ManagedColor
#include <ManagedColor.h>
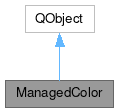
Public Member Functions | |
ManagedColor (const QString &colorModel, const QString &colorDepth, const QString &colorProfile, QObject *parent=0) | |
ManagedColor (KoColor color, QObject *parent=0) | |
ManagedColor (QObject *parent=0) | |
QString | colorDepth () const |
QColor | colorForCanvas (Canvas *canvas) const |
QString | colorModel () const |
QString | colorProfile () const |
QVector< float > | components () const |
QVector< float > | componentsOrdered () const |
void | fromXML (const QString &xml) |
bool | operator== (const ManagedColor &other) const |
bool | setColorProfile (const QString &colorProfile) |
bool | setColorSpace (const QString &colorModel, const QString &colorDepth, const QString &colorProfile) |
void | setComponents (const QVector< float > &values) |
QString | toQString () |
QString | toXML () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static ManagedColor * | fromQColor (const QColor &qcolor, Canvas *canvas=0) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The ManagedColor class is a class to handle colors that are color managed.
A managed color is a color of which we know the model(RGB, LAB, CMYK, etc), the bitdepth and the specific properties of its colorspace, such as the whitepoint, chromaticities, trc, etc, as represented by the color profile.
Krita has two color management systems. LCMS and OCIO. LCMS is the one handling the ICC profile stuff, and the major one handling that ManagedColor deals with. OCIO support is only in the display of the colors. ManagedColor has some support for it in colorForCanvas()
All colors in Krita are color managed. QColors are understood as RGB-type colors in the sRGB space.
We recommend you make a color like this:
Definition at line 45 of file ManagedColor.h.
Constructor & Destructor Documentation
◆ ManagedColor() [1/3]
|
explicit |
ManagedColor Create a ManagedColor that is black and transparent.
Definition at line 26 of file ManagedColor.cpp.
◆ ManagedColor() [2/3]
ManagedColor::ManagedColor | ( | const QString & | colorModel, |
const QString & | colorDepth, | ||
const QString & | colorProfile, | ||
QObject * | parent = 0 ) |
ManagedColor create a managed color with the given color space properties.
- See also
- setColorModel() for more details.
Definition at line 33 of file ManagedColor.cpp.
◆ ManagedColor() [3/3]
ManagedColor::ManagedColor | ( | KoColor | color, |
QObject * | parent = 0 ) |
Definition at line 44 of file ManagedColor.cpp.
◆ ~ManagedColor()
|
override |
Definition at line 51 of file ManagedColor.cpp.
Member Function Documentation
◆ colorDepth()
QString ManagedColor::colorDepth | ( | ) | const |
colorDepth A string describing the color depth of the image:
- U8: unsigned 8 bits integer, the most common type
- U16: unsigned 16 bits integer
- F16: half, 16 bits floating point. Only available if Krita was built with OpenEXR
- F32: 32 bits floating point
- Returns
- the color depth.
Definition at line 92 of file ManagedColor.cpp.
◆ colorForCanvas()
colorForCanvas
- Parameters
-
canvas the canvas whose color management you'd like to use. In Krita, different views have separate canvasses, and these can have different OCIO configurations active.
- Returns
- the QColor as it would be displaying on the canvas. This result can be used to draw widgets with the correct configuration applied.
Definition at line 60 of file ManagedColor.cpp.
◆ colorModel()
QString ManagedColor::colorModel | ( | ) | const |
colorModel retrieve the current color model of this document:
- A: Alpha mask
- RGBA: RGB with alpha channel (The actual order of channels is most often BGR!)
- XYZA: XYZ with alpha channel
- LABA: LAB with alpha channel
- CMYKA: CMYK with alpha channel
- GRAYA: Gray with alpha channel
- YCbCrA: YCbCr with alpha channel
- Returns
- the internal color model string.
Definition at line 97 of file ManagedColor.cpp.
◆ colorProfile()
QString ManagedColor::colorProfile | ( | ) | const |
- Returns
- the name of the current color profile
Definition at line 102 of file ManagedColor.cpp.
◆ components()
QVector< float > ManagedColor::components | ( | ) | const |
components
- Returns
- a QVector containing the channel/components of this color normalized. This includes the alphachannel.
Definition at line 123 of file ManagedColor.cpp.
◆ componentsOrdered()
QVector< float > ManagedColor::componentsOrdered | ( | ) | const |
- Returns
- same as Components, except the values are ordered to the display.
Definition at line 130 of file ManagedColor.cpp.
◆ fromQColor()
|
static |
fromQColor is the (approximate) reverse of colorForCanvas()
- Parameters
-
qcolor the QColor to convert to a KoColor. canvas the canvas whose color management you'd like to use.
- Returns
- the approximated ManagedColor, to use for canvas resources.
Definition at line 76 of file ManagedColor.cpp.
◆ fromXML()
void ManagedColor::fromXML | ( | const QString & | xml | ) |
Unserialize a color following Create's swatch color specification available at https://web.archive.org/web/20110826002520/http://create.freedesktop.org/wiki/Swatches_-_color_file_format/Draft.
- Parameters
-
xml an XML color
- Returns
- the unserialized color, or an empty color object if the function failed to unserialize the color
Definition at line 157 of file ManagedColor.cpp.
◆ operator==()
bool ManagedColor::operator== | ( | const ManagedColor & | other | ) | const |
Definition at line 55 of file ManagedColor.cpp.
◆ setColorProfile()
bool ManagedColor::setColorProfile | ( | const QString & | colorProfile | ) |
setColorProfile set the color profile of the image to the given profile.
The profile has to be registered with krita and be compatible with the current color model and depth; the image data is not converted.
- Parameters
-
colorProfile
- Returns
- false if the colorProfile name does not correspond to to a registered profile or if assigning the profile failed.
Definition at line 107 of file ManagedColor.cpp.
◆ setColorSpace()
bool ManagedColor::setColorSpace | ( | const QString & | colorModel, |
const QString & | colorDepth, | ||
const QString & | colorProfile ) |
setColorSpace convert the nodes and the image to the given colorspace.
The conversion is done with Perceptual as intent, High Quality and No LCMS Optimizations as flags and no blackpoint compensation.
- Parameters
-
colorModel A string describing the color model of the image: - A: Alpha mask
- RGBA: RGB with alpha channel (The actual order of channels is most often BGR!)
- XYZA: XYZ with alpha channel
- LABA: LAB with alpha channel
- CMYKA: CMYK with alpha channel
- GRAYA: Gray with alpha channel
- YCbCrA: YCbCr with alpha channel
colorDepth A string describing the color depth of the image: - U8: unsigned 8 bits integer, the most common type
- U16: unsigned 16 bits integer
- F16: half, 16 bits floating point. Only available if Krita was built with OpenEXR
- F32: 32 bits floating point
colorProfile a valid color profile for this color model and color depth combination.
- Returns
- false the combination of these arguments does not correspond to a colorspace.
Definition at line 115 of file ManagedColor.cpp.
◆ setComponents()
void ManagedColor::setComponents | ( | const QVector< float > & | values | ) |
setComponents Set the channel/components with normalized values.
For integer colorspace, this obviously means the limit is between 0.0-1.0, but for floating point colorspaces, 2.4 or 103.5 are still meaningful (if bright) values.
- Parameters
-
values the QVector containing the new channel/component values. These should be normalized.
Definition at line 142 of file ManagedColor.cpp.
◆ toQString()
QString ManagedColor::toQString | ( | ) |
toQString create a user-visible string of the channel names and the channel values
- Returns
- a string that can be used to display the values of this color to the user.
Definition at line 171 of file ManagedColor.cpp.
◆ toXML()
QString ManagedColor::toXML | ( | ) | const |
Serialize this color following Create's swatch color specification available at https://web.archive.org/web/20110826002520/http://create.freedesktop.org/wiki/Swatches_-_color_file_format/Draft.
Definition at line 147 of file ManagedColor.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:12:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.