MessageList::Core::Item
#include <item.h>
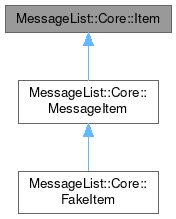
Classes | |
class | ChildItemStats |
Public Types | |
enum | InitialExpandStatus : uint8_t { ExpandNeeded , NoExpandNeeded , ExpandExecuted } |
enum | Type : uint8_t { GroupHeader , Message , InvisibleRoot } |
Protected Member Functions | |
Item (Type type) | |
Item (Type type, ItemPrivate *dd) | |
Protected Attributes | |
ItemPrivate *const | d_ptr |
Detailed Description
A single item of the MessageList tree managed by MessageList::Model.
This class stores basic information needed in all the subclasses which at the moment of writing are MessageItem and GroupHeaderItem.
Member Enumeration Documentation
◆ InitialExpandStatus
enum MessageList::Core::Item::InitialExpandStatus : uint8_t |
Specifies the initial expand status for the item that should be applied when it's attached to the viewable tree.
Needed as a workaround for QTreeView limitations in handling item expansion.
Enumerator | |
---|---|
ExpandNeeded | Must expand when this item becomes viewable. |
NoExpandNeeded | No expand needed at all. |
ExpandExecuted | Item already expanded. |
◆ Type
enum MessageList::Core::Item::Type : uint8_t |
Constructor & Destructor Documentation
◆ Item()
|
protected |
◆ ~Item()
|
virtual |
Member Function Documentation
◆ appendChildItem()
◆ childItem()
|
nodiscard |
◆ childItemCount()
|
nodiscard |
◆ childItems()
◆ childItemStats()
void Item::childItemStats | ( | ChildItemStats & | stats | ) | const |
◆ date()
◆ deepestItem()
|
nodiscard |
◆ displayReceiver()
|
nodiscard |
◆ displaySender()
|
nodiscard |
◆ displaySenderOrReceiver()
|
nodiscard |
◆ dump()
void Item::dump | ( | const QString & | prefix | ) |
◆ firstChildItem()
|
nodiscard |
◆ folder()
const QString & Item::folder | ( | ) | const |
◆ formattedDate()
|
nodiscard |
◆ formattedMaxDate()
|
nodiscard |
◆ formattedSize()
|
nodiscard |
◆ hasAncestor()
|
nodiscard |
◆ hasChildren()
|
nodiscard |
◆ indexOfChildItem()
int Item::indexOfChildItem | ( | Item * | item | ) | const |
◆ initialExpandStatus()
|
nodiscard |
◆ initialSetup()
◆ isViewable()
|
nodiscard |
◆ itemAbove()
|
nodiscard |
◆ itemAboveChild()
◆ itemBelow()
|
nodiscard |
◆ itemBelowChild()
◆ itemId()
◆ killAllChildItems()
void Item::killAllChildItems | ( | ) |
◆ maxDate()
time_t Item::maxDate | ( | ) | const |
◆ parent()
|
nodiscard |
◆ parentCollectionId()
◆ rawAppendChildItem()
void Item::rawAppendChildItem | ( | Item * | child | ) |
◆ receiver()
const QString & Item::receiver | ( | ) | const |
◆ recomputeMaxDate()
bool Item::recomputeMaxDate | ( | ) |
◆ sender()
|
nodiscard |
◆ senderOrReceiver()
const QString & Item::senderOrReceiver | ( | ) | const |
Returns the sender or the receiver, depending on the underlying StorageModel settings.
◆ setDate()
void Item::setDate | ( | time_t | date | ) |
◆ setFolder()
void Item::setFolder | ( | const QString & | folder | ) |
◆ setIndexGuess()
void Item::setIndexGuess | ( | int | index | ) |
Sets the cached guess for the index of this item in the parent's child list.
This is used to speed up the index lookup with the following algorithm: Ask the parent if this item is at the position specified by index guess (this costs ~O(1)). If the position matches we have finished, if it doesn't then perform a linear search via indexOfChildItem() (which costs ~O(n)).
◆ setInitialExpandStatus()
void Item::setInitialExpandStatus | ( | InitialExpandStatus | initialExpandStatus | ) |
◆ setItemId()
◆ setMaxDate()
void Item::setMaxDate | ( | time_t | date | ) |
◆ setParent()
void Item::setParent | ( | Item * | pParent | ) |
◆ setParentCollectionId()
◆ setReceiver()
void Item::setReceiver | ( | const QString & | receiver | ) |
◆ setSender()
void Item::setSender | ( | const QString & | sender | ) |
◆ setSize()
void Item::setSize | ( | size_t | size | ) |
◆ setStatus()
void Item::setStatus | ( | Akonadi::MessageStatus | status | ) |
◆ setSubject()
void Item::setSubject | ( | const QString & | subject | ) |
◆ setSubjectAndStatus()
void Item::setSubjectAndStatus | ( | const QString & | subject, |
Akonadi::MessageStatus | status ) |
This is meant to be called right after the constructor for MessageItem objects.
It sets up several items at once (so even if not inlined it's still a single call).
◆ setViewable()
void Item::setViewable | ( | Model * | model, |
bool | bViewable ) |
◆ size()
size_t Item::size | ( | ) | const |
◆ status()
const Akonadi::MessageStatus & Item::status | ( | ) | const |
◆ statusDescription()
|
nodiscard |
◆ subject()
const QString & Item::subject | ( | ) | const |
◆ takeChildItem()
◆ topmostNonRoot()
|
nodiscard |
◆ type()
|
nodiscard |
◆ useReceiver()
bool Item::useReceiver | ( | ) | const |
Member Data Documentation
◆ d_ptr
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 31 2025 12:05:42 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.