PieChart
#include <PieChart.h>
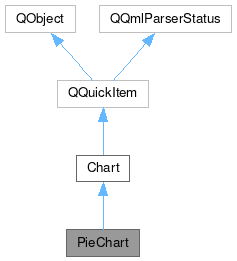
Properties | |
QColor | backgroundColor |
bool | filled |
qreal | fromAngle |
RangeGroup * | range |
bool | smoothEnds |
qreal | spacing |
qreal | thickness |
qreal | toAngle |
![]() | |
ChartDataSource * | colorSource |
int | highlight |
IndexingMode | indexingMode |
ChartDataSource * | nameSource |
ChartDataSource * | shortNameSource |
QQmlListProperty< ChartDataSource > | valueSources |
![]() | |
activeFocus | |
activeFocusOnTab | |
antialiasing | |
baselineOffset | |
childrenRect | |
clip | |
containmentMask | |
enabled | |
focus | |
height | |
implicitHeight | |
implicitWidth | |
opacity | |
parent | |
rotation | |
scale | |
smooth | |
state | |
transformOrigin | |
visible | |
width | |
x | |
y | |
z | |
![]() | |
objectName | |
Public Member Functions | |
PieChart (QQuickItem *parent=nullptr) | |
QColor | backgroundColor () const |
Q_SIGNAL void | backgroundColorChanged () |
bool | filled () const |
Q_SIGNAL void | filledChanged () |
qreal | fromAngle () const |
Q_SIGNAL void | fromAngleChanged () |
RangeGroup * | range () const |
void | setBackgroundColor (const QColor &color) |
void | setFilled (bool newFilled) |
void | setFromAngle (qreal newFromAngle) |
void | setSmoothEnds (bool newSmoothEnds) |
void | setSpacing (qreal newSpacing) |
void | setThickness (qreal newThickness) |
void | setToAngle (qreal newToAngle) |
bool | smoothEnds () const |
Q_SIGNAL void | smoothEndsChanged () |
qreal | spacing () const |
Q_SIGNAL void | spacingChanged () |
qreal | thickness () const |
Q_SIGNAL void | thicknessChanged () |
qreal | toAngle () const |
Q_SIGNAL void | toAngleChanged () |
![]() | |
Chart (QQuickItem *parent=nullptr) | |
ChartDataSource * | colorSource () const |
Q_SIGNAL void | colorSourceChanged () |
Q_SIGNAL void | dataChanged () |
int | highlight () const |
Q_SIGNAL void | highlightChanged () |
IndexingMode | indexingMode () const |
Q_SIGNAL void | indexingModeChanged () |
Q_INVOKABLE void | insertValueSource (int index, ChartDataSource *source) |
ChartDataSource * | nameSource () const |
Q_SIGNAL void | nameSourceChanged () |
Q_INVOKABLE void | removeValueSource (int index) |
Q_INVOKABLE void | removeValueSource (QObject *source) |
void | resetHighlight () |
void | setColorSource (ChartDataSource *colorSource) |
void | setHighlight (int highlight) |
void | setIndexingMode (IndexingMode newIndexingMode) |
void | setNameSource (ChartDataSource *nameSource) |
void | setShortNameSource (ChartDataSource *shortNameSource) |
ChartDataSource * | shortNameSource () const |
Q_SIGNAL void | shortNameSourceChanged () |
QList< ChartDataSource * > | valueSources () const |
Q_SIGNAL void | valueSourcesChanged () |
DataSourcesProperty | valueSourcesProperty () |
![]() | |
QQuickItem (QQuickItem *parent) | |
Qt::MouseButtons | acceptedMouseButtons () const const |
bool | acceptHoverEvents () const const |
bool | acceptTouchEvents () const const |
void | activeFocusChanged (bool) |
bool | activeFocusOnTab () const const |
void | activeFocusOnTabChanged (bool) |
bool | antialiasing () const const |
void | antialiasingChanged (bool) |
qreal | baselineOffset () const const |
void | baselineOffsetChanged (qreal) |
QBindable< qreal > | bindableHeight () |
QBindable< qreal > | bindableWidth () |
QBindable< qreal > | bindableX () |
QBindable< qreal > | bindableY () |
virtual QRectF | boundingRect () const const |
QQuickItem * | childAt (qreal x, qreal y) const const |
QList< QQuickItem * > | childItems () const const |
QRectF | childrenRect () |
void | childrenRectChanged (const QRectF &) |
bool | clip () const const |
void | clipChanged (bool) |
virtual QRectF | clipRect () const const |
QObject * | containmentMask () const const |
void | containmentMaskChanged () |
virtual bool | contains (const QPointF &point) const const |
QCursor | cursor () const const |
void | dumpItemTree () const const |
void | enabledChanged () |
void | ensurePolished () |
bool | filtersChildMouseEvents () const const |
Flags | flags () const const |
void | focusChanged (bool) |
void | forceActiveFocus () |
void | forceActiveFocus (Qt::FocusReason reason) |
void | grabMouse () |
QSharedPointer< QQuickItemGrabResult > | grabToImage (const QSize &targetSize) |
void | grabTouchPoints (const QList< int > &ids) |
bool | hasActiveFocus () const const |
bool | hasFocus () const const |
qreal | height () const const |
void | heightChanged () |
qreal | implicitHeight () const const |
void | implicitHeightChanged () |
qreal | implicitWidth () const const |
void | implicitWidthChanged () |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
bool | isAncestorOf (const QQuickItem *child) const const |
bool | isEnabled () const const |
bool | isFocusScope () const const |
virtual bool | isTextureProvider () const const |
bool | isVisible () const const |
bool | keepMouseGrab () const const |
bool | keepTouchGrab () const const |
QPointF | mapFromGlobal (const QPointF &point) const const |
QPointF | mapFromItem (const QQuickItem *item, const QPointF &point) const const |
QPointF | mapFromScene (const QPointF &point) const const |
QRectF | mapRectFromItem (const QQuickItem *item, const QRectF &rect) const const |
QRectF | mapRectFromScene (const QRectF &rect) const const |
QRectF | mapRectToItem (const QQuickItem *item, const QRectF &rect) const const |
QRectF | mapRectToScene (const QRectF &rect) const const |
QPointF | mapToGlobal (const QPointF &point) const const |
QPointF | mapToItem (const QQuickItem *item, const QPointF &point) const const |
QPointF | mapToScene (const QPointF &point) const const |
QQuickItem * | nextItemInFocusChain (bool forward) |
qreal | opacity () const const |
void | opacityChanged () |
void | parentChanged (QQuickItem *) |
QQuickItem * | parentItem () const const |
void | polish () |
void | resetAntialiasing () |
void | resetHeight () |
void | resetWidth () |
qreal | rotation () const const |
void | rotationChanged () |
qreal | scale () const const |
void | scaleChanged () |
QQuickItem * | scopedFocusItem () const const |
void | setAcceptedMouseButtons (Qt::MouseButtons buttons) |
void | setAcceptHoverEvents (bool enabled) |
void | setAcceptTouchEvents (bool enabled) |
void | setActiveFocusOnTab (bool) |
void | setAntialiasing (bool) |
void | setBaselineOffset (qreal) |
void | setClip (bool) |
void | setContainmentMask (QObject *mask) |
void | setCursor (const QCursor &cursor) |
void | setEnabled (bool) |
void | setFiltersChildMouseEvents (bool filter) |
void | setFlag (Flag flag, bool enabled) |
void | setFlags (Flags flags) |
void | setFocus (bool focus, Qt::FocusReason reason) |
void | setFocus (bool) |
void | setHeight (qreal) |
void | setImplicitHeight (qreal) |
void | setImplicitWidth (qreal) |
void | setKeepMouseGrab (bool keep) |
void | setKeepTouchGrab (bool keep) |
void | setOpacity (qreal) |
void | setParentItem (QQuickItem *parent) |
void | setRotation (qreal) |
void | setScale (qreal) |
void | setSize (const QSizeF &size) |
void | setSmooth (bool) |
void | setState (const QString &) |
void | setTransformOrigin (TransformOrigin) |
void | setVisible (bool) |
void | setWidth (qreal) |
void | setX (qreal) |
void | setY (qreal) |
void | setZ (qreal) |
QSizeF | size () const const |
bool | smooth () const const |
void | smoothChanged (bool) |
void | stackAfter (const QQuickItem *sibling) |
void | stackBefore (const QQuickItem *sibling) |
QString | state () const const |
void | stateChanged (const QString &) |
virtual QSGTextureProvider * | textureProvider () const const |
TransformOrigin | transformOrigin () const const |
void | transformOriginChanged (TransformOrigin) |
void | ungrabMouse () |
void | ungrabTouchPoints () |
void | unsetCursor () |
void | update () |
QQuickItem * | viewportItem () const const |
void | visibleChanged () |
qreal | width () const const |
void | widthChanged () |
QQuickWindow * | window () const const |
void | windowChanged (QQuickWindow *window) |
qreal | x () const const |
void | xChanged () |
qreal | y () const const |
void | yChanged () |
qreal | z () const const |
void | zChanged () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() |
Protected Member Functions | |
void | onDataChanged () override |
QSGNode * | updatePaintNode (QSGNode *node, UpdatePaintNodeData *data) override |
![]() | |
void | componentComplete () override |
QColor | desaturate (const QColor &input) |
![]() | |
virtual bool | childMouseEventFilter (QQuickItem *item, QEvent *event) |
virtual void | classBegin () override |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual bool | event (QEvent *ev) override |
virtual void | focusInEvent (QFocusEvent *) |
virtual void | focusOutEvent (QFocusEvent *) |
virtual void | geometryChange (const QRectF &newGeometry, const QRectF &oldGeometry) |
bool | heightValid () const const |
virtual void | hoverEnterEvent (QHoverEvent *event) |
virtual void | hoverLeaveEvent (QHoverEvent *event) |
virtual void | hoverMoveEvent (QHoverEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
bool | isComponentComplete () const const |
virtual void | itemChange (ItemChange change, const ItemChangeData &value) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | mouseUngrabEvent () |
virtual void | releaseResources () |
virtual void | touchEvent (QTouchEvent *event) |
virtual void | touchUngrabEvent () |
void | updateInputMethod (Qt::InputMethodQueries queries) |
virtual void | updatePolish () |
virtual void | wheelEvent (QWheelEvent *event) |
bool | widthValid () const const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
An item to render a pie chart.
This item will render a Pie chart based on the valueSources supplied to it. By default it will set indexingMode to IndexSourceValues, meaning that it will use the individual values of the valueSources to render sections of the chart.
Usage example
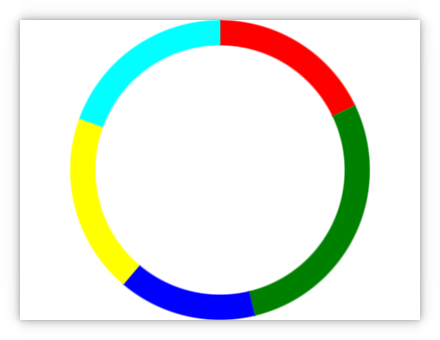
Multiple Value Sources
Multiple valueSources are rendered as consecutive pie charts in the same item. The first valueSource will become the outermost circle and consecutive valueSources will be rendered as continuously smaller circles. When rendering multiple value sources, filled will only affect the last value source. All other value sources will be rendered according to thickness, with spacing amount of space between them.
Definition at line 48 of file PieChart.h.
Property Documentation
◆ backgroundColor
|
readwrite |
Sets a colour to use to fill remaining space on the pie.
The default is transparent.
Definition at line 99 of file PieChart.h.
◆ filled
|
readwrite |
Whether to use a filled pie or not.
If true, the last pie rendered will be rendered as a filled circle. The default is false.
Definition at line 72 of file PieChart.h.
◆ fromAngle
|
readwrite |
The starting angle of the arc used for the entire pie.
When set, instead of rendering a full circle, the pie will be rendered as an arc. The default is 0.
Definition at line 109 of file PieChart.h.
◆ range
|
read |
The range of values to display in this PieChart.
When set to "automatic", the values will be divided across the entire chart.
- See also
- RangeGroup
Definition at line 64 of file PieChart.h.
◆ smoothEnds
|
readwrite |
Smooth the ends of pie sections.
When true, this will try to smooth the ends of sections. This works best when filled is false. The default is false.
Definition at line 130 of file PieChart.h.
◆ spacing
|
readwrite |
The amount of spacing between pies when rendering multiple value sources.
The default is 0.
Definition at line 90 of file PieChart.h.
◆ thickness
|
readwrite |
The thickness of an individual pie, in pixels.
If filled is set, this is ignored for the last pie. The default is 10px.
Definition at line 81 of file PieChart.h.
◆ toAngle
|
readwrite |
The end angle of the arc used for the entire pie.
When set, instead of rendering a full circle, the pie will be rendered as an arc. The default is 360.
Definition at line 118 of file PieChart.h.
Constructor & Destructor Documentation
◆ PieChart()
|
explicit |
Definition at line 17 of file PieChart.cpp.
Member Function Documentation
◆ backgroundColor()
QColor PieChart::backgroundColor | ( | ) | const |
Definition at line 78 of file PieChart.cpp.
◆ filled()
bool PieChart::filled | ( | ) | const |
Definition at line 30 of file PieChart.cpp.
◆ fromAngle()
qreal PieChart::fromAngle | ( | ) | const |
Definition at line 93 of file PieChart.cpp.
◆ onDataChanged()
|
overrideprotectedvirtual |
◆ range()
RangeGroup * PieChart::range | ( | ) | const |
Definition at line 25 of file PieChart.cpp.
◆ setBackgroundColor()
void PieChart::setBackgroundColor | ( | const QColor & | color | ) |
Definition at line 83 of file PieChart.cpp.
◆ setFilled()
void PieChart::setFilled | ( | bool | newFilled | ) |
Definition at line 35 of file PieChart.cpp.
◆ setFromAngle()
void PieChart::setFromAngle | ( | qreal | newFromAngle | ) |
Definition at line 98 of file PieChart.cpp.
◆ setSmoothEnds()
void PieChart::setSmoothEnds | ( | bool | newSmoothEnds | ) |
Definition at line 130 of file PieChart.cpp.
◆ setSpacing()
void PieChart::setSpacing | ( | qreal | newSpacing | ) |
Definition at line 67 of file PieChart.cpp.
◆ setThickness()
void PieChart::setThickness | ( | qreal | newThickness | ) |
Definition at line 51 of file PieChart.cpp.
◆ setToAngle()
void PieChart::setToAngle | ( | qreal | newToAngle | ) |
Definition at line 114 of file PieChart.cpp.
◆ smoothEnds()
bool PieChart::smoothEnds | ( | ) | const |
Definition at line 125 of file PieChart.cpp.
◆ spacing()
qreal PieChart::spacing | ( | ) | const |
Definition at line 62 of file PieChart.cpp.
◆ thickness()
qreal PieChart::thickness | ( | ) | const |
Definition at line 46 of file PieChart.cpp.
◆ toAngle()
qreal PieChart::toAngle | ( | ) | const |
Definition at line 109 of file PieChart.cpp.
◆ updatePaintNode()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Reimplemented from QQuickItem.
Definition at line 141 of file PieChart.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 17:01:23 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.