KParts::PartManager
#include <KParts/PartManager>
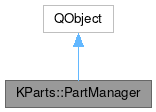
Public Types | |
enum | Reason { ReasonLeftClick = 100 , ReasonMidClick , ReasonRightClick , NoReason } |
enum | SelectionPolicy { Direct , TriState } |
Properties | |
bool | allowNestedParts |
bool | ignoreScrollBars |
SelectionPolicy | selectionPolicy |
![]() | |
objectName | |
Signals | |
void | activePartChanged (KParts::Part *newPart) |
void | partAdded (KParts::Part *part) |
void | partRemoved (KParts::Part *part) |
Public Member Functions | |
PartManager (QWidget *parent) | |
PartManager (QWidget *topLevel, QObject *parent) | |
short int | activationButtonMask () const |
virtual Part * | activePart () const |
virtual QWidget * | activeWidget () const |
void | addManagedTopLevelWidget (const QWidget *topLevel) |
virtual void | addPart (Part *part, bool setActive=true) |
bool | allowNestedParts () const |
bool | eventFilter (QObject *obj, QEvent *ev) override |
bool | ignoreScrollBars () const |
const QList< Part * > | parts () const |
int | reason () const |
void | removeManagedTopLevelWidget (const QWidget *topLevel) |
virtual void | removePart (Part *part) |
virtual void | replacePart (Part *oldPart, Part *newPart, bool setActive=true) |
SelectionPolicy | selectionPolicy () const |
void | setActivationButtonMask (short int buttonMask) |
virtual void | setActivePart (Part *part, QWidget *widget=nullptr) |
void | setAllowNestedParts (bool allow) |
void | setIgnoreScrollBars (bool ignore) |
void | setSelectionPolicy (SelectionPolicy policy) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Slots | |
void | slotManagedTopLevelWidgetDestroyed () |
void | slotObjectDestroyed () |
void | slotWidgetDestroyed () |
Protected Member Functions | |
void | setIgnoreExplictFocusRequests (bool) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
The part manager is an object which knows about a collection of parts (even nested ones) and handles activation/deactivation.
Applications that want to embed parts without merging GUIs only use a KParts::PartManager. Those who want to merge GUIs use a KParts::MainWindow for example, in addition to a part manager.
Parts know about the part manager to add nested parts to it. See also KParts::Part::manager() and KParts::Part::setManager().
Definition at line 36 of file partmanager.h.
Member Enumeration Documentation
◆ Reason
This extends QFocusEvent::Reason with the non-focus-event reasons for partmanager to activate a part.
To test for "any focusin reason", use < ReasonLeftClick NoReason usually means: explicit activation with setActivePart.
Definition at line 52 of file partmanager.h.
◆ SelectionPolicy
Selection policy. The default policy of a PartManager is Direct.
Definition at line 44 of file partmanager.h.
Property Documentation
◆ allowNestedParts
|
readwrite |
Definition at line 40 of file partmanager.h.
◆ ignoreScrollBars
|
readwrite |
Definition at line 41 of file partmanager.h.
◆ selectionPolicy
|
readwrite |
Definition at line 39 of file partmanager.h.
Constructor & Destructor Documentation
◆ PartManager() [1/2]
PartManager::PartManager | ( | QWidget * | parent | ) |
Constructs a part manager.
- Parameters
-
parent The toplevel widget (window / dialog) the partmanager should monitor for activation/selection events
Definition at line 90 of file partmanager.cpp.
◆ PartManager() [2/2]
Constructs a part manager.
- Parameters
-
topLevel The toplevel widget (window / dialog ) the partmanager should monitor for activation/selection events parent The parent QObject.
Definition at line 101 of file partmanager.cpp.
◆ ~PartManager()
|
override |
Definition at line 112 of file partmanager.cpp.
Member Function Documentation
◆ activationButtonMask()
short int PartManager::activationButtonMask | ( | ) | const |
- See also
- setActivationButtonMask
Definition at line 161 of file partmanager.cpp.
◆ activePart()
|
virtual |
Returns the active part.
Definition at line 428 of file partmanager.cpp.
◆ activePartChanged
|
signal |
Emitted when the active part has changed.
- See also
- setActivePart()
◆ activeWidget()
|
virtual |
Returns the active widget of the current active part (see activePart ).
Definition at line 433 of file partmanager.cpp.
◆ addManagedTopLevelWidget()
void PartManager::addManagedTopLevelWidget | ( | const QWidget * | topLevel | ) |
Adds the topLevel
widget to the list of managed toplevel widgets.
Usually a PartManager only listens for events (for activation/selection) for one toplevel widget (and its children) , the one specified in the constructor. Sometimes however (like for example when using the KDE dockwidget library) , it is necessary to extend this.
Definition at line 458 of file partmanager.cpp.
◆ addPart()
|
virtual |
Adds a part to the manager.
Sets it to the active part automatically if setActive
is true (default).
Definition at line 290 of file partmanager.cpp.
◆ allowNestedParts()
bool PartManager::allowNestedParts | ( | ) | const |
- See also
- setAllowNestedParts
Definition at line 141 of file partmanager.cpp.
◆ eventFilter()
Reimplemented from QObject.
Definition at line 166 of file partmanager.cpp.
◆ ignoreScrollBars()
bool PartManager::ignoreScrollBars | ( | ) | const |
- See also
- setIgnoreScrollBars
Definition at line 151 of file partmanager.cpp.
◆ partAdded
|
signal |
Emitted when a new part has been added.
- See also
- addPart()
◆ partRemoved
|
signal |
Emitted when a part has been removed.
- See also
- removePart()
◆ parts()
Returns the list of parts being managed by the partmanager.
Definition at line 453 of file partmanager.cpp.
◆ reason()
int PartManager::reason | ( | ) | const |
- Returns
- the reason for the last activePartChanged signal emitted.
- See also
- Reason
Definition at line 483 of file partmanager.cpp.
◆ removeManagedTopLevelWidget()
void PartManager::removeManagedTopLevelWidget | ( | const QWidget * | topLevel | ) |
Removes the topLevel
widget from the list of managed toplevel widgets.
- See also
- addManagedTopLevelWidget
Definition at line 472 of file partmanager.cpp.
◆ removePart()
|
virtual |
Removes a part from the manager (this does not delete the object) .
Sets the active part to 0 if part
is the activePart() .
Definition at line 326 of file partmanager.cpp.
◆ replacePart()
Replaces oldPart
with newPart
, and sets newPart
as active if setActive
is true.
This is an optimised version of removePart + addPart
Definition at line 344 of file partmanager.cpp.
◆ selectionPolicy()
PartManager::SelectionPolicy PartManager::selectionPolicy | ( | ) | const |
Returns the current selection policy.
Definition at line 131 of file partmanager.cpp.
◆ setActivationButtonMask()
void PartManager::setActivationButtonMask | ( | short int | buttonMask | ) |
Specifies which mouse buttons the partmanager should react upon.
By default it reacts on all mouse buttons (LMB/MMB/RMB).
- Parameters
-
buttonMask a combination of Qt::ButtonState values e.g. Qt::LeftButton | Qt::MiddleButton
Definition at line 156 of file partmanager.cpp.
◆ setActivePart()
Sets the active part.
The active part receives activation events.
widget
can be used to specify which widget was responsible for the activation. This is important if you have multiple views for a document/part , like in KOffice .
Definition at line 361 of file partmanager.cpp.
◆ setAllowNestedParts()
void PartManager::setAllowNestedParts | ( | bool | allow | ) |
Specifies whether the partmanager should handle/allow nested parts or not.
This is a property the shell has to set/specify. Per default we assume that the shell cannot handle nested parts. However in case of a KOffice shell for example we allow nested parts. A Part is nested (a child part) if its parent object inherits KParts::Part. If a child part is activated and nested parts are not allowed/handled, then the top parent part in the tree is activated.
Definition at line 136 of file partmanager.cpp.
◆ setIgnoreExplictFocusRequests()
|
protected |
Sets whether the PartManager ignores explicit set focus requests from the part.
By default this option is set to false. Set it to true to prevent the part from sending explicit set focus requests to the client application.
- Since
- 4.10
Definition at line 488 of file partmanager.cpp.
◆ setIgnoreScrollBars()
void PartManager::setIgnoreScrollBars | ( | bool | ignore | ) |
Specifies whether the partmanager should ignore mouse click events for scrollbars or not.
If the partmanager ignores them, then clicking on the scrollbars of a non-active/non-selected part will not change the selection or activation state.
The default value is false (read: scrollbars are NOT ignored).
Definition at line 146 of file partmanager.cpp.
◆ setSelectionPolicy()
void PartManager::setSelectionPolicy | ( | SelectionPolicy | policy | ) |
Sets the selection policy of the partmanager.
Definition at line 126 of file partmanager.cpp.
◆ slotManagedTopLevelWidgetDestroyed
|
protectedslot |
Definition at line 477 of file partmanager.cpp.
◆ slotObjectDestroyed
|
protectedslot |
Removes a part when it is destroyed.
Definition at line 438 of file partmanager.cpp.
◆ slotWidgetDestroyed
|
protectedslot |
Definition at line 444 of file partmanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:56:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.