returns a QSet with all Fields that were changed since the incidence was created or resetDirtyFields() was called.
- See also
- resetDirtyFields()
Definition at line 566 of file incidencebase.cpp.
◆ dtStart()
|
virtual |
Returns an incidence's starting date/time as a QDateTime.
- See also
- setDtStart().
Reimplemented in KCalendarCore::Todo.
Definition at line 262 of file incidencebase.cpp.
◆ duration()
Duration IncidenceBase::duration | ( | ) | const |
Returns the length of the incidence duration.
- See also
- setDuration()
Definition at line 467 of file incidencebase.cpp.
◆ endUpdates()
void IncidenceBase::endUpdates | ( | ) |
Call this when a group of updates is complete, to notify observers that the instance has changed.
This should be called in conjunction with startUpdates().
Definition at line 536 of file incidencebase.cpp.
◆ equals()
|
protectedvirtual |
Provides polymorfic comparison for equality.
Only called by IncidenceBase::operator==() which guarantees that incidenceBase
is of the right type.
- Parameters
-
incidenceBase is the IncidenceBase to compare against.
- Returns
- true if the incidences are equal; false otherwise.
Reimplemented in KCalendarCore::Event, KCalendarCore::FreeBusy, KCalendarCore::Incidence, KCalendarCore::Journal, and KCalendarCore::Todo.
Definition at line 117 of file incidencebase.cpp.
◆ hasDuration()
bool IncidenceBase::hasDuration | ( | ) | const |
Returns true if the incidence has a duration; false otherwise.
- See also
- setHasDuration()
Definition at line 477 of file incidencebase.cpp.
◆ isReadOnly()
bool IncidenceBase::isReadOnly | ( | ) | const |
Returns true the object is read-only; false otherwise.
- See also
- setReadOnly()
Definition at line 241 of file incidencebase.cpp.
◆ lastModified()
QDateTime IncidenceBase::lastModified | ( | ) | const |
Returns the time the incidence was last modified.
- See also
- setLastModified()
Definition at line 201 of file incidencebase.cpp.
◆ magicSerializationIdentifier()
|
static |
Constant that identifies KCalendarCore data in a binary stream.
static
- Since
- 4.12
Definition at line 597 of file incidencebase.cpp.
◆ mimeType()
|
pure virtual |
Returns the Akonadi specific sub MIME type of a KCalendarCore::IncidenceBase item, e.g.
getting "application/x-vnd.akonadi.calendar.event" for a KCalendarCore::Event.
Implemented in KCalendarCore::Event, KCalendarCore::FreeBusy, KCalendarCore::Journal, and KCalendarCore::Todo.
◆ operator!=()
bool IncidenceBase::operator!= | ( | const IncidenceBase & | ib | ) | const |
Compares this with IncidenceBase ib
for inequality.
- Parameters
-
ib is the IncidenceBase to compare against.
- Returns
- true if the incidences are /not/ equal; false otherwise.
Definition at line 112 of file incidencebase.cpp.
◆ operator=()
IncidenceBase & IncidenceBase::operator= | ( | const IncidenceBase & | other | ) |
Assignment operator.
All data belonging to derived classes are also copied.
- See also
- assign(). The caller guarantees that both types match.
Dirty field FieldUnknown will be set.
- Parameters
-
other is the IncidenceBase to assign.
Definition at line 80 of file incidencebase.cpp.
◆ operator==()
bool IncidenceBase::operator== | ( | const IncidenceBase & | ib | ) | const |
Compares this with IncidenceBase ib
for equality.
All data belonging to derived classes are also compared.
- See also
- equals().
- Parameters
-
ib is the IncidenceBase to compare against.
- Returns
- true if the incidences are equal; false otherwise.
Definition at line 102 of file incidencebase.cpp.
◆ organizer()
Person IncidenceBase::organizer | ( | ) | const |
Returns the Person associated with this incidence.
If no Person was set through setOrganizer(), a default Person() is returned.
Definition at line 231 of file incidencebase.cpp.
◆ recurrenceId()
|
virtual |
Returns the incidence recurrenceId.
- Returns
- incidences recurrenceId value
- See also
- setRecurrenceId().
Reimplemented in KCalendarCore::Incidence.
Definition at line 556 of file incidencebase.cpp.
◆ registerObserver()
void IncidenceBase::registerObserver | ( | IncidenceBase::IncidenceObserver * | observer | ) |
Register observer.
The observer is notified when the observed object changes.
- Parameters
-
observer is a pointer to an IncidenceObserver object that will be watching this incidence.
- See also
- unRegisterObserver()
Definition at line 495 of file incidencebase.cpp.
◆ removeComment()
bool IncidenceBase::removeComment | ( | const QString & | comment | ) |
Removes a comment from the incidence.
Removes the first comment whose string is an exact match for the specified string in comment
.
- Parameters
-
comment is the QString containing the comment to remove.
- Returns
- true if match found, false otherwise.
- See also
- addComment().
Definition at line 302 of file incidencebase.cpp.
◆ removeContact()
bool IncidenceBase::removeContact | ( | const QString & | contact | ) |
Removes a contact from the incidence.
Removes the first contact whose string is an exact match for the specified string in contact
.
- Parameters
-
contact is the QString containing the contact to remove.
- Returns
- true if match found, false otherwise.
- See also
- addContact().
Definition at line 338 of file incidencebase.cpp.
◆ resetDirtyFields()
void IncidenceBase::resetDirtyFields | ( | ) |
◆ serialize()
|
protectedvirtual |
Sub-type specific serialization.
Reimplemented in KCalendarCore::Incidence.
Definition at line 586 of file incidencebase.cpp.
◆ setAllDay()
|
virtual |
Sets whether the incidence is all-day, i.e.
has a date but no time attached to it.
- Parameters
-
allDay sets whether the incidence is all-day.
- See also
- allDay()
Reimplemented in KCalendarCore::Event, KCalendarCore::Incidence, and KCalendarCore::Todo.
Definition at line 272 of file incidencebase.cpp.
◆ setAttendees()
void IncidenceBase::setAttendees | ( | const Attendee::List & | attendees, |
bool | doUpdate = true ) |
Set the attendees of this incidence.
This replaces all previously set attendees, unlike addAttendee.
- Parameters
-
attendees A list of attendees. doUpdate If true the Observers are notified, if false they are not.
Definition at line 392 of file incidencebase.cpp.
◆ setDateTime()
|
pure virtual |
Sets the date/time corresponding to the specified DateTimeRole.
- Parameters
-
dateTime is QDateTime value to set. role is a DateTimeRole.
Implemented in KCalendarCore::Event, KCalendarCore::FreeBusy, KCalendarCore::Journal, and KCalendarCore::Todo.
◆ setDirtyFields()
void IncidenceBase::setDirtyFields | ( | const QSet< IncidenceBase::Field > & | dirtyFields | ) |
Sets which fields are dirty.
- See also
- dirtyFields()
- Since
- 4.8
Definition at line 581 of file incidencebase.cpp.
◆ setDtStart()
|
virtual |
Sets the incidence's starting date/time with a QDateTime.
- Parameters
-
dtStart is the incidence start date/time.
- See also
- dtStart().
Reimplemented in KCalendarCore::Event, KCalendarCore::FreeBusy, and KCalendarCore::Incidence.
Definition at line 246 of file incidencebase.cpp.
◆ setDuration()
|
virtual |
Sets the incidence duration.
- Parameters
-
duration the incidence duration
- See also
- duration()
Reimplemented in KCalendarCore::Event.
Definition at line 458 of file incidencebase.cpp.
◆ setFieldDirty()
|
protected |
Marks Field field
as dirty.
- Parameters
-
field is the Field type to mark as dirty.
- See also
- dirtyFields()
Definition at line 571 of file incidencebase.cpp.
◆ setHasDuration()
void IncidenceBase::setHasDuration | ( | bool | hasDuration | ) |
Sets if the incidence has a duration.
- Parameters
-
hasDuration true if the incidence has a duration; false otherwise.
- See also
- hasDuration()
Definition at line 472 of file incidencebase.cpp.
◆ setLastModified()
|
virtual |
Sets the time the incidence was last modified to lm
.
It is stored as a UTC date/time.
- Parameters
-
lm is the QDateTime when the incidence was last modified.
- See also
- lastModified()
Reimplemented in KCalendarCore::Incidence.
Definition at line 185 of file incidencebase.cpp.
◆ setOrganizer() [1/2]
void IncidenceBase::setOrganizer | ( | const Person & | organizer | ) |
Sets the organizer for the incidence.
- Parameters
-
organizer is a non-null Person to use as the incidence organizer.
- See also
- organizer(), setOrganizer(const QString &)
Definition at line 206 of file incidencebase.cpp.
◆ setOrganizer() [2/2]
void IncidenceBase::setOrganizer | ( | const QString & | organizer | ) |
Sets the incidence organizer to any string organizer
.
- Parameters
-
organizer is a string to use as the incidence organizer.
- See also
- organizer(), setOrganizer(const Person &)
Definition at line 219 of file incidencebase.cpp.
◆ setReadOnly()
|
virtual |
Sets readonly status.
- Parameters
-
readOnly if set, the incidence is read-only; else the incidence can be modified.
- See also
- isReadOnly().
Reimplemented in KCalendarCore::Incidence.
Definition at line 236 of file incidencebase.cpp.
◆ setUid()
void IncidenceBase::setUid | ( | const QString & | uid | ) |
Sets the unique id for the incidence to uid
.
- Parameters
-
uid is the string containing the incidence uid.
- See also
- uid()
Definition at line 170 of file incidencebase.cpp.
◆ setUrl()
void IncidenceBase::setUrl | ( | const QUrl & | url | ) |
Sets the incidences url.
This property can be used to point to a more dynamic rendition of the incidence. I.e. a website related to the incidence.
- Parameters
-
url of the incience.
- See also
- url()
- Since
- 4.12
Definition at line 482 of file incidencebase.cpp.
◆ shiftTimes()
Shift the times of the incidence so that they appear at the same clock time as before but in a new time zone.
The shift is done from a viewing time zone rather than from the actual incidence time zone.
For example, shifting an incidence whose start time is 09:00 America/New York, using an old viewing time zone (oldSpec
) of Europe/London, to a new time zone (newSpec
) of Europe/Paris, will result in the time being shifted from 14:00 (which is the London time of the incidence start) to 14:00 Paris time.
- Parameters
-
oldZone the time zone which provides the clock times newZone the new time zone
Reimplemented in KCalendarCore::Event, KCalendarCore::FreeBusy, KCalendarCore::Incidence, and KCalendarCore::Todo.
Definition at line 285 of file incidencebase.cpp.
◆ startUpdates()
void IncidenceBase::startUpdates | ( | ) |
Call this when a group of updates is going to be made.
This suppresses change notifications until endUpdates() is called, at which point updated() will automatically be called.
Definition at line 530 of file incidencebase.cpp.
◆ type()
|
pure virtual |
Returns the incidence type.
Implemented in KCalendarCore::Event, KCalendarCore::FreeBusy, KCalendarCore::Journal, and KCalendarCore::Todo.
◆ typeStr()
|
pure virtual |
Prints the type of incidence as a string.
Implemented in KCalendarCore::Event, KCalendarCore::FreeBusy, KCalendarCore::Journal, and KCalendarCore::Todo.
◆ uid()
QString IncidenceBase::uid | ( | ) | const |
Returns the unique id (uid) for the incidence.
- See also
- setUid()
Definition at line 180 of file incidencebase.cpp.
◆ unRegisterObserver()
void IncidenceBase::unRegisterObserver | ( | IncidenceBase::IncidenceObserver * | observer | ) |
Unregister observer.
It isn't notified anymore about changes.
- Parameters
-
observer is a pointer to an IncidenceObserver object that will be watching this incidence.
- See also
- registerObserver().
Definition at line 502 of file incidencebase.cpp.
◆ update()
void IncidenceBase::update | ( | ) |
Call this to notify the observers after the IncidenceBase object will be changed.
Definition at line 507 of file incidencebase.cpp.
◆ updated()
void IncidenceBase::updated | ( | ) |
Call this to notify the observers after the IncidenceBase object has changed.
Definition at line 518 of file incidencebase.cpp.
◆ uri()
QUrl IncidenceBase::uri | ( | ) | const |
Returns the uri for the incidence, of form urn:x-ical:<uid>
Definition at line 576 of file incidencebase.cpp.
◆ url()
QUrl IncidenceBase::url | ( | ) | const |
Returns the url.
- Returns
- incidences url value
- See also
- setUrl()
- Since
- 4.12
Definition at line 490 of file incidencebase.cpp.
◆ virtual_hook()
|
protectedpure virtual |
Standard trick to add virtuals later.
- Parameters
-
id is any integer unique to this class which we will use to identify the method to be called. data is a pointer to some glob of data, typically a struct.
Implemented in KCalendarCore::Event, KCalendarCore::FreeBusy, KCalendarCore::Journal, and KCalendarCore::Todo.
Member Data Documentation
◆ d_ptr
|
protected |
Definition at line 754 of file incidencebase.h.
◆ mReadOnly
|
protected |
Identifies a read-only incidence.
Definition at line 749 of file incidencebase.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 20 2024 11:54:13 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.