KDbValidator
#include <KDbValidator.h>
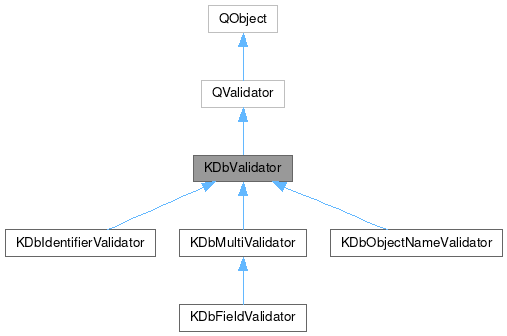
Public Types | |
enum | Result { Error = 0 , Ok = 1 , Warning = 2 } |
![]() | |
enum | State |
Public Member Functions | |
KDbValidator (QObject *parent=nullptr) | |
bool | acceptsEmptyValue () const |
void | addChildValidator (KDbValidator *v) |
Result | check (const QString &valueName, const QVariant &v, QString *message, QString *details) |
void | setAcceptsEmptyValue (bool set) |
QValidator::State | validate (QString &input, int &pos) const override |
![]() | |
QValidator (QObject *parent) | |
void | changed () |
virtual void | fixup (QString &input) const const |
QLocale | locale () const const |
void | setLocale (const QLocale &locale) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static const QString | messageColumnNotEmpty () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
virtual Result | internalCheck (const QString &valueName, const QVariant &value, QString *message, QString *details) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
Acceptable | |
Intermediate | |
Invalid | |
![]() | |
typedef | QObjectList |
Detailed Description
A validator extending QValidator with offline-checking for value's validity.
The offline-checking for value's validity is provided by KDbValidator::check() method. The validator groups two purposes into one container:
- string validator for line editors (online checking, "on typing");
- offline-checking for QVariant values, reimplementing validate().
It also offers error and warning messages for check() method. You may need to reimplement:
- QValidator::State validate(QString& input, int& pos) const
- Result check(const QString &valueName, const QVariant &v, QString *message, QString *details)
Definition at line 41 of file KDbValidator.h.
Member Enumeration Documentation
◆ Result
enum KDbValidator::Result |
Definition at line 45 of file KDbValidator.h.
Constructor & Destructor Documentation
◆ KDbValidator()
|
explicit |
Definition at line 49 of file KDbValidator.cpp.
◆ ~KDbValidator()
|
override |
Definition at line 55 of file KDbValidator.cpp.
Member Function Documentation
◆ acceptsEmptyValue()
bool KDbValidator::acceptsEmptyValue | ( | ) | const |
- Returns
- true if the validator accepts empty values
- See also
- setAcceptsEmptyValue()
Definition at line 95 of file KDbValidator.cpp.
◆ addChildValidator()
void KDbValidator::addChildValidator | ( | KDbValidator * | v | ) |
Adds a child validator v.
◆ check()
KDbValidator::Result KDbValidator::check | ( | const QString & | valueName, |
const QVariant & | v, | ||
QString * | message, | ||
QString * | details ) |
Checks if value value is ok and returns one of Result value:
- Error is returned on error;
- Ok on success;
- Warning if there is something to warn about. In any case except Ok, i18n'ed message will be set in message and (optionally) datails are set in details, e.g. for use in a message box. valueName can be used to contruct message as well, for example: "[valueName] is not a valid login name". Depending on acceptsEmptyValue(), immediately accepts empty values or not.
Definition at line 60 of file KDbValidator.cpp.
◆ internalCheck()
|
protectedvirtual |
Definition at line 75 of file KDbValidator.cpp.
◆ messageColumnNotEmpty()
|
static |
A generic error/warning message "... value has to be entered.".
Definition at line 100 of file KDbValidator.cpp.
◆ setAcceptsEmptyValue()
void KDbValidator::setAcceptsEmptyValue | ( | bool | set | ) |
Sets accepting empty values on (true) or off (false). By default the validator does not accepts empty values.
Definition at line 90 of file KDbValidator.cpp.
◆ validate()
|
overridevirtual |
This implementation always returns value QValidator::Acceptable.
Implements QValidator.
Definition at line 85 of file KDbValidator.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:05:18 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.