SkyObject
#include <skyobject.h>
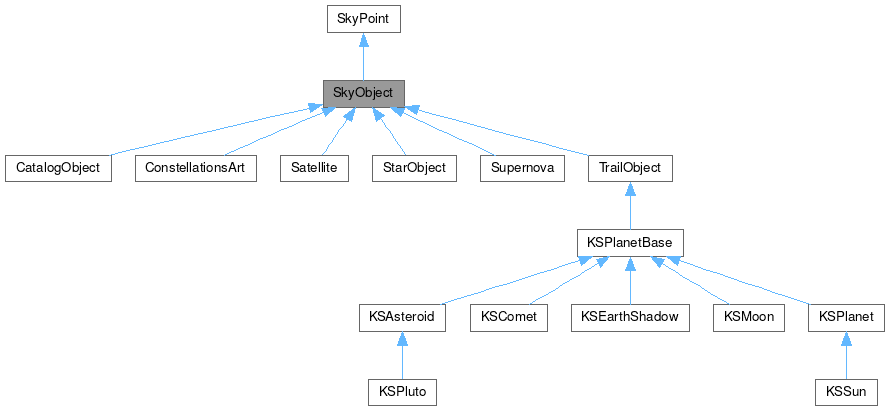
Public Types | |
enum | TYPE { STAR = 0 , CATALOG_STAR = 1 , PLANET = 2 , OPEN_CLUSTER = 3 , GLOBULAR_CLUSTER = 4 , GASEOUS_NEBULA = 5 , PLANETARY_NEBULA = 6 , SUPERNOVA_REMNANT = 7 , GALAXY = 8 , COMET = 9 , ASTEROID = 10 , CONSTELLATION = 11 , MOON = 12 , ASTERISM = 13 , GALAXY_CLUSTER = 14 , DARK_NEBULA = 15 , QUASAR = 16 , MULT_STAR = 17 , RADIO_SOURCE = 18 , SATELLITE = 19 , SUPERNOVA = 20 , NUMBER_OF_KNOWN_TYPES = 21 , TYPE_UNKNOWN = 255 } |
typedef qint64 | UID |
Public Member Functions | |
SkyObject (int t, double r, double d, float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
SkyObject (int t=TYPE_UNKNOWN, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
virtual | ~SkyObject () override=default |
virtual SkyObject * | clone () const |
virtual UID | getUID () const |
bool | hashBeenUpdated () |
bool | hasLongName () const |
bool | hasName () const |
bool | hasName2 () const |
virtual void | initPopupMenu (KSPopupMenu *pmenu) |
bool | isSolarSystem () const |
virtual double | labelOffset () const |
virtual QString | labelString () const |
virtual QString | longname (void) const |
float | mag () const |
QString | messageFromTitle (const QString &imageTitle) const |
virtual QString | name (void) const |
QString | name2 (void) const |
virtual double | pa () const |
SkyPoint | recomputeCoords (const KStarsDateTime &dt, const GeoLocation *geo=nullptr) const |
SkyPoint | recomputeHorizontalCoords (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | riseSetTime (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
dms | riseSetTimeAz (const KStarsDateTime &dt, const GeoLocation *geo, bool rst) const |
QTime | riseSetTimeUT (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
void | setLongName (const QString &longname=QString()) |
void | setName (const QString &name) |
void | setName2 (const QString &name2=QString()) |
void | setType (int t) |
void | showPopupMenu (KSPopupMenu *pmenu, const QPoint &pos) |
dms | transitAltitude (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTime (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTimeUT (const KStarsDateTime &dt, const GeoLocation *geo) const |
QString | translatedLongName () const |
QString | translatedName () const |
QString | translatedName2 () const |
int | type (void) const |
QString | typeName () const |
![]() | |
SkyPoint () | |
SkyPoint (const CachingDms &r, const CachingDms &d) | |
SkyPoint (const dms &r, const dms &d) | |
SkyPoint (double r, double d) | |
void | aberrate (const KSNumbers *num, bool reverse=false) |
void | addEterms (void) |
double | airmass () const |
const dms & | alt () const |
dms | altRefracted () const |
dms | angularDistanceTo (const SkyPoint *sp, double *const positionAngle=nullptr) const |
void | apparentCoord (long double jd0, long double jdf) |
const dms & | az () const |
void | B1950ToJ2000 (void) |
bool | bendlight () |
SkyPoint | catalogueCoord (long double jdf) |
bool | checkBendLight () |
bool | checkCircumpolar (const dms *gLat) const |
const CachingDms & | dec () const |
const CachingDms & | dec0 () const |
SkyPoint | deprecess (const KSNumbers *num, long double epoch=J2000) |
void | Equatorial1950ToGalactic (dms &galLong, dms &galLat) |
void | EquatorialToHorizontal (const CachingDms *LST, const CachingDms *lat) |
void | EquatorialToHorizontal (const dms *LST, const dms *lat) |
SkyPoint | Eterms (void) |
void | findEcliptic (const CachingDms *Obliquity, dms &EcLong, dms &EcLat) |
void | GalacticToEquatorial1950 (const dms *galLong, const dms *galLat) |
long double | getLastPrecessJD () const |
void | HorizontalToEquatorial (const dms *LST, const dms *lat) |
void | HorizontalToEquatorialICRS (const dms *LST, const dms *lat, const long double jdf) |
void | HorizontalToEquatorialNow () |
bool | isValid () const |
void | J2000ToB1950 (void) |
double | maxAlt (const dms &lat) const |
double | minAlt (const dms &lat) const |
SkyPoint | moveAway (const SkyPoint &from, double dist) const |
void | nutate (const KSNumbers *num, const bool reverse=false) |
bool | operator== (SkyPoint &p) const |
dms | parallacticAngle (const CachingDms &LST, const CachingDms &lat) |
void | precessFromAnyEpoch (long double jd0, long double jdf) |
const CachingDms & | ra () const |
const CachingDms & | ra0 () const |
void | set (const dms &r, const dms &d) |
void | setAlt (dms alt) |
void | setAlt (double alt) |
void | setAltRefracted (dms alt_apparent) |
void | setAltRefracted (double alt_apparent) |
void | setAz (dms az) |
void | setAz (double az) |
void | setDec (const CachingDms &d) |
void | setDec (dms d) |
void | setDec (double d) |
void | setDec0 (const CachingDms &d) |
void | setDec0 (dms d) |
void | setDec0 (double d) |
void | setFromEcliptic (const CachingDms *Obliquity, const dms &EcLong, const dms &EcLat) |
void | setRA (const CachingDms &r) |
void | setRA (dms &r) |
void | setRA (double r) |
void | setRA0 (CachingDms r) |
void | setRA0 (dms r) |
void | setRA0 (double r) |
void | subtractEterms (void) |
virtual void | updateCoords (const KSNumbers *num, bool includePlanets=true, const CachingDms *lat=nullptr, const CachingDms *LST=nullptr, bool forceRecompute=false) |
virtual void | updateCoordsNow (const KSNumbers *num) |
double | vGeocentric (double vhelio, long double jd) |
double | vGeoToVHelio (double vgeo, long double jd) |
double | vHeliocentric (double vlsr, long double jd) |
double | vHelioToVlsr (double vhelio, long double jd) |
double | vREarth (long double jd0) |
double | vRSite (double vsite[3]) |
double | vRSun (long double jd) |
double | vTopocentric (double vgeo, double vsite[3]) |
double | vTopoToVGeo (double vtopo, double vsite[3]) |
Static Public Member Functions | |
static QString | typeName (const int t) |
static QString | typeShortName (const int t) |
![]() | |
static dms | findAltitude (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | refract (const dms alt, bool conditional=true) |
static double | refract (const double alt, bool conditional=true) |
static double | refractionCorr (double alt) |
static SkyPoint | timeTransformed (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | unrefract (const dms alt, bool conditional=true) |
static double | unrefract (const double alt, bool conditional=true) |
Static Public Attributes | |
static const UID | invalidUID = ~0 |
static const UID | UID_DEEPSKY = 2 |
static const UID | UID_GALAXY = 1 |
static const UID | UID_SOLARSYS = 3 |
static const UID | UID_STAR = 0 |
![]() | |
static const double | altCrit = -1.0 |
static bool | implementationIsLibnova = false |
Protected Member Functions | |
void | setMag (float m) |
![]() | |
void | precess (const KSNumbers *num) |
Protected Attributes | |
bool | has_been_updated = true |
QString | LongName |
QString | Name |
QString | Name2 |
![]() | |
long double | lastPrecessJD { 0 } |
Detailed Description
Provides all necessary information about an object in the sky: its coordinates, name(s), type, magnitude, and QStringLists of URLs for images and webpages regarding the object.
Information about an object in the sky.
- Version
- 1.0
Definition at line 49 of file skyobject.h.
Member Typedef Documentation
◆ UID
typedef qint64 SkyObject::UID |
Type for Unique object IDenticator.
Each object has unique ID (UID). For different objects UIDs must be different.
Definition at line 57 of file skyobject.h.
Member Enumeration Documentation
◆ TYPE
enum SkyObject::TYPE |
The type classification of the SkyObject.
- Note
- Keep TYPE_UNKNOWN at 255. To find out how many known types exist, keep the NUMBER_OF_KNOWN_TYPES at the highest non-Unknown value. This is a fake type that can be used in comparisons and for loops.
Definition at line 119 of file skyobject.h.
Constructor & Destructor Documentation
◆ SkyObject() [1/2]
|
explicit |
Constructor.
Set SkyObject data according to arguments.
- Parameters
-
t Type of object r catalog Right Ascension d catalog Declination m magnitude (brightness) n Primary name n2 Secondary name lname Long name (common name)
Definition at line 30 of file skyobject.cpp.
◆ SkyObject() [2/2]
SkyObject::SkyObject | ( | int | t, |
double | r, | ||
double | d, | ||
float | m = 0.0, | ||
const QString & | n = QString(), | ||
const QString & | n2 = QString(), | ||
const QString & | lname = QString() ) |
Constructor.
Set SkyObject data according to arguments. Differs from above function only in data type of RA and Dec.
- Parameters
-
t Type of object r catalog Right Ascension d catalog Declination m magnitude (brightness) n Primary name n2 Secondary name lname Long name (common name)
Definition at line 40 of file skyobject.cpp.
◆ ~SkyObject()
|
overridevirtualdefault |
Destructor (empty)
Member Function Documentation
◆ clone()
|
virtual |
Create copy of object.
This method is virtual copy constructor. It allows for safe copying of objects. In other words, KSPlanet object stored in SkyObject pointer will be copied as KSPlanet.
Each subclass of SkyObject MUST implement clone method. There is no checking to ensure this, though.
- Returns
- pointer to newly allocated object. Caller takes full responsibility for deallocating it.
Reimplemented in CatalogObject, KSAsteroid, KSComet, KSMoon, KSPlanet, KSPluto, KSSun, Satellite, StarObject, Supernova, and TrailObject.
Definition at line 50 of file skyobject.cpp.
◆ getUID()
|
virtual |
Return UID for object.
This method should be reimplemented in all concrete subclasses. Implementation for SkyObject just returns invalidUID. It's required SkyObject is not an abstract class.
Reimplemented in CatalogObject, KSAsteroid, KSComet, KSMoon, KSPlanet, KSSun, and StarObject.
Definition at line 509 of file skyobject.cpp.
◆ hashBeenUpdated()
|
inline |
hashBeenUpdated
- Returns
- whether the coordinates of the object have been updated
This is used for faster filtering.
Definition at line 383 of file skyobject.h.
◆ hasLongName()
|
inline |
Definition at line 351 of file skyobject.h.
◆ hasName()
|
inline |
Definition at line 341 of file skyobject.h.
◆ hasName2()
|
inline |
Definition at line 346 of file skyobject.h.
◆ initPopupMenu()
|
virtual |
Initialize the popup menut.
This function should call correct initialization function in KSPopupMenu. By overloading the function, we don't have to check the object type when we need the menu.
Reimplemented in CatalogObject, KSMoon, Satellite, StarObject, Supernova, and TrailObject.
Definition at line 67 of file skyobject.cpp.
◆ isSolarSystem()
|
inline |
- Returns
- true if the object is a solar system body.
Definition at line 253 of file skyobject.h.
◆ labelOffset()
|
virtual |
- Returns
- the pixel distance for offseting the object's name label
- Note
- overridden in StarObject, DeepSkyObject, KSPlanetBase
Reimplemented in CatalogObject, KSPlanetBase, and StarObject.
Definition at line 504 of file skyobject.cpp.
◆ labelString()
|
virtual |
- Returns
- the string used to label the object on the map In the default implementation, this just returns translatedName() Overridden by StarObject.
Reimplemented in CatalogObject, and StarObject.
Definition at line 499 of file skyobject.cpp.
◆ longname()
|
inlinevirtual |
- Returns
- object's common (long) name
Reimplemented in StarObject.
Definition at line 182 of file skyobject.h.
◆ mag()
|
inline |
- Returns
- object's magnitude
Definition at line 236 of file skyobject.h.
◆ messageFromTitle()
Given the Image title from a URL file, try to convert it to an image credit string.
Definition at line 444 of file skyobject.cpp.
◆ name()
|
inlinevirtual |
- Returns
- object's primary name.
Reimplemented in StarObject.
Definition at line 154 of file skyobject.h.
◆ name2()
|
inline |
- Returns
- object's secondary name
Definition at line 168 of file skyobject.h.
◆ pa()
|
inlinevirtual |
- Returns
- the object's position angle. This is overridden in KSPlanetBase and DeepSkyObject; for all other SkyObjects, this returns 0.0.
Reimplemented in CatalogObject, ConstellationsArt, and KSPlanetBase.
Definition at line 245 of file skyobject.h.
◆ recomputeCoords()
SkyPoint SkyObject::recomputeCoords | ( | const KStarsDateTime & | dt, |
const GeoLocation * | geo = nullptr ) const |
The equatorial coordinates for the object on date dt are computed and returned, but the object's internal coordinates are not modified.
- Returns
- the coordinates of the selected object for the time given by jd
- Parameters
-
dt date/time for which the coords will be computed. geo pointer to geographic location (used for solar system only)
- Note
- Does not update the horizontal coordinates. Call EquatorialToHorizontal for that.
Definition at line 295 of file skyobject.cpp.
◆ recomputeHorizontalCoords()
SkyPoint SkyObject::recomputeHorizontalCoords | ( | const KStarsDateTime & | dt, |
const GeoLocation * | geo ) const |
Like recomputeCoords, but also calls EquatorialToHorizontal before returning.
Definition at line 329 of file skyobject.cpp.
◆ riseSetTime()
QTime SkyObject::riseSetTime | ( | const KStarsDateTime & | dt, |
const GeoLocation * | geo, | ||
bool | rst, | ||
bool | exact = true ) const |
Determine the time at which the point will rise or set.
Because solar system objects move across the sky, it is necessary to iterate on the solution. We compute the rise/set time for the object's current position, then compute the object's position at that time. Finally, we recompute then rise/set time for the new coordinates. Further iteration is not necessary, even for the most swiftly-moving object (the Moon).
- Returns
- the local time that the object will rise
- Parameters
-
dt current UT date/time geo current geographic location rst If true, compute rise time. If false, compute set time. exact If true, use a second iteration for more accurate time
Definition at line 93 of file skyobject.cpp.
◆ riseSetTimeAz()
dms SkyObject::riseSetTimeAz | ( | const KStarsDateTime & | dt, |
const GeoLocation * | geo, | ||
bool | rst ) const |
- Returns
- the Azimuth time when the object will rise or set. This function recomputes set or rise UT times.
- Parameters
-
dt target date/time geo GeoLocation object rst Boolen. If true will compute rise time. If false will compute set time.
Definition at line 190 of file skyobject.cpp.
◆ riseSetTimeUT()
QTime SkyObject::riseSetTimeUT | ( | const KStarsDateTime & | dt, |
const GeoLocation * | geo, | ||
bool | rst, | ||
bool | exact = true ) const |
- Returns
- the UT time when the object will rise or set
- Parameters
-
dt target date/time geo pointer to Geographic location rst Boolean. If true will compute rise time. If false will compute set time. exact If true, use a second iteration for more accurate time
Definition at line 127 of file skyobject.cpp.
◆ setLongName()
Set the object's long name.
- Parameters
-
longname the object's long name.
Definition at line 76 of file skyobject.cpp.
◆ setMag()
|
inlineprotected |
Set the object's sorting magnitude.
- Parameters
-
m the object's magnitude.
Definition at line 472 of file skyobject.h.
◆ setName()
|
inline |
Set the object's primary name.
- Parameters
-
name the object's primary name
Definition at line 392 of file skyobject.h.
◆ setName2()
Set the object's secondary name.
- Parameters
-
name2 the object's secondary name.
Definition at line 401 of file skyobject.h.
◆ setType()
|
inline |
Set the object's type identifier to the argument.
- Parameters
-
t the object's type identifier (e.g., "SkyObject::PLANETARY_NEBULA")
- See also
- enum TYPE
Definition at line 222 of file skyobject.h.
◆ showPopupMenu()
void SkyObject::showPopupMenu | ( | KSPopupMenu * | pmenu, |
const QPoint & | pos ) |
Show Type-specific popup menu.
Overloading is done in the function initPopupMenu
Definition at line 56 of file skyobject.cpp.
◆ transitAltitude()
dms SkyObject::transitAltitude | ( | const KStarsDateTime & | dt, |
const GeoLocation * | geo ) const |
- Returns
- the altitude of the object at the moment it transits the meridian.
- Parameters
-
dt target date/time geo pointer to the geographic location
Definition at line 244 of file skyobject.cpp.
◆ transitTime()
QTime SkyObject::transitTime | ( | const KStarsDateTime & | dt, |
const GeoLocation * | geo ) const |
The same iteration technique described in riseSetTime() is used here.
- Returns
- the local time that the object will transit the meridian.
- Parameters
-
dt target date/time geo pointer to the geographic location
Definition at line 239 of file skyobject.cpp.
◆ transitTimeUT()
QTime SkyObject::transitTimeUT | ( | const KStarsDateTime & | dt, |
const GeoLocation * | geo ) const |
- Returns
- the universal time that the object will transit the meridian.
- Parameters
-
dt target date/time geo pointer to the geographic location
Definition at line 221 of file skyobject.cpp.
◆ translatedLongName()
|
inline |
- Returns
- object's common (long) name, translated to local language.
Definition at line 190 of file skyobject.h.
◆ translatedName()
|
inline |
- Returns
- object's primary name, translated to local language.
Definition at line 160 of file skyobject.h.
◆ translatedName2()
|
inline |
- Returns
- object's secondary name, translated to local language.
Definition at line 174 of file skyobject.h.
◆ type()
|
inline |
◆ typeName() [1/2]
QString SkyObject::typeName | ( | ) | const |
- Returns
- the type name for this object
- Note
- This just calls the static method by the same name, with the appropriate type number. See SkyObject::typeName( const int )
Definition at line 439 of file skyobject.cpp.
◆ typeName() [2/2]
|
static |
- Returns
- A translated string indicating the type name for a given type number
- Parameters
-
t The type number
- Note
- Note the existence of a SkyObject::typeName( void ) method that is not static and returns the type of this object.
Definition at line 338 of file skyobject.cpp.
◆ typeShortName()
|
static |
Definition at line 389 of file skyobject.cpp.
Member Data Documentation
◆ has_been_updated
|
protected |
Definition at line 488 of file skyobject.h.
◆ invalidUID
|
static |
◆ LongName
|
protected |
Definition at line 482 of file skyobject.h.
◆ Name
|
protected |
Definition at line 482 of file skyobject.h.
◆ Name2
|
protected |
Definition at line 482 of file skyobject.h.
◆ UID_DEEPSKY
|
static |
Definition at line 62 of file skyobject.h.
◆ UID_GALAXY
|
static |
Definition at line 61 of file skyobject.h.
◆ UID_SOLARSYS
|
static |
Definition at line 63 of file skyobject.h.
◆ UID_STAR
|
static |
Kind of UID.
Definition at line 60 of file skyobject.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.