MailTransport::TransportManager
#include <transportmanager.h>
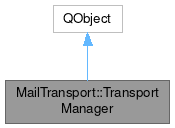
Public Types | |
enum | ShowCondition { Always , IfNoTransportExists } |
Signals | |
Q_SCRIPTABLE void | changesCommitted () |
void | passwordsChanged () |
void | transportRemoved (int id, const QString &name) |
void | transportRenamed (int id, const QString &oldName, const QString &newName) |
Q_SCRIPTABLE void | transportsChanged () |
Public Member Functions | |
~TransportManager () override | |
void | addTransport (Transport *transport) |
bool | configureTransport (const QString &identifier, Transport *transport, QWidget *parent) |
Transport * | createTransport () const |
MAILTRANSPORT_DEPRECATED TransportJob * | createTransportJob (const QString &transport) |
MAILTRANSPORT_DEPRECATED TransportJob * | createTransportJob (Transport::Id transportId) |
Q_SCRIPTABLE int | defaultTransportId () const |
Q_SCRIPTABLE QString | defaultTransportName () const |
void | initializeTransport (const QString &identifier, Transport *transport) |
Q_SCRIPTABLE bool | isEmpty () const |
void | loadPasswordsAsync () |
void | removePasswordFromWallet (Transport::Id id) |
Q_SCRIPTABLE void | removeTransport (int id) |
MAILTRANSPORT_DEPRECATED void | schedule (TransportJob *job) |
Q_SCRIPTABLE void | setDefaultTransport (int id) |
bool | showTransportCreationDialog (QWidget *parent, ShowCondition showCondition=Always) |
Transport * | transportById (Transport::Id id, bool def=true) const |
Transport * | transportByName (const QString &name, bool def=true) const |
Q_SCRIPTABLE QList< int > | transportIds () const |
Q_SCRIPTABLE QStringList | transportNames () const |
QList< Transport * > | transports () const |
TransportType::List | types () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static TransportManager * | self () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
TransportManager () | |
void | loadPasswords () |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
Detailed Description
Central transport management interface.
This class manages the creation, configuration, and removal of mail transports, as well as the loading and storing of mail transport settings.
It also handles the creation of transport jobs, although that behaviour is deprecated and you are encouraged to use MessageQueueJob.
- See also
- MessageQueueJob.
Definition at line 34 of file transportmanager.h.
Member Enumeration Documentation
◆ ShowCondition
Describes when to show the transport creation dialog.
Definition at line 140 of file transportmanager.h.
Constructor & Destructor Documentation
◆ ~TransportManager()
|
override |
Destructor.
Definition at line 125 of file transportmanager.cpp.
◆ TransportManager()
|
protected |
Singleton class, the only instance resides in the static object sSelf.
Definition at line 105 of file transportmanager.cpp.
Member Function Documentation
◆ addTransport()
void TransportManager::addTransport | ( | Transport * | transport | ) |
Adds the given transport.
The object ownership is transferred to TransportMananger, ie. you must not delete transport
.
- Parameters
-
transport The Transport object to add.
Definition at line 184 of file transportmanager.cpp.
◆ changesCommitted
|
signal |
Internal signal to synchronize all TransportManager instances.
This signal is emitted by the instance writing the changes. You probably want to use transportsChanged() instead.
◆ configureTransport()
bool TransportManager::configureTransport | ( | const QString & | identifier, |
Transport * | transport, | ||
QWidget * | parent ) |
Open a configuration dialog for an existing transport.
- Parameters
-
identifier The identifier. transport The transport to configure. It can be a new transport, or one already managed by TransportManager. parent The parent widget for the dialog.
- Returns
- True if the user clicked Ok, false if the user cancelled.
- Since
- 4.4
Definition at line 246 of file transportmanager.cpp.
◆ createTransport()
Transport * TransportManager::createTransport | ( | ) | const |
Creates a new, empty Transport object.
The object is owned by the caller. If you want to add the Transport permanently (eg. after configuring it) call addTransport().
Definition at line 176 of file transportmanager.cpp.
◆ createTransportJob() [1/2]
TransportJob * TransportManager::createTransportJob | ( | const QString & | transport | ) |
Creates a mail transport job for the given transport identifier, or transport name.
Returns 0 if the specified transport is invalid.
- Parameters
-
transport A string defining a mail transport.
- Deprecated
- use MessageQueueJob to queue messages and rely on the Dispatcher Agent to send them.
Definition at line 271 of file transportmanager.cpp.
◆ createTransportJob() [2/2]
TransportJob * TransportManager::createTransportJob | ( | Transport::Id | transportId | ) |
Creates a mail transport job for the given transport identifier.
Returns 0 if the specified transport is invalid.
- Parameters
-
transportId The transport identifier.
- Deprecated
- use MessageQueueJob to queue messages and rely on the Dispatcher Agent to send them.
Definition at line 255 of file transportmanager.cpp.
◆ defaultTransportId()
int TransportManager::defaultTransportId | ( | ) | const |
Returns the default transport identifier.
Invalid if there are no transports at all.
Definition at line 326 of file transportmanager.cpp.
◆ defaultTransportName()
QString TransportManager::defaultTransportName | ( | ) | const |
Returns the default transport name.
Definition at line 317 of file transportmanager.cpp.
◆ initializeTransport()
Definition at line 238 of file transportmanager.cpp.
◆ isEmpty()
bool TransportManager::isEmpty | ( | ) | const |
Returns true if there are no mail transports at all.
Definition at line 292 of file transportmanager.cpp.
◆ loadPasswords()
|
protected |
Loads all passwords synchronously.
Definition at line 509 of file transportmanager.cpp.
◆ loadPasswordsAsync()
void TransportManager::loadPasswordsAsync | ( | ) |
Tries to load passwords asynchronously from KWallet if needed.
The passwordsChanged() signal is emitted once the passwords have been loaded. Nothing happens if the passwords were already available.
Definition at line 532 of file transportmanager.cpp.
◆ passwordsChanged
|
signal |
Emitted when passwords have been loaded from the wallet.
If you made a deep copy of a transport, you should call updatePasswordState() for the cloned transport to ensure its password is updated as well.
◆ removePasswordFromWallet()
void TransportManager::removePasswordFromWallet | ( | Transport::Id | id | ) |
Definition at line 340 of file transportmanager.cpp.
◆ removeTransport()
void TransportManager::removeTransport | ( | int | id | ) |
Deletes the specified transport.
- Parameters
-
id The identifier of the mail transport to remove.
Definition at line 347 of file transportmanager.cpp.
◆ schedule()
void TransportManager::schedule | ( | TransportJob * | job | ) |
Executes the given transport job.
This is the preferred way to start transport jobs. It takes care of asynchronously loading passwords from KWallet if necessary.
- Parameters
-
job The completely configured transport job to execute.
- Deprecated
- use MessageQueueJob to queue messages and rely on the Dispatcher Agent to send them.
Definition at line 198 of file transportmanager.cpp.
◆ self()
|
static |
Returns the TransportManager instance.
Definition at line 130 of file transportmanager.cpp.
◆ setDefaultTransport()
void TransportManager::setDefaultTransport | ( | int | id | ) |
Sets the default transport.
The change will be in effect immediately.
- Parameters
-
id The identifier of the new default transport.
Definition at line 331 of file transportmanager.cpp.
◆ showTransportCreationDialog()
bool TransportManager::showTransportCreationDialog | ( | QWidget * | parent, |
ShowCondition | showCondition = Always ) |
Shows a dialog for creating and configuring a new transport.
- Parameters
-
parent Parent widget of the dialog. showCondition the condition under which the dialog is shown at all
- Returns
- True if a new transport has been created and configured.
- Since
- 4.4
Definition at line 215 of file transportmanager.cpp.
◆ transportById()
Transport * TransportManager::transportById | ( | Transport::Id | id, |
bool | def = true ) const |
Returns the Transport object with the given id.
- Parameters
-
id The identifier of the Transport. def if set to true, the default transport will be returned if the specified Transport object could not be found, 0 otherwise.
- Returns
- A Transport object for immediate use. It might become invalid as soon as the event loop is entered again due to remote changes. If you need to store a Transport object, store the transport identifier instead.
Definition at line 139 of file transportmanager.cpp.
◆ transportByName()
Returns the transport object with the given name.
- Parameters
-
name The transport name. def if set to true, the default transport will be returned if the specified Transport object could not be found, 0 otherwise.
- Returns
- A Transport object for immediate use, see transportById() for limitations.
Definition at line 153 of file transportmanager.cpp.
◆ transportIds()
QList< int > TransportManager::transportIds | ( | ) | const |
Returns a list of transport identifiers.
Definition at line 297 of file transportmanager.cpp.
◆ transportNames()
QStringList TransportManager::transportNames | ( | ) | const |
Returns a list of transport names.
Definition at line 307 of file transportmanager.cpp.
◆ transportRemoved
|
signal |
Emitted when a transport is deleted.
- Parameters
-
id The identifier of the deleted transport. name The name of the deleted transport.
◆ transportRenamed
|
signal |
Emitted when a transport has been renamed.
- Parameters
-
id The identifier of the renamed transport. oldName The old name. newName The new name.
◆ transports()
Returns a list of all available transports.
Note: The Transport objects become invalid as soon as a change occur, so they are only suitable for immediate use.
Definition at line 166 of file transportmanager.cpp.
◆ transportsChanged
|
signal |
Emitted when transport settings have changed (by this or any other TransportManager instance).
◆ types()
|
nodiscard |
Returns a list of all available transport types.
Definition at line 171 of file transportmanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:51:45 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.