KAbstractConfigModule
#include <kabstractconfigmodule.h>
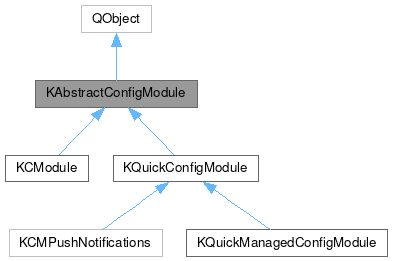
Public Types | |
enum | Button { NoAdditionalButton = 0 , Help = 1 , Default = 2 , Apply = 4 , Export = 8 } |
typedef QFlags< Button > | Buttons |
Properties | |
KAbstractConfigModule::Buttons | buttons |
bool | defaultsIndicatorsVisible |
QString | description |
QString | name |
bool | needsAuthorization |
bool | needsSave |
bool | representsDefaults |
![]() | |
objectName | |
Public Member Functions | |
KAbstractConfigModule (QObject *parent, const KPluginMetaData &metaData) | |
Q_SIGNAL void | activationRequested (const QVariantList &args) |
QString | authActionName () const |
Q_SIGNAL void | authActionNameChanged () |
Buttons | buttons () const |
Q_SIGNAL void | buttonsChanged () |
virtual void | defaults () |
bool | defaultsIndicatorsVisible () const |
Q_SIGNAL void | defaultsIndicatorsVisibleChanged () |
QString | description () const |
virtual void | load () |
KPluginMetaData | metaData () const |
QString | name () const |
bool | needsAuthorization () const |
bool | needsSave () const |
Q_SIGNAL void | needsSaveChanged () |
bool | representsDefaults () const |
Q_SIGNAL void | representsDefaultsChanged () |
virtual void | save () |
void | setAuthActionName (const QString &action) |
void | setButtons (const Buttons btn) |
void | setDefaultsIndicatorsVisible (bool visible) |
void | setNeedsSave (bool needs) |
void | setRepresentsDefaults (bool defaults) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Base class for QML and QWidgets config modules.
- Since
- 6.0
Definition at line 24 of file kabstractconfigmodule.h.
Member Typedef Documentation
◆ Buttons
typedef QFlags< Button > KAbstractConfigModule::Buttons |
Definition at line 51 of file kabstractconfigmodule.h.
Member Enumeration Documentation
◆ Button
An enumeration type for the buttons used by this module.
You should only use Help, Default and Apply. The rest is obsolete. NoAdditionalButton can be used when we do not want have other button that Ok Cancel
- See also
- ConfigModule::buttons
- ConfigModule::setButtons
Definition at line 43 of file kabstractconfigmodule.h.
Property Documentation
◆ buttons
|
readwrite |
Definition at line 28 of file kabstractconfigmodule.h.
◆ defaultsIndicatorsVisible
|
readwrite |
Definition at line 29 of file kabstractconfigmodule.h.
◆ description
|
read |
Definition at line 34 of file kabstractconfigmodule.h.
◆ name
|
read |
Definition at line 33 of file kabstractconfigmodule.h.
◆ needsAuthorization
|
read |
Definition at line 30 of file kabstractconfigmodule.h.
◆ needsSave
|
readwrite |
Definition at line 32 of file kabstractconfigmodule.h.
◆ representsDefaults
|
readwrite |
Definition at line 31 of file kabstractconfigmodule.h.
Constructor & Destructor Documentation
◆ KAbstractConfigModule()
|
explicit |
Definition at line 29 of file kabstractconfigmodule.cpp.
Member Function Documentation
◆ activationRequested()
Q_SIGNAL void KAbstractConfigModule::activationRequested | ( | const QVariantList & | args | ) |
This signal will be emited by a single-instance application (such as System Settings) to request activation and update arguments to a module that is already running.
The module should connect to this signal when it needs to handle the activation request and specially the new arguments
- Parameters
-
args A list of arguments that get passed to the module
◆ authActionName()
QString KAbstractConfigModule::authActionName | ( | ) | const |
Returns the action previously set with setAuthActionName().
By default this will be a empty string.
- Returns
- The action that has to be authorized to execute the save() method.
Definition at line 77 of file kabstractconfigmodule.cpp.
◆ authActionNameChanged()
Q_SIGNAL void KAbstractConfigModule::authActionNameChanged | ( | ) |
The auth action name has changed.
◆ buttons()
KAbstractConfigModule::Buttons KAbstractConfigModule::buttons | ( | ) | const |
Indicate which buttons will be used.
The return value is a value or'ed together from the Button enumeration type.
- See also
- ConfigModule::setButtons
Definition at line 37 of file kabstractconfigmodule.cpp.
◆ buttonsChanged()
Q_SIGNAL void KAbstractConfigModule::buttonsChanged | ( | ) |
Buttons to display changed.
◆ defaults()
|
virtual |
Sets the configuration to default values.
This method is called when the user clicks the "Default" button.
Reimplemented in KCModule.
Definition at line 92 of file kabstractconfigmodule.cpp.
◆ defaultsIndicatorsVisible()
bool KAbstractConfigModule::defaultsIndicatorsVisible | ( | ) | const |
- Returns
- defaultness indicator visibility
Definition at line 126 of file kabstractconfigmodule.cpp.
◆ defaultsIndicatorsVisibleChanged()
Q_SIGNAL void KAbstractConfigModule::defaultsIndicatorsVisibleChanged | ( | ) |
Emitted when kcm need to display indicators for field with non default value.
◆ description()
QString KAbstractConfigModule::description | ( | ) | const |
- Returns
- the description of the config module
Definition at line 62 of file kabstractconfigmodule.cpp.
◆ load()
|
virtual |
Load the configuration data into the module.
The load method sets the user interface elements of the module to reflect the current settings stored in the configuration files.
This method is invoked whenever the module should read its configuration (most of the times from a config file) and update the user interface. This happens when the user clicks the "Reset" button in the control center, to undo all of his changes and restore the currently valid settings. It is also called right after construction.
Reimplemented in KCModule.
Definition at line 82 of file kabstractconfigmodule.cpp.
◆ metaData()
KPluginMetaData KAbstractConfigModule::metaData | ( | ) | const |
Returns the metaData that was used when instantiating the plugin.
Definition at line 140 of file kabstractconfigmodule.cpp.
◆ name()
QString KAbstractConfigModule::name | ( | ) | const |
- Returns
- the name of the config module
Definition at line 57 of file kabstractconfigmodule.cpp.
◆ needsAuthorization()
bool KAbstractConfigModule::needsAuthorization | ( | ) | const |
- Returns
- true, if the authActionName is not empty
- See also
- setAuthActionName
Definition at line 52 of file kabstractconfigmodule.cpp.
◆ needsSave()
bool KAbstractConfigModule::needsSave | ( | ) | const |
True when the module has something changed and needs save.
Definition at line 106 of file kabstractconfigmodule.cpp.
◆ needsSaveChanged()
Q_SIGNAL void KAbstractConfigModule::needsSaveChanged | ( | ) |
Indicate that the state of the modules contents has changed.
This signal is emitted whenever the state of the configuration shown in the module changes. It allows the module container to keep track of unsaved changes.
◆ representsDefaults()
bool KAbstractConfigModule::representsDefaults | ( | ) | const |
True when the module state represents the default settings.
Definition at line 121 of file kabstractconfigmodule.cpp.
◆ representsDefaultsChanged()
Q_SIGNAL void KAbstractConfigModule::representsDefaultsChanged | ( | ) |
Indicate that the state of the modules contents has changed in a way that it might represents the defaults settings, or stopped representing them.
◆ save()
|
virtual |
The save method stores the config information as shown in the user interface in the config files.
This method is called when the user clicks "Apply" or "Ok".
Reimplemented in KCModule.
Definition at line 87 of file kabstractconfigmodule.cpp.
◆ setAuthActionName()
void KAbstractConfigModule::setAuthActionName | ( | const QString & | action | ) |
Set if the module's save() method requires authorization to be executed.
It will still have to execute the action itself using the KAuth library, so this method is not technically needed to perform the action, but using this method will ensure that hosting applications like System Settings or kcmshell behave correctly.
- Parameters
-
action the action that will be used by this ConfigModule
Definition at line 67 of file kabstractconfigmodule.cpp.
◆ setButtons()
void KAbstractConfigModule::setButtons | ( | const Buttons | btn | ) |
Sets the buttons to display.
Help: shows a "Help" button.
Default: shows a "Use Defaults" button.
Apply: shows an "Ok", "Apply" and "Cancel" button.
If Apply is not specified, kcmshell will show a "Close" button.
- See also
- ConfigModule::buttons
Definition at line 42 of file kabstractconfigmodule.cpp.
◆ setDefaultsIndicatorsVisible()
void KAbstractConfigModule::setDefaultsIndicatorsVisible | ( | bool | visible | ) |
Change defaultness indicator visibility.
- Parameters
-
visible
Definition at line 131 of file kabstractconfigmodule.cpp.
◆ setNeedsSave()
void KAbstractConfigModule::setNeedsSave | ( | bool | needs | ) |
Set this property to true when the user changes something in the module, signaling that a save (such as user pressing Ok or Apply) is needed.
Definition at line 96 of file kabstractconfigmodule.cpp.
◆ setRepresentsDefaults()
void KAbstractConfigModule::setRepresentsDefaults | ( | bool | defaults | ) |
Set this property to true when the user sets the state of the module to the default settings (e.g.
clicking Defaults would do nothing).
Definition at line 111 of file kabstractconfigmodule.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:53:48 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.