MessageList::Core::MessageItem
#include <messageitem.h>
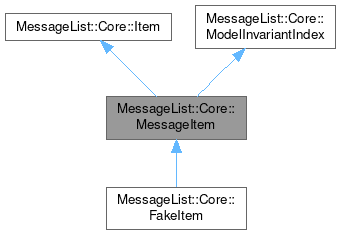
Public Types | |
enum | EncryptionState { NotEncrypted , PartiallyEncrypted , FullyEncrypted , EncryptionStateUnknown } |
enum | SignatureState { NotSigned , PartiallySigned , FullySigned , SignatureStateUnknown } |
enum | ThreadingStatus { PerfectParentFound , ImperfectParentFound , ParentMissing , NonThreadable } |
![]() | |
enum | InitialExpandStatus : uint8_t { ExpandNeeded , NoExpandNeeded , ExpandExecuted } |
enum | Type : uint8_t { GroupHeader , Message , InvisibleRoot } |
Static Public Member Functions | |
static void | setGeneralFont (const QFont &font) |
static void | setImportantMessageColor (const QColor &color) |
static void | setImportantMessageFont (const QFont &font) |
static void | setToDoMessageColor (const QColor &color) |
static void | setToDoMessageFont (const QFont &font) |
static void | setUnreadMessageColor (const QColor &color) |
static void | setUnreadMessageFont (const QFont &font) |
Protected Member Functions | |
MessageItem (MessageItemPrivate *dd) | |
![]() | |
Item (Type type) | |
Item (Type type, ItemPrivate *dd) | |
Additional Inherited Members | |
![]() | |
ItemPrivate *const | d_ptr |
Detailed Description
The MessageItem class.
Definition at line 35 of file messageitem.h.
Member Enumeration Documentation
◆ EncryptionState
enum MessageList::Core::MessageItem::EncryptionState |
Definition at line 68 of file messageitem.h.
◆ SignatureState
enum MessageList::Core::MessageItem::SignatureState |
Definition at line 75 of file messageitem.h.
◆ ThreadingStatus
Definition at line 61 of file messageitem.h.
Constructor & Destructor Documentation
◆ MessageItem() [1/2]
|
explicit |
Definition at line 219 of file messageitem.cpp.
◆ MessageItem() [2/2]
|
explicitprotected |
Definition at line 225 of file messageitem.cpp.
Member Function Documentation
◆ aboutToBeRemoved()
|
nodiscard |
Definition at line 418 of file messageitem.cpp.
◆ accessibleText()
QString MessageItem::accessibleText | ( | const MessageList::Core::Theme * | theme, |
int | columnIndex ) |
Definition at line 497 of file messageitem.cpp.
◆ akonadiItem()
Akonadi::Item MessageList::Core::MessageItem::akonadiItem | ( | ) | const |
Definition at line 448 of file messageitem.cpp.
◆ backgroundColor()
const QColor & MessageItem::backgroundColor | ( | ) | const |
Definition at line 297 of file messageitem.cpp.
◆ encryptionState()
|
nodiscard |
Definition at line 346 of file messageitem.cpp.
◆ findTag()
const MessageItem::Tag * MessageItem::findTag | ( | const QString & | szTagId | ) | const |
Returns Tag associated to this message that has the specified id or 0 if no such tag exists.
mTagList will be 0 in 99% of the cases.
Definition at line 250 of file messageitem.cpp.
◆ inReplyToIdMD5()
|
nodiscard |
Definition at line 370 of file messageitem.cpp.
◆ invalidateTagCache()
void MessageItem::invalidateTagCache | ( | ) |
Deletes all cached tags.
The next time someone asks this item for the tags, they are fetched again
Definition at line 271 of file messageitem.cpp.
◆ isBold()
|
inlinenodiscard |
Definition at line 105 of file messageitem.h.
◆ isItalic()
|
inlinenodiscard |
Definition at line 110 of file messageitem.h.
◆ messageIdMD5()
|
nodiscard |
Definition at line 358 of file messageitem.cpp.
◆ referencesIdMD5()
|
nodiscard |
Definition at line 382 of file messageitem.cpp.
◆ setAboutToBeRemoved()
void MessageItem::setAboutToBeRemoved | ( | bool | aboutToBeRemoved | ) |
Definition at line 424 of file messageitem.cpp.
◆ setAkonadiItem()
void MessageList::Core::MessageItem::setAkonadiItem | ( | const Akonadi::Item & | item | ) |
Definition at line 454 of file messageitem.cpp.
◆ setEncryptionState()
void MessageItem::setEncryptionState | ( | EncryptionState | state | ) |
Definition at line 352 of file messageitem.cpp.
◆ setGeneralFont()
|
static |
Definition at line 552 of file messageitem.cpp.
◆ setImportantMessageColor()
|
static |
Definition at line 542 of file messageitem.cpp.
◆ setImportantMessageFont()
|
static |
Definition at line 562 of file messageitem.cpp.
◆ setInReplyToIdMD5()
void MessageItem::setInReplyToIdMD5 | ( | MD5Hash | md5 | ) |
Definition at line 376 of file messageitem.cpp.
◆ setMessageIdMD5()
void MessageItem::setMessageIdMD5 | ( | MD5Hash | md5 | ) |
Definition at line 364 of file messageitem.cpp.
◆ setReferencesIdMD5()
void MessageItem::setReferencesIdMD5 | ( | MD5Hash | md5 | ) |
Definition at line 388 of file messageitem.cpp.
◆ setSignatureState()
void MessageItem::setSignatureState | ( | SignatureState | state | ) |
Definition at line 340 of file messageitem.cpp.
◆ setStrippedSubjectMD5()
void MessageItem::setStrippedSubjectMD5 | ( | MD5Hash | md5 | ) |
Definition at line 412 of file messageitem.cpp.
◆ setSubjectIsPrefixed()
void MessageItem::setSubjectIsPrefixed | ( | bool | subjectIsPrefixed | ) |
Definition at line 394 of file messageitem.cpp.
◆ setThreadingStatus()
void MessageItem::setThreadingStatus | ( | ThreadingStatus | threadingStatus | ) |
Definition at line 436 of file messageitem.cpp.
◆ setToDoMessageColor()
|
static |
Definition at line 547 of file messageitem.cpp.
◆ setToDoMessageFont()
|
static |
Definition at line 567 of file messageitem.cpp.
◆ setUnreadMessageColor()
|
static |
Definition at line 537 of file messageitem.cpp.
◆ setUnreadMessageFont()
|
static |
Definition at line 557 of file messageitem.cpp.
◆ signatureState()
|
nodiscard |
Definition at line 334 of file messageitem.cpp.
◆ strippedSubjectMD5()
|
nodiscard |
Definition at line 406 of file messageitem.cpp.
◆ subjectIsPrefixed()
|
nodiscard |
Definition at line 400 of file messageitem.cpp.
◆ subTreeToList()
void MessageItem::subTreeToList | ( | QList< MessageItem * > & | list | ) |
Appends the whole subtree originating at this item to the specified list.
This item is included!
Definition at line 524 of file messageitem.cpp.
◆ tagList()
|
virtual |
Returns the list of tags for this item.
Reimplemented in MessageList::Core::FakeItem.
Definition at line 233 of file messageitem.cpp.
◆ tagListDescription()
|
nodiscard |
Definition at line 256 of file messageitem.cpp.
◆ textColor()
const QColor & MessageItem::textColor | ( | ) | const |
Definition at line 277 of file messageitem.cpp.
◆ threadingStatus()
|
nodiscard |
Definition at line 430 of file messageitem.cpp.
◆ topmostMessage()
MessageItem * MessageItem::topmostMessage | ( | ) |
Definition at line 460 of file messageitem.cpp.
◆ uniqueId()
|
nodiscard |
Definition at line 442 of file messageitem.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:51:55 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.