KAuthorized
#include <kauthorized.h>
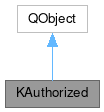
Public Types | |
enum | GenericAction { OPEN_WITH = 1 , EDITFILETYPE , OPTIONS_SHOW_TOOLBAR , SWITCH_APPLICATION_LANGUAGE , BOOKMARKS } |
enum | GenericRestriction { SHELL_ACCESS = 1 , GHNS , LINEEDIT_REVEAL_PASSWORD , LINEEDIT_TEXT_COMPLETION , MOVABLE_TOOLBARS , RUN_DESKTOP_FILES } |
Static Public Member Functions | |
static Q_INVOKABLE bool | authorize (const QString &action) |
static Q_INVOKABLE bool | authorize (GenericRestriction action) |
static Q_INVOKABLE bool | authorizeAction (const QString &action) |
static Q_INVOKABLE bool | authorizeAction (GenericAction action) |
static Q_INVOKABLE bool | authorizeControlModule (const QString &pluginId) |
static KAuthorized * | create (QQmlEngine *, QJSEngine *) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The functions in this namespace provide the core of the Kiosk action restriction system; the KIO and KXMLGui frameworks build on this.
The relevant settings are read from the application's KSharedConfig instance, so actions can be disabled on a per-application or global basis (by using the kdeglobals file).
Definition at line 31 of file kauthorized.h.
Member Enumeration Documentation
◆ GenericAction
- Since
- 5.88
Definition at line 56 of file kauthorized.h.
◆ GenericRestriction
The enum values lower cased represent the action that is authorized For example the SHELL_ACCESS value is converted to the "shell_access" string.
- Since
- 5.88
Definition at line 41 of file kauthorized.h.
Member Function Documentation
◆ authorize() [1/2]
|
static |
Returns whether the user is permitted to perform a certain action.
All settings are read from the "[KDE Action Restrictions]" group. For example, if kdeglobals contains
[KDE Action Restrictions][$i] shell_access=false
then
will return false
.
This method is intended for actions that do not necessarily have a one-to-one correspondence with a menu or toolbar item (ie: a QAction in a KXMLGui application). "shell_access" is an example of such a "generic" action.
The convention for actions like "File->New" is to prepend the action name with "action/", for example "action/file_new". This is what authorizeAction() does.
- Parameters
-
action The name of the action.
- Returns
true
if the action is authorized,false
otherwise.
- See also
- authorizeAction()
Definition at line 215 of file kauthorized.cpp.
◆ authorize() [2/2]
|
static |
Returns whether the user is permitted to perform a common action.
The enum values lower cased represent the action that is passed in to authorize(QString)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Since
- 5.88
Definition at line 230 of file kauthorized.cpp.
◆ authorizeAction() [1/2]
|
static |
Returns whether the user is permitted to perform a certain action.
This behaves like authorize(), except that "action/" is prepended to action
. So if kdeglobals contains
[KDE Action Restrictions][$i] action/file_new=false
then
will return false
.
KXMLGui-based applications should not normally need to call this function, as KActionCollection will do it automatically.
- Parameters
-
action The name of a QAction action.
- Returns
true
if the QAction is authorized,false
otherwise.
- Since
- 5.24
- See also
- authorize()
Definition at line 241 of file kauthorized.cpp.
◆ authorizeAction() [2/2]
|
static |
Overload to authorize common actions.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Since
- 5.88
Definition at line 254 of file kauthorized.cpp.
◆ authorizeControlModule()
|
static |
Returns whether the user is permitted to use a certain control module.
All settings are read from the "[KDE Control Module Restrictions]" group. For example, if kdeglobals contains
[KDE Control Module Restrictions][$i] kcm_desktop-settings=false
then
will return false
.
- Parameters
-
pluginId The desktop menu ID for the control module.
- Returns
true
if access to the module is authorized,false
otherwise.
Definition at line 264 of file kauthorized.cpp.
◆ create()
|
inlinestatic |
Definition at line 164 of file kauthorized.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 4 2025 12:06:01 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.