Syndication::Loader
#include <loader.h>
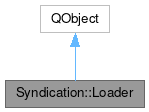
Signals | |
void | loadingComplete (Syndication::Loader *loader, Syndication::FeedPtr feed, Syndication::ErrorCode error) |
Public Member Functions | |
void | abort () |
QUrl | discoveredFeedURL () const |
ErrorCode | errorCode () const |
void | loadFrom (const QUrl &url, DataRetriever *retriever) |
int | retrieverError () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static Loader * | create () |
static Loader * | create (QObject *object, const char *slot) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class is the preferred way of loading feed sources.
Usage is very straightforward:
This creates a Loader object, connects it's loadingComplete() signal to your custom slot and then makes it load the file 'http://www.blah.org/foobar.rdf'. You could've done something like this as well:
That'd make the Loader use a custom algorithm for retrieving the RSS data; 'OutputRetriever' will make it execute the script '/home/myself/some-script.py' and assume whatever that script prints to stdout is RSS/Azom markup. This is e.g. handy for conversion scripts, which download a HTML file and convert it's contents into RSS markup.
No matter what kind of retrieval algorithm you employ, your 'slotLoadingComplete' method might look like this:
Member Function Documentation
◆ abort()
void Syndication::Loader::abort | ( | ) |
aborts the loading process
Definition at line 83 of file loader.cpp.
◆ create() [1/2]
|
static |
Constructs a Loader instance.
This is pretty much what the default constructor would do, except that it ensures that all Loader instances have been allocated on the heap (this is required so that Loader's can delete themselves safely after they emitted the loadingComplete() signal.).
- Returns
- A pointer to a new Loader instance.
Definition at line 40 of file loader.cpp.
◆ create() [2/2]
Convenience method.
Does the same as the above method except that it also does the job of connecting the loadingComplete() signal to the given slot for you.
- Parameters
-
object A QObject which features the specified slot slot Which slot to connect to.
Definition at line 45 of file loader.cpp.
◆ discoveredFeedURL()
QUrl Syndication::Loader::discoveredFeedURL | ( | ) | const |
returns the URL of a feed discovered in the feed source
Definition at line 95 of file loader.cpp.
◆ errorCode()
Syndication::ErrorCode Syndication::Loader::errorCode | ( | ) | const |
Retrieves the error code of the last loading process (if any).
Definition at line 78 of file loader.cpp.
◆ loadFrom()
void Syndication::Loader::loadFrom | ( | const QUrl & | url, |
DataRetriever * | retriever ) |
Loads the feed source referenced by the given URL using the specified retrieval algorithm.
Make sure that you connected to the loadingComplete() signal before calling this method so that you're guaranteed to get notified when the loading finished.
- Note
- A Loader object cannot load from multiple URLs simultaneously; consequently, subsequent calls to loadFrom will be discarded silently, only the first loadFrom request will be executed.
- Parameters
-
url A URL referencing the input file. retriever A subclass of DataRetriever which implements a specialized retrieval behaviour. Note that the ownership of the retriever is transferred to the Loader, i.e. the Loader will delete it when it doesn't need it anymore.
- See also
- DataRetriever, Loader::loadingComplete()
Definition at line 59 of file loader.cpp.
◆ loadingComplete
|
signal |
This signal gets emitted when the loading process triggered by calling loadFrom() finished.
- Parameters
-
loader A pointer pointing to the loader object which emitted this signal; this is handy in case you connect multiple loaders to a single slot. feed In case errortus is Success, this parameter holds the parsed feed. If fetching/parsing failed, feed is NULL. error An error code telling whether there were any problems while retrieving or parsing the data.
◆ retrieverError()
int Syndication::Loader::retrieverError | ( | ) | const |
the error code returned from the retriever.
Use this if you use your custom retriever implementation and need the specific error, not covered by errorCode().
Definition at line 73 of file loader.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Nov 29 2024 11:48:37 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.