ThreadWeaver::Queue
#include <queue.h>
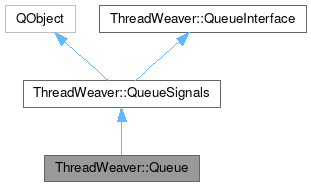
Classes | |
struct | GlobalQueueFactory |
Public Member Functions | |
Queue (QObject *parent=nullptr) | |
Queue (QueueSignals *implementation, QObject *parent=nullptr) | |
~Queue () override | |
int | currentNumberOfThreads () const override |
void | dequeue () override |
bool | dequeue (const JobPointer &) override |
void | enqueue (const JobPointer &job) |
void | enqueue (const QList< JobPointer > &jobs) override |
void | finish () override |
bool | isEmpty () const override |
bool | isIdle () const override |
int | maximumNumberOfThreads () const override |
int | queueLength () const override |
void | requestAbort () override |
void | reschedule () override |
void | resume () override |
void | setMaximumNumberOfThreads (int cap) override |
void | shutDown () override |
const State * | state () const override |
QueueStream | stream () |
void | suspend () override |
![]() | |
QueueSignals (QObject *parent=nullptr) | |
QueueSignals (ThreadWeaver::Private::QueueSignals_Private *d, QObject *parent=nullptr) | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static ThreadWeaver::Queue * | instance () |
static void | setGlobalQueueFactory (GlobalQueueFactory *factory) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
void | finished () |
void | stateChanged (ThreadWeaver::State *) |
void | suspended () |
![]() | |
typedef | QObjectList |
![]() | |
ThreadWeaver::Private::QueueSignals_Private * | d () |
const ThreadWeaver::Private::QueueSignals_Private * | d () const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Queue implements a ThreadWeaver job queue.
Queues process jobs enqueued in them by automatically assigning them to worker threads they manage. Applications using ThreadWeaver can make use of a global Queue which is instantiated on demand, or create multiple queues as needed. A job assigned to a queue will be processed by that specific queue.
Worker threads are created by the queues as needed. To create a customized global queue, see GlobalQueueFactory.
Constructor & Destructor Documentation
◆ Queue() [1/2]
|
explicit |
◆ Queue() [2/2]
|
explicit |
Construct a queue with a customized implementation The queue takes ownership and will delete the implementation upon destruction.
Construct a Queue, specifying the QueueSignals implementation to use.
The QueueSignals instance is usually a Weaver object, which may be customized for specific application needs. The Weaver instance will take ownership of the implementation object and deletes it when destructed.
- See also
- Weaver
- GlobalQueueFactory
◆ ~Queue()
|
override |
Destruct the Queue object.
If the queue is not already in Destructed state, the destructor will call shutDown() to make sure enqueued jobs are completed and the queue is idle. The queue implementation will be destroyed.
- See also
- shutDown()
- ThreadWeaver::Destructed
Member Function Documentation
◆ currentNumberOfThreads()
|
overridevirtual |
Returns the current number of threads in the inventory.
Implements ThreadWeaver::QueueInterface.
◆ dequeue() [1/2]
|
overridevirtual |
Remove all queued jobs.
All waiting jobs will be dequeued. The semantics are the same as for dequeue(JobInterface).
- See also
- dequeue(JobInterface)
Implements ThreadWeaver::QueueInterface.
◆ dequeue() [2/2]
|
overridevirtual |
Remove a job from the queue.
If the job was queued but not started so far, it is removed from the queue.
You can always call dequeue, it will return true if the job was dequeued. However if the job is not in the queue anymore, it is already being executed, it is too late to dequeue, and dequeue will return false. The return value is thread-safe - if true is returned, the job was still waiting, and has been dequeued. If not, the job was not waiting in the queue.
Modifying queued jobs is best done on a suspended queue. Often, for example at the end of an application, it is sufficient to dequeue all jobs (which leaves only the ones mid-air in threads), call finish (that will wait for all the mid air jobs to complete), and then exit. Without dequeue(), all jobs in the queue would be executed during finish().
- See also
- requestAbort for aborting jobs during execution
- Returns
- true if the job was waiting and has been dequeued
- false if the job was not found waiting in the queue
Implements ThreadWeaver::QueueInterface.
◆ enqueue() [1/2]
void Queue::enqueue | ( | const JobPointer & | job | ) |
◆ enqueue() [2/2]
|
overridevirtual |
Queue a vector of jobs.
It depends on the state if execution of the job will be attempted immediately. In suspended state, jobs can be added to the queue, but the threads remain suspended. In WorkongHard state, an idle thread may immediately execute the job, or it might be queued if all threads are busy.
JobPointer is a shared pointer. This means the object pointed to will be deleted if this object is the last remaining reference to it. Keep a JobPointer to the job to avoid automatic deletion.
Implements ThreadWeaver::QueueInterface.
◆ finish()
|
overridevirtual |
Finish all queued operations, then return.
This method is used in imperative (not event driven) programs that cannot react on events to have the controlling (main) thread wait wait for the jobs to finish. The call will block the calling thread and return when all queued jobs have been processed.
Warning: This will suspend your thread! Warning: If one of your jobs enters an infinite loop, this will never return!
Implements ThreadWeaver::QueueInterface.
◆ instance()
|
static |
Access the application-global Queue.
In some cases, the global queue is sufficient for the applications purpose. The global queue will only be created if this method is actually called in the lifetime of the application.
The Q(Core)Application object must exist when instance() is called for the first time. The global queue will be destroyed when Q(Core)Application is destructed. After that, the instance() method returns zero.
◆ isEmpty()
|
overridevirtual |
Is the queue empty?
The queue is empty if no more jobs are queued.
Implements ThreadWeaver::QueueInterface.
◆ isIdle()
|
overridevirtual |
Is the weaver idle?
The weaver is idle if no jobs are queued and no jobs are processed by the threads.
Implements ThreadWeaver::QueueInterface.
◆ maximumNumberOfThreads()
|
overridevirtual |
Get the maximum number of threads this Weaver may start.
Implements ThreadWeaver::QueueInterface.
◆ queueLength()
|
overridevirtual |
Returns the number of pending jobs.
This will return the number of queued jobs. Jobs that are currently being executed are not part of the queue. All jobs in the queue are waiting to be executed.
Implements ThreadWeaver::QueueInterface.
◆ requestAbort()
|
overridevirtual |
Request aborts of the currently executed jobs.
It is important to understand that aborts are requested, but cannot be guaranteed, as not all Job classes support it. It is up to the application to decide if and how job aborts are necessary.
Implements ThreadWeaver::QueueInterface.
◆ reschedule()
|
overridevirtual |
Reschedule the jobs in the queue.
This method triggers a scheduling attempt to perform jobs. It will schedule enqueued jobs to be executed by idle threads. It should only be necessary to call it if the canRun() status of a job changed spontaneously due to external reasons.
Implements ThreadWeaver::QueueInterface.
◆ resume()
|
overridevirtual |
◆ setGlobalQueueFactory()
|
static |
Set the factory object that will create the global queue.
Once set, the global queue factory will be deleted when the global ThreadWeaver pool is deleted. The factory object needs to be set before the global ThreadWeaver pool is instantiated. Call this method before Q(Core)Application is constructed.
◆ setMaximumNumberOfThreads()
|
overridevirtual |
Set the maximum number of threads this Weaver object may start.
Implements ThreadWeaver::QueueInterface.
◆ shutDown()
|
overridevirtual |
Shut down the queue.
Tells all threads to exit, and changes to Destructed state. It is safe to destroy the queue once this method returns.
Implements ThreadWeaver::QueueInterface.
◆ state()
|
overridevirtual |
Return the state of the weaver object.
Implements ThreadWeaver::QueueInterface.
◆ stream()
QueueStream Queue::stream | ( | ) |
Create a QueueStream to enqueue jobs into this queue.
◆ suspend()
|
overridevirtual |
Suspend job execution.
When suspending, all threads are allowed to finish the currently assigned job but will not receive a new assignment. When all threads are done processing the assigned job, the signal suspended will() be emitted. If you call suspend() and there are no jobs left to be done, you will immediately receive the suspended() signal.
Implements ThreadWeaver::QueueInterface.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:54:35 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.