AbstractApplicationItem
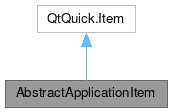
Properties | |
Item | background |
color | color |
Item | contentItem |
OverlayDrawer | contextDrawer |
bool | controlsVisible |
font | font |
Item | footer |
OverlayDrawer | globalDrawer |
KTAbstractApplicationHeader | header |
Locale | locale |
TMenuBar | menuBar |
alias | overlay |
Item | pageStack |
bool | wideScreen |
Public Member Functions | |
void | applicationWindow () |
void | hidePassiveNotification (index=0) |
void | showPassiveNotification (message, timeout, actionText, callBack) |
![]() | |
childAt (real x, real y) | |
bool | contains (point point) |
dumpItemTree () | |
forceActiveFocus () | |
forceActiveFocus (Qt::FocusReason reason) | |
bool | grabToImage (callback, targetSize) |
point | mapFromGlobal (real x, real y) |
point | mapFromItem (Item item, point p) |
point | mapFromItem (Item item, real x, real y) |
rect | mapFromItem (Item item, real x, real y, real width, real height) |
rect | mapFromItem (Item item, rect r) |
point | mapToGlobal (real x, real y) |
point | mapToItem (Item item, point p) |
point | mapToItem (Item item, real x, real y) |
rect | mapToItem (Item item, real x, real y, real width, real height) |
rect | mapToItem (Item item, rect r) |
nextItemInFocusChain (bool forward) | |
Detailed Description
An item that provides the features of AbstractApplicationWindow without the window itself.
This allows embedding into a larger application. Unless you need extra flexibility it is recommended to use ApplicationItem instead.
Example usage:
Definition at line 56 of file AbstractApplicationItem.qml.
Property Documentation
◆ background
|
read |
This property holds the background of the Application UI.
Definition at line 184 of file AbstractApplicationItem.qml.
◆ color
|
read |
This property holds the color for the background.
default: Kirigami.Theme.backgroundColor
Definition at line 180 of file AbstractApplicationItem.qml.
◆ contentItem
|
read |
This property holds the Item of the main part of the Application UI.
- Remarks
- This property is read-only
Definition at line 174 of file AbstractApplicationItem.qml.
◆ contextDrawer
|
read |
This property holds the drawer for context-dependent actions.
The drawer that will be opened by sliding from the right screen edge or by dragging the ActionButton to the left.
- Note
- It is recommended to use the ContextDrawer type here.
The contents of the context drawer should depend from what page is loaded in the main pageStack
Example usage:
Definition at line 169 of file AbstractApplicationItem.qml.
◆ controlsVisible
|
read |
This property sets whether the standard chrome of the app is visible.
These are the action button, the drawer handles and the application header.
default: true
Definition at line 109 of file AbstractApplicationItem.qml.
◆ font
|
read |
This property holds the font for this item.
default: Kirigami.Theme.defaultFont
Definition at line 71 of file AbstractApplicationItem.qml.
◆ footer
|
read |
This property holds an item that can be used as a footer for the application.
Definition at line 101 of file AbstractApplicationItem.qml.
◆ globalDrawer
|
read |
This property holds the drawer for global actions.
Thos drawer can be opened by sliding from the left screen edge or by dragging the ActionButton to the right.
- Note
- It is recommended to use the GlobalDrawer here.
Definition at line 119 of file AbstractApplicationItem.qml.
◆ header
|
read |
This property holds an item that can be used as a title for the application.
Scrolling the main page will make it taller or shorter (through the point of going away).
It's a behavior similar to the typical mobile web browser addressbar.
The minimum, preferred and maximum heights of the item can be controlled with
Layout.minimumHeight
: default is 0, i.e. hiddenLayout.preferredHeight
: default is Kirigami.Units.gridUnit * 1.6Layout.maximumHeight
: default is Kirigami.Units.gridUnit * 3
To achieve a titlebar that stays completely fixed, just set the 3 sizes as the same.
Definition at line 97 of file AbstractApplicationItem.qml.
◆ locale
|
read |
This property holds the locale for this item.
Definition at line 75 of file AbstractApplicationItem.qml.
◆ menuBar
|
read |
This property holds an item that can be used as a menuBar for the application.
Definition at line 79 of file AbstractApplicationItem.qml.
◆ overlay
|
read |
Definition at line 186 of file AbstractApplicationItem.qml.
◆ pageStack
|
read |
This property holds the stack used to allocate the pages and to manage the transitions between them.
Put a container here, such as QtQuick.Controls.StackView.
Definition at line 65 of file AbstractApplicationItem.qml.
◆ wideScreen
|
read |
This property tells us whether the application is in "widescreen" mode.
This is enabled on desktops or horizontal tablets.
- Note
- Different styles can have their own logic for deciding this.
Definition at line 127 of file AbstractApplicationItem.qml.
Member Function Documentation
◆ applicationWindow()
void AbstractApplicationItem::applicationWindow | ( | ) |
This property gets application windows object anywhere in the application.
- Returns
- a pointer to this item.
◆ hidePassiveNotification()
void AbstractApplicationItem::hidePassiveNotification | ( | index | = 0 | ) |
This function hides the passive notification at specified index, if any is shown.
- Parameters
-
index Index of the notification to hide. Default is 0 (oldest notification).
◆ showPassiveNotification()
void AbstractApplicationItem::showPassiveNotification | ( | message | , |
timeout | , | ||
actionText | , | ||
callBack | ) |
This function shows a little passive notification at the bottom of the app window lasting for few seconds, with an optional action button.
- Parameters
-
message The text message to be shown to the user. timeout How long to show the message: possible values: "short", "long" or the number of milliseconds actionText Text in the action button, if any. callBack A JavaScript function that will be executed when the user clicks the button.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:16 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.