NetworkManager::PppSetting
#include <pppsetting.h>
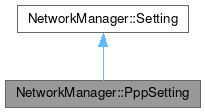
Public Types | |
typedef QList< Ptr > | List |
typedef QSharedPointer< PppSetting > | Ptr |
![]() | |
typedef QList< Ptr > | List |
enum | MacAddressRandomization { MacAddressRandomizationDefault = 0 , MacAddressRandomizationNever , MacAddressRandomizationAlways } |
typedef QSharedPointer< Setting > | Ptr |
typedef QFlags< SecretFlagType > | SecretFlags |
enum | SecretFlagType { None = 0 , AgentOwned = 0x01 , NotSaved = 0x02 , NotRequired = 0x04 } |
enum | SettingType { Adsl , Cdma , Gsm , Infiniband , Ipv4 , Ipv6 , Ppp , Pppoe , Security8021x , Serial , Vpn , Wired , Wireless , WirelessSecurity , Bluetooth , OlpcMesh , Vlan , Wimax , Bond , Bridge , BridgePort , Team , Generic , Tun , Vxlan , IpTunnel , Proxy , User , OvsBridge , OvsInterface , OvsPatch , OvsPort , Match , Tc , TeamPort , Macsec , Dcb , WireGuard } |
Public Member Functions | |
PppSetting (const Ptr &other) | |
quint32 | baud () const |
bool | cRtsCts () const |
void | fromMap (const QVariantMap &setting) override |
quint32 | lcpEchoFailure () const |
quint32 | lcpEchoInterval () const |
bool | mppeStateful () const |
quint32 | mru () const |
quint32 | mtu () const |
QString | name () const override |
bool | noAuth () const |
bool | noBsdComp () const |
bool | noDeflate () const |
bool | noVjComp () const |
bool | refuseChap () const |
bool | refuseEap () const |
bool | refuseMschap () const |
bool | refuseMschapv2 () const |
bool | refusePap () const |
bool | requireMppe () const |
bool | requireMppe128 () const |
void | setBaud (quint32 baud) |
void | setCRtsCts (bool control) |
void | setLcpEchoFailure (quint32 number) |
void | setLcpEchoInterval (quint32 interval) |
void | setMppeStateful (bool used) |
void | setMru (quint32 mru) |
void | setMtu (quint32 mtu) |
void | setNoAuth (bool require) |
void | setNoBsdComp (bool require) |
void | setNoDeflate (bool require) |
void | setNoVjComp (bool require) |
void | setRefuseChap (bool refuse) |
void | setRefuseEap (bool refuse) |
void | setRefuseMschap (bool refuse) |
void | setRefuseMschapv2 (bool refuse) |
void | setRefusePap (bool refuse) |
void | setRequireMppe (bool require) |
void | setRequireMppe128 (bool require) |
QVariantMap | toMap () const override |
![]() | |
Setting (const Ptr &setting) | |
Setting (SettingType type) | |
bool | isNull () const |
virtual QStringList | needSecrets (bool requestNew=false) const |
virtual void | secretsFromMap (const QVariantMap &map) |
virtual void | secretsFromStringMap (const NMStringMap &map) |
virtual QVariantMap | secretsToMap () const |
virtual NMStringMap | secretsToStringMap () const |
void | setInitialized (bool initialized) |
void | setType (SettingType type) |
SettingType | type () const |
Protected Attributes | |
PppSettingPrivate * | d_ptr |
![]() | |
SettingPrivate * | d_ptr |
Additional Inherited Members | |
![]() | |
static QString | typeAsString (SettingType type) |
static SettingType | typeFromString (const QString &type) |
Detailed Description
Represents ppp setting.
Definition at line 22 of file pppsetting.h.
Member Typedef Documentation
◆ List
typedef QList<Ptr> NetworkManager::PppSetting::List |
Definition at line 26 of file pppsetting.h.
◆ Ptr
Definition at line 25 of file pppsetting.h.
Constructor & Destructor Documentation
◆ PppSetting() [1/2]
NetworkManager::PppSetting::PppSetting | ( | ) |
Definition at line 35 of file pppsetting.cpp.
◆ PppSetting() [2/2]
|
explicit |
Definition at line 41 of file pppsetting.cpp.
◆ ~PppSetting()
|
override |
Definition at line 65 of file pppsetting.cpp.
Member Function Documentation
◆ baud()
quint32 NetworkManager::PppSetting::baud | ( | ) | const |
Definition at line 266 of file pppsetting.cpp.
◆ cRtsCts()
bool NetworkManager::PppSetting::cRtsCts | ( | ) | const |
Definition at line 252 of file pppsetting.cpp.
◆ fromMap()
|
overridevirtual |
Must be reimplemented, default implementation does nothing.
Reimplemented from NetworkManager::Setting.
Definition at line 329 of file pppsetting.cpp.
◆ lcpEchoFailure()
quint32 NetworkManager::PppSetting::lcpEchoFailure | ( | ) | const |
Definition at line 308 of file pppsetting.cpp.
◆ lcpEchoInterval()
quint32 NetworkManager::PppSetting::lcpEchoInterval | ( | ) | const |
Definition at line 322 of file pppsetting.cpp.
◆ mppeStateful()
bool NetworkManager::PppSetting::mppeStateful | ( | ) | const |
Definition at line 238 of file pppsetting.cpp.
◆ mru()
quint32 NetworkManager::PppSetting::mru | ( | ) | const |
Definition at line 280 of file pppsetting.cpp.
◆ mtu()
quint32 NetworkManager::PppSetting::mtu | ( | ) | const |
Definition at line 294 of file pppsetting.cpp.
◆ name()
|
overridevirtual |
Must be reimplemented, default implementationd does nothing.
Reimplemented from NetworkManager::Setting.
Definition at line 70 of file pppsetting.cpp.
◆ noAuth()
bool NetworkManager::PppSetting::noAuth | ( | ) | const |
Definition at line 84 of file pppsetting.cpp.
◆ noBsdComp()
bool NetworkManager::PppSetting::noBsdComp | ( | ) | const |
Definition at line 168 of file pppsetting.cpp.
◆ noDeflate()
bool NetworkManager::PppSetting::noDeflate | ( | ) | const |
Definition at line 182 of file pppsetting.cpp.
◆ noVjComp()
bool NetworkManager::PppSetting::noVjComp | ( | ) | const |
Definition at line 196 of file pppsetting.cpp.
◆ refuseChap()
bool NetworkManager::PppSetting::refuseChap | ( | ) | const |
Definition at line 126 of file pppsetting.cpp.
◆ refuseEap()
bool NetworkManager::PppSetting::refuseEap | ( | ) | const |
Definition at line 98 of file pppsetting.cpp.
◆ refuseMschap()
bool NetworkManager::PppSetting::refuseMschap | ( | ) | const |
Definition at line 140 of file pppsetting.cpp.
◆ refuseMschapv2()
bool NetworkManager::PppSetting::refuseMschapv2 | ( | ) | const |
Definition at line 154 of file pppsetting.cpp.
◆ refusePap()
bool NetworkManager::PppSetting::refusePap | ( | ) | const |
Definition at line 112 of file pppsetting.cpp.
◆ requireMppe()
bool NetworkManager::PppSetting::requireMppe | ( | ) | const |
Definition at line 210 of file pppsetting.cpp.
◆ requireMppe128()
bool NetworkManager::PppSetting::requireMppe128 | ( | ) | const |
Definition at line 224 of file pppsetting.cpp.
◆ setBaud()
void NetworkManager::PppSetting::setBaud | ( | quint32 | baud | ) |
Definition at line 259 of file pppsetting.cpp.
◆ setCRtsCts()
void NetworkManager::PppSetting::setCRtsCts | ( | bool | control | ) |
Definition at line 245 of file pppsetting.cpp.
◆ setLcpEchoFailure()
void NetworkManager::PppSetting::setLcpEchoFailure | ( | quint32 | number | ) |
Definition at line 301 of file pppsetting.cpp.
◆ setLcpEchoInterval()
void NetworkManager::PppSetting::setLcpEchoInterval | ( | quint32 | interval | ) |
Definition at line 315 of file pppsetting.cpp.
◆ setMppeStateful()
void NetworkManager::PppSetting::setMppeStateful | ( | bool | used | ) |
Definition at line 231 of file pppsetting.cpp.
◆ setMru()
void NetworkManager::PppSetting::setMru | ( | quint32 | mru | ) |
Definition at line 273 of file pppsetting.cpp.
◆ setMtu()
void NetworkManager::PppSetting::setMtu | ( | quint32 | mtu | ) |
Definition at line 287 of file pppsetting.cpp.
◆ setNoAuth()
void NetworkManager::PppSetting::setNoAuth | ( | bool | require | ) |
Definition at line 77 of file pppsetting.cpp.
◆ setNoBsdComp()
void NetworkManager::PppSetting::setNoBsdComp | ( | bool | require | ) |
Definition at line 161 of file pppsetting.cpp.
◆ setNoDeflate()
void NetworkManager::PppSetting::setNoDeflate | ( | bool | require | ) |
Definition at line 175 of file pppsetting.cpp.
◆ setNoVjComp()
void NetworkManager::PppSetting::setNoVjComp | ( | bool | require | ) |
Definition at line 189 of file pppsetting.cpp.
◆ setRefuseChap()
void NetworkManager::PppSetting::setRefuseChap | ( | bool | refuse | ) |
Definition at line 119 of file pppsetting.cpp.
◆ setRefuseEap()
void NetworkManager::PppSetting::setRefuseEap | ( | bool | refuse | ) |
Definition at line 91 of file pppsetting.cpp.
◆ setRefuseMschap()
void NetworkManager::PppSetting::setRefuseMschap | ( | bool | refuse | ) |
Definition at line 133 of file pppsetting.cpp.
◆ setRefuseMschapv2()
void NetworkManager::PppSetting::setRefuseMschapv2 | ( | bool | refuse | ) |
Definition at line 147 of file pppsetting.cpp.
◆ setRefusePap()
void NetworkManager::PppSetting::setRefusePap | ( | bool | refuse | ) |
Definition at line 105 of file pppsetting.cpp.
◆ setRequireMppe()
void NetworkManager::PppSetting::setRequireMppe | ( | bool | require | ) |
Definition at line 203 of file pppsetting.cpp.
◆ setRequireMppe128()
void NetworkManager::PppSetting::setRequireMppe128 | ( | bool | require | ) |
Definition at line 217 of file pppsetting.cpp.
◆ toMap()
|
overridevirtual |
Must be reimplemented, default implementationd does nothing.
Reimplemented from NetworkManager::Setting.
Definition at line 404 of file pppsetting.cpp.
Member Data Documentation
◆ d_ptr
|
protected |
Definition at line 92 of file pppsetting.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 31 2025 12:04:57 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.