Scripting::Field
#include <KReportScriptField.h>
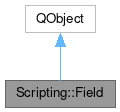
Public Slots | |
QColor | backgroundColor () const |
int | backgroundOpacity () const |
QColor | foregroundColor () const |
int | horizontalAlignment () const |
QColor | lineColor () const |
int | lineStyle () const |
int | lineWeight () const |
QPointF | position () const |
void | setBackgroundColor (const QColor &) |
void | setBackgroundOpacity (int) |
void | setForegroundColor (const QColor &) |
void | setHorizonalAlignment (int) |
void | setLineColor (const QColor &) |
void | setLineStyle (int) |
void | setLineWeight (int) |
void | setPosition (const QPointF &) |
void | setSize (const QSizeF &) |
void | setSource (const QString &s) |
void | setVerticalAlignment (int) |
QSizeF | size () const |
QString | source () const |
int | verticalAlignment () const |
Public Member Functions | |
Field (KReportItemField *) | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Field script interface.
The user facing interface for scripting report fields
Definition at line 37 of file KReportScriptField.h.
Constructor & Destructor Documentation
◆ Field()
|
explicit |
Definition at line 26 of file KReportScriptField.cpp.
◆ ~Field()
|
override |
Definition at line 32 of file KReportScriptField.cpp.
Member Function Documentation
◆ backgroundColor
|
slot |
- Returns
- the background color of the lable
Definition at line 112 of file KReportScriptField.cpp.
◆ backgroundOpacity
|
slot |
- Returns
- the opacity of the field
Definition at line 130 of file KReportScriptField.cpp.
◆ foregroundColor
|
slot |
- Returns
- the foreground (text) color of the field
Definition at line 121 of file KReportScriptField.cpp.
◆ horizontalAlignment
|
slot |
- Returns
- the horizontal alignment as an integer Valid values are left: -1, center: 0, right; 1
Definition at line 46 of file KReportScriptField.cpp.
◆ lineColor
|
slot |
- Returns
- the border line color of the field
Definition at line 139 of file KReportScriptField.cpp.
◆ lineStyle
|
slot |
- Returns
- the border line style of the field. Values are from Qt::Penstyle range 0-5
Definition at line 157 of file KReportScriptField.cpp.
◆ lineWeight
|
slot |
- Returns
- the border line weight (thickness) of the field
Definition at line 148 of file KReportScriptField.cpp.
◆ position
|
slot |
- Returns
- the position of the field in points
Definition at line 169 of file KReportScriptField.cpp.
◆ setBackgroundColor
|
slot |
Set the background color of the field to the given color.
Definition at line 116 of file KReportScriptField.cpp.
◆ setBackgroundOpacity
|
slot |
Sets the background opacity of the field Valid values are in the range 0-100.
Definition at line 134 of file KReportScriptField.cpp.
◆ setForegroundColor
|
slot |
Sets the foreground (text) color of the field to the given color.
Definition at line 125 of file KReportScriptField.cpp.
◆ setHorizonalAlignment
|
slot |
Sets the horizontal alignment Valid values for alignment are left: -1, center: 0, right; 1.
Definition at line 61 of file KReportScriptField.cpp.
◆ setLineColor
|
slot |
Sets the border line color of the field to the given color.
Definition at line 143 of file KReportScriptField.cpp.
◆ setLineStyle
|
slot |
Sets the border line style of the field to the given style in the range 0-5.
Definition at line 161 of file KReportScriptField.cpp.
◆ setLineWeight
|
slot |
Sets the border line weight (thickness) of the field.
Definition at line 152 of file KReportScriptField.cpp.
◆ setPosition
|
slot |
Sets the position of the field to the given point coordinates.
Definition at line 173 of file KReportScriptField.cpp.
◆ setSize
|
slot |
Sets the size of the field to the given size in points.
Definition at line 182 of file KReportScriptField.cpp.
◆ setSource
|
slot |
Sets the data source for the field element.
- See also
- source()
Definition at line 41 of file KReportScriptField.cpp.
◆ setVerticalAlignment
|
slot |
Sets the vertical alignment Valid values for aligmnt are top: -1, middle: 0, bottom: 1.
Definition at line 94 of file KReportScriptField.cpp.
◆ size
|
slot |
- Returns
- the size of the field in points
Definition at line 178 of file KReportScriptField.cpp.
◆ source
|
slot |
- Returns
- the data source for the field element The source can be a column name or a valid script expression if prefixed with a '='.
Definition at line 36 of file KReportScriptField.cpp.
◆ verticalAlignment
|
slot |
- Returns
- the vertical alignment Valid values are top: -1, middle: 0, bottom: 1
Definition at line 79 of file KReportScriptField.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 28 2025 11:58:24 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.