Akonadi::ItemFetchJob
#include <itemfetchjob.h>
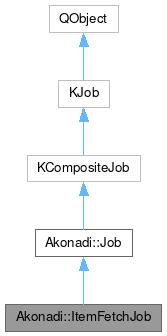
Public Types | |
enum | DeliveryOption { ItemGetter = 0x1 , EmitItemsIndividually = 0x2 , EmitItemsInBatches = 0x4 , Default = ItemGetter | EmitItemsInBatches } |
typedef QFlags< DeliveryOption > | DeliveryOptions |
![]() | |
enum | Error { ConnectionFailed = UserDefinedError , ProtocolVersionMismatch , UserCanceled , Unknown , UserError = UserDefinedError + 42 } |
using | List = QList<Job *> |
![]() | |
typedef QFlags< Capability > | Capabilities |
enum | Capability |
enum | Unit |
Signals | |
void | itemsReceived (const Akonadi::Item::List &items) |
![]() | |
void | aboutToStart (Akonadi::Job *job) |
void | writeFinished (Akonadi::Job *job) |
![]() | |
void | description (KJob *job, const QString &title, const QPair< QString, QString > &field1=QPair< QString, QString >(), const QPair< QString, QString > &field2=QPair< QString, QString >()) |
void | finished (KJob *job) |
void | infoMessage (KJob *job, const QString &message) |
void | percentChanged (KJob *job, unsigned long percent) |
void | processedAmountChanged (KJob *job, KJob::Unit unit, qulonglong amount) |
void | processedSize (KJob *job, qulonglong size) |
void | result (KJob *job) |
void | resumed (KJob *job) |
void | speed (KJob *job, unsigned long speed) |
void | suspended (KJob *job) |
void | totalAmountChanged (KJob *job, KJob::Unit unit, qulonglong amount) |
void | totalSize (KJob *job, qulonglong size) |
void | warning (KJob *job, const QString &message) |
Public Member Functions | |
ItemFetchJob (const Collection &collection, QObject *parent=nullptr) | |
ItemFetchJob (const Item &item, QObject *parent=nullptr) | |
ItemFetchJob (const Item::List &items, QObject *parent=nullptr) | |
ItemFetchJob (const QList< Item::Id > &items, QObject *parent=nullptr) | |
ItemFetchJob (const Tag &tag, QObject *parent=nullptr) | |
~ItemFetchJob () override | |
void | clearItems () |
int | count () const |
DeliveryOptions | deliveryOptions () const |
ItemFetchScope & | fetchScope () |
Item::List | items () const |
void | setCollection (const Collection &collection) |
void | setDeliveryOption (DeliveryOptions options) |
void | setFetchScope (const ItemFetchScope &fetchScope) |
void | setLimit (int limit, int start, Qt::SortOrder order=Qt::DescendingOrder) |
![]() | |
Job (QObject *parent=nullptr) | |
~Job () override | |
QString | errorString () const final |
void | start () override |
![]() | |
KCompositeJob (QObject *parent=nullptr) | |
![]() | |
KJob (QObject *parent=nullptr) | |
Capabilities | capabilities () const |
int | error () const |
QString | errorText () const |
bool | exec () |
bool | isAutoDelete () const |
bool | isFinishedNotificationHidden () const |
bool | isStartedWithExec () const |
bool | isSuspended () const |
unsigned long | percent () const |
Q_SCRIPTABLE qulonglong | processedAmount (Unit unit) const |
void | setAutoDelete (bool autodelete) |
void | setFinishedNotificationHidden (bool hide=true) |
void | setUiDelegate (KJobUiDelegate *delegate) |
Q_SCRIPTABLE qulonglong | totalAmount (Unit unit) const |
KJobUiDelegate * | uiDelegate () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
bool | doHandleResponse (qint64 tag, const Protocol::CommandPtr &response) override |
void | doStart () override |
![]() | |
bool | addSubjob (KJob *job) override |
bool | doKill () override |
void | emitWriteFinished () |
bool | removeSubjob (KJob *job) override |
![]() | |
void | clearSubjobs () |
bool | hasSubjobs () const |
const QList< KJob * > & | subjobs () const |
![]() | |
virtual bool | doResume () |
virtual bool | doSuspend () |
void | emitPercent (qulonglong processedAmount, qulonglong totalAmount) |
void | emitResult () |
void | emitSpeed (unsigned long speed) |
bool | isFinished () const |
void | setCapabilities (Capabilities capabilities) |
void | setError (int errorCode) |
void | setErrorText (const QString &errorText) |
void | setPercent (unsigned long percentage) |
void | setProcessedAmount (Unit unit, qulonglong amount) |
void | setProgressUnit (Unit unit) |
void | setTotalAmount (Unit unit, qulonglong amount) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
bool | kill (KJob::KillVerbosity verbosity=KJob::Quietly) |
bool | resume () |
bool | suspend () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
Bytes | |
Directories | |
Files | |
Items | |
Killable | |
NoCapabilities | |
Suspendable | |
UnitsCount | |
![]() | |
typedef | QObjectList |
![]() | |
void | slotResult (KJob *job) override |
![]() | |
virtual void | slotInfoMessage (KJob *job, const QString &message) |
virtual void | slotResult (KJob *job) |
Detailed Description
Job that fetches items from the Akonadi storage.
This class is used to fetch items from the Akonadi storage. Which parts of the items (e.g. headers only, attachments or all) can be specified by the ItemFetchScope.
Note that ItemFetchJob does not refresh the Akonadi storage from the backend; this is unnecessary due to the fact that backend updates automatically trigger an update to the Akonadi database whenever they occur (unless the resource is offline).
Note that items can not be created in the root collection (Collection::root()) and therefore can not be fetched from there either. That is - an item fetch in the root collection will yield an empty list.
Example:
Definition at line 69 of file itemfetchjob.h.
Member Typedef Documentation
◆ DeliveryOptions
Definition at line 200 of file itemfetchjob.h.
Member Enumeration Documentation
◆ DeliveryOption
Enumerator | |
---|---|
ItemGetter | items available through items() |
EmitItemsIndividually | emitted via signal upon reception |
EmitItemsInBatches | emitted via signal in bulk (collected and emitted delayed via timer) |
Definition at line 194 of file itemfetchjob.h.
Constructor & Destructor Documentation
◆ ItemFetchJob() [1/5]
|
explicit |
Creates a new item fetch job that retrieves all items inside the given collection.
- Parameters
-
collection The parent collection to fetch all items from. parent The parent object.
Definition at line 110 of file itemfetchjob.cpp.
◆ ItemFetchJob() [2/5]
Creates a new item fetch job that retrieves the specified item.
If the item has a uid set, this is used to identify the item on the Akonadi server. If only a remote identifier is available, that is used. However, as remote identifiers are not necessarily globally unique, you need to specify the collection to search in in that case, using setCollection().
For internal use only when using remote identifiers, the resource search context can be set globally by ResourceSelectJob.
- Parameters
-
item The item to fetch. parent The parent object.
Definition at line 120 of file itemfetchjob.cpp.
◆ ItemFetchJob() [3/5]
|
explicit |
Creates a new item fetch job that retrieves the specified items.
If the items have a uid set, this is used to identify the item on the Akonadi server. If only a remote identifier is available, that is used. However, as remote identifiers are not necessarily globally unique, you need to specify the collection to search in in that case, using setCollection().
For internal use only when using remote identifiers, the resource search context can be set globally by ResourceSelectJob.
- Parameters
-
items The items to fetch. parent The parent object.
- Since
- 4.4
Definition at line 129 of file itemfetchjob.cpp.
◆ ItemFetchJob() [4/5]
Convenience ctor equivalent to ItemFetchJob(const Item::List &items, QObject *parent = nullptr)
- Since
- 4.8
Definition at line 138 of file itemfetchjob.cpp.
◆ ItemFetchJob() [5/5]
Creates a new item fetch job that retrieves all items tagged with specified tag
.
- Parameters
-
tag The tag to fetch all items from. parent The parent object.
- Since
- 4.14
Definition at line 149 of file itemfetchjob.cpp.
◆ ~ItemFetchJob()
|
overridedefault |
Destroys the item fetch job.
Member Function Documentation
◆ clearItems()
void ItemFetchJob::clearItems | ( | ) |
Save memory by clearing the fetched items.
- Since
- 4.12
Definition at line 223 of file itemfetchjob.cpp.
◆ count()
int ItemFetchJob::count | ( | ) | const |
Returns the total number of retrieved items.
This works also without the ItemGetter DeliveryOption.
- Since
- 4.14
Definition at line 265 of file itemfetchjob.cpp.
◆ deliveryOptions()
ItemFetchJob::DeliveryOptions ItemFetchJob::deliveryOptions | ( | ) | const |
◆ doHandleResponse()
|
overrideprotectedvirtual |
This method should be reimplemented in the concrete jobs in case you want to handle incoming data.
It will be called on received data from the backend. The default implementation does nothing.
- Parameters
-
tag The tag of the corresponding command, empty if this is an untagged response. response The received response
- Returns
- Implementations should return true if the last response was processed and the job can emit result. Return false if more responses from server are expected.
Reimplemented from Akonadi::Job.
Definition at line 179 of file itemfetchjob.cpp.
◆ doStart()
|
overrideprotectedvirtual |
This method must be reimplemented in the concrete jobs.
It will be called after the job has been started and a connection to the Akonadi backend has been established.
Implements Akonadi::Job.
Definition at line 161 of file itemfetchjob.cpp.
◆ fetchScope()
ItemFetchScope & ItemFetchJob::fetchScope | ( | ) |
Returns the item fetch scope.
Since this returns a reference it can be used to conveniently modify the current scope in-place, i.e. by calling a method on the returned reference without storing it in a local variable. See the ItemFetchScope documentation for an example.
- Returns
- a reference to the current item fetch scope
- See also
- setFetchScope() for replacing the current item fetch scope
Definition at line 237 of file itemfetchjob.cpp.
◆ items()
|
nodiscard |
Returns the fetched items.
This returns an empty list when not using the ItemGetter DeliveryOption.
- Note
- The items are invalid before the result(KJob*) signal has been emitted or if an error occurred.
Definition at line 216 of file itemfetchjob.cpp.
◆ itemsReceived
|
signal |
This signal is emitted whenever new items have been fetched completely.
- Note
- This is an optimization; instead of waiting for the end of the job and calling items(), you can connect to this signal and get the items incrementally.
- Parameters
-
items The fetched items.
◆ setCollection()
void ItemFetchJob::setCollection | ( | const Collection & | collection | ) |
Specifies the collection the item is in.
This is only required when retrieving an item based on its remote id which might not be unique globally.
- See also
- ResourceSelectJob (for internal use only)
Definition at line 244 of file itemfetchjob.cpp.
◆ setDeliveryOption()
void ItemFetchJob::setDeliveryOption | ( | DeliveryOptions | options | ) |
Sets the mechanisms by which the items should be fetched.
- Since
- 4.13
Definition at line 251 of file itemfetchjob.cpp.
◆ setFetchScope()
void ItemFetchJob::setFetchScope | ( | const ItemFetchScope & | fetchScope | ) |
Sets the item fetch scope.
The ItemFetchScope controls how much of an item's data is fetched from the server, e.g. whether to fetch the full item payload or only meta data.
- Parameters
-
fetchScope The new scope for item fetch operations.
- See also
- fetchScope()
- Since
- 4.4
Definition at line 230 of file itemfetchjob.cpp.
◆ setLimit()
void ItemFetchJob::setLimit | ( | int | limit, |
int | start, | ||
Qt::SortOrder | order = Qt::DescendingOrder ) |
Sets the limit of fetched items.
- Parameters
-
limit the maximum number of items to retrieve. The default value for limit
is -1, indicating no limit.start specifies the offset of the first item to retrieve. order specifies whether items will be fetched starting with the highest or lowest ID of the item.
Definition at line 272 of file itemfetchjob.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:52:53 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.