KGAPI2::People::Person
#include <person.h>
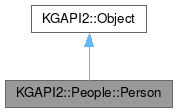
Public Types | |
enum class | AgeRange { AGE_RANGE_UNSPECIFIED , LESS_THAN_EIGHTEEN , EIGHTEEN_TO_TWENTY , TWENTY_ONE_OR_OLDER } |
Static Public Member Functions | |
static PersonPtr | fromJSON (const QJsonObject &obj) |
static PersonPtr | fromKContactsAddressee (const KContacts::Addressee &addressee) |
Detailed Description
Information about a person merged from various data sources such as the authenticated user's contacts and profile data.
Most fields can have multiple items. The items in a field have no guaranteed order, but each non-empty field is guaranteed to have exactly one field with metadata.primary
set to true.
- Since
- 5.23.0
Member Enumeration Documentation
◆ AgeRange
|
strong |
Constructor & Destructor Documentation
◆ Person()
|
explicit |
Construcuts a new Person.
Definition at line 436 of file person.cpp.
◆ ~Person()
|
default |
Destructor.
Member Function Documentation
◆ addAddress()
void KGAPI2::People::Person::addAddress | ( | const Address & | value | ) |
Appends the given value
to the list of addresses.
Definition at line 895 of file person.cpp.
◆ addBiography()
void KGAPI2::People::Person::addBiography | ( | const Biography & | value | ) |
Appends the given value
to the list of biographies.
Definition at line 716 of file person.cpp.
◆ addBirthday()
void KGAPI2::People::Person::addBirthday | ( | const Birthday & | value | ) |
Appends the given value
to the list of birthdays.
Definition at line 558 of file person.cpp.
◆ addBraggingRights()
void KGAPI2::People::Person::addBraggingRights | ( | const BraggingRights & | value | ) |
Appends the given value
to the list of braggingRights.
Definition at line 529 of file person.cpp.
◆ addCalendarUrl()
void KGAPI2::People::Person::addCalendarUrl | ( | const CalendarUrl & | value | ) |
Appends the given value
to the list of calendarUrls.
Definition at line 866 of file person.cpp.
◆ addClientData()
void KGAPI2::People::Person::addClientData | ( | const ClientData & | value | ) |
Appends the given value
to the list of clientData.
Definition at line 504 of file person.cpp.
◆ addEmailAddress()
void KGAPI2::People::Person::addEmailAddress | ( | const EmailAddress & | value | ) |
Appends the given value
to the list of emailAddresses.
Definition at line 479 of file person.cpp.
◆ addEvent()
void KGAPI2::People::Person::addEvent | ( | const Event & | value | ) |
Appends the given value
to the list of events.
Definition at line 979 of file person.cpp.
◆ addExternalId()
void KGAPI2::People::Person::addExternalId | ( | const ExternalId & | value | ) |
Appends the given value
to the list of externalIds.
Definition at line 658 of file person.cpp.
◆ addFileAs()
void KGAPI2::People::Person::addFileAs | ( | const FileAs & | value | ) |
Appends the given value
to the list of fileAses.
Definition at line 1171 of file person.cpp.
◆ addGender()
void KGAPI2::People::Person::addGender | ( | const Gender & | value | ) |
Appends the given value
to the list of genders.
Definition at line 1146 of file person.cpp.
◆ addImClient()
void KGAPI2::People::Person::addImClient | ( | const ImClient & | value | ) |
Appends the given value
to the list of imClients.
Definition at line 954 of file person.cpp.
◆ addInterest()
void KGAPI2::People::Person::addInterest | ( | const Interest & | value | ) |
Appends the given value
to the list of interests.
Definition at line 1071 of file person.cpp.
◆ addLocation()
void KGAPI2::People::Person::addLocation | ( | const Location & | value | ) |
Appends the given value
to the list of locations.
Definition at line 633 of file person.cpp.
◆ addMembership()
void KGAPI2::People::Person::addMembership | ( | const Membership & | value | ) |
Appends the given value
to the list of memberships.
Definition at line 766 of file person.cpp.
◆ addMiscKeyword()
void KGAPI2::People::Person::addMiscKeyword | ( | const MiscKeyword & | value | ) |
Appends the given value
to the list of miscKeywords.
Definition at line 1033 of file person.cpp.
◆ addName()
void KGAPI2::People::Person::addName | ( | const Name & | value | ) |
Appends the given value
to the list of names.
Definition at line 841 of file person.cpp.
◆ addNickname()
void KGAPI2::People::Person::addNickname | ( | const Nickname & | value | ) |
Appends the given value
to the list of nicknames.
Definition at line 454 of file person.cpp.
◆ addOccupation()
void KGAPI2::People::Person::addOccupation | ( | const Occupation & | value | ) |
Appends the given value
to the list of occupations.
Definition at line 1008 of file person.cpp.
◆ addOrganization()
void KGAPI2::People::Person::addOrganization | ( | const Organization & | value | ) |
Appends the given value
to the list of organizations.
Definition at line 1096 of file person.cpp.
◆ addPersonLocale()
void KGAPI2::People::Person::addPersonLocale | ( | const PersonLocale & | value | ) |
Appends the given value
to the list of locales.
Definition at line 608 of file person.cpp.
◆ addPhoneNumber()
void KGAPI2::People::Person::addPhoneNumber | ( | const PhoneNumber & | value | ) |
Appends the given value
to the list of phoneNumbers.
Definition at line 929 of file person.cpp.
◆ addRelation()
void KGAPI2::People::Person::addRelation | ( | const Relation & | value | ) |
Appends the given value
to the list of relations.
Definition at line 583 of file person.cpp.
◆ addResidence()
void KGAPI2::People::Person::addResidence | ( | const Residence & | value | ) |
Appends the given value
to the list of residences.
Definition at line 816 of file person.cpp.
◆ addresses()
The person's street addresses.
Definition at line 885 of file person.cpp.
◆ addSipAddress()
void KGAPI2::People::Person::addSipAddress | ( | const SipAddress & | value | ) |
Appends the given value
to the list of sipAddresses.
Definition at line 741 of file person.cpp.
◆ addSkill()
void KGAPI2::People::Person::addSkill | ( | const Skill & | value | ) |
Appends the given value
to the list of skills.
Definition at line 791 of file person.cpp.
◆ addUrl()
void KGAPI2::People::Person::addUrl | ( | const Url & | value | ) |
Appends the given value
to the list of urls.
Definition at line 1121 of file person.cpp.
◆ addUserDefined()
void KGAPI2::People::Person::addUserDefined | ( | const UserDefined & | value | ) |
Appends the given value
to the list of userDefined.
Definition at line 691 of file person.cpp.
◆ ageRange()
Person::Person::AgeRange KGAPI2::People::Person::ageRange | ( | ) | const |
Output only.
DEPRECATED (Please use person.ageRanges
instead) The person's age range.
Definition at line 1186 of file person.cpp.
◆ ageRanges()
QList< AgeRangeType > KGAPI2::People::Person::ageRanges | ( | ) | const |
◆ biographies()
The person's biographies.
This field is a singleton for contact sources.
Definition at line 706 of file person.cpp.
◆ birthdays()
The person's birthdays.
This field is a singleton for contact sources.
Definition at line 548 of file person.cpp.
◆ braggingRights()
QList< BraggingRights > KGAPI2::People::Person::braggingRights | ( | ) | const |
DEPRECATED: No data will be returned The person's bragging rights.
Definition at line 519 of file person.cpp.
◆ calendarUrls()
QList< CalendarUrl > KGAPI2::People::Person::calendarUrls | ( | ) | const |
The person's calendar URLs.
Definition at line 856 of file person.cpp.
◆ clearAddresses()
void KGAPI2::People::Person::clearAddresses | ( | ) |
Clears the list of addresses.
Definition at line 905 of file person.cpp.
◆ clearBiographies()
void KGAPI2::People::Person::clearBiographies | ( | ) |
Clears the list of biographies.
Definition at line 726 of file person.cpp.
◆ clearBirthdays()
void KGAPI2::People::Person::clearBirthdays | ( | ) |
Clears the list of birthdays.
Definition at line 568 of file person.cpp.
◆ clearBraggingRights()
void KGAPI2::People::Person::clearBraggingRights | ( | ) |
Clears the list of braggingRights.
Definition at line 539 of file person.cpp.
◆ clearCalendarUrls()
void KGAPI2::People::Person::clearCalendarUrls | ( | ) |
Clears the list of calendarUrls.
Definition at line 876 of file person.cpp.
◆ clearClientData()
void KGAPI2::People::Person::clearClientData | ( | ) |
Clears the list of clientData.
Definition at line 514 of file person.cpp.
◆ clearEmailAddresses()
void KGAPI2::People::Person::clearEmailAddresses | ( | ) |
Clears the list of emailAddresses.
Definition at line 489 of file person.cpp.
◆ clearEvents()
void KGAPI2::People::Person::clearEvents | ( | ) |
Clears the list of events.
Definition at line 989 of file person.cpp.
◆ clearExternalIds()
void KGAPI2::People::Person::clearExternalIds | ( | ) |
Clears the list of externalIds.
Definition at line 668 of file person.cpp.
◆ clearFileAses()
void KGAPI2::People::Person::clearFileAses | ( | ) |
Clears the list of fileAses.
Definition at line 1181 of file person.cpp.
◆ clearGenders()
void KGAPI2::People::Person::clearGenders | ( | ) |
Clears the list of genders.
Definition at line 1156 of file person.cpp.
◆ clearImClients()
void KGAPI2::People::Person::clearImClients | ( | ) |
Clears the list of imClients.
Definition at line 964 of file person.cpp.
◆ clearInterests()
void KGAPI2::People::Person::clearInterests | ( | ) |
Clears the list of interests.
Definition at line 1081 of file person.cpp.
◆ clearLocales()
void KGAPI2::People::Person::clearLocales | ( | ) |
Clears the list of locales.
Definition at line 618 of file person.cpp.
◆ clearLocations()
void KGAPI2::People::Person::clearLocations | ( | ) |
Clears the list of locations.
Definition at line 643 of file person.cpp.
◆ clearMemberships()
void KGAPI2::People::Person::clearMemberships | ( | ) |
Clears the list of memberships.
Definition at line 776 of file person.cpp.
◆ clearMiscKeywords()
void KGAPI2::People::Person::clearMiscKeywords | ( | ) |
Clears the list of miscKeywords.
Definition at line 1043 of file person.cpp.
◆ clearNames()
void KGAPI2::People::Person::clearNames | ( | ) |
Clears the list of names.
Definition at line 851 of file person.cpp.
◆ clearNicknames()
void KGAPI2::People::Person::clearNicknames | ( | ) |
Clears the list of nicknames.
Definition at line 464 of file person.cpp.
◆ clearOccupations()
void KGAPI2::People::Person::clearOccupations | ( | ) |
Clears the list of occupations.
Definition at line 1018 of file person.cpp.
◆ clearOrganizations()
void KGAPI2::People::Person::clearOrganizations | ( | ) |
Clears the list of organizations.
Definition at line 1106 of file person.cpp.
◆ clearPhoneNumbers()
void KGAPI2::People::Person::clearPhoneNumbers | ( | ) |
Clears the list of phoneNumbers.
Definition at line 939 of file person.cpp.
◆ clearRelations()
void KGAPI2::People::Person::clearRelations | ( | ) |
Clears the list of relations.
Definition at line 593 of file person.cpp.
◆ clearResidences()
void KGAPI2::People::Person::clearResidences | ( | ) |
Clears the list of residences.
Definition at line 826 of file person.cpp.
◆ clearSipAddresses()
void KGAPI2::People::Person::clearSipAddresses | ( | ) |
Clears the list of sipAddresses.
Definition at line 751 of file person.cpp.
◆ clearSkills()
void KGAPI2::People::Person::clearSkills | ( | ) |
Clears the list of skills.
Definition at line 801 of file person.cpp.
◆ clearUrls()
void KGAPI2::People::Person::clearUrls | ( | ) |
Clears the list of urls.
Definition at line 1131 of file person.cpp.
◆ clearUserDefined()
void KGAPI2::People::Person::clearUserDefined | ( | ) |
Clears the list of userDefined.
Definition at line 701 of file person.cpp.
◆ clientData()
QList< ClientData > KGAPI2::People::Person::clientData | ( | ) | const |
The person's client data.
Definition at line 494 of file person.cpp.
◆ coverPhotos()
QList< CoverPhoto > KGAPI2::People::Person::coverPhotos | ( | ) | const |
◆ emailAddresses()
QList< EmailAddress > KGAPI2::People::Person::emailAddresses | ( | ) | const |
The person's email addresses.
For people.connections.list
and otherContacts.list
the number of email addresses is limited to 100. If a Person has more email addresses the entire set can be obtained by calling GetPeople.
Definition at line 469 of file person.cpp.
◆ etag()
QString KGAPI2::People::Person::etag | ( | ) | const |
The HTTP entity tag of the resource.
Used for web cache validation.
Definition at line 910 of file person.cpp.
◆ events()
The person's events.
Definition at line 969 of file person.cpp.
◆ externalIds()
QList< ExternalId > KGAPI2::People::Person::externalIds | ( | ) | const |
The person's external IDs.
Definition at line 648 of file person.cpp.
◆ fileAses()
The person's file-ases.
Definition at line 1161 of file person.cpp.
◆ fromJSON()
|
static |
Definition at line 1195 of file person.cpp.
◆ fromKContactsAddressee()
|
static |
Definition at line 1563 of file person.cpp.
◆ genders()
The person's genders.
This field is a singleton for contact sources.
Definition at line 1136 of file person.cpp.
◆ imClients()
The person's instant messaging clients.
Definition at line 944 of file person.cpp.
◆ interests()
The person's interests.
Definition at line 1061 of file person.cpp.
◆ locales()
QList< PersonLocale > KGAPI2::People::Person::locales | ( | ) | const |
The person's locale preferences.
Definition at line 598 of file person.cpp.
◆ locations()
The person's locations.
Definition at line 623 of file person.cpp.
◆ memberships()
QList< Membership > KGAPI2::People::Person::memberships | ( | ) | const |
The person's group memberships.
Definition at line 756 of file person.cpp.
◆ metadata()
PersonMetadata KGAPI2::People::Person::metadata | ( | ) | const |
◆ miscKeywords()
QList< MiscKeyword > KGAPI2::People::Person::miscKeywords | ( | ) | const |
The person's miscellaneous keywords.
Definition at line 1023 of file person.cpp.
◆ names()
The person's names.
This field is a singleton for contact sources.
Definition at line 831 of file person.cpp.
◆ nicknames()
The person's nicknames.
Definition at line 444 of file person.cpp.
◆ occupations()
QList< Occupation > KGAPI2::People::Person::occupations | ( | ) | const |
The person's occupations.
Definition at line 998 of file person.cpp.
◆ operator==()
bool KGAPI2::People::Person::operator== | ( | const Person & | other | ) | const |
Definition at line 1570 of file person.cpp.
◆ organizations()
QList< Organization > KGAPI2::People::Person::organizations | ( | ) | const |
The person's past or current organizations.
Definition at line 1086 of file person.cpp.
◆ phoneNumbers()
QList< PhoneNumber > KGAPI2::People::Person::phoneNumbers | ( | ) | const |
The person's phone numbers.
For people.connections.list
and otherContacts.list
the number of phone numbers is limited to 100. If a Person has more phone numbers the entire set can be obtained by calling GetPeople.
Definition at line 919 of file person.cpp.
◆ photos()
◆ relations()
The person's relations.
Definition at line 573 of file person.cpp.
◆ relationshipInterests()
QList< RelationshipInterest > KGAPI2::People::Person::relationshipInterests | ( | ) | const |
Output only.
DEPRECATED: No data will be returned The person's relationship interests.
Definition at line 1048 of file person.cpp.
◆ relationshipStatuses()
QList< RelationshipStatus > KGAPI2::People::Person::relationshipStatuses | ( | ) | const |
Output only.
DEPRECATED: No data will be returned The person's relationship statuses.
Definition at line 544 of file person.cpp.
◆ removeAddress()
void KGAPI2::People::Person::removeAddress | ( | const Address & | value | ) |
Removes the given value
from the list of addresses if it exists.
Definition at line 900 of file person.cpp.
◆ removeBiography()
void KGAPI2::People::Person::removeBiography | ( | const Biography & | value | ) |
Removes the given value
from the list of biographies if it exists.
Definition at line 721 of file person.cpp.
◆ removeBirthday()
void KGAPI2::People::Person::removeBirthday | ( | const Birthday & | value | ) |
Removes the given value
from the list of birthdays if it exists.
Definition at line 563 of file person.cpp.
◆ removeBraggingRights()
void KGAPI2::People::Person::removeBraggingRights | ( | const BraggingRights & | value | ) |
Removes the given value
from the list of braggingRights if it exists.
Definition at line 534 of file person.cpp.
◆ removeCalendarUrl()
void KGAPI2::People::Person::removeCalendarUrl | ( | const CalendarUrl & | value | ) |
Removes the given value
from the list of calendarUrls if it exists.
Definition at line 871 of file person.cpp.
◆ removeClientData()
void KGAPI2::People::Person::removeClientData | ( | const ClientData & | value | ) |
Removes the given value
from the list of clientData if it exists.
Definition at line 509 of file person.cpp.
◆ removeEmailAddress()
void KGAPI2::People::Person::removeEmailAddress | ( | const EmailAddress & | value | ) |
Removes the given value
from the list of emailAddresses if it exists.
Definition at line 484 of file person.cpp.
◆ removeEvent()
void KGAPI2::People::Person::removeEvent | ( | const Event & | value | ) |
Removes the given value
from the list of events if it exists.
Definition at line 984 of file person.cpp.
◆ removeExternalId()
void KGAPI2::People::Person::removeExternalId | ( | const ExternalId & | value | ) |
Removes the given value
from the list of externalIds if it exists.
Definition at line 663 of file person.cpp.
◆ removeFileAs()
void KGAPI2::People::Person::removeFileAs | ( | const FileAs & | value | ) |
Removes the given value
from the list of fileAses if it exists.
Definition at line 1176 of file person.cpp.
◆ removeGender()
void KGAPI2::People::Person::removeGender | ( | const Gender & | value | ) |
Removes the given value
from the list of genders if it exists.
Definition at line 1151 of file person.cpp.
◆ removeImClient()
void KGAPI2::People::Person::removeImClient | ( | const ImClient & | value | ) |
Removes the given value
from the list of imClients if it exists.
Definition at line 959 of file person.cpp.
◆ removeInterest()
void KGAPI2::People::Person::removeInterest | ( | const Interest & | value | ) |
Removes the given value
from the list of interests if it exists.
Definition at line 1076 of file person.cpp.
◆ removeLocation()
void KGAPI2::People::Person::removeLocation | ( | const Location & | value | ) |
Removes the given value
from the list of locations if it exists.
Definition at line 638 of file person.cpp.
◆ removeMembership()
void KGAPI2::People::Person::removeMembership | ( | const Membership & | value | ) |
Removes the given value
from the list of memberships if it exists.
Definition at line 771 of file person.cpp.
◆ removeMiscKeyword()
void KGAPI2::People::Person::removeMiscKeyword | ( | const MiscKeyword & | value | ) |
Removes the given value
from the list of miscKeywords if it exists.
Definition at line 1038 of file person.cpp.
◆ removeName()
void KGAPI2::People::Person::removeName | ( | const Name & | value | ) |
Removes the given value
from the list of names if it exists.
Definition at line 846 of file person.cpp.
◆ removeNickname()
void KGAPI2::People::Person::removeNickname | ( | const Nickname & | value | ) |
Removes the given value
from the list of nicknames if it exists.
Definition at line 459 of file person.cpp.
◆ removeOccupation()
void KGAPI2::People::Person::removeOccupation | ( | const Occupation & | value | ) |
Removes the given value
from the list of occupations if it exists.
Definition at line 1013 of file person.cpp.
◆ removeOrganization()
void KGAPI2::People::Person::removeOrganization | ( | const Organization & | value | ) |
Removes the given value
from the list of organizations if it exists.
Definition at line 1101 of file person.cpp.
◆ removePersonLocale()
void KGAPI2::People::Person::removePersonLocale | ( | const PersonLocale & | value | ) |
Removes the given value
from the list of locales if it exists.
Definition at line 613 of file person.cpp.
◆ removePhoneNumber()
void KGAPI2::People::Person::removePhoneNumber | ( | const PhoneNumber & | value | ) |
Removes the given value
from the list of phoneNumbers if it exists.
Definition at line 934 of file person.cpp.
◆ removeRelation()
void KGAPI2::People::Person::removeRelation | ( | const Relation & | value | ) |
Removes the given value
from the list of relations if it exists.
Definition at line 588 of file person.cpp.
◆ removeResidence()
void KGAPI2::People::Person::removeResidence | ( | const Residence & | value | ) |
Removes the given value
from the list of residences if it exists.
Definition at line 821 of file person.cpp.
◆ removeSipAddress()
void KGAPI2::People::Person::removeSipAddress | ( | const SipAddress & | value | ) |
Removes the given value
from the list of sipAddresses if it exists.
Definition at line 746 of file person.cpp.
◆ removeSkill()
void KGAPI2::People::Person::removeSkill | ( | const Skill & | value | ) |
Removes the given value
from the list of skills if it exists.
Definition at line 796 of file person.cpp.
◆ removeUrl()
void KGAPI2::People::Person::removeUrl | ( | const Url & | value | ) |
Removes the given value
from the list of urls if it exists.
Definition at line 1126 of file person.cpp.
◆ removeUserDefined()
void KGAPI2::People::Person::removeUserDefined | ( | const UserDefined & | value | ) |
Removes the given value
from the list of userDefined if it exists.
Definition at line 696 of file person.cpp.
◆ residences()
DEPRECATED: (Please use person.locations
instead) The person's residences.
Definition at line 806 of file person.cpp.
◆ resourceName()
QString KGAPI2::People::Person::resourceName | ( | ) | const |
The resource name for the person, assigned by the server.
An ASCII string with a max length of 27 characters, in the form of people/{person_id}
.
Definition at line 1052 of file person.cpp.
◆ setAddresses()
Sets value of the addresses property.
Definition at line 890 of file person.cpp.
◆ setBiographies()
Sets value of the biographies property.
Definition at line 711 of file person.cpp.
◆ setBirthdays()
Sets value of the birthdays property.
Definition at line 553 of file person.cpp.
◆ setBraggingRights()
void KGAPI2::People::Person::setBraggingRights | ( | const QList< BraggingRights > & | value | ) |
Sets value of the braggingRights property.
Definition at line 524 of file person.cpp.
◆ setCalendarUrls()
void KGAPI2::People::Person::setCalendarUrls | ( | const QList< CalendarUrl > & | value | ) |
Sets value of the calendarUrls property.
Definition at line 861 of file person.cpp.
◆ setClientData()
void KGAPI2::People::Person::setClientData | ( | const QList< ClientData > & | value | ) |
Sets value of the clientData property.
Definition at line 499 of file person.cpp.
◆ setEmailAddresses()
void KGAPI2::People::Person::setEmailAddresses | ( | const QList< EmailAddress > & | value | ) |
Sets value of the emailAddresses property.
Definition at line 474 of file person.cpp.
◆ setEtag()
void KGAPI2::People::Person::setEtag | ( | const QString & | value | ) |
Sets value of the etag property.
Definition at line 915 of file person.cpp.
◆ setEvents()
Sets value of the events property.
Definition at line 974 of file person.cpp.
◆ setExternalIds()
void KGAPI2::People::Person::setExternalIds | ( | const QList< ExternalId > & | value | ) |
Sets value of the externalIds property.
Definition at line 653 of file person.cpp.
◆ setFileAses()
Sets value of the fileAses property.
Definition at line 1166 of file person.cpp.
◆ setGenders()
Sets value of the genders property.
Definition at line 1141 of file person.cpp.
◆ setImClients()
Sets value of the imClients property.
Definition at line 949 of file person.cpp.
◆ setInterests()
Sets value of the interests property.
Definition at line 1066 of file person.cpp.
◆ setLocales()
void KGAPI2::People::Person::setLocales | ( | const QList< PersonLocale > & | value | ) |
Sets value of the locales property.
Definition at line 603 of file person.cpp.
◆ setLocations()
Sets value of the locations property.
Definition at line 628 of file person.cpp.
◆ setMemberships()
void KGAPI2::People::Person::setMemberships | ( | const QList< Membership > & | value | ) |
Sets value of the memberships property.
Definition at line 761 of file person.cpp.
◆ setMiscKeywords()
void KGAPI2::People::Person::setMiscKeywords | ( | const QList< MiscKeyword > & | value | ) |
Sets value of the miscKeywords property.
Definition at line 1028 of file person.cpp.
◆ setNames()
Sets value of the names property.
Definition at line 836 of file person.cpp.
◆ setNicknames()
Sets value of the nicknames property.
Definition at line 449 of file person.cpp.
◆ setOccupations()
void KGAPI2::People::Person::setOccupations | ( | const QList< Occupation > & | value | ) |
Sets value of the occupations property.
Definition at line 1003 of file person.cpp.
◆ setOrganizations()
void KGAPI2::People::Person::setOrganizations | ( | const QList< Organization > & | value | ) |
Sets value of the organizations property.
Definition at line 1091 of file person.cpp.
◆ setPhoneNumbers()
void KGAPI2::People::Person::setPhoneNumbers | ( | const QList< PhoneNumber > & | value | ) |
Sets value of the phoneNumbers property.
Definition at line 924 of file person.cpp.
◆ setRelations()
Sets value of the relations property.
Definition at line 578 of file person.cpp.
◆ setResidences()
Sets value of the residences property.
Definition at line 811 of file person.cpp.
◆ setResourceName()
void KGAPI2::People::Person::setResourceName | ( | const QString & | value | ) |
Sets value of the resourceName property.
Definition at line 1057 of file person.cpp.
◆ setSipAddresses()
void KGAPI2::People::Person::setSipAddresses | ( | const QList< SipAddress > & | value | ) |
Sets value of the sipAddresses property.
Definition at line 736 of file person.cpp.
◆ setSkills()
Sets value of the skills property.
Definition at line 786 of file person.cpp.
◆ setUrls()
Sets value of the urls property.
Definition at line 1116 of file person.cpp.
◆ setUserDefined()
void KGAPI2::People::Person::setUserDefined | ( | const QList< UserDefined > & | value | ) |
Sets value of the userDefined property.
Definition at line 686 of file person.cpp.
◆ sipAddresses()
QList< SipAddress > KGAPI2::People::Person::sipAddresses | ( | ) | const |
The person's SIP addresses.
Definition at line 731 of file person.cpp.
◆ skills()
The person's skills.
Definition at line 781 of file person.cpp.
◆ taglines()
Output only.
DEPRECATED: No data will be returned The person's taglines.
Definition at line 677 of file person.cpp.
◆ toJSON()
QJsonValue KGAPI2::People::Person::toJSON | ( | ) | const |
Definition at line 1305 of file person.cpp.
◆ toKContactsAddressee()
KContacts::Addressee KGAPI2::People::Person::toKContactsAddressee | ( | ) | const |
Definition at line 1558 of file person.cpp.
◆ urls()
The person's associated URLs.
Definition at line 1111 of file person.cpp.
◆ userDefined()
QList< UserDefined > KGAPI2::People::Person::userDefined | ( | ) | const |
The person's user defined data.
Definition at line 681 of file person.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Apr 27 2024 22:14:20 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.