AgentInstance
#include <agentinstance.h>
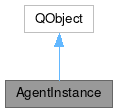
Public Types | |
using | Ptr = QSharedPointer<AgentInstance> |
Public Member Functions | |
AgentInstance (AgentManager &manager) | |
QString | agentType () const |
virtual void | cleanup () |
virtual void | configure (qlonglong windowId)=0 |
org::freedesktop::Akonadi::Agent::Control * | controlInterface () const |
bool | hasAgentInterface () const |
bool | hasPreprocessorInterface () const |
bool | hasResourceInterface () const |
QString | identifier () const |
bool | isOnline () const |
bool | obtainAgentInterface () |
bool | obtainPreprocessorInterface () |
bool | obtainResourceInterface () |
org::freedesktop::Akonadi::Preprocessor * | preProcessorInterface () const |
int | progress () const |
virtual void | quit () |
org::freedesktop::Akonadi::Resource * | resourceInterface () const |
QString | resourceName () const |
virtual void | restartWhenIdle ()=0 |
org::freedesktop::Akonadi::Agent::Search * | searchInterface () const |
void | setIdentifier (const QString &identifier) |
virtual bool | start (const AgentType &agentInfo)=0 |
int | status () const |
org::freedesktop::Akonadi::Agent::Status * | statusInterface () const |
QString | statusMessage () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Slots | |
void | advancedStatusChanged (const QVariantMap &status) |
void | error (const QString &msg) |
void | errorHandler (const QDBusError &error) |
void | onlineChanged (bool state) |
void | percentChanged (int percent) |
void | refreshAgentStatus () |
void | refreshResourceStatus () |
void | resourceNameChanged (const QString &name) |
void | statusChanged (int status, const QString &statusMsg) |
void | statusMessageChanged (const QString &msg) |
void | statusStateChanged (int status) |
void | warning (const QString &msg) |
Protected Member Functions | |
void | setAgentType (const QString &agentType) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
Represents one agent instance and takes care of communication with it.
The agent exposes multiple D-Bus interfaces. The Control and the Status interfaces are implemented by all the agents. The Resource and Preprocessor interfaces are obviously implemented only by the agents impersonating resources or preprocessors.
Definition at line 34 of file akonadicontrol/agentinstance.h.
Member Typedef Documentation
◆ Ptr
Definition at line 38 of file akonadicontrol/agentinstance.h.
Constructor & Destructor Documentation
◆ AgentInstance()
|
explicit |
Definition at line 12 of file akonadicontrol/agentinstance.cpp.
◆ ~AgentInstance()
|
overridedefault |
Definition at line 28 of file core/agentinstance.cpp.
Member Function Documentation
◆ advancedStatusChanged
|
protectedslot |
Definition at line 94 of file akonadicontrol/agentinstance.cpp.
◆ agentType()
|
inlinenodiscard |
Definition at line 54 of file akonadicontrol/agentinstance.h.
◆ cleanup()
|
virtual |
Definition at line 28 of file akonadicontrol/agentinstance.cpp.
◆ controlInterface()
|
inline |
Definition at line 105 of file akonadicontrol/agentinstance.h.
◆ error
|
protectedslot |
Definition at line 123 of file akonadicontrol/agentinstance.cpp.
◆ errorHandler
|
protectedslot |
Definition at line 173 of file akonadicontrol/agentinstance.cpp.
◆ hasAgentInterface()
|
inlinenodiscard |
Definition at line 95 of file akonadicontrol/agentinstance.h.
◆ hasPreprocessorInterface()
|
inlinenodiscard |
Definition at line 100 of file akonadicontrol/agentinstance.h.
◆ hasResourceInterface()
|
inlinenodiscard |
Definition at line 90 of file akonadicontrol/agentinstance.h.
◆ identifier()
|
inlinenodiscard |
Set/get the unique identifier of this AgentInstance.
Definition at line 44 of file akonadicontrol/agentinstance.h.
◆ isOnline()
|
inlinenodiscard |
Definition at line 74 of file akonadicontrol/agentinstance.h.
◆ obtainAgentInterface()
bool AgentInstance::obtainAgentInterface | ( | ) |
Definition at line 35 of file akonadicontrol/agentinstance.cpp.
◆ obtainPreprocessorInterface()
bool AgentInstance::obtainPreprocessorInterface | ( | ) |
Definition at line 78 of file akonadicontrol/agentinstance.cpp.
◆ obtainResourceInterface()
bool AgentInstance::obtainResourceInterface | ( | ) |
Definition at line 65 of file akonadicontrol/agentinstance.cpp.
◆ onlineChanged
|
protectedslot |
Definition at line 128 of file akonadicontrol/agentinstance.cpp.
◆ percentChanged
|
protectedslot |
Definition at line 109 of file akonadicontrol/agentinstance.cpp.
◆ preProcessorInterface()
|
inline |
Definition at line 125 of file akonadicontrol/agentinstance.h.
◆ progress()
|
inlinenodiscard |
Definition at line 69 of file akonadicontrol/agentinstance.h.
◆ quit()
|
virtual |
Definition at line 19 of file akonadicontrol/agentinstance.cpp.
◆ refreshAgentStatus
|
protectedslot |
Definition at line 146 of file akonadicontrol/agentinstance.cpp.
◆ refreshResourceStatus
|
protectedslot |
Definition at line 163 of file akonadicontrol/agentinstance.cpp.
◆ resourceInterface()
|
inline |
Definition at line 120 of file akonadicontrol/agentinstance.h.
◆ resourceName()
|
inlinenodiscard |
Definition at line 79 of file akonadicontrol/agentinstance.h.
◆ resourceNameChanged
|
protectedslot |
Definition at line 137 of file akonadicontrol/agentinstance.cpp.
◆ searchInterface()
|
inline |
Definition at line 115 of file akonadicontrol/agentinstance.h.
◆ setAgentType()
|
inlineprotected |
Definition at line 155 of file akonadicontrol/agentinstance.h.
◆ setIdentifier()
|
inline |
Definition at line 49 of file akonadicontrol/agentinstance.h.
◆ status()
|
inlinenodiscard |
Definition at line 59 of file akonadicontrol/agentinstance.h.
◆ statusChanged
|
protectedslot |
Definition at line 84 of file akonadicontrol/agentinstance.cpp.
◆ statusInterface()
|
inline |
Definition at line 110 of file akonadicontrol/agentinstance.h.
◆ statusMessage()
|
inlinenodiscard |
Definition at line 64 of file akonadicontrol/agentinstance.h.
◆ statusMessageChanged
|
protectedslot |
Definition at line 104 of file akonadicontrol/agentinstance.cpp.
◆ statusStateChanged
|
protectedslot |
Definition at line 99 of file akonadicontrol/agentinstance.cpp.
◆ warning
|
protectedslot |
Definition at line 118 of file akonadicontrol/agentinstance.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:52:53 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.