Akonadi::AgentBase
#include <agentbase.h>
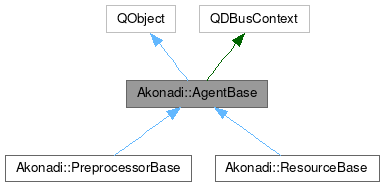
Classes | |
class | Observer |
class | ObserverV2 |
class | ObserverV3 |
Public Types | |
enum | Status { Idle = 0 , Running , Broken , NotConfigured } |
Signals | |
void | abortRequested () |
void | advancedStatus (const QVariantMap &status) |
void | agentActivitiesChanged (const QStringList &activities) |
void | agentActivitiesEnabledChanged (bool enabled) |
void | agentNameChanged (const QString &name) |
void | configurationDialogAccepted () |
void | configurationDialogRejected () |
void | error (const QString &message) |
void | onlineChanged (bool online) |
void | percent (int progress) |
void | reloadConfiguration () |
void | status (int status, const QString &message=QString()) |
void | warning (const QString &message) |
Public Slots | |
virtual void | configure (WId windowId) |
Public Member Functions | |
QStringList | activities () const |
bool | activitiesEnabled () const |
QString | agentName () const |
virtual void | cleanup () |
QString | identifier () const |
virtual int | progress () const |
virtual QString | progressMessage () const |
void | registerObserver (Observer *observer) |
void | setActivities (const QStringList &activities) |
void | setActivitiesEnabled (bool enabled) |
void | setAgentName (const QString &name) |
virtual int | status () const |
virtual QString | statusMessage () const |
WId | winIdForDialogs () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
template<typename T> | |
static int | initCore (int argc, char **argv) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
AgentBase (const QString &id) | |
~AgentBase () override | |
virtual KAboutData | aboutData () const |
virtual void | aboutToQuit () |
void | changeProcessed () |
ChangeRecorder * | changeRecorder () const |
KSharedConfigPtr | config () |
virtual void | doSetOnline (bool online) |
bool | isOnline () const |
QString | programName () const |
void | setNeedsNetwork (bool needsNetwork) |
void | setOnline (bool state) |
void | setTemporaryOffline (int makeOnlineInSeconds=300) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
bool | calledFromDBus () const const |
QDBusConnection | connection () const const |
bool | isDelayedReply () const const |
void | sendErrorReply (const QString &name, const QString &msg) const const |
void | sendErrorReply (QDBusError::ErrorType type, const QString &msg) const const |
void | setDelayedReply (bool enable) const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
Detailed Description
The base class for all Akonadi agents and resources.
This class is a base class for all Akonadi agents, which covers the real agent processes and all resources.
It provides:
- lifetime management
- change monitoring and recording
- configuration interface
- problem reporting
Akonadi Server supports several ways to launch agents and resources:
- As a separate application (
- See also
- AKONADI_AGENT_MAIN)
- As a thread in the AgentServer
- As a separate process, using the akonadi_agent_launcher
The idea is this, the agent or resource is written as a plugin instead of an executable (
- See also
- AgentFactory). In the AgentServer case, the AgentServer looks up the plugin and launches the agent in a separate thread. In the launcher case, a new akonadi_agent_launcher process is started for each agent or resource instance.
When making an Agent or Resource suitable for running in the AgentServer some extra caution is needed. Because multiple instances of several kinds of agents run in the same process, one cannot blindly use global objects like KGlobal. For this reasons several methods where added to avoid problems in this context, most notably AgentBase::config(). Additionally, one cannot use QDBusConnection::sessionBus() with dbus < 1.4, because of a multithreading bug in libdbus. Instead one should use QDBusConnection::sessionBus() which works around this problem.
Definition at line 73 of file agentbase.h.
Member Enumeration Documentation
◆ Status
This enum describes the different states the agent can be in.
Enumerator | |
---|---|
Idle | The agent does currently nothing. |
Running | The agent is working on something. |
Broken | The agent encountered an error state. |
NotConfigured | The agent is lacking required configuration. |
Definition at line 397 of file agentbase.h.
Constructor & Destructor Documentation
◆ AgentBase()
|
protected |
Creates an agent base.
- Parameters
-
id The instance id of the agent.
◆ ~AgentBase()
|
overrideprotected |
Destroys the agent base.
Member Function Documentation
◆ abortRequested
|
signal |
Emitted when another application has remotely asked the agent to abort its current operation.
Connect to this signal if your agent supports abortion. After aborting and cleaning up, agents should return to Idle status.
- Since
- 4.4
◆ aboutData()
|
protectedvirtual |
Definition at line 979 of file agentbase.cpp.
◆ aboutToQuit()
|
protectedvirtual |
This method is called whenever the agent application is about to quit.
Reimplement this method to do session cleanup (e.g. disconnecting from groupware server).
Definition at line 1037 of file agentbase.cpp.
◆ activities()
|
nodiscard |
◆ activitiesEnabled()
|
nodiscard |
◆ advancedStatus
|
signal |
This signal should be emitted whenever the status of the agent has been changed.
- Parameters
-
status The object that describes the status change.
- Since
- 4.6
◆ agentActivitiesChanged
|
signal |
This signal is emitted whenever the user has changed activities.
- Since
- 6.3
◆ agentActivitiesEnabledChanged
|
signal |
This signal is emitted whenever the user has changed enabled activities.
- Since
- 6.3
◆ agentName()
|
nodiscard |
◆ agentNameChanged
|
signal |
This signal is emitted whenever the name of the agent has changed.
- Parameters
-
name The new name of the agent.
- Since
- 4.3
◆ changeProcessed()
|
protected |
Marks the current change as processes and replays the next change if change recording is enabled (noop otherwise).
This method is called from the default implementation of the change notification slots. While not required when not using change recording, it is nevertheless recommended to call this method when done with processing a change notification.
Definition at line 1218 of file agentbase.cpp.
◆ changeRecorder()
|
protected |
Returns the Akonadi::ChangeRecorder object used for monitoring.
Use this to configure which parts you want to monitor.
Definition at line 1224 of file agentbase.cpp.
◆ cleanup()
|
virtual |
This method is called when the agent is removed from the system, so it can do some cleanup stuff.
- Note
- If you reimplement this in a subclass make sure to call this base implementation at the end.
Definition at line 1043 of file agentbase.cpp.
◆ config()
|
protected |
Returns the config object for this Agent.
Definition at line 1229 of file agentbase.cpp.
◆ configurationDialogAccepted
|
signal |
This signal is emitted whenever the user has accepted the configuration dialog.
- Note
- Implementors of agents/resources are responsible to emit this signal if the agent/resource reimplements configure().
- Since
- 4.4
◆ configurationDialogRejected
|
signal |
This signal is emitted whenever the user has rejected the configuration dialog.
- Note
- Implementors of agents/resources are responsible to emit this signal if the agent/resource reimplements configure().
- Since
- 4.4
◆ configure
|
virtualslot |
This method is called whenever the agent shall show its configuration dialog to the user.
It will be automatically called when the agent is started for the first time.
- Parameters
-
windowId The parent window id.
- Note
- If the method is reimplemented it has to emit the configurationDialogAccepted() or configurationDialogRejected() signals depending on the users choice.
Definition at line 989 of file agentbase.cpp.
◆ doSetOnline()
|
protectedvirtual |
This method is called whenever the online
status has changed.
Reimplement this method to react on online status changes.
- Parameters
-
online online status
Reimplemented in Akonadi::ResourceBase.
Definition at line 974 of file agentbase.cpp.
◆ error
|
signal |
This signal shall be used to report errors.
- Parameters
-
message The i18n'ed error message.
◆ identifier()
|
nodiscard |
Returns the instance identifier of this agent.
Definition at line 1139 of file agentbase.cpp.
◆ initCore()
|
inlinestatic |
Use this method in the main function of your agent application to initialize your agent subclass.
This method also takes care of creating a QCoreApplication object and parsing command line arguments.
- Note
- In case the given class is also derived from AgentBase::Observer it gets registered as its own observer (see AgentBase::Observer), e.g.
agentInstance->registerObserver( agentInstance );
- Parameters
-
argc number of arguments argv arguments for the function
Definition at line 429 of file agentbase.h.
◆ isOnline()
|
protected |
Returns whether the agent is currently online.
Definition at line 892 of file agentbase.cpp.
◆ onlineChanged
|
signal |
Emitted when the online state changed.
- Parameters
-
online The online state.
- Since
- 4.2
◆ percent
|
signal |
This signal should be emitted whenever the progress of an action in the agent (e.g.
data transfer, connection establishment to remote server etc.) has changed.
- Parameters
-
progress The progress of the action in percent.
◆ programName()
|
nodiscardprotected |
Get the application display name.
◆ progress()
|
nodiscardvirtual |
This method returns the current progress of the agent in percentage.
Definition at line 878 of file agentbase.cpp.
◆ progressMessage()
|
nodiscardvirtual |
This method returns an i18n'ed description of the current progress.
Definition at line 885 of file agentbase.cpp.
◆ registerObserver()
void AgentBase::registerObserver | ( | Observer * | observer | ) |
Registers the given observer for reacting on monitored or recorded changes.
- Parameters
-
observer The change handler to register. No ownership transfer, i.e. the caller stays owner of the pointer and can reset the registration by calling this method with 0
Definition at line 1088 of file agentbase.cpp.
◆ reloadConfiguration
|
signal |
Emitted if another application has changed the agent's configuration remotely and called AgentInstance::reconfigure().
- Since
- 4.2
◆ setActivities()
void AgentBase::setActivities | ( | const QStringList & | activities | ) |
This method is used to set the activities of the agent.
- Since
- 6.3
Definition at line 1178 of file agentbase.cpp.
◆ setActivitiesEnabled()
void AgentBase::setActivitiesEnabled | ( | bool | enabled | ) |
This method is used to enabled the activities of the agent.
- Since
- 6.3
Definition at line 1206 of file agentbase.cpp.
◆ setAgentName()
void AgentBase::setAgentName | ( | const QString & | name | ) |
This method is used to set the name of the agent.
- Since
- 4.3
- Parameters
-
name name of the agent
Definition at line 1144 of file agentbase.cpp.
◆ setNeedsNetwork()
|
protected |
Sets whether the agent needs network or not.
- Since
- 4.2
- Todo
- use this in combination with QNetworkConfiguration to change the onLine status of the agent.
- Parameters
-
needsNetwork true
if the agents needs network. Defaults tofalse
Definition at line 899 of file agentbase.cpp.
◆ setOnline()
|
protected |
Sets whether the agent shall be online or not.
Definition at line 913 of file agentbase.cpp.
◆ setTemporaryOffline()
|
protected |
Sets the agent offline but will make it online again after a given time.
Use this method when the agent detects some problem with its backend but it wants to retry all pending operations after some time - e.g. a server can not be reached currently
Example usage:
- Since
- 4.13
- Parameters
-
makeOnlineInSeconds timeout in seconds after which the agent changes to online
Definition at line 930 of file agentbase.cpp.
◆ status() [1/2]
|
nodiscardvirtual |
This method returns the current status code of the agent.
The following return values are possible:
- 0 - Idle
- 1 - Running
- 2 - Broken
- 3 - NotConfigured
Definition at line 864 of file agentbase.cpp.
◆ status [2/2]
This signal should be emitted whenever the status of the agent has been changed.
- Parameters
-
status The new Status code. message A i18n'ed description of the new status.
◆ statusMessage()
|
nodiscardvirtual |
This method returns an i18n'ed description of the current status code.
Definition at line 871 of file agentbase.cpp.
◆ warning
|
signal |
This signal shall be used to report warnings.
- Parameters
-
message The i18n'ed warning message.
◆ winIdForDialogs()
|
nodiscard |
This method returns the windows id, which should be used for dialogs.
Definition at line 1002 of file agentbase.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:53:10 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.