KCalendarCore::Alarm
#include <alarm.h>
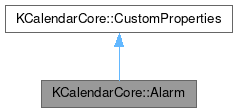
Public Types | |
typedef QList< Ptr > | List |
typedef QSharedPointer< Alarm > | Ptr |
enum | Type { Invalid , Display , Procedure , Email , Audio } |
Protected Member Functions | |
void | customPropertyUpdated () override |
virtual void | virtual_hook (int id, void *data) |
![]() | |
virtual void | customPropertyUpdate () |
Additional Inherited Members | |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
Detailed Description
Represents an alarm notification.
Alarms are user notifications that occur at specified times. Notifications can be on-screen pop-up dialogs, email messages, the playing of audio files, or the running of another program.
Alarms always belong to a parent Incidence.
Member Typedef Documentation
◆ List
typedef QList<Ptr> KCalendarCore::Alarm::List |
◆ Ptr
typedef QSharedPointer<Alarm> KCalendarCore::Alarm::Ptr |
Member Enumeration Documentation
◆ Type
Constructor & Destructor Documentation
◆ Alarm() [1/2]
|
explicit |
◆ Alarm() [2/2]
Alarm::Alarm | ( | const Alarm & | other | ) |
◆ ~Alarm()
Member Function Documentation
◆ addMailAddress()
void Alarm::addMailAddress | ( | const Person & | mailAlarmAddress | ) |
◆ addMailAttachment()
void Alarm::addMailAttachment | ( | const QString & | mailAttachFile | ) |
◆ audioFile()
QString Alarm::audioFile | ( | ) | const |
Returns the audio file name for an Audio alarm type.
Returns an empty string if the alarm is not an Audio type.
- See also
- setAudioAlarm(), setAudioFile()
◆ customPropertyUpdated()
|
overrideprotectedvirtual |
CustomProperties::customPropertyUpdated()
Reimplemented from KCalendarCore::CustomProperties.
◆ duration()
Duration Alarm::duration | ( | ) | const |
◆ enabled()
bool Alarm::enabled | ( | ) | const |
Returns the alarm enabled status: true (enabled) or false (disabled).
- See also
- setEnabled(), toggleAlarm()
◆ endOffset()
Duration Alarm::endOffset | ( | ) | const |
Returns offset of alarm in time relative to the end of the event.
If the alarm's time is not defined in terms of an offset relative to the end of the event, returns zero.
- See also
- setEndOffset(), hasEndOffset()
◆ endTime()
QDateTime Alarm::endTime | ( | ) | const |
◆ hasEndOffset()
bool Alarm::hasEndOffset | ( | ) | const |
Returns whether the alarm is defined in terms of an offset relative to the end of the event.
- See also
- endOffset(), setEndOffset()
◆ hasLocationRadius()
bool Alarm::hasLocationRadius | ( | ) | const |
Returns true if alarm has location radius defined.
- See also
- setLocationRadius()
◆ hasStartOffset()
bool Alarm::hasStartOffset | ( | ) | const |
Returns whether the alarm is defined in terms of an offset relative to the start of the parent Incidence.
- See also
- startOffset(), setStartOffset()
◆ hasTime()
bool Alarm::hasTime | ( | ) | const |
◆ locationRadius()
int Alarm::locationRadius | ( | ) | const |
Returns the location radius in meters.
- See also
- setLocationRadius()
◆ mailAddresses()
Person::List Alarm::mailAddresses | ( | ) | const |
◆ mailAttachments()
QStringList Alarm::mailAttachments | ( | ) | const |
◆ mailSubject()
QString Alarm::mailSubject | ( | ) | const |
Returns the subject line string for an Email alarm type.
Returns an empty string if the alarm is not an Email type.
- See also
- setMailSubject()
◆ mailText()
QString Alarm::mailText | ( | ) | const |
Returns the body text for an Email alarm type.
Returns an empty string if the alarm is not an Email type.
- See also
- setMailText()
◆ nextRepetition()
Returns the date/time of the alarm's initial occurrence or its next repetition after a given time.
- Parameters
-
preTime the date/time after which to find the next repetition.
- Returns
- the date/time of the next repetition, or an invalid date/time if the specified time is at or after the alarm's last repetition.
- See also
- previousRepetition()
◆ nextTime()
Returns the next alarm trigger date/time after given date/time.
Takes recurrent incidences into account.
- Parameters
-
preTime date/time from where to start ignoreRepetitions don't take repetitions into account
- See also
- nextRepetition()
◆ operator!=()
bool Alarm::operator!= | ( | const Alarm & | a | ) | const |
◆ operator=()
◆ operator==()
bool Alarm::operator== | ( | const Alarm & | a | ) | const |
◆ parentUid()
QString Alarm::parentUid | ( | ) | const |
Returns the parent's incidence UID of the alarm.
- See also
- setParent()
◆ previousRepetition()
Returns the date/time of the alarm's latest repetition or, if none, its initial occurrence before a given time.
- Parameters
-
afterTime is the date/time before which to find the latest repetition.
- Returns
- the date and time of the latest repetition, or an invalid date/time if the specified time is at or before the alarm's initial occurrence.
- See also
- nextRepetition()
◆ programArguments()
QString Alarm::programArguments | ( | ) | const |
◆ programFile()
QString Alarm::programFile | ( | ) | const |
◆ repeatCount()
int Alarm::repeatCount | ( | ) | const |
Returns how many times an alarm may repeats after its initial occurrence.
- See also
- setRepeatCount()
◆ setAudioAlarm()
Sets the Audio type for this alarm and the name of the audio file to play when the alarm is triggered.
- Parameters
-
audioFile is the name of the audio file to play when the alarm is triggered.
- See also
- setAudioFile(), audioFile()
◆ setAudioFile()
void Alarm::setAudioFile | ( | const QString & | audioFile | ) |
Sets the name of the audio file to play when the audio alarm is triggered.
Ignored if the alarm is not an Audio type.
- Parameters
-
audioFile is the name of the audio file to play when the alarm is triggered.
- See also
- setAudioAlarm(), audioFile()
◆ setDisplayAlarm()
◆ setEmailAlarm()
void Alarm::setEmailAlarm | ( | const QString & | subject, |
const QString & | text, | ||
const Person::List & | addressees, | ||
const QStringList & | attachments = QStringList() ) |
Sets the Email type for this alarm and the email subject
, text
, addresses
, and attachments
that make up an email message to be sent when the alarm is triggered.
- Parameters
-
subject is the email subject. text is a string containing the body of the email message. addressees is Person list of email addresses. attachments is a a QStringList of optional file names of email attachments.
◆ setEnabled()
void Alarm::setEnabled | ( | bool | enable | ) |
Sets the enabled status of the alarm.
- Parameters
-
enable if true, then enable the alarm; else disable the alarm.
- See also
- enabled(), toggleAlarm()
◆ setEndOffset()
void Alarm::setEndOffset | ( | const Duration & | offset | ) |
Sets the alarm offset relative to the end of the parent Incidence.
- Parameters
-
offset is a Duration to be used as a time relative to the end of the parent Incidence to be used as the alarm trigger.
- See also
- setStartOffset(), startOffset(), endOffset()
◆ setHasLocationRadius()
void Alarm::setHasLocationRadius | ( | bool | hasLocationRadius | ) |
Set if the location radius for the alarm has been defined.
- Parameters
-
hasLocationRadius if true, then this alarm has a location radius.
- See also
- setLocationRadius()
◆ setLocationRadius()
void Alarm::setLocationRadius | ( | int | locationRadius | ) |
Set location radius for the alarm.
This means that alarm will be triggered when user approaches the location. Given value will be stored into custom properties as X-LOCATION-RADIUS.
- Parameters
-
locationRadius radius in meters
- See also
- locationRadius()
◆ setMailAddress()
void Alarm::setMailAddress | ( | const Person & | mailAlarmAddress | ) |
Sets the email address of an Email type alarm.
Ignored if the alarm is not an Email type.
- Parameters
-
mailAlarmAddress is a Person to receive a mail message when an Email type alarm is triggered.
◆ setMailAddresses()
void Alarm::setMailAddresses | ( | const Person::List & | mailAlarmAddresses | ) |
Sets a list of email addresses of an Email type alarm.
Ignored if the alarm is not an Email type.
- Parameters
-
mailAlarmAddresses is a Person list to receive a mail message when an Email type alarm is triggered.
◆ setMailAttachment()
void Alarm::setMailAttachment | ( | const QString & | mailAttachFile | ) |
Sets the filename to attach to a mail message for an Email alarm type.
Ignored if the alarm is not an Email type.
- Parameters
-
mailAttachFile is a string containing a filename to be attached to an email message to send when the Email type alarm is triggered.
◆ setMailAttachments()
void Alarm::setMailAttachments | ( | const QStringList & | mailAttachFiles | ) |
◆ setMailSubject()
void Alarm::setMailSubject | ( | const QString & | mailAlarmSubject | ) |
Sets the subject line of a mail message for an Email alarm type.
Ignored if the alarm is not an Email type.
- Parameters
-
mailAlarmSubject is a string to be used as a subject line of an email message to send when the Email type alarm is triggered.
◆ setMailText()
void Alarm::setMailText | ( | const QString & | text | ) |
Sets the body text for an Email alarm type.
Ignored if the alarm is not an Email type.
- Parameters
-
text is a string containing the body text of a mail message when an Email type alarm is triggered.
◆ setParent()
void Alarm::setParent | ( | Incidence * | parent | ) |
Sets the parent
Incidence of the alarm.
- Parameters
-
parent is alarm parent Incidence to set.
- See also
- parentUid()
◆ setProcedureAlarm()
void Alarm::setProcedureAlarm | ( | const QString & | programFile, |
const QString & | arguments = QString() ) |
Sets the Procedure type for this alarm and the program (with arguments) to execute when the alarm is triggered.
- Parameters
-
programFile is the name of the program file to execute when the alarm is triggered. arguments is a string of arguments to supply to programFile
.
◆ setProgramArguments()
void Alarm::setProgramArguments | ( | const QString & | arguments | ) |
Sets the program arguments string when the alarm is triggered.
Ignored if the alarm is not a Procedure type.
- Parameters
-
arguments is a string of arguments to supply to the program.
◆ setProgramFile()
void Alarm::setProgramFile | ( | const QString & | programFile | ) |
Sets the program file to execute when the alarm is triggered.
Ignored if the alarm is not a Procedure type.
- Parameters
-
programFile is the name of the program file to execute when the alarm is triggered.
◆ setRepeatCount()
void Alarm::setRepeatCount | ( | int | alarmRepeatCount | ) |
Sets how many times an alarm is to repeat itself after its initial occurrence (w/snoozes).
- Parameters
-
alarmRepeatCount is the number of times an alarm may repeat, excluding the initial occurrence.
- See also
- repeatCount()
◆ setSnoozeTime()
void Alarm::setSnoozeTime | ( | const Duration & | alarmSnoozeTime | ) |
Sets the snooze time interval for the alarm.
- Parameters
-
alarmSnoozeTime the time between snoozes.
- See also
- snoozeTime()
◆ setStartOffset()
void Alarm::setStartOffset | ( | const Duration & | offset | ) |
Sets the alarm offset relative to the start of the parent Incidence.
- Parameters
-
offset is a Duration to be used as a time relative to the start of the parent Incidence to be used as the alarm trigger.
- See also
- setEndOffset(), startOffset(), endOffset()
◆ setText()
void Alarm::setText | ( | const QString & | text | ) |
Sets the description text
to be displayed when the alarm is triggered.
Ignored if the alarm is not a display alarm.
- Parameters
-
text is the description to display when the alarm is triggered.
- See also
- setDisplayAlarm(), text()
◆ setTime()
void Alarm::setTime | ( | const QDateTime & | alarmTime | ) |
◆ setType()
void Alarm::setType | ( | Alarm::Type | type | ) |
◆ shiftTimes()
Shift the times of the alarm so that they appear at the same clock time as before but in a new time zone.
The shift is done from a viewing time zone rather than from the actual alarm time zone.
For example, shifting an alarm whose start time is 09:00 America/New York, using an old viewing time zone (oldZone
) of Europe/London, to a new time zone (newZone
) of Europe/Paris, will result in the time being shifted from 14:00 (which is the London time of the alarm start) to 14:00 Paris time.
- Parameters
-
oldZone the time zone which provides the clock times newZone the new time zone
◆ snoozeTime()
Duration Alarm::snoozeTime | ( | ) | const |
◆ startOffset()
Duration Alarm::startOffset | ( | ) | const |
Returns offset of alarm in time relative to the start of the parent Incidence.
If the alarm's time is not defined in terms of an offset relative to the start of the event, returns zero.
- See also
- setStartOffset(), hasStartOffset()
◆ text()
QString Alarm::text | ( | ) | const |
◆ time()
QDateTime Alarm::time | ( | ) | const |
◆ toggleAlarm()
void Alarm::toggleAlarm | ( | ) |
Toggles the alarm status, i.e, an enable alarm becomes disabled and a disabled alarm becomes enabled.
- See also
- enabled(), setEnabled()
◆ type()
Alarm::Type Alarm::type | ( | ) | const |
◆ virtual_hook()
|
protectedvirtual |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:53:23 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.