Palette
#include <Palette.h>
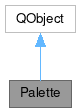
Public Slots | |
void | addEntry (Swatch entry, QString groupName=QString()) |
void | addGroup (QString name) |
Q_DECL_DEPRECATED void | changeGroupName (QString oldGroupName, QString newGroupName) |
int | colorsCountTotal () |
Q_DECL_DEPRECATED Swatch * | colorSetEntryByIndex (int index) |
Q_DECL_DEPRECATED Swatch * | colorSetEntryFromGroup (int index, const QString &groupName) |
int | columnCount () |
QString | comment () |
Swatch * | entryByIndex (int index) |
Swatch * | entryByIndexFromGroup (int index, const QString &groupName) |
QStringList | groupNames () const |
void | moveGroup (const QString &groupName, const QString &groupNameInsertBefore=QString()) |
int | numberOfEntries () const |
void | removeEntry (int index, const QString &groupName) |
void | removeGroup (QString name, bool keepColors=true) |
void | renameGroup (QString oldGroupName, QString newGroupName) |
bool | save () |
void | setColumnCount (int columns) |
void | setComment (QString comment) |
Public Member Functions | |
Palette (Resource *resource, QObject *parent=0) | |
bool | operator!= (const Palette &other) const |
bool | operator== (const Palette &other) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The Palette class Palette is a resource object that stores organised color data.
It's purpose is to allow artists to save colors and store them.
An example for printing all the palettes and the entries:
Constructor & Destructor Documentation
◆ Palette()
Definition at line 18 of file Palette.cpp.
◆ ~Palette()
|
override |
Definition at line 24 of file Palette.cpp.
Member Function Documentation
◆ addEntry
add a color entry to a group.
Color is appended to the end.
- Parameters
-
entry the entry groupName the name of the group to add to.
Definition at line 125 of file Palette.cpp.
◆ addGroup
|
slot |
Palette content can be organized in groups.
This method allows to add a new group in palette.
- Parameters
-
name The name of the new group to add.
Definition at line 76 of file Palette.cpp.
◆ changeGroupName
changeGroupName change the group name.
DEPRECATED: use renameGroup()
instead
- Parameters
-
oldGroupName the old groupname to change. newGroupName the new name to change it into.
- Returns
- whether successful. Reasons for failure include not knowing have oldGroupName
Definition at line 150 of file Palette.cpp.
◆ colorsCountTotal
|
slot |
number of colors (swatches) in palette NOTE: same as numberOfEntries()
- Returns
- total number of colors
Definition at line 88 of file Palette.cpp.
◆ colorSetEntryByIndex
|
slot |
colorSetEntryByIndex get the colorsetEntry from the global index.
DEPRECATED: use entryByIndex()
instead
- Parameters
-
index the global index
- Returns
- the colorset entry
Definition at line 94 of file Palette.cpp.
◆ colorSetEntryFromGroup
colorSetEntryFromGroup
DEPRECATED: use entryByIndexFromGroup()
instead
- Parameters
-
index index in the group. groupName the name of the group to get the color from.
- Returns
- the colorsetentry.
Definition at line 110 of file Palette.cpp.
◆ columnCount
|
slot |
Palettes are defined in grids.
The number of column define grid width. The number of rows will depend of columns and total number of entries.
- Returns
- the number of columns this palette is set to use.
Definition at line 46 of file Palette.cpp.
◆ comment
|
slot |
the comment or description associated with the palette.
- Returns
- A string for which value contains the comment/description of palette.
Definition at line 58 of file Palette.cpp.
◆ entryByIndex
|
slot |
get color (swatch) from the global index.
- Parameters
-
index the global index
- Returns
- The Swatch color for given index.
Definition at line 100 of file Palette.cpp.
◆ entryByIndexFromGroup
get color (swatch) from the given group index.
- Parameters
-
index index in the group. groupName the name of the group to get the color from.
- Returns
- The Swatch color for given index within given group name.
Definition at line 116 of file Palette.cpp.
◆ groupNames
|
slot |
Palette content can be organized in groups.
- Returns
- The list of group names (list of string). This list follow the order the groups are defined in palette.
Definition at line 70 of file Palette.cpp.
◆ moveGroup
|
slot |
Move the group groupName
to position before group groupNameInsertBefore
.
- Parameters
-
groupName group to move. groupNameInsertBefore reference group for which groupName
have to be moved before.
Definition at line 161 of file Palette.cpp.
◆ numberOfEntries
|
slot |
number of colors (swatches) in palette NOTE: same as colorsCountTotal()
- Returns
- total number of colors
Definition at line 40 of file Palette.cpp.
◆ operator!=()
bool Palette::operator!= | ( | const Palette & | other | ) | const |
Definition at line 34 of file Palette.cpp.
◆ operator==()
bool Palette::operator== | ( | const Palette & | other | ) | const |
Definition at line 29 of file Palette.cpp.
◆ removeEntry
|
slot |
remove from defined group a color entry designed by given index.
- Parameters
-
index index in the group. groupName the name of the group within which color have to be removed.
Definition at line 130 of file Palette.cpp.
◆ removeGroup
|
slot |
Palette content can be organized in groups.
This method allows to remove an existing group from palette.
- Parameters
-
name The name of the new group to remve. keepColors whether or not to delete all the colors inside, or to move them to the default group.
Definition at line 82 of file Palette.cpp.
◆ renameGroup
rename a group
- Parameters
-
oldGroupName the old groupname to change. newGroupName the new name to change it into.
Definition at line 156 of file Palette.cpp.
◆ save
|
slot |
save the palette
WARNING: this method does nothing and need to be implemented!
- Returns
- always False
Definition at line 166 of file Palette.cpp.
◆ setColumnCount
|
slot |
Palettes are defined in grids.
The number of column define grid width, this value can be defined. The number of rows will depend of columns and total number of entries.
- Parameters
-
columns Set the amount of columns this palette should use.
Definition at line 52 of file Palette.cpp.
◆ setComment
|
slot |
the comment or description associated with the palette.
- Parameters
-
comment set the comment or description associated with the palette.
Definition at line 64 of file Palette.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 21 2025 11:55:31 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.