NetworkManager::AccessPoint
#include <accesspoint.h>
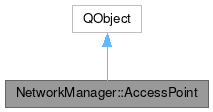
Public Types | |
typedef QFlags< Capability > | Capabilities |
enum | Capability { None = 0x0 , Privacy = 0x1 } |
typedef QList< Ptr > | List |
enum | OperationMode { Unknown = 0 , Adhoc , Infra , ApMode } |
typedef QSharedPointer< AccessPoint > | Ptr |
enum | WpaFlag { PairWep40 = 0x1 , PairWep104 = 0x2 , PairTkip = 0x4 , PairCcmp = 0x8 , GroupWep40 = 0x10 , GroupWep104 = 0x20 , GroupTkip = 0x40 , GroupCcmp = 0x80 , KeyMgmtPsk = 0x100 , KeyMgmt8021x = 0x200 , KeyMgmtSAE = 0x400 , KeyMgmtOWE = 0x800 , KeyMgmtOWETM = 0x1000 , KeyMgmtEapSuiteB192 = 0x2000 } |
typedef QFlags< WpaFlag > | WpaFlags |
Signals | |
void | bandwidthChanged (uint bandwidth) |
void | bitRateChanged (int bitrate) |
void | capabilitiesChanged (AccessPoint::Capabilities caps) |
void | frequencyChanged (uint frequency) |
void | lastSeenChanged (int lastSeen) |
void | rsnFlagsChanged (AccessPoint::WpaFlags flags) |
void | signalStrengthChanged (int strength) |
void | ssidChanged (const QString &ssid) |
void | wpaFlagsChanged (AccessPoint::WpaFlags flags) |
Public Member Functions | |
AccessPoint (const QString &path, QObject *parent=nullptr) | |
uint | bandwidth () const |
Capabilities | capabilities () const |
uint | frequency () const |
QString | hardwareAddress () const |
int | lastSeen () const |
uint | maxBitRate () const |
OperationMode | mode () const |
QByteArray | rawSsid () const |
AccessPoint::WpaFlags | rsnFlags () const |
int | signalStrength () const |
QString | ssid () const |
QString | uni () const |
AccessPoint::WpaFlags | wpaFlags () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static OperationMode | convertOperationMode (uint mode) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Represents an access point.
Definition at line 27 of file accesspoint.h.
Member Typedef Documentation
◆ Capabilities
Definition at line 68 of file accesspoint.h.
◆ List
typedef QList<Ptr> NetworkManager::AccessPoint::List |
Definition at line 32 of file accesspoint.h.
◆ Ptr
Definition at line 31 of file accesspoint.h.
◆ WpaFlags
typedef QFlags< WpaFlag > NetworkManager::AccessPoint::WpaFlags |
Definition at line 70 of file accesspoint.h.
Member Enumeration Documentation
◆ Capability
General capabilities of an access point.
Enumerator | |
---|---|
None | Null capability - says nothing about the access point. |
Privacy | Access point supports privacy measures. |
Definition at line 45 of file accesspoint.h.
◆ OperationMode
The access point's current operating mode.
Enumerator | |
---|---|
Unknown | not associated with a network |
Adhoc | part of an adhoc network |
Infra | a station in an infrastructure wireless network |
ApMode | access point in an infrastructure network |
Definition at line 36 of file accesspoint.h.
◆ WpaFlag
Flags describing the access point's capabilities according to WPA (Wifi Protected Access)
Definition at line 52 of file accesspoint.h.
Constructor & Destructor Documentation
◆ AccessPoint()
Definition at line 50 of file accesspoint.cpp.
◆ ~AccessPoint()
|
override |
Definition at line 70 of file accesspoint.cpp.
Member Function Documentation
◆ bandwidth()
uint AccessPoint::bandwidth | ( | ) | const |
The bandwidth announced by the access point in MHz.
- Since
- 6.12.0.
Definition at line 148 of file accesspoint.cpp.
◆ bandwidthChanged
|
signal |
This signal is emitted when bandwidth announced by the access point changes.
- Parameters
-
lastSeen the bandwidth announced by the access point in MHz.
- Since
- 6.12.0
- See also
- bandwidth
◆ bitRateChanged
|
signal |
This signal is emitted when the bitrate of this network has changed.
- Parameters
-
bitrate the new bitrate value for this network
◆ capabilities()
NetworkManager::AccessPoint::Capabilities AccessPoint::capabilities | ( | ) | const |
- Returns
- capabilities of an access point
Definition at line 88 of file accesspoint.cpp.
◆ capabilitiesChanged
|
signal |
This signal is emitted when the capabilities of this network have changed.
- Parameters
-
caps the new capabilities
◆ convertOperationMode()
|
static |
Helper method to convert wire representation of operation mode
to enum.
Definition at line 154 of file accesspoint.cpp.
◆ frequency()
uint AccessPoint::frequency | ( | ) | const |
- Returns
- The radio channel frequency in use by the access point, in MHz.
Definition at line 118 of file accesspoint.cpp.
◆ frequencyChanged
|
signal |
This signal is emitted when the frequency used by this Access Point changes.
- Parameters
-
frequency the new frequency
◆ hardwareAddress()
QString AccessPoint::hardwareAddress | ( | ) | const |
- Returns
- The hardware address (BSSID) of the access point.
Definition at line 82 of file accesspoint.cpp.
◆ lastSeen()
int AccessPoint::lastSeen | ( | ) | const |
- Returns
- The timestamp (in CLOCK_BOOTTIME seconds) for the last time the access point was found in scan results. A value of -1 means the access point has never been found in scan results.
- Since
- 5.14.0
Definition at line 142 of file accesspoint.cpp.
◆ lastSeenChanged
|
signal |
This signal is emitted when the timestamp for the last time the access point was found in scan results changes.
- Parameters
-
lastSeen the timestamp for the last time the access point was found in scan results.
- Since
- 5.14.0
- See also
- lastSeen
◆ maxBitRate()
uint AccessPoint::maxBitRate | ( | ) | const |
- Returns
- The maximum bitrate this access point is capable of, in kilobits/second (Kb/s).
Definition at line 124 of file accesspoint.cpp.
◆ mode()
NetworkManager::AccessPoint::OperationMode AccessPoint::mode | ( | ) | const |
- Returns
- Describes the operating mode of the access point.
Definition at line 130 of file accesspoint.cpp.
◆ rawSsid()
QByteArray AccessPoint::rawSsid | ( | ) | const |
- Returns
- raw SSID, encoded as a byte array
Definition at line 112 of file accesspoint.cpp.
◆ rsnFlags()
NetworkManager::AccessPoint::WpaFlags AccessPoint::rsnFlags | ( | ) | const |
- Returns
- Flags describing the access point's capabilities according to the RSN (Robust Secure Network) protocol.
- See also
- WpaFlag
Definition at line 100 of file accesspoint.cpp.
◆ rsnFlagsChanged
|
signal |
This signal is emitted when the RSN(WPA2) flags in use by this access point change.
- Parameters
-
flags the new flags
◆ signalStrength()
int AccessPoint::signalStrength | ( | ) | const |
- Returns
- The current signal quality of the access point, in percent.
Definition at line 136 of file accesspoint.cpp.
◆ signalStrengthChanged
|
signal |
This signal is emitted when the signal strength of this network has changed.
- Parameters
-
strength the new signal strength value for this network
◆ ssid()
QString AccessPoint::ssid | ( | ) | const |
- Returns
- The Service Set Identifier identifying the access point.
Definition at line 106 of file accesspoint.cpp.
◆ ssidChanged
|
signal |
This signal is emitted when the ssid of this Access Point changes.
- Parameters
-
ssid the new SSID
◆ uni()
QString AccessPoint::uni | ( | ) | const |
- Returns
- path of the access point
Definition at line 76 of file accesspoint.cpp.
◆ wpaFlags()
NetworkManager::AccessPoint::WpaFlags AccessPoint::wpaFlags | ( | ) | const |
- Returns
- flags describing the access point's capabilities according to WPA (Wifi Protected Access).
- See also
- WpaFlag
Definition at line 94 of file accesspoint.cpp.
◆ wpaFlagsChanged
|
signal |
This signal is emitted when the WPA flags in use by this access point change.
- Parameters
-
flags the new flags
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:52:50 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.