Akonadi::StandardCalendarActionManager
#include <standardcalendaractionmanager.h>
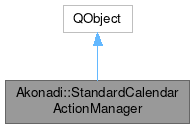
Public Types | |
enum | Type { CreateEvent = StandardActionManager::LastType + 1 , CreateTodo , CreateSubTodo , CreateJournal , EditIncidence , LastType } |
![]() | |
typedef | QObjectList |
Signals | |
void | actionStateUpdated () |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Manages calendar specific actions for collection and item views.
- Since
- 4.6
Definition at line 39 of file standardcalendaractionmanager.h.
Member Enumeration Documentation
◆ Type
Describes the supported actions.
Definition at line 46 of file standardcalendaractionmanager.h.
Constructor & Destructor Documentation
◆ StandardCalendarActionManager()
|
explicit |
Creates a new standard calendar action manager.
- Parameters
-
actionCollection The action collection to operate on. parent The parent widget.
Definition at line 477 of file standardcalendaractionmanager.cpp.
◆ ~StandardCalendarActionManager()
|
overridedefault |
Destroys the standard calendar action manager.
Member Function Documentation
◆ action() [1/2]
QAction * StandardCalendarActionManager::action | ( | StandardActionManager::Type | type | ) | const |
Returns the action of the given type, 0 if it has not been created (yet).
- Parameters
-
type the type of action to return
Definition at line 611 of file standardcalendaractionmanager.cpp.
◆ action() [2/2]
QAction * StandardCalendarActionManager::action | ( | StandardCalendarActionManager::Type | type | ) | const |
Returns the action of the given type, 0 if it has not been created (yet).
Definition at line 606 of file standardcalendaractionmanager.cpp.
◆ actionStateUpdated
|
signal |
This signal is emitted whenever the action state has been updated.
In case you have special needs for changing the state of some actions, connect to this signal and adjust the action state.
◆ createAction() [1/2]
QAction * StandardCalendarActionManager::createAction | ( | StandardActionManager::Type | type | ) |
Creates the action of the given type and adds it to the action collection specified in the constructor if it does not exist yet.
The action is connected to its default implementation provided by this class.
- Parameters
-
type the type of action to create
Definition at line 583 of file standardcalendaractionmanager.cpp.
◆ createAction() [2/2]
QAction * StandardCalendarActionManager::createAction | ( | StandardCalendarActionManager::Type | type | ) |
Creates the action of the given type and adds it to the action collection specified in the constructor if it does not exist yet.
The action is connected to its default implementation provided by this class.
- Parameters
-
type the type of action to create
Definition at line 514 of file standardcalendaractionmanager.cpp.
◆ createAllActions()
void StandardCalendarActionManager::createAllActions | ( | ) |
Convenience method to create all standard actions.
- See also
- createAction()
Definition at line 593 of file standardcalendaractionmanager.cpp.
◆ interceptAction() [1/2]
void StandardCalendarActionManager::interceptAction | ( | StandardActionManager::Type | type, |
bool | intercept = true ) |
Sets whether the default implementation for the given action type
shall be executed when the action is triggered.
- Parameters
-
intercept If false
, the default implementation will be executed, iftrue
no action is taken.
Definition at line 630 of file standardcalendaractionmanager.cpp.
◆ interceptAction() [2/2]
void StandardCalendarActionManager::interceptAction | ( | StandardCalendarActionManager::Type | type, |
bool | intercept = true ) |
Sets whether the default implementation for the given action type
shall be executed when the action is triggered.
- Parameters
-
intercept If false
, the default implementation will be executed, iftrue
no action is taken.
Definition at line 621 of file standardcalendaractionmanager.cpp.
◆ selectedCollections()
|
nodiscard |
Returns the list of collections that are currently selected.
The list is empty if no collection is currently selected.
Definition at line 635 of file standardcalendaractionmanager.cpp.
◆ selectedItems()
|
nodiscard |
Returns the list of items that are currently selected.
The list is empty if no item is currently selected.
Definition at line 640 of file standardcalendaractionmanager.cpp.
◆ setActionText()
void StandardCalendarActionManager::setActionText | ( | StandardActionManager::Type | type, |
const KLocalizedString & | text ) |
Sets the label of the action type
to text
, which is used during updating the action state and substituted according to the number of selected objects.
This is mainly useful to customize the label of actions that can operate on multiple objects.
Example:
Definition at line 616 of file standardcalendaractionmanager.cpp.
◆ setCollectionPropertiesPageNames()
void StandardCalendarActionManager::setCollectionPropertiesPageNames | ( | const QStringList & | names | ) |
Definition at line 655 of file standardcalendaractionmanager.cpp.
◆ setCollectionSelectionModel()
void StandardCalendarActionManager::setCollectionSelectionModel | ( | QItemSelectionModel * | selectionModel | ) |
Sets the collection selection model based on which the collection related actions should operate.
If none is set, all collection actions will be disabled.
- Parameters
-
selectionModel the selection model for collections
Definition at line 485 of file standardcalendaractionmanager.cpp.
◆ setContextText() [1/2]
void StandardCalendarActionManager::setContextText | ( | StandardActionManager::Type | type, |
StandardActionManager::TextContext | context, | ||
const KLocalizedString & | text ) |
Sets the text
of the action type
for the given context
.
Definition at line 650 of file standardcalendaractionmanager.cpp.
◆ setContextText() [2/2]
void StandardCalendarActionManager::setContextText | ( | StandardActionManager::Type | type, |
StandardActionManager::TextContext | context, | ||
const QString & | text ) |
Sets the text
of the action type
for the given context
.
Definition at line 645 of file standardcalendaractionmanager.cpp.
◆ setItemSelectionModel()
void StandardCalendarActionManager::setItemSelectionModel | ( | QItemSelectionModel * | selectionModel | ) |
Sets the item selection model based on which the item related actions should operate.
If none is set, all item actions will be disabled.
- Parameters
-
selectionModel the selection model for items
Definition at line 502 of file standardcalendaractionmanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:57:41 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.