LineChart
#include <LineChart.h>
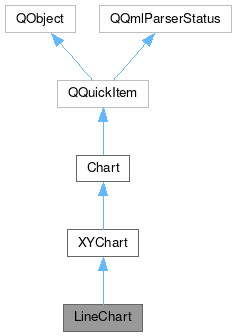
Properties | |
ChartDataSource * | fillColorSource |
qreal | fillOpacity |
bool | interpolate |
qreal | lineWidth |
QQmlComponent * | pointDelegate |
![]() | |
Direction | direction |
bool | stacked |
RangeGroup * | xRange |
RangeGroup * | yRange |
![]() | |
ChartDataSource * | colorSource |
int | highlight |
IndexingMode | indexingMode |
ChartDataSource * | nameSource |
ChartDataSource * | shortNameSource |
QQmlListProperty< ChartDataSource > | valueSources |
![]() | |
activeFocus | |
activeFocusOnTab | |
antialiasing | |
baselineOffset | |
childrenRect | |
clip | |
containmentMask | |
enabled | |
focus | |
height | |
implicitHeight | |
implicitWidth | |
opacity | |
parent | |
rotation | |
scale | |
smooth | |
state | |
transformOrigin | |
visible | |
width | |
x | |
y | |
z | |
![]() | |
objectName | |
Public Member Functions | |
LineChart (QQuickItem *parent=nullptr) | |
ChartDataSource * | fillColorSource () const |
Q_SIGNAL void | fillColorSourceChanged () |
qreal | fillOpacity () const |
Q_SIGNAL void | fillOpacityChanged () |
bool | interpolate () const |
Q_SIGNAL void | interpolateChanged () |
qreal | lineWidth () const |
Q_SIGNAL void | lineWidthChanged () |
QQmlComponent * | pointDelegate () const |
Q_SIGNAL void | pointDelegateChanged () |
void | setFillColorSource (ChartDataSource *newFillColorSource) |
void | setFillOpacity (qreal opacity) |
void | setInterpolate (bool newInterpolate) |
void | setLineWidth (qreal width) |
void | setPointDelegate (QQmlComponent *newPointDelegate) |
![]() | |
XYChart (QQuickItem *parent=nullptr) | |
~XYChart () override=default | |
ComputedRange | computedRange () const |
Q_SIGNAL void | computedRangeChanged () |
virtual XYChart::Direction | direction () const |
Q_SIGNAL void | directionChanged () |
virtual void | setDirection (XYChart::Direction newDirection) |
void | setStacked (bool newStacked) |
bool | stacked () const |
Q_SIGNAL void | stackedChanged () |
virtual RangeGroup * | xRange () const |
virtual RangeGroup * | yRange () const |
![]() | |
Chart (QQuickItem *parent=nullptr) | |
ChartDataSource * | colorSource () const |
Q_SIGNAL void | colorSourceChanged () |
Q_SIGNAL void | dataChanged () |
int | highlight () const |
Q_SIGNAL void | highlightChanged () |
IndexingMode | indexingMode () const |
Q_SIGNAL void | indexingModeChanged () |
Q_INVOKABLE void | insertValueSource (int index, ChartDataSource *source) |
ChartDataSource * | nameSource () const |
Q_SIGNAL void | nameSourceChanged () |
Q_INVOKABLE void | removeValueSource (int index) |
Q_INVOKABLE void | removeValueSource (QObject *source) |
void | resetHighlight () |
void | setColorSource (ChartDataSource *colorSource) |
void | setHighlight (int highlight) |
void | setIndexingMode (IndexingMode newIndexingMode) |
void | setNameSource (ChartDataSource *nameSource) |
void | setShortNameSource (ChartDataSource *shortNameSource) |
ChartDataSource * | shortNameSource () const |
Q_SIGNAL void | shortNameSourceChanged () |
QList< ChartDataSource * > | valueSources () const |
Q_SIGNAL void | valueSourcesChanged () |
DataSourcesProperty | valueSourcesProperty () |
![]() | |
QQuickItem (QQuickItem *parent) | |
Qt::MouseButtons | acceptedMouseButtons () const const |
bool | acceptHoverEvents () const const |
bool | acceptTouchEvents () const const |
void | activeFocusChanged (bool) |
bool | activeFocusOnTab () const const |
void | activeFocusOnTabChanged (bool) |
bool | antialiasing () const const |
void | antialiasingChanged (bool) |
qreal | baselineOffset () const const |
void | baselineOffsetChanged (qreal) |
QBindable< qreal > | bindableHeight () |
QBindable< qreal > | bindableWidth () |
QBindable< qreal > | bindableX () |
QBindable< qreal > | bindableY () |
virtual QRectF | boundingRect () const const |
QQuickItem * | childAt (qreal x, qreal y) const const |
QList< QQuickItem * > | childItems () const const |
QRectF | childrenRect () |
void | childrenRectChanged (const QRectF &) |
bool | clip () const const |
void | clipChanged (bool) |
virtual QRectF | clipRect () const const |
QObject * | containmentMask () const const |
void | containmentMaskChanged () |
virtual bool | contains (const QPointF &point) const const |
QCursor | cursor () const const |
void | dumpItemTree () const const |
void | enabledChanged () |
void | ensurePolished () |
bool | filtersChildMouseEvents () const const |
Flags | flags () const const |
void | focusChanged (bool) |
void | forceActiveFocus () |
void | forceActiveFocus (Qt::FocusReason reason) |
void | grabMouse () |
QSharedPointer< QQuickItemGrabResult > | grabToImage (const QSize &targetSize) |
void | grabTouchPoints (const QList< int > &ids) |
bool | hasActiveFocus () const const |
bool | hasFocus () const const |
qreal | height () const const |
void | heightChanged () |
qreal | implicitHeight () const const |
void | implicitHeightChanged () |
qreal | implicitWidth () const const |
void | implicitWidthChanged () |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
bool | isAncestorOf (const QQuickItem *child) const const |
bool | isEnabled () const const |
bool | isFocusScope () const const |
virtual bool | isTextureProvider () const const |
bool | isVisible () const const |
bool | keepMouseGrab () const const |
bool | keepTouchGrab () const const |
QPointF | mapFromGlobal (const QPointF &point) const const |
QPointF | mapFromItem (const QQuickItem *item, const QPointF &point) const const |
QPointF | mapFromScene (const QPointF &point) const const |
QRectF | mapRectFromItem (const QQuickItem *item, const QRectF &rect) const const |
QRectF | mapRectFromScene (const QRectF &rect) const const |
QRectF | mapRectToItem (const QQuickItem *item, const QRectF &rect) const const |
QRectF | mapRectToScene (const QRectF &rect) const const |
QPointF | mapToGlobal (const QPointF &point) const const |
QPointF | mapToItem (const QQuickItem *item, const QPointF &point) const const |
QPointF | mapToScene (const QPointF &point) const const |
QQuickItem * | nextItemInFocusChain (bool forward) |
qreal | opacity () const const |
void | opacityChanged () |
void | parentChanged (QQuickItem *) |
QQuickItem * | parentItem () const const |
void | polish () |
void | resetAntialiasing () |
void | resetHeight () |
void | resetWidth () |
qreal | rotation () const const |
void | rotationChanged () |
qreal | scale () const const |
void | scaleChanged () |
QQuickItem * | scopedFocusItem () const const |
void | setAcceptedMouseButtons (Qt::MouseButtons buttons) |
void | setAcceptHoverEvents (bool enabled) |
void | setAcceptTouchEvents (bool enabled) |
void | setActiveFocusOnTab (bool) |
void | setAntialiasing (bool) |
void | setBaselineOffset (qreal) |
void | setClip (bool) |
void | setContainmentMask (QObject *mask) |
void | setCursor (const QCursor &cursor) |
void | setEnabled (bool) |
void | setFiltersChildMouseEvents (bool filter) |
void | setFlag (Flag flag, bool enabled) |
void | setFlags (Flags flags) |
void | setFocus (bool focus, Qt::FocusReason reason) |
void | setFocus (bool) |
void | setHeight (qreal) |
void | setImplicitHeight (qreal) |
void | setImplicitWidth (qreal) |
void | setKeepMouseGrab (bool keep) |
void | setKeepTouchGrab (bool keep) |
void | setOpacity (qreal) |
void | setParentItem (QQuickItem *parent) |
void | setRotation (qreal) |
void | setScale (qreal) |
void | setSize (const QSizeF &size) |
void | setSmooth (bool) |
void | setState (const QString &) |
void | setTransformOrigin (TransformOrigin) |
void | setVisible (bool) |
void | setWidth (qreal) |
void | setX (qreal) |
void | setY (qreal) |
void | setZ (qreal) |
QSizeF | size () const const |
bool | smooth () const const |
void | smoothChanged (bool) |
void | stackAfter (const QQuickItem *sibling) |
void | stackBefore (const QQuickItem *sibling) |
QString | state () const const |
void | stateChanged (const QString &) |
virtual QSGTextureProvider * | textureProvider () const const |
TransformOrigin | transformOrigin () const const |
void | transformOriginChanged (TransformOrigin) |
void | ungrabMouse () |
void | ungrabTouchPoints () |
void | unsetCursor () |
void | update () |
QQuickItem * | viewportItem () const const |
void | visibleChanged () |
qreal | width () const const |
void | widthChanged () |
QQuickWindow * | window () const const |
void | windowChanged (QQuickWindow *window) |
qreal | x () const const |
void | xChanged () |
qreal | y () const const |
void | yChanged () |
qreal | z () const const |
void | zChanged () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static LineChartAttached * | qmlAttachedProperties (QObject *object) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
void | geometryChange (const QRectF &newGeometry, const QRectF &oldGeometry) override |
void | onDataChanged () override |
QSGNode * | updatePaintNode (QSGNode *node, QQuickItem::UpdatePaintNodeData *data) override |
void | updatePolish () override |
![]() | |
void | setComputedRange (ComputedRange range) |
virtual void | updateComputedRange () |
![]() | |
void | componentComplete () override |
QColor | desaturate (const QColor &input) |
![]() | |
virtual bool | childMouseEventFilter (QQuickItem *item, QEvent *event) |
virtual void | classBegin () override |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual bool | event (QEvent *ev) override |
virtual void | focusInEvent (QFocusEvent *) |
virtual void | focusOutEvent (QFocusEvent *) |
bool | heightValid () const const |
virtual void | hoverEnterEvent (QHoverEvent *event) |
virtual void | hoverLeaveEvent (QHoverEvent *event) |
virtual void | hoverMoveEvent (QHoverEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
bool | isComponentComplete () const const |
virtual void | itemChange (ItemChange change, const ItemChangeData &value) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | mouseUngrabEvent () |
virtual void | releaseResources () |
virtual void | touchEvent (QTouchEvent *event) |
virtual void | touchUngrabEvent () |
void | updateInputMethod (Qt::InputMethodQueries queries) |
virtual QSGNode * | updatePaintNode (QSGNode *oldNode, UpdatePaintNodeData *updatePaintNodeData) |
virtual void | wheelEvent (QWheelEvent *event) |
bool | widthValid () const const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
enum class | Direction { ZeroAtStart , ZeroAtEnd } |
![]() | |
using | DataSourcesProperty = QQmlListProperty<ChartDataSource> |
enum | IndexingMode { IndexSourceValues = 1 , IndexEachSource , IndexAllValues } |
![]() | |
enum | Flag |
enum | ItemChange |
enum | TransformOrigin |
![]() | |
Bottom | |
BottomLeft | |
BottomRight | |
Center | |
typedef | Flags |
ItemAcceptsDrops | |
ItemAcceptsInputMethod | |
ItemActiveFocusHasChanged | |
ItemAntialiasingHasChanged | |
ItemChildAddedChange | |
ItemChildRemovedChange | |
ItemClipsChildrenToShape | |
ItemDevicePixelRatioHasChanged | |
ItemEnabledHasChanged | |
ItemHasContents | |
ItemIsFocusScope | |
ItemIsViewport | |
ItemObservesViewport | |
ItemOpacityHasChanged | |
ItemParentHasChanged | |
ItemRotationHasChanged | |
ItemSceneChange | |
ItemVisibleHasChanged | |
Left | |
Right | |
Top | |
TopLeft | |
TopRight | |
![]() | |
typedef | QObjectList |
Detailed Description
A line chart.
Usage example
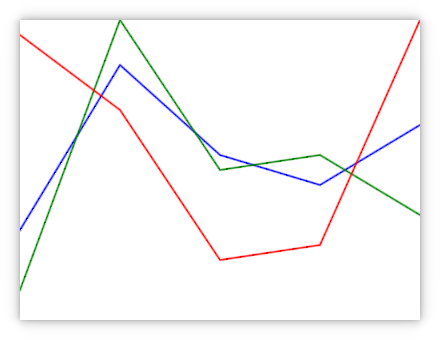
Definition at line 80 of file LineChart.h.
Property Documentation
◆ fillColorSource
|
readwrite |
A data source that supplies color values for the line charts' fill area.
If this is not set (the default), the normal color source will be used, with the fillOpacity used as its opacity.
Definition at line 119 of file LineChart.h.
◆ fillOpacity
|
readwrite |
The opacity of the area below a line.
The default is 0.0. Note that if fillColorSource is set, this value is ignored.
Definition at line 109 of file LineChart.h.
◆ interpolate
|
readwrite |
Interpolate the values in the chart so that the lines become smoothed.
Definition at line 92 of file LineChart.h.
◆ lineWidth
|
readwrite |
The width of a line in the chart.
Definition at line 99 of file LineChart.h.
◆ pointDelegate
|
readwrite |
A delegate that will be placed at each line chart point.
When this is not null, the specified component will be used to instantiate an object for each point in the chart. These objects will then be placed centered at positions corresponding to the points on the chart. Each instance will have access to the attached properties of LineChartAttached through LineChart attached object.
- Note
- The component assigned to this property is expected to create a QQuickItem, since the created object needs to be positioned.
Definition at line 135 of file LineChart.h.
Constructor & Destructor Documentation
◆ LineChart()
|
explicit |
Definition at line 106 of file LineChart.cpp.
Member Function Documentation
◆ fillColorSource()
ChartDataSource * LineChart::fillColorSource | ( | ) | const |
Definition at line 159 of file LineChart.cpp.
◆ fillOpacity()
qreal LineChart::fillOpacity | ( | ) | const |
Definition at line 121 of file LineChart.cpp.
◆ geometryChange()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 325 of file LineChart.cpp.
◆ interpolate()
bool LineChart::interpolate | ( | ) | const |
Definition at line 111 of file LineChart.cpp.
◆ lineWidth()
qreal LineChart::lineWidth | ( | ) | const |
Definition at line 116 of file LineChart.cpp.
◆ onDataChanged()
|
overrideprotectedvirtual |
Called when the data of a value source changes.
This method should be reimplemented by subclasses. It is called whenever the data of one of the value sources changes. Subclasses should use this to make sure that they update whatever internal state they use for rendering, then call update() to schedule rendering the item.
Implements Chart.
Definition at line 319 of file LineChart.cpp.
◆ pointDelegate()
QQmlComponent * LineChart::pointDelegate | ( | ) | const |
Definition at line 175 of file LineChart.cpp.
◆ qmlAttachedProperties()
|
inlinestatic |
Definition at line 140 of file LineChart.h.
◆ setFillColorSource()
void LineChart::setFillColorSource | ( | ChartDataSource * | newFillColorSource | ) |
Definition at line 164 of file LineChart.cpp.
◆ setFillOpacity()
void LineChart::setFillOpacity | ( | qreal | opacity | ) |
Definition at line 148 of file LineChart.cpp.
◆ setInterpolate()
void LineChart::setInterpolate | ( | bool | newInterpolate | ) |
Definition at line 126 of file LineChart.cpp.
◆ setLineWidth()
void LineChart::setLineWidth | ( | qreal | width | ) |
Definition at line 137 of file LineChart.cpp.
◆ setPointDelegate()
void LineChart::setPointDelegate | ( | QQmlComponent * | newPointDelegate | ) |
Definition at line 180 of file LineChart.cpp.
◆ updatePaintNode()
|
overrideprotected |
Definition at line 271 of file LineChart.cpp.
◆ updatePolish()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 195 of file LineChart.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:53:38 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.