KSAsteroid
#include <ksasteroid.h>
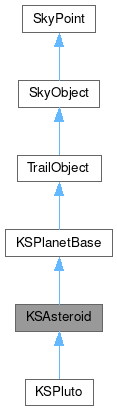
Public Member Functions | |
KSAsteroid (int catN, const QString &s, const QString &image_file, long double JD, double a, double e, dms i, dms w, dms N, dms M, double H, double G) | |
~KSAsteroid () override=default | |
KSAsteroid * | clone () const override |
double | getAbsoluteMagnitude () const |
float | getAlbedo () const |
float | getDiameter () const |
QString | getDimensions () const |
double | getEarthMOID () const |
QString | getOrbitClass () const |
QString | getOrbitID () const |
double | getPerihelion () const |
float | getPeriod () const |
float | getRotationPeriod () const |
double | getSlopeParameter () const |
SkyObject::UID | getUID () const override |
bool | isNEO () const |
bool | loadData () override |
void | setAlbedo (float albedo) |
void | setDiameter (float diam) |
void | setDimensions (QString dim) |
void | setEarthMOID (double earth_moid) |
void | setNEO (bool neo) |
void | setOrbitClass (QString orbit_class) |
void | setOrbitID (QString orbit_id) |
void | setPerihelion (double perihelion) |
void | setPeriod (float per) |
void | setRotationPeriod (float rot_per) |
bool | toCalculate () |
bool | toDraw () |
![]() | |
KSPlanetBase (const QString &s=i18n("unnamed"), const QString &image_file=QString(), const QColor &c=Qt::white, double pSize=0) | |
~KSPlanetBase () override=default | |
double | angSize () const |
QColor & | color () |
const dms & | ecLat () const |
void | EclipticToEquatorial (const CachingDms *Obliquity) |
const dms & | ecLong () const |
void | EquatorialToEcliptic (const CachingDms *Obliquity) |
void | findPosition (const KSNumbers *num, const CachingDms *lat=nullptr, const CachingDms *LST=nullptr, const KSPlanetBase *Earth=nullptr) |
const dms & | helEcLat () const |
const dms & | helEcLong () const |
const QImage & | image () const |
void | init (const QString &s, const QString &image_file, const QColor &c, double pSize) |
bool | isMajorPlanet () const |
double | labelOffset () const override |
double | pa () const override |
dms | phase () |
double | physicalSize () const |
double | rearth () const |
double | rsun () const |
void | setAngularSize (double size) |
void | setColor (const QColor &c) |
void | setEcLat (dms elat) |
void | setEcLong (dms elong) |
void | setPA (double p) |
void | setPhysicalSize (double size) |
void | setRearth (const KSPlanetBase *Earth) |
void | setRearth (double r) |
void | setRsun (double r) |
void | updateCoords (const KSNumbers *num, bool includePlanets=true, const CachingDms *lat=nullptr, const CachingDms *LST=nullptr, bool forceRecompute=false) override |
![]() | |
TrailObject (int t, double r, double d, float m=0.0, const QString &n=QString()) | |
TrailObject (int t=TYPE_UNKNOWN, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString()) | |
void | addToTrail (const QString &label=QString()) |
void | clearTrail () |
void | clipTrail () |
TrailObject * | clone () const override |
void | drawTrail (SkyPainter *skyp) const |
bool | hasTrail () const |
void | initPopupMenu (KSPopupMenu *pmenu) override |
const QList< SkyPoint > & | trail () const |
void | updateTrail (dms *LST, const dms *lat) |
![]() | |
SkyObject (int t, double r, double d, float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
SkyObject (int t=TYPE_UNKNOWN, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
virtual | ~SkyObject () override=default |
bool | hashBeenUpdated () |
bool | hasLongName () const |
bool | hasName () const |
bool | hasName2 () const |
bool | isSolarSystem () const |
virtual QString | labelString () const |
virtual QString | longname (void) const |
float | mag () const |
QString | messageFromTitle (const QString &imageTitle) const |
virtual QString | name (void) const |
QString | name2 (void) const |
SkyPoint | recomputeCoords (const KStarsDateTime &dt, const GeoLocation *geo=nullptr) const |
SkyPoint | recomputeHorizontalCoords (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | riseSetTime (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
dms | riseSetTimeAz (const KStarsDateTime &dt, const GeoLocation *geo, bool rst) const |
QTime | riseSetTimeUT (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
void | setLongName (const QString &longname=QString()) |
void | setName (const QString &name) |
void | setName2 (const QString &name2=QString()) |
void | setType (int t) |
void | showPopupMenu (KSPopupMenu *pmenu, const QPoint &pos) |
dms | transitAltitude (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTime (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTimeUT (const KStarsDateTime &dt, const GeoLocation *geo) const |
QString | translatedLongName () const |
QString | translatedName () const |
QString | translatedName2 () const |
int | type (void) const |
QString | typeName () const |
![]() | |
SkyPoint () | |
SkyPoint (const CachingDms &r, const CachingDms &d) | |
SkyPoint (const dms &r, const dms &d) | |
SkyPoint (double r, double d) | |
void | aberrate (const KSNumbers *num, bool reverse=false) |
void | addEterms (void) |
double | airmass () const |
const dms & | alt () const |
dms | altRefracted () const |
dms | angularDistanceTo (const SkyPoint *sp, double *const positionAngle=nullptr) const |
void | apparentCoord (long double jd0, long double jdf) |
const dms & | az () const |
void | B1950ToJ2000 (void) |
bool | bendlight () |
SkyPoint | catalogueCoord (long double jdf) |
bool | checkBendLight () |
bool | checkCircumpolar (const dms *gLat) const |
const CachingDms & | dec () const |
const CachingDms & | dec0 () const |
SkyPoint | deprecess (const KSNumbers *num, long double epoch=J2000) |
void | Equatorial1950ToGalactic (dms &galLong, dms &galLat) |
void | EquatorialToHorizontal (const CachingDms *LST, const CachingDms *lat) |
void | EquatorialToHorizontal (const dms *LST, const dms *lat) |
SkyPoint | Eterms (void) |
void | findEcliptic (const CachingDms *Obliquity, dms &EcLong, dms &EcLat) |
void | GalacticToEquatorial1950 (const dms *galLong, const dms *galLat) |
long double | getLastPrecessJD () const |
void | HorizontalToEquatorial (const dms *LST, const dms *lat) |
void | HorizontalToEquatorialICRS (const dms *LST, const dms *lat, const long double jdf) |
void | HorizontalToEquatorialNow () |
bool | isValid () const |
void | J2000ToB1950 (void) |
double | maxAlt (const dms &lat) const |
double | minAlt (const dms &lat) const |
SkyPoint | moveAway (const SkyPoint &from, double dist) const |
void | nutate (const KSNumbers *num, const bool reverse=false) |
bool | operator== (SkyPoint &p) const |
dms | parallacticAngle (const CachingDms &LST, const CachingDms &lat) |
void | precessFromAnyEpoch (long double jd0, long double jdf) |
const CachingDms & | ra () const |
const CachingDms & | ra0 () const |
void | set (const dms &r, const dms &d) |
void | setAlt (dms alt) |
void | setAlt (double alt) |
void | setAltRefracted (dms alt_apparent) |
void | setAltRefracted (double alt_apparent) |
void | setAz (dms az) |
void | setAz (double az) |
void | setDec (const CachingDms &d) |
void | setDec (dms d) |
void | setDec (double d) |
void | setDec0 (const CachingDms &d) |
void | setDec0 (dms d) |
void | setDec0 (double d) |
void | setFromEcliptic (const CachingDms *Obliquity, const dms &EcLong, const dms &EcLat) |
void | setRA (const CachingDms &r) |
void | setRA (dms &r) |
void | setRA (double r) |
void | setRA0 (CachingDms r) |
void | setRA0 (dms r) |
void | setRA0 (double r) |
void | subtractEterms (void) |
virtual void | updateCoordsNow (const KSNumbers *num) |
double | vGeocentric (double vhelio, long double jd) |
double | vGeoToVHelio (double vgeo, long double jd) |
double | vHeliocentric (double vlsr, long double jd) |
double | vHelioToVlsr (double vhelio, long double jd) |
double | vREarth (long double jd0) |
double | vRSite (double vsite[3]) |
double | vRSun (long double jd) |
double | vTopocentric (double vgeo, double vsite[3]) |
double | vTopoToVGeo (double vtopo, double vsite[3]) |
Static Public Attributes | |
static const SkyObject::TYPE | TYPE = SkyObject::ASTEROID |
![]() | |
static QVector< QColor > | planetColor |
![]() | |
static const int | MaxTrail = 400 |
![]() | |
static const UID | invalidUID = ~0 |
static const UID | UID_DEEPSKY = 2 |
static const UID | UID_GALAXY = 1 |
static const UID | UID_SOLARSYS = 3 |
static const UID | UID_STAR = 0 |
![]() | |
static const double | altCrit = -1.0 |
static bool | implementationIsLibnova = false |
Protected Member Functions | |
bool | findGeocentricPosition (const KSNumbers *num, const KSPlanetBase *Earth=nullptr) override |
void | set_a (double newa) |
void | set_e (double newe) |
void | set_i (double newi) |
void | set_M (double newM) |
void | set_N (double newN) |
void | set_P (double newP) |
void | set_w (double neww) |
void | setJD (long double jd) |
![]() | |
virtual double | findAngularSize () |
void | findPA (const KSNumbers *num) |
virtual void | findPhase () |
UID | solarsysUID (UID type) const |
![]() | |
void | setMag (float m) |
![]() | |
void | precess (const KSNumbers *num) |
Additional Inherited Members | |
![]() | |
enum | Planets { MERCURY = 0 , VENUS = 1 , MARS = 2 , JUPITER = 3 , SATURN = 4 , URANUS = 5 , NEPTUNE = 6 , SUN = 7 , MOON = 8 , EARTH_SHADOW = 9 , UNKNOWN_PLANET } |
![]() | |
enum | TYPE { STAR = 0 , CATALOG_STAR = 1 , PLANET = 2 , OPEN_CLUSTER = 3 , GLOBULAR_CLUSTER = 4 , GASEOUS_NEBULA = 5 , PLANETARY_NEBULA = 6 , SUPERNOVA_REMNANT = 7 , GALAXY = 8 , COMET = 9 , ASTEROID = 10 , CONSTELLATION = 11 , MOON = 12 , ASTERISM = 13 , GALAXY_CLUSTER = 14 , DARK_NEBULA = 15 , QUASAR = 16 , MULT_STAR = 17 , RADIO_SOURCE = 18 , SATELLITE = 19 , SUPERNOVA = 20 , NUMBER_OF_KNOWN_TYPES = 21 , TYPE_UNKNOWN = 255 } |
typedef qint64 | UID |
![]() | |
static KSPlanetBase * | createPlanet (int n) |
![]() | |
static void | clearTrailsExcept (SkyObject *o) |
![]() | |
static QString | typeName (const int t) |
static QString | typeShortName (const int t) |
![]() | |
static dms | findAltitude (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | refract (const dms alt, bool conditional=true) |
static double | refract (const double alt, bool conditional=true) |
static double | refractionCorr (double alt) |
static SkyPoint | timeTransformed (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | unrefract (const dms alt, bool conditional=true) |
static double | unrefract (const double alt, bool conditional=true) |
![]() | |
EclipticPosition | ep |
EclipticPosition | helEcPos |
QImage | m_image |
double | Phase {NaN::d} |
double | Rearth {NaN::d} |
![]() | |
QList< QString > | m_TrailLabels |
QList< SkyPoint > | Trail |
![]() | |
bool | has_been_updated = true |
QString | LongName |
QString | Name |
QString | Name2 |
![]() | |
long double | lastPrecessJD { 0 } |
![]() | |
static const UID | UID_SOL_ASTEROID = 1 |
static const UID | UID_SOL_BIGOBJ = 0 |
static const UID | UID_SOL_COMET = 2 |
![]() | |
static QSet< TrailObject * > | trailObjects |
Detailed Description
A subclass of KSPlanetBase that implements asteroids.
All elements are in the heliocentric ecliptic J2000 reference frame.
Check here for full description: https://ssd.jpl.nasa.gov/?sb_elem#legend
The orbital elements are stored as private member variables, and it provides methods to compute the ecliptic coordinates for any time from the orbital elements.
The orbital elements are:
- JD Epoch of element values
- a semi-major axis length (AU)
- e eccentricity of orbit
- i inclination angle (with respect to J2000.0 ecliptic plane)
- w argument of perihelion (w.r.t. J2000.0 ecliptic plane)
- N longitude of ascending node (J2000.0 ecliptic)
- M mean anomaly at epoch JD
- H absolute magnitude
- G slope parameter
- Version
- 1.0
Definition at line 41 of file ksasteroid.h.
Constructor & Destructor Documentation
◆ KSAsteroid()
KSAsteroid::KSAsteroid | ( | int | catN, |
const QString & | s, | ||
const QString & | image_file, | ||
long double | JD, | ||
double | a, | ||
double | e, | ||
dms | i, | ||
dms | w, | ||
dms | N, | ||
dms | M, | ||
double | H, | ||
double | G ) |
Constructor.
catN
number of asteroid s
the name of the asteroid image_file
the filename for an image of the asteroid JD
the Julian Day for the orbital elements a
the semi-major axis of the asteroid's orbit (AU) e
the eccentricity of the asteroid's orbit i
the inclination angle of the asteroid's orbit w
the argument of the orbit's perihelion N
the longitude of the orbit's ascending node M
the mean anomaly for the Julian Day H
absolute magnitude G
slope parameter
Definition at line 22 of file ksasteroid.cpp.
◆ ~KSAsteroid()
|
overridedefault |
Destructor (empty)
Member Function Documentation
◆ clone()
|
overridevirtual |
Create copy of object.
This method is virtual copy constructor. It allows for safe copying of objects. In other words, KSPlanet object stored in SkyObject pointer will be copied as KSPlanet.
Each subclass of SkyObject MUST implement clone method. There is no checking to ensure this, though.
- Returns
- pointer to newly allocated object. Caller takes full responsibility for deallocating it.
Reimplemented from SkyObject.
Reimplemented in KSPluto.
Definition at line 32 of file ksasteroid.cpp.
◆ findGeocentricPosition()
|
overrideprotectedvirtual |
Calculate the geocentric RA, Dec coordinates of the Asteroid.
- Note
- reimplemented from KSPlanetBase
- Parameters
-
num time-dependent values for the desired date Earth planet Earth (needed to calculate geocentric coords)
- Returns
- true if position was successfully calculated.
Implements KSPlanetBase.
Reimplemented in KSPluto.
Definition at line 39 of file ksasteroid.cpp.
◆ getAbsoluteMagnitude()
|
inline |
This lets other classes like KSPlanetBase access H and G values Used by KSPlanetBase::FindMagnitude.
Definition at line 77 of file ksasteroid.h.
◆ getAlbedo()
|
inline |
- Returns
- the asteroid's albedo
Definition at line 138 of file ksasteroid.h.
◆ getDiameter()
|
inline |
- Returns
- the asteroid's diameter
Definition at line 148 of file ksasteroid.h.
◆ getDimensions()
|
inline |
- Returns
- the asteroid's dimensions
Definition at line 158 of file ksasteroid.h.
◆ getEarthMOID()
|
inline |
- Returns
- the asteroid's earth minimum orbit intersection distance in AU
Definition at line 98 of file ksasteroid.h.
◆ getOrbitClass()
|
inline |
- Returns
- the asteroid's orbit class
Definition at line 118 of file ksasteroid.h.
◆ getOrbitID()
|
inline |
- Returns
- the asteroid's orbit solution ID
Definition at line 108 of file ksasteroid.h.
◆ getPerihelion()
|
inline |
- Returns
- Perihelion distance
Definition at line 88 of file ksasteroid.h.
◆ getPeriod()
|
inline |
- Returns
- the asteroid's period
Definition at line 178 of file ksasteroid.h.
◆ getRotationPeriod()
|
inline |
- Returns
- the asteroid's rotation period
Definition at line 168 of file ksasteroid.h.
◆ getSlopeParameter()
|
inline |
Definition at line 78 of file ksasteroid.h.
◆ getUID()
|
overridevirtual |
◆ isNEO()
|
inline |
- Returns
- true if the asteroid is a near earth object
Definition at line 128 of file ksasteroid.h.
◆ loadData()
|
overridevirtual |
This is inherited from KSPlanetBase.
We don't use it in this class, so it is empty.
Implements KSPlanetBase.
Definition at line 269 of file ksasteroid.cpp.
◆ set_a()
|
inlineprotected |
Definition at line 206 of file ksasteroid.h.
◆ set_e()
|
inlineprotected |
Definition at line 207 of file ksasteroid.h.
◆ set_i()
|
inlineprotected |
Definition at line 209 of file ksasteroid.h.
◆ set_M()
|
inlineprotected |
Definition at line 211 of file ksasteroid.h.
◆ set_N()
|
inlineprotected |
Definition at line 212 of file ksasteroid.h.
◆ set_P()
|
inlineprotected |
Definition at line 208 of file ksasteroid.h.
◆ set_w()
|
inlineprotected |
Definition at line 210 of file ksasteroid.h.
◆ setAlbedo()
void KSAsteroid::setAlbedo | ( | float | albedo | ) |
Sets the asteroid's albedo.
Definition at line 170 of file ksasteroid.cpp.
◆ setDiameter()
void KSAsteroid::setDiameter | ( | float | diam | ) |
Sets the asteroid's diameter.
Definition at line 175 of file ksasteroid.cpp.
◆ setDimensions()
void KSAsteroid::setDimensions | ( | QString | dim | ) |
Sets the asteroid's dimensions.
Definition at line 180 of file ksasteroid.cpp.
◆ setEarthMOID()
void KSAsteroid::setEarthMOID | ( | double | earth_moid | ) |
Sets the asteroid's earth minimum orbit intersection distance.
Definition at line 165 of file ksasteroid.cpp.
◆ setJD()
|
inlineprotected |
Definition at line 213 of file ksasteroid.h.
◆ setNEO()
void KSAsteroid::setNEO | ( | bool | neo | ) |
Sets if the comet is a near earth object.
Definition at line 185 of file ksasteroid.cpp.
◆ setOrbitClass()
void KSAsteroid::setOrbitClass | ( | QString | orbit_class | ) |
Sets the asteroid's orbit class.
Definition at line 190 of file ksasteroid.cpp.
◆ setOrbitID()
void KSAsteroid::setOrbitID | ( | QString | orbit_id | ) |
Sets the asteroid's orbit solution ID.
Definition at line 195 of file ksasteroid.cpp.
◆ setPerihelion()
void KSAsteroid::setPerihelion | ( | double | perihelion | ) |
Sets the asteroid's perihelion distance.
Definition at line 160 of file ksasteroid.cpp.
◆ setPeriod()
void KSAsteroid::setPeriod | ( | float | per | ) |
Sets the asteroid's period.
Definition at line 200 of file ksasteroid.cpp.
◆ setRotationPeriod()
void KSAsteroid::setRotationPeriod | ( | float | rot_per | ) |
Sets the asteroid's rotation period.
Definition at line 263 of file ksasteroid.cpp.
◆ toCalculate()
bool KSAsteroid::toCalculate | ( | ) |
◆ toDraw()
|
inline |
toDraw
- Returns
- whether to draw the asteroid
Note that you'd check for other, older filtering methids upn implementing this on other types! (a.k.a find nearest)
Definition at line 188 of file ksasteroid.h.
Member Data Documentation
◆ TYPE
|
static |
Definition at line 64 of file ksasteroid.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.