Ekos::Scheduler
#include <scheduler.h>
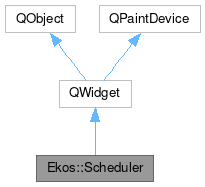
Public Types | |
enum | SchedulerColumns { SCHEDCOL_NAME = 0 , SCHEDCOL_STATUS , SCHEDCOL_CAPTURES , SCHEDCOL_ALTITUDE , SCHEDCOL_STARTTIME , SCHEDCOL_ENDTIME } |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
Signals | |
void | jobEnded (const QString &jobName, const QString &endReason) |
void | jobStarted (const QString &jobName) |
void | jobsUpdated (QJsonArray jobsList) |
void | newStatus (Ekos::SchedulerState state) |
void | newTarget (const QString &) |
void | settingsUpdated (const QVariantMap &settings) |
void | targetDistance (double distance) |
void | weatherChanged (ISD::Weather::Status state) |
Public Member Functions | |
Scheduler () | |
Scheduler (const QString path, const QString interface, const QString &ekosPathStr, const QString &ekosInterfaceStr) | |
SchedulerJob * | activeJob () |
Q_INVOKABLE void | addJob (SchedulerJob *job=nullptr) |
void | addObject (SkyObject *object) |
bool | fillJobFromUI (SchedulerJob *job) |
QVariantMap | getAllSettings () const |
QString | getCurrentJobName () |
ErrorHandlingStrategy | getErrorHandlingStrategy () |
QJsonObject | getSchedulerSettings () |
void | handleConfigChanged () |
bool | importMosaic (const QJsonObject &payload) |
bool | loadFile (const QUrl &path) |
QSharedPointer< SchedulerModuleState > | moduleState () const |
void | prepareGUI () |
QSharedPointer< SchedulerProcess > | process () |
QString | profile () |
void | removeJob () |
void | removeOneJob (int index) |
bool | saveFile (const QUrl &path) |
void | saveJob (SchedulerJob *job=nullptr) |
void | setAllSettings (const QVariantMap &settings) |
void | setErrorHandlingStrategy (ErrorHandlingStrategy strategy) |
void | setProfile (const QString &profile) |
void | setSequence (const QString &sequenceFileURL) |
void | settleSettings () |
Q_SCRIPTABLE void | sortJobsPerAltitude () |
void | toggleScheduler () |
Q_INVOKABLE void | updateJob (int index=-1) |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
virtual QSize | minimumSizeHint () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
virtual QSize | sizeHint () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
Public Attributes | |
OpsAlignmentSettings * | m_OpsAlignmentSettings { nullptr } |
OpsJobsSettings * | m_OpsJobsSettings { nullptr } |
OpsOffsetSettings * | m_OpsOffsetSettings { nullptr } |
OpsScriptsSettings * | m_OpsScriptsSettings { nullptr } |
![]() | |
DrawChildren | |
DrawWindowBackground | |
IgnoreMask | |
typedef | RenderFlags |
![]() | |
typedef | QObjectList |
![]() | |
PdmDepth | |
PdmDevicePixelRatio | |
PdmDevicePixelRatioScaled | |
PdmDpiX | |
PdmDpiY | |
PdmHeight | |
PdmHeightMM | |
PdmNumColors | |
PdmPhysicalDpiX | |
PdmPhysicalDpiY | |
PdmWidth | |
PdmWidthMM | |
Protected Slots | |
void | checkTwilightWarning (bool enabled) |
Q_INVOKABLE void | clearJobTable () |
Q_INVOKABLE void | clearLog () |
void | clickQueueTable (QModelIndex index) |
void | handleSchedulerSleeping (bool shutdown, bool sleep) |
void | handleSchedulerStateChanged (SchedulerState newState) |
void | handleSetPaused () |
void | interfaceReady (QDBusInterface *iface) |
Q_INVOKABLE bool | load (bool clearQueue, const QString &filename=QString()) |
Q_INVOKABLE void | loadJob (QModelIndex i) |
Q_INVOKABLE bool | modifyJob (int index=-1) |
void | moveJobDown () |
void | moveJobUp () |
void | pause () |
void | queueTableSelectionChanged (const QItemSelection &selected, const QItemSelection &deselected) |
bool | reorderJobs (QList< SchedulerJob * > reordered_sublist) |
Q_INVOKABLE void | resetJobEdit () |
void | resumeCheckStatus () |
bool | save () |
void | saveAs () |
void | schedulerStopped () |
void | selectFITS () |
void | selectObject () |
void | selectSequence () |
void | selectShutdownScript () |
void | selectStartupScript () |
void | setJobAddApply (bool add_mode) |
void | setJobManipulation (bool can_reorder, bool can_delete, bool is_lead) |
void | setWeatherStatus (ISD::Weather::Status status) |
void | syncGUIToGeneralSettings () |
void | syncGUIToJob (SchedulerJob *job) |
void | updateNightTime (SchedulerJob const *job=nullptr) |
void | updateSchedulerURL (const QString &fileURL) |
Protected Member Functions | |
void | insertJobTableRow (int row, bool above=true) |
void | setDirty () |
void | updateCellStyle (SchedulerJob *job, QTableWidgetItem *cell) |
void | updateJobTable (SchedulerJob *job=nullptr) |
void | watchJobChanges (bool enable) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | changeEvent (QEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEnterEvent *event) |
virtual bool | event (QEvent *event) override |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | paintEvent (QPaintEvent *event) |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The Ekos scheduler is a simple scheduler class to orchestrate automated multi object observation jobs.
- Version
- 1.2
Definition at line 54 of file scheduler.h.
Member Enumeration Documentation
◆ SchedulerColumns
Columns, in the same order as UI.
Definition at line 63 of file scheduler.h.
Constructor & Destructor Documentation
◆ Scheduler() [1/2]
Ekos::Scheduler::Scheduler | ( | ) |
Constructor, the starndard scheduler constructor.
Definition at line 86 of file scheduler.cpp.
◆ Scheduler() [2/2]
Ekos::Scheduler::Scheduler | ( | const QString | path, |
const QString | interface, | ||
const QString & | ekosPathStr, | ||
const QString & | ekosInterfaceStr ) |
DebugConstructor, a constructor used in testing with a mock ekos.
Definition at line 92 of file scheduler.cpp.
Member Function Documentation
◆ activeJob()
SchedulerJob * Ekos::Scheduler::activeJob | ( | ) |
Definition at line 2526 of file scheduler.cpp.
◆ addJob()
void Ekos::Scheduler::addJob | ( | SchedulerJob * | job = nullptr | ) |
addJob Add a new job from form values
Definition at line 817 of file scheduler.cpp.
◆ addObject()
void Ekos::Scheduler::addObject | ( | SkyObject * | object | ) |
Definition at line 631 of file scheduler.cpp.
◆ checkTwilightWarning
|
protectedslot |
checkWeather Check weather status and act accordingly depending on the current status of the scheduler and running jobs.
displayTwilightWarning Display twilight warning to user if it is unchecked.
Definition at line 2237 of file scheduler.cpp.
◆ clearJobTable
|
protectedslot |
clearJobTable delete all rows in the job table
Definition at line 2032 of file scheduler.cpp.
◆ clearLog
|
protectedslot |
clearLog Clears log entry
Definition at line 2041 of file scheduler.cpp.
◆ clickQueueTable
|
protectedslot |
jobSelectionChanged Update UI state when the job list is clicked once.
Definition at line 1325 of file scheduler.cpp.
◆ fillJobFromUI()
bool Ekos::Scheduler::fillJobFromUI | ( | SchedulerJob * | job | ) |
createJob Create a new job from form values.
- Parameters
-
job job to be filled from UI values
- Returns
- true iff update was successful
Definition at line 867 of file scheduler.cpp.
◆ getAllSettings()
QVariantMap Ekos::Scheduler::getAllSettings | ( | ) | const |
Definition at line 2726 of file scheduler.cpp.
◆ getCurrentJobName()
QString Ekos::Scheduler::getCurrentJobName | ( | ) |
Definition at line 430 of file scheduler.cpp.
◆ getErrorHandlingStrategy()
ErrorHandlingStrategy Ekos::Scheduler::getErrorHandlingStrategy | ( | ) |
retrieve the error handling strategy from the UI
Definition at line 2188 of file scheduler.cpp.
◆ handleConfigChanged()
void Ekos::Scheduler::handleConfigChanged | ( | ) |
handleConfigChanged Update UI after changes to the global configuration
Definition at line 616 of file scheduler.cpp.
◆ handleSchedulerSleeping
|
protectedslot |
handleSchedulerSleeping Update UI if scheduler is set to sleep
- Parameters
-
shutdown flag if a preemptive shutdown is executed sleep flag if the scheduler will sleep
Definition at line 2394 of file scheduler.cpp.
◆ handleSchedulerStateChanged
|
protectedslot |
handleSchedulerStateChanged Update UI when the scheduler state changes
Definition at line 2404 of file scheduler.cpp.
◆ handleSetPaused
|
protectedslot |
handleSetPaused Update the UI when {
- See also
- #setPaused()} is called.
Definition at line 2433 of file scheduler.cpp.
◆ importMosaic()
bool Ekos::Scheduler::importMosaic | ( | const QJsonObject & | payload | ) |
importMosaic Import mosaic into planner and generate jobs for the scheduler.
- Parameters
-
payload metadata for the mosaic information.
- Note
- Only Telescopius.com mosaic format is now supported.
Definition at line 2448 of file scheduler.cpp.
◆ insertJobTableRow()
|
protected |
insertJobTableRow Insert a new row (empty) into the job table
- Parameters
-
row row number (starting with 0) above insert above the given row (=true) or below (=false)
Definition at line 1758 of file scheduler.cpp.
◆ interfaceReady
|
protectedslot |
checkInterfaceReady Sometimes syncProperties() is not sufficient since the ready signal could have fired already and cannot be relied on to know once a module interface is ready.
Therefore, we explicitly check if the module interface is ready.
- Parameters
-
iface interface to test for readiness.
Definition at line 2290 of file scheduler.cpp.
◆ load
load Open a file dialog to select an ESL file, and load its contents.
- Parameters
-
clearQueue Clear the queue before loading, or append ESL contents to queue. filename If not empty, this file will be used instead of poping up a dialog.
Definition at line 1982 of file scheduler.cpp.
◆ loadFile()
bool Ekos::Scheduler::loadFile | ( | const QUrl & | path | ) |
loadFile Load scheduler jobs from disk
- Parameters
-
path Oath to esl file to load jobs from
- Returns
- True if successful, false otherwise
Definition at line 1977 of file scheduler.cpp.
◆ loadJob
|
protectedslot |
editJob Edit an observation job
- Parameters
-
i index model in queue table
Definition at line 1250 of file scheduler.cpp.
◆ modifyJob
|
protectedslot |
Definition at line 1236 of file scheduler.cpp.
◆ moduleState()
|
inline |
Definition at line 197 of file scheduler.h.
◆ moveJobDown
|
protectedslot |
moveJobDown Move the selected job down in the list.
Definition at line 1499 of file scheduler.cpp.
◆ moveJobUp
|
protectedslot |
moveJobUp Move the selected job up in the job list.
Definition at line 1426 of file scheduler.cpp.
◆ pause
|
protectedslot |
Definition at line 1895 of file scheduler.cpp.
◆ prepareGUI()
void Ekos::Scheduler::prepareGUI | ( | ) |
prepareGUI Perform once only GUI prep processing
Definition at line 435 of file scheduler.cpp.
◆ process()
|
inline |
Definition at line 202 of file scheduler.h.
◆ profile()
|
inline |
Definition at line 132 of file scheduler.h.
◆ queueTableSelectionChanged
|
protectedslot |
Update scheduler parameters to the currently selected scheduler job.
- Parameters
-
selected table position deselected table position
Definition at line 1290 of file scheduler.cpp.
◆ removeJob()
void Ekos::Scheduler::removeJob | ( | ) |
Remove a job from current table row.
- Parameters
-
index
Definition at line 1835 of file scheduler.cpp.
◆ removeOneJob()
void Ekos::Scheduler::removeOneJob | ( | int | index | ) |
Remove a job by selecting a table row.
- Parameters
-
index
Definition at line 1879 of file scheduler.cpp.
◆ reorderJobs
|
protectedslot |
reorderJobs Change the order of jobs in the UI based on a subset of its jobs.
Definition at line 1397 of file scheduler.cpp.
◆ resetJobEdit
|
protectedslot |
Definition at line 1807 of file scheduler.cpp.
◆ resumeCheckStatus
|
protectedslot |
resumeCheckStatus If the scheduler primary loop was suspended due to weather or sleep event, resume it again.
Definition at line 2181 of file scheduler.cpp.
◆ save
|
protectedslot |
Definition at line 2066 of file scheduler.cpp.
◆ saveAs
|
protectedslot |
Definition at line 2046 of file scheduler.cpp.
◆ saveFile()
bool Ekos::Scheduler::saveFile | ( | const QUrl & | path | ) |
saveFile Save scheduler jobs to disk
- Parameters
-
path Path to esl file to save jobs to
- Returns
- True if successful, false otherwise
Definition at line 2052 of file scheduler.cpp.
◆ saveJob()
void Ekos::Scheduler::saveJob | ( | SchedulerJob * | job = nullptr | ) |
addToQueue Construct a SchedulerJob and add it to the queue or save job settings from current form values.
jobUnderEdit determines whether to add or edit
Definition at line 964 of file scheduler.cpp.
◆ schedulerStopped
|
protectedslot |
schedulerStopped React when the process engine has stopped the scheduler
Definition at line 1931 of file scheduler.cpp.
◆ selectFITS
|
protectedslot |
Selects FITS file for solving.
Definition at line 652 of file scheduler.cpp.
◆ selectObject
|
protectedslot |
select object from KStars's find dialog.
Definition at line 622 of file scheduler.cpp.
◆ selectSequence
|
protectedslot |
Selects sequence queue.
Definition at line 778 of file scheduler.cpp.
◆ selectShutdownScript
|
protectedslot |
Selects sequence queue.
Definition at line 802 of file scheduler.cpp.
◆ selectStartupScript
|
protectedslot |
Selects sequence queue.
Definition at line 787 of file scheduler.cpp.
◆ setAllSettings()
void Ekos::Scheduler::setAllSettings | ( | const QVariantMap & | settings | ) |
Definition at line 2770 of file scheduler.cpp.
◆ setDirty()
|
protected |
Marks the currently selected SchedulerJob as modified change.
This triggers job re-evaluation. Next time save button is invoked, the complete content is written to disk.
Definition at line 2133 of file scheduler.cpp.
◆ setErrorHandlingStrategy()
void Ekos::Scheduler::setErrorHandlingStrategy | ( | ErrorHandlingStrategy | strategy | ) |
select the error handling strategy (no restart, restart after all terminated, restart immediately)
Definition at line 2199 of file scheduler.cpp.
◆ setJobAddApply
|
protectedslot |
setJobAddApply Set first button state to add new job or apply changes.
Definition at line 1333 of file scheduler.cpp.
◆ setJobManipulation
|
protectedslot |
setJobManipulation Enable or disable job manipulation buttons.
Definition at line 1350 of file scheduler.cpp.
◆ setProfile()
|
inline |
Definition at line 128 of file scheduler.h.
◆ setSequence()
void Ekos::Scheduler::setSequence | ( | const QString & | sequenceFileURL | ) |
Set the file URL pointing to the capture sequence file.
- Parameters
-
sequenceFileURL URL of the capture sequence file
Definition at line 765 of file scheduler.cpp.
◆ settleSettings()
void Ekos::Scheduler::settleSettings | ( | ) |
settleSettings Run this function after timeout from debounce timer to update database and emit settingsChanged signal.
This is required so we don't overload output.
Definition at line 2717 of file scheduler.cpp.
◆ setWeatherStatus
|
protectedslot |
Definition at line 2335 of file scheduler.cpp.
◆ sortJobsPerAltitude()
void Ekos::Scheduler::sortJobsPerAltitude | ( | ) |
◆ syncGUIToGeneralSettings
|
protectedslot |
syncGUIToGeneralSettings set all UI fields that are not job specific
Definition at line 1187 of file scheduler.cpp.
◆ syncGUIToJob
|
protectedslot |
set all GUI fields to the values of the given scheduler job
Definition at line 1075 of file scheduler.cpp.
◆ toggleScheduler()
void Ekos::Scheduler::toggleScheduler | ( | ) |
Definition at line 1884 of file scheduler.cpp.
◆ updateCellStyle()
|
protected |
Update the style of a cell, depending on the job's state.
Definition at line 1799 of file scheduler.cpp.
◆ updateJob()
void Ekos::Scheduler::updateJob | ( | int | index = -1 | ) |
addJob Add a new job from form values
Definition at line 851 of file scheduler.cpp.
◆ updateJobTable()
|
protected |
updateJobTable Update the job's row in the job table.
If the row does not exist, it will be created on the fly. If job is null, update the entire table
- Parameters
-
job
Definition at line 1569 of file scheduler.cpp.
◆ updateNightTime
|
protectedslot |
updateNightTime update the Twilight restriction with the argument job properties.
- Parameters
-
job SchedulerJob for which to display the next dawn and dusk, or the job currently selected if null, or today's next dawn and dusk if no job is selected.
Definition at line 1213 of file scheduler.cpp.
◆ updateSchedulerURL
|
protectedslot |
updateSchedulerURL Update scheduler URL after succesful loading a new file.
Definition at line 1283 of file scheduler.cpp.
◆ watchJobChanges()
|
protected |
Enables signal watch on SchedulerJob form values in order to apply changes to current job.
- Parameters
-
enable is the toggle flag, true to watch for changes, false to ignore them.
Definition at line 459 of file scheduler.cpp.
Member Data Documentation
◆ m_OpsAlignmentSettings
OpsAlignmentSettings* Ekos::Scheduler::m_OpsAlignmentSettings { nullptr } |
Definition at line 118 of file scheduler.h.
◆ m_OpsJobsSettings
OpsJobsSettings* Ekos::Scheduler::m_OpsJobsSettings { nullptr } |
Definition at line 119 of file scheduler.h.
◆ m_OpsOffsetSettings
OpsOffsetSettings* Ekos::Scheduler::m_OpsOffsetSettings { nullptr } |
Definition at line 117 of file scheduler.h.
◆ m_OpsScriptsSettings
OpsScriptsSettings* Ekos::Scheduler::m_OpsScriptsSettings { nullptr } |
Definition at line 120 of file scheduler.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:41 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.