CatalogsDB::AsyncDBManager
#include <catalogsdb.h>
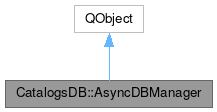
Public Types | |
template<typename... Args> | |
using | DBManagerMethod = CatalogObjectList (DBManager::*)(Args...) |
Signals | |
void | resultReady (void) |
void | threadReady (void) |
Public Slots | |
void | find_objects_by_name (const QString &name, const int limit=-1) |
void | find_objects_by_name_exact (const QString &name) |
void | init () |
std::unique_ptr< CatalogObjectList > | result () |
Public Member Functions | |
template<typename... Args> | |
AsyncDBManager (Args... args) | |
void | _find_objects_by_name (const QString &name, const int limit, const bool exactMatchOnly) |
template<typename... Args> | |
void | execute (DBManagerMethod< Args... > dbManagerMethod, Args... args) |
template<typename... Args> | |
void | init (Args &&... args) |
std::shared_ptr< DBManager > | manager () |
std::shared_ptr< QThread > | thread () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
A concurrent wrapper around.
- See also
- CatalogsDB::DBManager
This wrapper can be instantiated from the main thread. It spawns its own thread and moves itself to the thread, allowing the main thread to call DB operations without blocking the user interface. It provides a generic wrapper interface,
- See also
- AsyncDBManager::execute(), to call the methods of DBManager.
Since it is hard to use signal-slot communication with a generic wrapper like
- See also
- AsyncDBManager::execute(), a wrapper is provided for the two most likely overloads of
- DBManager::find_objects_by_name, which are time-consuming and frequently used, and these can be directly invoked from QMetaObject::invokeMethod or an appropriate signal
The void override of
- See also
- AsyncDBManager::init() does the most commonly done thing, which is to open the DSO database
Definition at line 745 of file catalogsdb.h.
Member Typedef Documentation
◆ DBManagerMethod
using CatalogsDB::AsyncDBManager::DBManagerMethod = CatalogObjectList (DBManager::*)(Args...) |
Definition at line 758 of file catalogsdb.h.
Constructor & Destructor Documentation
◆ AsyncDBManager()
|
inline |
Constructor, does nothing.
Call init() to do the actual setup after the QThread stars
Definition at line 764 of file catalogsdb.h.
◆ ~AsyncDBManager()
|
inline |
Definition at line 786 of file catalogsdb.h.
Member Function Documentation
◆ _find_objects_by_name()
|
inline |
execute(), but specialized to find_objects_by_name
@fixme Code duplication needed to support older compilers
Definition at line 840 of file catalogsdb.h.
◆ execute()
|
inline |
A generic wrapper to call any method on DBManager that returns a CatalogObjectList.
For practical use examples, see
- See also
- AsyncDBManager::find_objects_by_name below which uses this wrapper
Definition at line 820 of file catalogsdb.h.
◆ find_objects_by_name
|
inlineslot |
Calls the given DBManager method, storing the result for later retrieval.
dbManagerMethod
method to execute (must return a CatalogObjectList) args
arguments to supply to the method
Definition at line 870 of file catalogsdb.h.
◆ find_objects_by_name_exact
|
inlineslot |
Definition at line 884 of file catalogsdb.h.
◆ init [1/2]
|
inlineslot |
Definition at line 858 of file catalogsdb.h.
◆ init() [2/2]
|
inline |
Construct the DBManager object.
Should be done in the thread corresponding to this object
Definition at line 807 of file catalogsdb.h.
◆ manager()
|
inline |
Return a pointer to the DBManager, for non-DB functionalities.
Definition at line 795 of file catalogsdb.h.
◆ result
|
inlineslot |
Returns the result of the previous call, or a nullptr if none exists.
Definition at line 898 of file catalogsdb.h.
◆ thread()
|
inline |
Return a pointer to the QThread object.
Definition at line 800 of file catalogsdb.h.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:41 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.