ThreadWeaver::QueueInterface
#include <queueinterface.h>
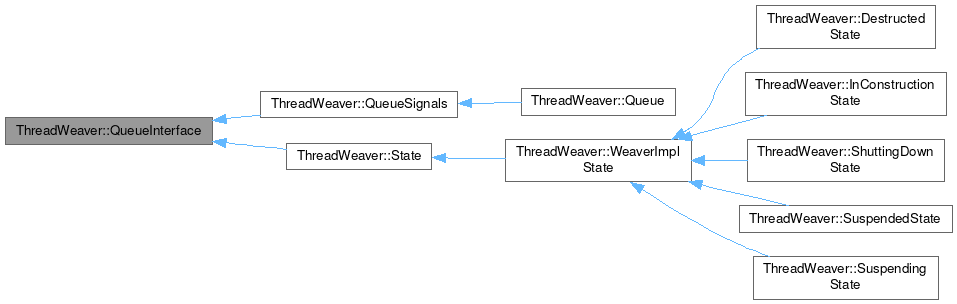
Public Member Functions | |
virtual int | currentNumberOfThreads () const =0 |
virtual void | dequeue ()=0 |
virtual bool | dequeue (const JobPointer &job)=0 |
virtual void | enqueue (const QList< JobPointer > &jobs)=0 |
virtual void | finish ()=0 |
virtual bool | isEmpty () const =0 |
virtual bool | isIdle () const =0 |
virtual int | maximumNumberOfThreads () const =0 |
virtual int | queueLength () const =0 |
virtual void | requestAbort ()=0 |
virtual void | reschedule ()=0 |
virtual void | resume ()=0 |
virtual void | setMaximumNumberOfThreads (int cap)=0 |
virtual void | shutDown ()=0 |
virtual const State * | state () const =0 |
virtual void | suspend ()=0 |
Detailed Description
WeaverInterface provides a common interface for weaver implementations.
In most cases, it is sufficient for an application to hold exactly one ThreadWeaver job queue. To execute jobs in a specific order, use job dependencies. To limit the number of jobs of a certain type that can be executed at the same time, use resource restrictions. To handle special requirements of the application when it comes to the order of execution of jobs, implement a special queue policy and apply it to the jobs.
Users of the ThreadWeaver API are encouraged to program to this interface, instead of the implementation. This way, implementation changes will not affect user programs.
This interface can be used for example to implement adapters and decorators. The member documentation is provided in the Weaver and WeaverImpl classes.
Definition at line 44 of file queueinterface.h.
Constructor & Destructor Documentation
◆ ~QueueInterface()
|
inlinevirtual |
Definition at line 47 of file queueinterface.h.
Member Function Documentation
◆ currentNumberOfThreads()
|
pure virtual |
Returns the current number of threads in the inventory.
Implemented in ThreadWeaver::DestructedState, ThreadWeaver::Queue, ThreadWeaver::Weaver, and ThreadWeaver::WeaverImplState.
◆ dequeue() [1/2]
|
pure virtual |
Remove all queued jobs.
All waiting jobs will be dequeued. The semantics are the same as for dequeue(JobInterface).
- See also
- dequeue(JobInterface)
Implemented in ThreadWeaver::DestructedState, ThreadWeaver::Queue, ThreadWeaver::Weaver, and ThreadWeaver::WeaverImplState.
◆ dequeue() [2/2]
|
pure virtual |
Remove a job from the queue.
If the job was queued but not started so far, it is removed from the queue.
You can always call dequeue, it will return true if the job was dequeued. However if the job is not in the queue anymore, it is already being executed, it is too late to dequeue, and dequeue will return false. The return value is thread-safe - if true is returned, the job was still waiting, and has been dequeued. If not, the job was not waiting in the queue.
Modifying queued jobs is best done on a suspended queue. Often, for example at the end of an application, it is sufficient to dequeue all jobs (which leaves only the ones mid-air in threads), call finish (that will wait for all the mid air jobs to complete), and then exit. Without dequeue(), all jobs in the queue would be executed during finish().
- See also
- requestAbort for aborting jobs during execution
- Returns
- true if the job was waiting and has been dequeued
- false if the job was not found waiting in the queue
Implemented in ThreadWeaver::DestructedState, ThreadWeaver::Queue, ThreadWeaver::Weaver, and ThreadWeaver::WeaverImplState.
◆ enqueue()
|
pure virtual |
Queue a vector of jobs.
It depends on the state if execution of the job will be attempted immediately. In suspended state, jobs can be added to the queue, but the threads remain suspended. In WorkongHard state, an idle thread may immediately execute the job, or it might be queued if all threads are busy.
JobPointer is a shared pointer. This means the object pointed to will be deleted if this object is the last remaining reference to it. Keep a JobPointer to the job to avoid automatic deletion.
Implemented in ThreadWeaver::DestructedState, ThreadWeaver::Queue, ThreadWeaver::Weaver, and ThreadWeaver::WeaverImplState.
◆ finish()
|
pure virtual |
Finish all queued operations, then return.
This method is used in imperative (not event driven) programs that cannot react on events to have the controlling (main) thread wait wait for the jobs to finish. The call will block the calling thread and return when all queued jobs have been processed.
Warning: This will suspend your thread! Warning: If one of your jobs enters an infinite loop, this will never return!
Implemented in ThreadWeaver::DestructedState, ThreadWeaver::Queue, ThreadWeaver::Weaver, and ThreadWeaver::WeaverImplState.
◆ isEmpty()
|
pure virtual |
Is the queue empty?
The queue is empty if no more jobs are queued.
Implemented in ThreadWeaver::DestructedState, ThreadWeaver::Queue, ThreadWeaver::Weaver, and ThreadWeaver::WeaverImplState.
◆ isIdle()
|
pure virtual |
Is the weaver idle?
The weaver is idle if no jobs are queued and no jobs are processed by the threads.
Implemented in ThreadWeaver::DestructedState, ThreadWeaver::Queue, ThreadWeaver::Weaver, and ThreadWeaver::WeaverImplState.
◆ maximumNumberOfThreads()
|
pure virtual |
Get the maximum number of threads this Weaver may start.
Implemented in ThreadWeaver::DestructedState, ThreadWeaver::Queue, ThreadWeaver::Weaver, and ThreadWeaver::WeaverImplState.
◆ queueLength()
|
pure virtual |
Returns the number of pending jobs.
This will return the number of queued jobs. Jobs that are currently being executed are not part of the queue. All jobs in the queue are waiting to be executed.
Implemented in ThreadWeaver::DestructedState, ThreadWeaver::Queue, ThreadWeaver::Weaver, and ThreadWeaver::WeaverImplState.
◆ requestAbort()
|
pure virtual |
Request aborts of the currently executed jobs.
It is important to understand that aborts are requested, but cannot be guaranteed, as not all Job classes support it. It is up to the application to decide if and how job aborts are necessary.
Implemented in ThreadWeaver::DestructedState, ThreadWeaver::Queue, ThreadWeaver::Weaver, and ThreadWeaver::WeaverImplState.
◆ reschedule()
|
pure virtual |
Reschedule the jobs in the queue.
This method triggers a scheduling attempt to perform jobs. It will schedule enqueued jobs to be executed by idle threads. It should only be necessary to call it if the canRun() status of a job changed spontaneously due to external reasons.
Implemented in ThreadWeaver::Queue, ThreadWeaver::Weaver, and ThreadWeaver::WeaverImplState.
◆ resume()
|
pure virtual |
Resume job queueing.
- See also
- suspend
Implemented in ThreadWeaver::DestructedState, ThreadWeaver::InConstructionState, ThreadWeaver::Queue, ThreadWeaver::ShuttingDownState, ThreadWeaver::SuspendedState, ThreadWeaver::SuspendingState, and ThreadWeaver::Weaver.
◆ setMaximumNumberOfThreads()
|
pure virtual |
Set the maximum number of threads this Weaver object may start.
Implemented in ThreadWeaver::DestructedState, ThreadWeaver::Queue, ThreadWeaver::Weaver, and ThreadWeaver::WeaverImplState.
◆ shutDown()
|
pure virtual |
Shut down the queue.
Tells all threads to exit, and changes to Destructed state. It is safe to destroy the queue once this method returns.
Implemented in ThreadWeaver::DestructedState, ThreadWeaver::Queue, ThreadWeaver::ShuttingDownState, ThreadWeaver::Weaver, and ThreadWeaver::WeaverImplState.
◆ state()
|
pure virtual |
Return the state of the weaver object.
Implemented in ThreadWeaver::Queue, ThreadWeaver::Weaver, and ThreadWeaver::WeaverImplState.
◆ suspend()
|
pure virtual |
Suspend job execution.
When suspending, all threads are allowed to finish the currently assigned job but will not receive a new assignment. When all threads are done processing the assigned job, the signal suspended will() be emitted. If you call suspend() and there are no jobs left to be done, you will immediately receive the suspended() signal.
Implemented in ThreadWeaver::DestructedState, ThreadWeaver::InConstructionState, ThreadWeaver::Queue, ThreadWeaver::ShuttingDownState, ThreadWeaver::SuspendedState, ThreadWeaver::SuspendingState, and ThreadWeaver::Weaver.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 11 2025 11:48:22 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.