KCalendarCore::Todo
#include <todo.h>
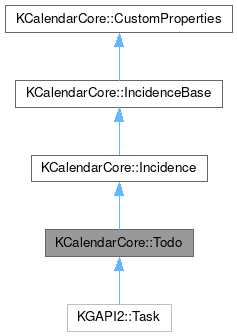
Public Member Functions | |
Todo () | |
Todo (const Incidence &other) | |
Todo (const Todo &other) | |
~Todo () override | |
Todo * | clone () const override |
QDateTime | completed () const |
QDateTime | dateTime (DateTimeRole role) const override |
QDateTime | dtDue (bool first=false) const |
QDateTime | dtRecurrence () const |
QDateTime | dtStart () const override |
QDateTime | dtStart (bool first) const |
bool | hasCompletedDate () const |
bool | hasDueDate () const |
bool | hasStartDate () const |
QLatin1String | iconName (const QDateTime &recurrenceId={}) const override |
bool | isCompleted () const |
bool | isInProgress (bool first) const |
bool | isNotStarted (bool first) const |
bool | isOpenEnded () const |
bool | isOverdue () const |
QLatin1String | mimeType () const override |
int | percentComplete () const |
bool | recursOn (const QDate &date, const QTimeZone &timeZone) const override |
void | setAllDay (bool allDay) override |
void | setCompleted (bool completed) |
void | setCompleted (const QDateTime &completeDate) |
void | setDateTime (const QDateTime &dateTime, DateTimeRole role) override |
void | setDtDue (const QDateTime &dtDue, bool first=false) |
void | setDtRecurrence (const QDateTime &dt) |
void | setPercentComplete (int percent) |
void | shiftTimes (const QTimeZone &oldZone, const QTimeZone &newZone) override |
bool | supportsGroupwareCommunication () const override |
IncidenceType | type () const override |
QByteArray | typeStr () const override |
![]() | |
Incidence (IncidencePrivate *p) | |
~Incidence () override | |
void | addAlarm (const Alarm::Ptr &alarm) |
void | addAttachment (const Attachment &attachment) |
void | addConference (const Conference &conference) |
Alarm::List | alarms () const |
QString | altDescription () const |
Attachment::List | attachments () const |
Attachment::List | attachments (const QString &mime) const |
QStringList | categories () const |
QString | categoriesStr () const |
void | clearAlarms () |
void | clearAttachments () |
void | clearConferences () |
void | clearRecurrence () |
QString | color () const |
Conference::List | conferences () const |
QDateTime | created () const |
QString | customStatus () const |
void | deleteAttachments (const QString &mime) |
QString | description () const |
bool | descriptionIsRich () const |
virtual QDateTime | endDateForStart (const QDateTime &startDt) const |
float | geoLatitude () const |
float | geoLongitude () const |
bool | hasAltDescription () const |
bool | hasEnabledAlarms () const |
bool | hasGeo () const |
bool | hasRecurrenceId () const |
QString | instanceIdentifier () const |
bool | localOnly () const |
QString | location () const |
bool | locationIsRich () const |
Alarm::Ptr | newAlarm () |
int | priority () const |
void | recreate () |
Recurrence * | recurrence () const |
QDateTime | recurrenceId () const override |
ushort | recurrenceType () const |
void | recurrenceUpdated (Recurrence *recurrence) override |
bool | recurs () const |
bool | recursAt (const QDateTime &dt) const |
QString | relatedTo (RelType relType=RelTypeParent) const |
void | removeAlarm (const Alarm::Ptr &alarm) |
QStringList | resources () const |
int | revision () const |
QString | richDescription () const |
QString | richLocation () const |
QString | richSummary () const |
QString | schedulingID () const |
Secrecy | secrecy () const |
void | setAltDescription (const QString &altdescription) |
void | setCategories (const QString &catStr) |
void | setCategories (const QStringList &categories) |
void | setColor (const QString &colorName) |
void | setConferences (const Conference::List &conferences) |
void | setCreated (const QDateTime &dt) |
void | setCustomStatus (const QString &status) |
void | setDescription (const QString &description) |
void | setDescription (const QString &description, bool isRich) |
void | setDtStart (const QDateTime &dt) override |
void | setGeoLatitude (float geolatitude) |
void | setGeoLongitude (float geolongitude) |
void | setLastModified (const QDateTime &lm) override |
void | setLocalOnly (bool localonly) |
void | setLocation (const QString &location) |
void | setLocation (const QString &location, bool isRich) |
void | setPriority (int priority) |
void | setReadOnly (bool readonly) override |
void | setRecurrenceId (const QDateTime &recurrenceId) |
void | setRelatedTo (const QString &uid, RelType relType=RelTypeParent) |
void | setResources (const QStringList &resources) |
void | setRevision (int rev) |
void | setSchedulingID (const QString &sid, const QString &uid=QString()) |
void | setSecrecy (Secrecy secrecy) |
void | setStatus (Status status) |
void | setSummary (const QString &summary) |
void | setSummary (const QString &summary, bool isRich) |
void | setThisAndFuture (bool thisAndFuture) |
virtual QList< QDateTime > | startDateTimesForDate (const QDate &date, const QTimeZone &timeZone) const |
virtual QList< QDateTime > | startDateTimesForDateTime (const QDateTime &datetime) const |
Status | status () const |
QString | summary () const |
bool | summaryIsRich () const |
bool | thisAndFuture () const |
![]() | |
KCALENDARCORE_NO_EXPORT | IncidenceBase (IncidenceBasePrivate *p) |
~IncidenceBase () override | |
void | addAttendee (const Attendee &attendee, bool doUpdate=true) |
void | addComment (const QString &comment) |
void | addContact (const QString &contact) |
bool | allDay () const |
Attendee | attendeeByMail (const QString &email) const |
Attendee | attendeeByMails (const QStringList &emails, const QString &email=QString()) const |
Attendee | attendeeByUid (const QString &uid) const |
int | attendeeCount () const |
Attendee::List | attendees () const |
void | clearAttendees () |
void | clearComments () |
void | clearContacts () |
QStringList | comments () const |
QStringList | contacts () const |
QSet< IncidenceBase::Field > | dirtyFields () const |
Duration | duration () const |
void | endUpdates () |
bool | hasDuration () const |
bool | isReadOnly () const |
QDateTime | lastModified () const |
bool | operator!= (const IncidenceBase &ib) const |
IncidenceBase & | operator= (const IncidenceBase &other) |
bool | operator== (const IncidenceBase &ib) const |
Person | organizer () const |
void | registerObserver (IncidenceObserver *observer) |
bool | removeComment (const QString &comment) |
bool | removeContact (const QString &contact) |
void | resetDirtyFields () |
void | setAttendees (const Attendee::List &attendees, bool doUpdate=true) |
void | setDirtyFields (const QSet< IncidenceBase::Field > &) |
virtual void | setDuration (const Duration &duration) |
void | setHasDuration (bool hasDuration) |
void | setOrganizer (const Person &organizer) |
void | setOrganizer (const QString &organizer) |
void | setUid (const QString &uid) |
void | setUrl (const QUrl &url) |
void | startUpdates () |
QString | uid () const |
void | unRegisterObserver (IncidenceObserver *observer) |
void | update () |
void | updated () |
QUrl | uri () const |
QUrl | url () const |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &other) | |
virtual | ~CustomProperties () |
QMap< QByteArray, QString > | customProperties () const |
QString | customProperty (const QByteArray &app, const QByteArray &key) const |
QString | nonKDECustomProperty (const QByteArray &name) const |
QString | nonKDECustomPropertyParameters (const QByteArray &name) const |
CustomProperties & | operator= (const CustomProperties &other) |
bool | operator== (const CustomProperties &properties) const |
void | removeCustomProperty (const QByteArray &app, const QByteArray &key) |
void | removeNonKDECustomProperty (const QByteArray &name) |
void | setCustomProperties (const QMap< QByteArray, QString > &properties) |
void | setCustomProperty (const QByteArray &app, const QByteArray &key, const QString &value) |
void | setNonKDECustomProperty (const QByteArray &name, const QString &value, const QString ¶meters=QString()) |
Static Public Member Functions | |
static QLatin1String | todoMimeType () |
![]() | |
static QStringList | mimeTypes () |
![]() | |
static quint32 | magicSerializationIdentifier () |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
Protected Member Functions | |
IncidenceBase & | assign (const IncidenceBase &other) override |
bool | equals (const IncidenceBase &todo) const override |
void | virtual_hook (VirtualHook id, void *data) override |
![]() | |
Incidence (const Incidence &)=delete | |
Incidence (const Incidence &other, IncidencePrivate *p) | |
![]() | |
IncidenceBase (const IncidenceBase &)=delete | |
KCALENDARCORE_NO_EXPORT | IncidenceBase (const IncidenceBase &ib, IncidenceBasePrivate *p) |
void | customPropertyUpdate () override |
void | customPropertyUpdated () override |
void | setFieldDirty (IncidenceBase::Field field) |
Additional Inherited Members | |
![]() | |
QList< KCalendarCore::Attachment > | attachments |
QStringList | categories |
QList< KCalendarCore::Conference > | conferences |
QDateTime | created |
QString | description |
float | geoLatitude |
float | geoLongitude |
bool | hasGeo |
QString | location |
int | priority |
KCalendarCore::Incidence::Secrecy | secrecy |
KCalendarCore::Incidence::Status | status |
QString | summary |
![]() | |
bool | allDay |
QList< KCalendarCore::Attendee > | attendees |
QDateTime | dtStart |
QDateTime | lastModified |
KCalendarCore::Person | organizer |
QString | uid |
QUrl | url |
![]() | |
enum | VirtualHook |
![]() | |
IncidenceBasePrivate *const | d_ptr |
bool | mReadOnly |
Detailed Description
Member Typedef Documentation
◆ List
◆ Ptr
typedef QSharedPointer<Todo> KCalendarCore::Todo::Ptr |
Constructor & Destructor Documentation
◆ Todo() [1/3]
KCalendarCore::Todo::Todo | ( | ) |
Constructs an empty to-do.
◆ Todo() [2/3]
KCalendarCore::Todo::Todo | ( | const Todo & | other | ) |
Copy constructor.
- Parameters
-
other is the to-do to copy.
◆ Todo() [3/3]
KCalendarCore::Todo::Todo | ( | const Incidence & | other | ) |
Costructs a todo out of an incidence This constructs allows to make it easy to create a todo from an event.
- Parameters
-
other is the incidence to copy.
- Since
- 4.14
◆ ~Todo()
|
override |
Destroys a to-do.
Member Function Documentation
◆ assign()
|
overrideprotectedvirtual |
Provides polymorfic assignment.
- Parameters
-
other is the IncidenceBase to assign.
Reimplemented from KCalendarCore::Incidence.
◆ clone()
|
overridevirtual |
Returns an exact copy of this todo.
The returned object is owned by the caller.
- Returns
- A pointer to a Todo containing an exact copy of this object.
Implements KCalendarCore::Incidence.
◆ completed()
QDateTime Todo::completed | ( | ) | const |
Returns the to-do was completion datetime.
- Returns
- A QDateTime for the completion datetime of the to-do.
- See also
- hasCompletedDate()
◆ dateTime()
|
overridevirtual |
Returns a date/time corresponding to the specified DateTimeRole.
- Parameters
-
role is a DateTimeRole.
Implements KCalendarCore::IncidenceBase.
◆ dtDue()
QDateTime Todo::dtDue | ( | bool | first = false | ) | const |
Returns the todo due datetime.
- Parameters
-
first If true and the todo recurs, the due datetime of the first occurrence will be returned. If false and recurrent, the datetime of the current occurrence will be returned. If non-recurrent, the normal due datetime will be returned.
- Returns
- A QDateTime containing the todo due datetime.
◆ dtRecurrence()
QDateTime Todo::dtRecurrence | ( | ) | const |
Returns an identifier for the earliest uncompleted occurrence of a recurring Todo.
- Note
- Do not rely on the returned value to determine whether the Todo is completed; use
isCompleted()
instead.
◆ dtStart() [1/2]
|
overridevirtual |
Reimplemented from KCalendarCore::IncidenceBase.
◆ dtStart() [2/2]
QDateTime Todo::dtStart | ( | bool | first | ) | const |
Returns the start datetime of the todo.
- Parameters
-
first If true, the start datetime of the todo will be returned; also, if the todo recurs, the start datetime of the first occurrence will be returned. If false and the todo recurs, the relative start datetime will be returned, based on the datetime returned by dtRecurrence().
- Returns
- A QDateTime for the start datetime of the todo.
◆ equals()
|
overrideprotectedvirtual |
Compare this with todo
for equality.
- Parameters
-
todo is the to-do to compare.
Reimplemented from KCalendarCore::Incidence.
◆ hasCompletedDate()
bool Todo::hasCompletedDate | ( | ) | const |
Returns if the to-do has a completion datetime.
- Returns
- true if the to-do has a date associated with completion; false otherwise.
- See also
- setCompleted(), completed()
◆ hasDueDate()
bool Todo::hasDueDate | ( | ) | const |
◆ hasStartDate()
bool Todo::hasStartDate | ( | ) | const |
◆ iconName()
|
overridevirtual |
Returns the name of the icon that best represents this incidence.
- Parameters
-
recurrenceId Some recurring incidences might use a different icon, for example, completed to-do occurrences. Use this parameter to identify the specific occurrence in a recurring series.
Implements KCalendarCore::Incidence.
◆ isCompleted()
bool Todo::isCompleted | ( | ) | const |
Returns whether the todo is completed or not.
- Returns
- true if the todo is 100% completed, has status
StatusCompleted
, or has a completed date; false otherwise.
- See also
- isOverdue, isInProgress(), isOpenEnded(), isNotStarted(bool), setCompleted(), percentComplete()
◆ isInProgress()
bool Todo::isInProgress | ( | bool | first | ) | const |
Returns true, if the to-do is in-progress (started, or >0% completed); otherwise return false.
If the to-do is overdue, then it is not considered to be in-progress.
- Parameters
-
first If true, the start and due dates of the todo will be used; also, if the todo recurs, the start date and due date of the first occurrence will be used. If false and the todo recurs, the relative start and due dates will be used, based on the date returned by dtRecurrence().
- See also
- isOverdue(), isCompleted(), isOpenEnded(), isNotStarted(bool)
◆ isNotStarted()
bool Todo::isNotStarted | ( | bool | first | ) | const |
Returns true, if the to-do has yet to be started (no start date and 0% completed); otherwise return false.
- Parameters
-
first If true, the start date of the todo will be used; also, if the todo recurs, the start date of the first occurrence will be used. If false and the todo recurs, the relative start date will be used, based on the date returned by dtRecurrence().
- See also
- isOverdue(), isCompleted(), isInProgress(), isOpenEnded()
◆ isOpenEnded()
bool Todo::isOpenEnded | ( | ) | const |
Returns true, if the to-do is open-ended (no due date); false otherwise.
- See also
- isOverdue(), isCompleted(), isInProgress(), isNotStarted(bool)
◆ isOverdue()
bool Todo::isOverdue | ( | ) | const |
Returns true if this todo is overdue (e.g.
due date is lower than today and not completed), else false.
- See also
- isCompleted(), isInProgress(), isOpenEnded(), isNotStarted(bool)
◆ mimeType()
|
overridevirtual |
Returns the Akonadi specific sub MIME type of a KCalendarCore::IncidenceBase item, e.g.
getting "application/x-vnd.akonadi.calendar.event" for a KCalendarCore::Event.
Implements KCalendarCore::IncidenceBase.
◆ percentComplete()
int Todo::percentComplete | ( | ) | const |
Returns what percentage of the to-do is completed.
- Returns
- The percentage complete of the to-do as an integer between 0 and 100, inclusive.
- See also
- setPercentComplete(), isCompleted()
◆ recursOn()
Returns true if the date
specified is one on which the to-do will recur.
Todos are a special case, hence the overload. It adds an extra check, which make it return false if there's an occurrence between the recur start and today.
- Parameters
-
date is the date to check. timeZone is the time zone
Reimplemented from KCalendarCore::Incidence.
◆ setAllDay()
|
overridevirtual |
Sets whether the incidence is all-day, i.e.
has a date but no time attached to it.
- Parameters
-
allDay sets whether the incidence is all-day.
- See also
- allDay()
Reimplemented from KCalendarCore::Incidence.
◆ setCompleted() [1/2]
void Todo::setCompleted | ( | bool | completed | ) |
Sets completion percentage and status.
- Parameters
-
completed If true
, percentage complete is set to 100%, and status is set toStatusCompleted
; the completion date is not set or cleared. Iffalse
, percentage complete is set to 0%, status is set toStatusNone
, and the completion date is cleared.
- See also
- isCompleted(), percentComplete(), hasCompletedDate()
◆ setCompleted() [2/2]
void Todo::setCompleted | ( | const QDateTime & | completeDate | ) |
Marks this Todo, or its current recurrence, as completed.
If the todo does not recur, its completion percentage is set to 100%, and its completion date is set to completeDate
. If its status is not StatusNone, it is set to StatusCompleted.
- Note
- If
completeDate
is invalid, the completion date is cleared, but the todo is still "complete".
If the todo recurs, the first incomplete recurrence is marked complete.
- Parameters
-
completeDate is the to-do completion date.
- See also
- completed(), hasCompletedDate()
◆ setDateTime()
|
overridevirtual |
Sets the date/time corresponding to the specified DateTimeRole.
- Parameters
-
dateTime is QDateTime value to set. role is a DateTimeRole.
Implements KCalendarCore::IncidenceBase.
◆ setDtDue()
void KCalendarCore::Todo::setDtDue | ( | const QDateTime & | dtDue, |
bool | first = false ) |
Sets due date and time.
- Parameters
-
dtDue The due date/time. first If true and the todo recurs, the due date of the first occurrence will be set. If false and the todo recurs, the occurrence at that date/time becomes the current occurrence. If the todo does not recur, the due date of the todo will be set.
◆ setDtRecurrence()
void Todo::setDtRecurrence | ( | const QDateTime & | dt | ) |
◆ setPercentComplete()
void Todo::setPercentComplete | ( | int | percent | ) |
Sets what percentage of the to-do is completed.
To prevent inconsistency, if percent
is not 100, completed() is cleared, and if status() is StatusCompleted it is reset to StatusNone.
- Parameters
-
percent is the completion percentage. Values greater than 100 are treated as 100; values less than p are treated as 0.
- See also
- isCompleted(), setCompleted()
◆ shiftTimes()
Shift the times of the incidence so that they appear at the same clock time as before but in a new time zone.
The shift is done from a viewing time zone rather than from the actual incidence time zone.
For example, shifting an incidence whose start time is 09:00 America/New York, using an old viewing time zone (oldSpec
) of Europe/London, to a new time zone (newSpec
) of Europe/Paris, will result in the time being shifted from 14:00 (which is the London time of the incidence start) to 14:00 Paris time.
- Parameters
-
oldZone the time zone which provides the clock times newZone the new time zone
Reimplemented from KCalendarCore::Incidence.
◆ supportsGroupwareCommunication()
|
overridevirtual |
◆ todoMimeType()
|
static |
Returns the Akonadi specific sub MIME type of a KCalendarCore::Todo.
◆ type()
|
overridevirtual |
Returns the incidence type.
Implements KCalendarCore::IncidenceBase.
◆ typeStr()
|
overridevirtual |
Prints the type of incidence as a string.
Implements KCalendarCore::IncidenceBase.
◆ virtual_hook()
|
overrideprotectedvirtual |
Standard trick to add virtuals later.
- Parameters
-
id is any integer unique to this class which we will use to identify the method to be called. data is a pointer to some glob of data, typically a struct.
Implements KCalendarCore::IncidenceBase.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:53:23 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.