KCalendarCore::Incidence
#include <incidence.h>
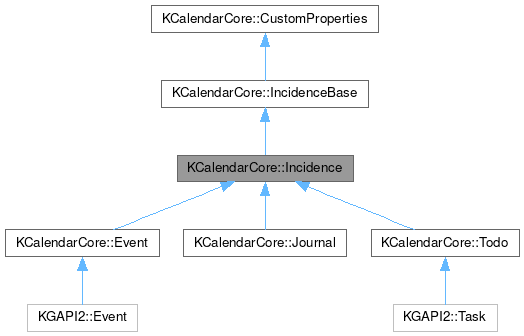
Properties | |
QList< KCalendarCore::Attachment > | attachments |
QStringList | categories |
QList< KCalendarCore::Conference > | conferences |
QDateTime | created |
QString | description |
float | geoLatitude |
float | geoLongitude |
bool | hasGeo |
QString | location |
int | priority |
KCalendarCore::Incidence::Secrecy | secrecy |
KCalendarCore::Incidence::Status | status |
QString | summary |
![]() | |
bool | allDay |
QList< KCalendarCore::Attendee > | attendees |
QDateTime | dtStart |
QDateTime | lastModified |
KCalendarCore::Person | organizer |
QString | uid |
QUrl | url |
Static Public Member Functions | |
static QStringList | mimeTypes () |
![]() | |
static quint32 | magicSerializationIdentifier () |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
Protected Member Functions | |
Incidence (const Incidence &)=delete | |
Incidence (const Incidence &other, IncidencePrivate *p) | |
IncidenceBase & | assign (const IncidenceBase &other) override |
void | deserialize (QDataStream &in) override |
bool | equals (const IncidenceBase &incidence) const override |
void | serialize (QDataStream &out) const override |
![]() | |
IncidenceBase (const IncidenceBase &)=delete | |
KCALENDARCORE_NO_EXPORT | IncidenceBase (const IncidenceBase &ib, IncidenceBasePrivate *p) |
void | customPropertyUpdate () override |
void | customPropertyUpdated () override |
void | setFieldDirty (IncidenceBase::Field field) |
virtual void | virtual_hook (VirtualHook id, void *data)=0 |
Additional Inherited Members | |
![]() | |
enum | VirtualHook |
![]() | |
IncidenceBasePrivate *const | d_ptr |
bool | mReadOnly |
Detailed Description
Provides the abstract base class common to non-FreeBusy (Events, To-dos, Journals) calendar components known as incidences.
Several properties are not allowed for VFREEBUSY objects (see rfc:2445), so they are not in IncidenceBase. The hierarchy is:
So IncidenceBase contains all properties that are common to all classes, and Incidence contains all additional properties that are common to Events, Todos and Journals, but are not allowed for FreeBusy entries.
Definition at line 59 of file incidence.h.
Member Typedef Documentation
◆ List
typedef QList<Ptr> KCalendarCore::Incidence::List |
List of incidences.
Definition at line 122 of file incidence.h.
◆ Ptr
A shared pointer to an Incidence.
Definition at line 117 of file incidence.h.
Member Enumeration Documentation
◆ RelType
The different types of RELTYPE values specified by the RFC.
Only RelTypeParent is supported for now.
Enumerator | |
---|---|
RelTypeParent | The related incidence is a parent. |
RelTypeChild | The related incidence is a child. |
RelTypeSibling | The related incidence is a peer. |
Definition at line 108 of file incidence.h.
◆ Secrecy
The different types of incidence access classifications.
Enumerator | |
---|---|
SecrecyPublic | Not secret (default) |
SecrecyPrivate | Secret to the owner. |
SecrecyConfidential | Secret to the owner and some others. |
Definition at line 97 of file incidence.h.
◆ Status
The different types of overall incidence status or confirmation.
The meaning is specific to the incidence type in context.
Definition at line 80 of file incidence.h.
Property Documentation
◆ attachments
|
read |
Definition at line 73 of file incidence.h.
◆ categories
|
readwrite |
Definition at line 68 of file incidence.h.
◆ conferences
|
read |
Definition at line 74 of file incidence.h.
◆ created
|
readwrite |
Definition at line 70 of file incidence.h.
◆ description
|
readwrite |
Definition at line 62 of file incidence.h.
◆ geoLatitude
|
readwrite |
Definition at line 66 of file incidence.h.
◆ geoLongitude
|
readwrite |
Definition at line 67 of file incidence.h.
◆ hasGeo
|
read |
Definition at line 65 of file incidence.h.
◆ location
|
readwrite |
Definition at line 64 of file incidence.h.
◆ priority
|
readwrite |
Definition at line 69 of file incidence.h.
◆ secrecy
|
readwrite |
Definition at line 71 of file incidence.h.
◆ status
|
readwrite |
Definition at line 72 of file incidence.h.
◆ summary
|
readwrite |
Definition at line 63 of file incidence.h.
Constructor & Destructor Documentation
◆ Incidence() [1/2]
Incidence::Incidence | ( | IncidencePrivate * | p | ) |
Constructs an empty incidence.
- Parameters
-
p (non-null) a Private data object provided by the instantiated class (Event, Todo, Journal, FreeBusy). It passes ownership of the object to IncidenceBase.
Definition at line 131 of file incidence.cpp.
◆ ~Incidence()
|
override |
Destroys an incidence.
Definition at line 147 of file incidence.cpp.
◆ Incidence() [2/2]
|
protected |
- Parameters
-
other is the incidence to copy. p (non-null) a Private data object provided by the instantiated class (Event, Todo, Journal, FreeBusy). It passes ownership of the object to IncidenceBase.
Definition at line 138 of file incidence.cpp.
Member Function Documentation
◆ addAlarm()
void Incidence::addAlarm | ( | const Alarm::Ptr & | alarm | ) |
Adds an alarm to the incidence.
- Parameters
-
alarm is a pointer to a valid Alarm object.
- See also
- removeAlarm().
Definition at line 892 of file incidence.cpp.
◆ addAttachment()
void Incidence::addAttachment | ( | const Attachment & | attachment | ) |
Adds an attachment to the incidence.
- Parameters
-
attachment a valid Attachment object.
Definition at line 713 of file incidence.cpp.
◆ addConference()
void Incidence::addConference | ( | const Conference & | conference | ) |
Adds a conference to the incidence.
- Parameters
-
conferene A conference to add.
- Since
- 5.77
Definition at line 936 of file incidence.cpp.
◆ alarms()
Alarm::List Incidence::alarms | ( | ) | const |
Returns a list of all incidence alarms.
Definition at line 878 of file incidence.cpp.
◆ altDescription()
QString Incidence::altDescription | ( | ) | const |
Returns the incidence alternative (=text/html) description.
- See also
- setAltDescription().
Definition at line 1163 of file incidence.cpp.
◆ assign()
|
overrideprotectedvirtual |
Provides polymorfic assignment.
- Parameters
-
other is the IncidenceBase to assign.
Reimplemented from KCalendarCore::IncidenceBase.
Reimplemented in KCalendarCore::Journal, and KCalendarCore::Todo.
Definition at line 166 of file incidence.cpp.
◆ attachments() [1/2]
Attachment::List Incidence::attachments | ( | ) | const |
Returns a list of all incidence attachments.
- See also
- attachments( const QString &).
Definition at line 740 of file incidence.cpp.
◆ attachments() [2/2]
Attachment::List Incidence::attachments | ( | const QString & | mime | ) | const |
Returns a list of all incidence attachments with the specified MIME type.
- Parameters
-
mime is a QString containing the MIME type.
- See also
- attachments().
Definition at line 746 of file incidence.cpp.
◆ categories()
QStringList Incidence::categories | ( | ) | const |
Returns the incidence categories as a list of strings.
Definition at line 504 of file incidence.cpp.
◆ categoriesStr()
QString Incidence::categoriesStr | ( | ) | const |
Returns the incidence categories as a comma separated string.
- See also
- categories().
Definition at line 510 of file incidence.cpp.
◆ clearAlarms()
void Incidence::clearAlarms | ( | ) |
◆ clearAttachments()
void Incidence::clearAttachments | ( | ) |
Removes all attachments and frees the memory used by them.
- See also
- deleteAttachments( const QString &).
Definition at line 758 of file incidence.cpp.
◆ clearConferences()
void Incidence::clearConferences | ( | ) |
◆ clearRecurrence()
void Incidence::clearRecurrence | ( | ) |
Removes all recurrence and exception rules and dates.
Definition at line 572 of file incidence.cpp.
◆ clone()
|
pure virtual |
Returns an exact copy of this incidence.
The returned object is owned by the caller.
Dirty fields are cleared.
Implemented in KCalendarCore::Event, KCalendarCore::Journal, and KCalendarCore::Todo.
◆ color()
QString Incidence::color | ( | ) | const |
Returns the color, if any is defined, for this incidence.
- Since
- : 5.76
Definition at line 550 of file incidence.cpp.
◆ conferences()
Conference::List Incidence::conferences | ( | ) | const |
Returns list of all incidence conferencing methods.
- Since
- 5.77
Definition at line 930 of file incidence.cpp.
◆ created()
QDateTime Incidence::created | ( | ) | const |
Returns the incidence creation date/time.
- See also
- setCreated().
Definition at line 333 of file incidence.cpp.
◆ customStatus()
QString Incidence::customStatus | ( | ) | const |
Returns the non-standard status value.
- See also
- setCustomStatus().
Definition at line 849 of file incidence.cpp.
◆ deleteAttachments()
void Incidence::deleteAttachments | ( | const QString & | mime | ) |
Removes all attachments of the specified MIME type from the incidence.
The memory used by all the removed attachments is freed.
- Parameters
-
mime is a QString containing the MIME type.
- See also
- deleteAttachment().
Definition at line 726 of file incidence.cpp.
◆ description()
QString Incidence::description | ( | ) | const |
Returns the incidence description.
- See also
- setDescription().
- richDescription().
Definition at line 402 of file incidence.cpp.
◆ descriptionIsRich()
bool Incidence::descriptionIsRich | ( | ) | const |
Returns true if incidence description contains RichText; false otherwise.
- See also
- setDescription(), description().
Definition at line 418 of file incidence.cpp.
◆ deserialize()
|
overrideprotectedvirtual |
Sub-type specific deserialization.
Reimplemented from KCalendarCore::IncidenceBase.
Definition at line 1207 of file incidence.cpp.
◆ endDateForStart()
Returns the end date/time of the incidence occurrence if it starts at specified date/time.
- Parameters
-
startDt is the specified starting date/time.
- Returns
- the corresponding end date/time for the occurrence; or the start date/time if the end date/time is invalid; or the end date/time if the start date/time is invalid.
Definition at line 699 of file incidence.cpp.
◆ equals()
|
overrideprotectedvirtual |
Compares this with Incidence incidence
for equality.
- Parameters
-
incidence is the Incidence to compare against.
- Returns
- true if the incidences are equal; false otherwise.
Reimplemented from KCalendarCore::IncidenceBase.
Reimplemented in KCalendarCore::Journal, and KCalendarCore::Todo.
Definition at line 180 of file incidence.cpp.
◆ geoLatitude()
float Incidence::geoLatitude | ( | ) | const |
Returns the incidence's geoLatitude as a value between -90.0 and 90.0 or INVALID_LATLON.
If either of geoLatitude() and geoLongitude() are INVALID_LATLON, then both are, and hasGeo() is false.
- Returns
- incidences geolatitude value
- See also
- setGeoLatitude().
Definition at line 1037 of file incidence.cpp.
◆ geoLongitude()
float Incidence::geoLongitude | ( | ) | const |
Returns the incidence's geoLongitude as a value between -180.0 and 180.0 or INVALID_LATLON.
If either of geoLatitude() and geoLongitude() are INVALID_LATLON, then both are, and hasGeo() is false.
- Returns
- incidences geolongitude value
- See also
- setGeoLongitude().
Definition at line 1065 of file incidence.cpp.
◆ hasAltDescription()
bool Incidence::hasAltDescription | ( | ) | const |
Returns true if the alternative (=text/html) description is available.
- See also
- setAltDescription(), altDescription()
Definition at line 1146 of file incidence.cpp.
◆ hasEnabledAlarms()
bool Incidence::hasEnabledAlarms | ( | ) | const |
Returns true if any of the incidence alarms are enabled; false otherwise.
Definition at line 922 of file incidence.cpp.
◆ hasGeo()
bool Incidence::hasGeo | ( | ) | const |
Returns true if the incidence has geo data, otherwise return false.
- See also
- setHasGeo(), setGeoLatitude(float), setGeoLongitude(float).
Definition at line 1030 of file incidence.cpp.
◆ hasRecurrenceId()
bool Incidence::hasRecurrenceId | ( | ) | const |
Returns true if the incidence has recurrenceId, otherwise return false.
- See also
- setRecurrenceId(QDateTime)
Definition at line 1093 of file incidence.cpp.
◆ iconName()
|
pure virtual |
Returns the name of the icon that best represents this incidence.
- Parameters
-
recurrenceId Some recurring incidences might use a different icon, for example, completed to-do occurrences. Use this parameter to identify the specific occurrence in a recurring serie.
Implemented in KCalendarCore::Event, KCalendarCore::Journal, and KCalendarCore::Todo.
◆ instanceIdentifier()
QString Incidence::instanceIdentifier | ( | ) | const |
Returns a unique identifier for a specific instance of an incidence.
Due to the recurrence-id, the uid is not unique for a KCalendarCore::Incidence.
- Since
- 4.11
Definition at line 255 of file incidence.cpp.
◆ localOnly()
bool Incidence::localOnly | ( | ) | const |
Get the localOnly status.
- Returns
- true if Local only, false otherwise.
- See also
- setLocalOnly()
Definition at line 299 of file incidence.cpp.
◆ location()
QString Incidence::location | ( | ) | const |
Returns the incidence location.
Do not use with journals.
- See also
- setLocation().
- richLocation().
Definition at line 984 of file incidence.cpp.
◆ locationIsRich()
bool Incidence::locationIsRich | ( | ) | const |
Returns true if incidence location contains RichText; false otherwise.
- See also
- setLocation(), location().
Definition at line 1000 of file incidence.cpp.
◆ mimeTypes()
|
static |
Returns the list of possible mime types in an Incidence object: "text/calendar" "application/x-vnd.akonadi.calendar.event" "application/x-vnd.akonadi.calendar.todo" "application/x-vnd.akonadi.calendar.journal".
static
- Since
- 4.12
Definition at line 1173 of file incidence.cpp.
◆ newAlarm()
Alarm::Ptr Incidence::newAlarm | ( | ) |
Create a new incidence alarm.
Definition at line 884 of file incidence.cpp.
◆ priority()
int Incidence::priority | ( | ) | const |
Returns the incidence priority.
- See also
- setPriority().
Definition at line 804 of file incidence.cpp.
◆ recreate()
void Incidence::recreate | ( | ) |
Recreate incidence.
The incidence is made a new unique incidence, but already stored information is preserved. Sets unique id, creation date, last modification date and revision number.
Definition at line 263 of file incidence.cpp.
◆ recurrence()
Recurrence * Incidence::recurrence | ( | ) | const |
Returns the recurrence rule associated with this incidence.
If there is none, returns an appropriate (non-0) object.
Definition at line 558 of file incidence.cpp.
◆ recurrenceId()
|
overridevirtual |
Returns the incidence recurrenceId.
- Returns
- incidences recurrenceId value
- See also
- setRecurrenceId().
Reimplemented from KCalendarCore::IncidenceBase.
Definition at line 1099 of file incidence.cpp.
◆ recurrenceType()
ushort Incidence::recurrenceType | ( | ) | const |
Returns the event's recurrence status.
See the enumeration at the top of this file for possible values.
Definition at line 579 of file incidence.cpp.
◆ recurrenceUpdated()
|
override |
Observer interface for the recurrence class.
If the recurrence is changed, this method will be called for the incidence the recurrence object belongs to.
- Parameters
-
recurrence is a pointer to a valid Recurrence object.
If the recurrence is changed, this method will be called for the incidence the recurrence object belongs to.
Definition at line 1131 of file incidence.cpp.
◆ recurs()
bool Incidence::recurs | ( | ) | const |
Returns whether the event recurs at all.
Definition at line 589 of file incidence.cpp.
◆ recursAt()
bool Incidence::recursAt | ( | const QDateTime & | dt | ) | const |
Returns true if the date/time specified is one at which the event will recur.
Times are rounded down to the nearest minute to determine the result.
- Parameters
-
dt is the date/time to check.
Definition at line 605 of file incidence.cpp.
◆ recursOn()
Returns true if the date specified is one on which the event will recur.
- Parameters
-
date date to check. timeZone time zone for the date
.
Reimplemented in KCalendarCore::Todo.
Definition at line 599 of file incidence.cpp.
◆ relatedTo()
QString Incidence::relatedTo | ( | RelType | relType = RelTypeParent | ) | const |
Returns a UID string for the incidence that is related to this one.
This function should only be used when constructing a calendar before the related incidence exists.
- Warning
- KCalendarCore only supports one related-to field per reltype for now.
- Parameters
-
relType specifies the relation type.
- See also
- setRelatedTo().
Definition at line 530 of file incidence.cpp.
◆ removeAlarm()
void Incidence::removeAlarm | ( | const Alarm::Ptr & | alarm | ) |
Removes the specified alarm from the incidence.
- Parameters
-
alarm is a pointer to a valid Alarm object.
- See also
- addAlarm().
Definition at line 901 of file incidence.cpp.
◆ resources()
QStringList Incidence::resources | ( | ) | const |
Returns the incidence resources as a list of strings.
- See also
- setResources().
Definition at line 780 of file incidence.cpp.
◆ revision()
int Incidence::revision | ( | ) | const |
Returns the number of revisions this incidence has seen.
- See also
- setRevision().
Definition at line 352 of file incidence.cpp.
◆ richDescription()
QString Incidence::richDescription | ( | ) | const |
Returns the incidence description in rich text format.
- See also
- setDescription().
- description().
Definition at line 408 of file incidence.cpp.
◆ richLocation()
QString Incidence::richLocation | ( | ) | const |
Returns the incidence location in rich text format.
- See also
- setLocation().
- location().
Definition at line 990 of file incidence.cpp.
◆ richSummary()
QString Incidence::richSummary | ( | ) | const |
Returns the incidence summary in rich text format.
- See also
- setSummary().
- summary().
Definition at line 450 of file incidence.cpp.
◆ schedulingID()
QString Incidence::schedulingID | ( | ) | const |
Returns the incidence scheduling ID.
Do not use with journals. If a scheduling ID is not set, then return the incidence UID.
- See also
- setSchedulingID().
Definition at line 1020 of file incidence.cpp.
◆ secrecy()
Incidence::Secrecy Incidence::secrecy | ( | ) | const |
Returns the incidence Secrecy.
- See also
- setSecrecy(), secrecyStr().
Definition at line 872 of file incidence.cpp.
◆ serialize()
|
overrideprotectedvirtual |
Sub-type specific serialization.
Reimplemented from KCalendarCore::IncidenceBase.
Definition at line 1179 of file incidence.cpp.
◆ setAllDay()
|
overridevirtual |
Sets whether the incidence is all-day, i.e.
has a date but no time attached to it.
- Parameters
-
allDay sets whether the incidence is all-day.
- See also
- allDay()
Reimplemented from KCalendarCore::IncidenceBase.
Reimplemented in KCalendarCore::Todo.
Definition at line 305 of file incidence.cpp.
◆ setAltDescription()
void Incidence::setAltDescription | ( | const QString & | altdescription | ) |
Sets the incidence's alternative (=text/html) description.
If the text is empty, the property is removed.
- Parameters
-
altdescription is the incidence altdescription string.
- See also
- altAltdescription().
Definition at line 1154 of file incidence.cpp.
◆ setCategories() [1/2]
void Incidence::setCategories | ( | const QString & | catStr | ) |
Sets the incidence category list based on a comma delimited string.
- Parameters
-
catStr is a QString containing a list of categories which are delimited by a comma character.
- See also
- setCategories( const QStringList &), categories().
Definition at line 479 of file incidence.cpp.
◆ setCategories() [2/2]
void Incidence::setCategories | ( | const QStringList & | categories | ) |
Sets the incidence category list.
- Parameters
-
categories is a list of category strings.
- See also
- setCategories( const QString &), categories().
Definition at line 466 of file incidence.cpp.
◆ setColor()
void Incidence::setColor | ( | const QString & | colorName | ) |
Set the incidence color, as added in RFC7986.
- Parameters
-
colorName a named color as defined in CSS3 color name, see https://www.w3.org/TR/css-color-3/#svg-color.
- Since
- : 5.76
Definition at line 536 of file incidence.cpp.
◆ setConferences()
void Incidence::setConferences | ( | const Conference::List & | conferences | ) |
Replaces all conferences in the incidence with given conferences
.
- Parameters
-
conferences New conferences to store in the incidence.
- Since
- 5.77
Definition at line 945 of file incidence.cpp.
◆ setCreated()
void Incidence::setCreated | ( | const QDateTime & | dt | ) |
Sets the incidence creation date/time.
It is stored as a UTC date/time.
- Parameters
-
dt is the creation date/time.
- See also
- created().
Definition at line 317 of file incidence.cpp.
◆ setCustomStatus()
void Incidence::setCustomStatus | ( | const QString & | status | ) |
Sets the incidence Status to a non-standard status value.
- Parameters
-
status is a non-standard status string. If empty, the incidence Status will be set to StatusNone.
- See also
- setStatus(), status() customStatus().
Definition at line 829 of file incidence.cpp.
◆ setDescription() [1/2]
void Incidence::setDescription | ( | const QString & | description | ) |
Sets the incidence description and tries to guess if the description is rich text.
- Parameters
-
description is the incidence description string.
- See also
- description().
Definition at line 397 of file incidence.cpp.
◆ setDescription() [2/2]
void Incidence::setDescription | ( | const QString & | description, |
bool | isRich ) |
Sets the incidence description.
- Parameters
-
description is the incidence description string. isRich if true indicates the description string contains richtext.
- See also
- description().
Definition at line 384 of file incidence.cpp.
◆ setDtStart()
|
overridevirtual |
Sets the incidence starting date/time.
- Parameters
-
dt is the starting date/time.
- See also
- IncidenceBase::dtStart().
Reimplemented from KCalendarCore::IncidenceBase.
Definition at line 358 of file incidence.cpp.
◆ setGeoLatitude()
void Incidence::setGeoLatitude | ( | float | geolatitude | ) |
Set the incidence's geoLatitude.
- Parameters
-
geolatitude is the incidence geolatitude to set; a value between -90.0 and 90.0, or INVALID_LATLON (or NaN, which is treated as INVALID_LATLON).
- See also
- geoLatitude().
Definition at line 1044 of file incidence.cpp.
◆ setGeoLongitude()
void Incidence::setGeoLongitude | ( | float | geolongitude | ) |
Set the incidence's geoLongitude.
- Parameters
-
geolongitude is the incidence geolongitude to set; a value between -180.0 and 180.0, or INVALID_LATLON (or NaN, which is treated as INVALID_LATLON).
- See also
- geoLongitude().
Definition at line 1072 of file incidence.cpp.
◆ setLastModified()
|
overridevirtual |
Sets the time the incidence was last modified to lm
.
It is stored as a UTC date/time.
- Parameters
-
lm is the QDateTime when the incidence was last modified.
- See also
- lastModified()
Reimplemented from KCalendarCore::IncidenceBase.
Definition at line 273 of file incidence.cpp.
◆ setLocalOnly()
void Incidence::setLocalOnly | ( | bool | localonly | ) |
Set localOnly state of incidence.
A local only incidence can be updated but it will not increase the revision number neither the modified date.
- Parameters
-
localonly If true, the incidence is set to localonly, if false the incidence is set to normal stat.
Definition at line 290 of file incidence.cpp.
◆ setLocation() [1/2]
void Incidence::setLocation | ( | const QString & | location | ) |
Sets the incidence location and tries to guess if the location is richtext.
Do not use with journals.
- Parameters
-
location is the incidence location string.
- See also
- location().
Definition at line 979 of file incidence.cpp.
◆ setLocation() [2/2]
void Incidence::setLocation | ( | const QString & | location, |
bool | isRich ) |
Sets the incidence location.
Do not use with journals.
- Parameters
-
location is the incidence location string. isRich if true indicates the location string contains richtext.
- See also
- location().
Definition at line 963 of file incidence.cpp.
◆ setPriority()
void Incidence::setPriority | ( | int | priority | ) |
Sets the incidences priority.
The priority must be an integer value between 0 and 9, where 0 is undefined, 1 is the highest, and 9 is the lowest priority (decreasing order).
- Parameters
-
priority is the incidence priority to set.
- See also
- priority().
Definition at line 786 of file incidence.cpp.
◆ setReadOnly()
|
overridevirtual |
Set readonly state of incidence.
- Parameters
-
readonly If true, the incidence is set to readonly, if false the incidence is set to readwrite.
Reimplemented from KCalendarCore::IncidenceBase.
Definition at line 281 of file incidence.cpp.
◆ setRecurrenceId()
void Incidence::setRecurrenceId | ( | const QDateTime & | recurrenceId | ) |
Set the incidences recurrenceId.
This field indicates that this is an exception to a recurring incidence. The uid of this incidence MUST be the same as the one of the recurring main incidence.
- Parameters
-
recurrenceId is the incidence recurrenceId to set
- See also
- recurrenceId().
Definition at line 1117 of file incidence.cpp.
◆ setRelatedTo()
void Incidence::setRelatedTo | ( | const QString & | uid, |
RelType | relType = RelTypeParent ) |
Relates another incidence to this one, by UID.
This function should only be used when constructing a calendar before the related incidence exists.
- Parameters
-
uid is a QString containing a UID for another incidence. relType specifies the relation type.
- Warning
- KCalendarCore only supports one related-to field per reltype for now.
- See also
- relatedTo().
Definition at line 516 of file incidence.cpp.
◆ setResources()
void Incidence::setResources | ( | const QStringList & | resources | ) |
Sets a list of incidence resources.
(Note: resources in this context means items used by the incidence such as money, fuel, hours, etc).
- Parameters
-
resources is a list of resource strings.
- See also
- resources().
Definition at line 767 of file incidence.cpp.
◆ setRevision()
void Incidence::setRevision | ( | int | rev | ) |
Sets the number of revisions this incidence has seen.
- Parameters
-
rev is the incidence revision number.
- See also
- revision().
Definition at line 339 of file incidence.cpp.
◆ setSchedulingID()
Set the incidence scheduling ID.
Do not use with journals. This is used for accepted invitations as the place to store the UID of the invitation. It is later used again if updates to the invitation comes in. If we did not set a new UID on incidences from invitations, we can end up with more than one resource having events with the same UID, if you have access to other peoples resources.
While constructing an incidence, when setting the scheduling ID, you will always want to set the incidence UID too. Instead of calling setUID() separately, you can pass the UID through uid
so both members are changed in one atomic operation ( don't forget that setUID() emits incidenceUpdated() and whoever catches that signal will have an half-initialized incidence, therefore, always set the schedulingID and UID at the same time, and never with two separate calls).
- Parameters
-
sid is a QString containing the scheduling ID. uid is a QString containing the incidence UID to set, if not specified, the current UID isn't changed, and this parameter is ignored.
- See also
- schedulingID().
Definition at line 1006 of file incidence.cpp.
◆ setSecrecy()
void Incidence::setSecrecy | ( | Incidence::Secrecy | secrecy | ) |
Sets the incidence Secrecy.
- Parameters
-
secrecy is the incidence Secrecy to set.
- See also
- secrecy(), secrecyStr().
Definition at line 859 of file incidence.cpp.
◆ setStatus()
void Incidence::setStatus | ( | Incidence::Status | status | ) |
Sets the incidence status to a standard Status value.
Events, Todos, and Journals each have a different set of valid statuses. Note that StatusX cannot be specified. Invalid statuses are logged and ignored.
- Parameters
-
status is the incidence Status to set.
- See also
- status(), setCustomStatus().
Definition at line 810 of file incidence.cpp.
◆ setSummary() [1/2]
void Incidence::setSummary | ( | const QString & | summary | ) |
Sets the incidence summary and tries to guess if the summary is richtext.
- Parameters
-
summary is the incidence summary string.
- See also
- summary().
Definition at line 439 of file incidence.cpp.
◆ setSummary() [2/2]
void Incidence::setSummary | ( | const QString & | summary, |
bool | isRich ) |
Sets the incidence summary.
- Parameters
-
summary is the incidence summary string. isRich if true indicates the summary string contains richtext.
- See also
- summary().
Definition at line 424 of file incidence.cpp.
◆ setThisAndFuture()
void Incidence::setThisAndFuture | ( | bool | thisAndFuture | ) |
Set to true if the exception also applies to all future occurrences.
This option is only relevant if the incidence has a recurrenceId set.
- Parameters
-
thisAndFuture value
- See also
- thisAndFuture(), setRecurrenceId()
- Since
- 4.11
Definition at line 1105 of file incidence.cpp.
◆ shiftTimes()
Shift the times of the incidence so that they appear at the same clock time as before but in a new time zone.
The shift is done from a viewing time zone rather than from the actual incidence time zone.
For example, shifting an incidence whose start time is 09:00 America/New York, using an old viewing time zone (oldSpec
) of Europe/London, to a new time zone (newSpec
) of Europe/Paris, will result in the time being shifted from 14:00 (which is the London time of the incidence start) to 14:00 Paris time.
- Parameters
-
oldZone the time zone which provides the clock times newZone the new time zone
Reimplemented from KCalendarCore::IncidenceBase.
Reimplemented in KCalendarCore::Todo.
Definition at line 367 of file incidence.cpp.
◆ startDateTimesForDate()
|
virtual |
Calculates the start date/time for all recurrences that happen at some time on the given date (might start before that date, but end on or after the given date).
- Parameters
-
date the date when the incidence should occur timeSpec time specification for date
.
- Returns
- the start date/time of all occurrences that overlap with the given date; an empty list if the incidence does not overlap with the date at all.
Definition at line 611 of file incidence.cpp.
◆ startDateTimesForDateTime()
Calculates the start date/time for all recurrences that happen at the given time.
- Parameters
-
datetime the date/time when the incidence should occur.
- Returns
- the start date/time of all occurrences that overlap with the given date/time; an empty list if the incidence does not happen at the given time at all.
Definition at line 655 of file incidence.cpp.
◆ status()
Incidence::Status Incidence::status | ( | ) | const |
Returns the incidence Status.
- See also
- setStatus(), setCustomStatus(), statusStr().
Definition at line 843 of file incidence.cpp.
◆ summary()
QString Incidence::summary | ( | ) | const |
Returns the incidence summary.
- See also
- setSummary().
- richSummary().
Definition at line 444 of file incidence.cpp.
◆ summaryIsRich()
bool Incidence::summaryIsRich | ( | ) | const |
Returns true if incidence summary contains RichText; false otherwise.
- See also
- setSummary(), summary().
Definition at line 460 of file incidence.cpp.
◆ supportsGroupwareCommunication()
|
pure virtual |
Returns true if the incidence type supports groupware communication.
- Since
- 4.10
Implemented in KCalendarCore::Event, KCalendarCore::Journal, and KCalendarCore::Todo.
◆ thisAndFuture()
bool Incidence::thisAndFuture | ( | ) | const |
Returns true if the exception also applies to all future occurrences.
- Returns
- incidences thisAndFuture value
- See also
- setThisAndFuture()
- Since
- 4.11
Definition at line 1111 of file incidence.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Tue Mar 26 2024 11:13:47 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.