KSPlanetBase
#include <ksplanetbase.h>
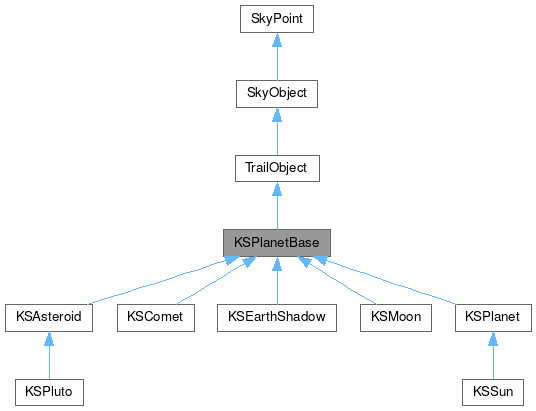
Public Types | |
enum | Planets { MERCURY = 0 , VENUS = 1 , MARS = 2 , JUPITER = 3 , SATURN = 4 , URANUS = 5 , NEPTUNE = 6 , SUN = 7 , MOON = 8 , EARTH_SHADOW = 9 , UNKNOWN_PLANET } |
![]() | |
enum | TYPE { STAR = 0 , CATALOG_STAR = 1 , PLANET = 2 , OPEN_CLUSTER = 3 , GLOBULAR_CLUSTER = 4 , GASEOUS_NEBULA = 5 , PLANETARY_NEBULA = 6 , SUPERNOVA_REMNANT = 7 , GALAXY = 8 , COMET = 9 , ASTEROID = 10 , CONSTELLATION = 11 , MOON = 12 , ASTERISM = 13 , GALAXY_CLUSTER = 14 , DARK_NEBULA = 15 , QUASAR = 16 , MULT_STAR = 17 , RADIO_SOURCE = 18 , SATELLITE = 19 , SUPERNOVA = 20 , NUMBER_OF_KNOWN_TYPES = 21 , TYPE_UNKNOWN = 255 } |
typedef qint64 | UID |
Public Member Functions | |
KSPlanetBase (const QString &s=i18n("unnamed"), const QString &image_file=QString(), const QColor &c=Qt::white, double pSize=0) | |
~KSPlanetBase () override=default | |
double | angSize () const |
QColor & | color () |
const dms & | ecLat () const |
void | EclipticToEquatorial (const CachingDms *Obliquity) |
const dms & | ecLong () const |
void | EquatorialToEcliptic (const CachingDms *Obliquity) |
void | findPosition (const KSNumbers *num, const CachingDms *lat=nullptr, const CachingDms *LST=nullptr, const KSPlanetBase *Earth=nullptr) |
const dms & | helEcLat () const |
const dms & | helEcLong () const |
const QImage & | image () const |
void | init (const QString &s, const QString &image_file, const QColor &c, double pSize) |
bool | isMajorPlanet () const |
double | labelOffset () const override |
virtual bool | loadData ()=0 |
double | pa () const override |
dms | phase () |
double | physicalSize () const |
double | rearth () const |
double | rsun () const |
void | setAngularSize (double size) |
void | setColor (const QColor &c) |
void | setEcLat (dms elat) |
void | setEcLong (dms elong) |
void | setPA (double p) |
void | setPhysicalSize (double size) |
void | setRearth (const KSPlanetBase *Earth) |
void | setRearth (double r) |
void | setRsun (double r) |
void | updateCoords (const KSNumbers *num, bool includePlanets=true, const CachingDms *lat=nullptr, const CachingDms *LST=nullptr, bool forceRecompute=false) override |
![]() | |
TrailObject (int t, double r, double d, float m=0.0, const QString &n=QString()) | |
TrailObject (int t=TYPE_UNKNOWN, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString()) | |
void | addToTrail (const QString &label=QString()) |
void | clearTrail () |
void | clipTrail () |
TrailObject * | clone () const override |
void | drawTrail (SkyPainter *skyp) const |
bool | hasTrail () const |
void | initPopupMenu (KSPopupMenu *pmenu) override |
const QList< SkyPoint > & | trail () const |
void | updateTrail (dms *LST, const dms *lat) |
![]() | |
SkyObject (int t, double r, double d, float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
SkyObject (int t=TYPE_UNKNOWN, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
virtual | ~SkyObject () override=default |
virtual UID | getUID () const |
bool | hashBeenUpdated () |
bool | hasLongName () const |
bool | hasName () const |
bool | hasName2 () const |
bool | isSolarSystem () const |
virtual QString | labelString () const |
virtual QString | longname (void) const |
float | mag () const |
QString | messageFromTitle (const QString &imageTitle) const |
virtual QString | name (void) const |
QString | name2 (void) const |
SkyPoint | recomputeCoords (const KStarsDateTime &dt, const GeoLocation *geo=nullptr) const |
SkyPoint | recomputeHorizontalCoords (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | riseSetTime (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
dms | riseSetTimeAz (const KStarsDateTime &dt, const GeoLocation *geo, bool rst) const |
QTime | riseSetTimeUT (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
void | setLongName (const QString &longname=QString()) |
void | setType (int t) |
void | showPopupMenu (KSPopupMenu *pmenu, const QPoint &pos) |
dms | transitAltitude (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTime (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTimeUT (const KStarsDateTime &dt, const GeoLocation *geo) const |
QString | translatedLongName () const |
QString | translatedName () const |
QString | translatedName2 () const |
int | type (void) const |
QString | typeName () const |
![]() | |
SkyPoint () | |
SkyPoint (const CachingDms &r, const CachingDms &d) | |
SkyPoint (const dms &r, const dms &d) | |
SkyPoint (double r, double d) | |
void | aberrate (const KSNumbers *num, bool reverse=false) |
void | addEterms (void) |
double | airmass () const |
const dms & | alt () const |
dms | altRefracted () const |
dms | angularDistanceTo (const SkyPoint *sp, double *const positionAngle=nullptr) const |
void | apparentCoord (long double jd0, long double jdf) |
const dms & | az () const |
void | B1950ToJ2000 (void) |
bool | bendlight () |
SkyPoint | catalogueCoord (long double jdf) |
bool | checkBendLight () |
bool | checkCircumpolar (const dms *gLat) const |
const CachingDms & | dec () const |
const CachingDms & | dec0 () const |
SkyPoint | deprecess (const KSNumbers *num, long double epoch=J2000) |
void | Equatorial1950ToGalactic (dms &galLong, dms &galLat) |
void | EquatorialToHorizontal (const CachingDms *LST, const CachingDms *lat) |
void | EquatorialToHorizontal (const dms *LST, const dms *lat) |
SkyPoint | Eterms (void) |
void | findEcliptic (const CachingDms *Obliquity, dms &EcLong, dms &EcLat) |
void | GalacticToEquatorial1950 (const dms *galLong, const dms *galLat) |
double | getLastPrecessJD () const |
void | HorizontalToEquatorial (const dms *LST, const dms *lat) |
void | J2000ToB1950 (void) |
double | maxAlt (const dms &lat) const |
double | minAlt (const dms &lat) const |
SkyPoint | moveAway (const SkyPoint &from, double dist) const |
void | nutate (const KSNumbers *num, const bool reverse=false) |
bool | operator== (SkyPoint &p) const |
dms | parallacticAngle (const CachingDms &LST, const CachingDms &lat) |
void | precessFromAnyEpoch (long double jd0, long double jdf) |
const CachingDms & | ra () const |
const CachingDms & | ra0 () const |
void | set (const dms &r, const dms &d) |
void | setAlt (dms alt) |
void | setAlt (double alt) |
void | setAltRefracted (dms alt_apparent) |
void | setAltRefracted (double alt_apparent) |
void | setAz (dms az) |
void | setAz (double az) |
void | setDec (const CachingDms &d) |
void | setDec (dms d) |
void | setDec (double d) |
void | setDec0 (const CachingDms &d) |
void | setDec0 (dms d) |
void | setDec0 (double d) |
void | setFromEcliptic (const CachingDms *Obliquity, const dms &EcLong, const dms &EcLat) |
void | setRA (const CachingDms &r) |
void | setRA (dms &r) |
void | setRA (double r) |
void | setRA0 (CachingDms r) |
void | setRA0 (dms r) |
void | setRA0 (double r) |
void | subtractEterms (void) |
virtual void | updateCoordsNow (const KSNumbers *num) |
double | vGeocentric (double vhelio, long double jd) |
double | vGeoToVHelio (double vgeo, long double jd) |
double | vHeliocentric (double vlsr, long double jd) |
double | vHelioToVlsr (double vhelio, long double jd) |
double | vREarth (long double jd0) |
double | vRSite (double vsite[3]) |
double | vRSun (long double jd) |
double | vTopocentric (double vgeo, double vsite[3]) |
double | vTopoToVGeo (double vtopo, double vsite[3]) |
Static Public Member Functions | |
static KSPlanetBase * | createPlanet (int n) |
![]() | |
static void | clearTrailsExcept (SkyObject *o) |
![]() | |
static QString | typeName (const int t) |
![]() | |
static dms | findAltitude (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | refract (const dms alt, bool conditional=true) |
static double | refract (const double alt, bool conditional=true) |
static double | refractionCorr (double alt) |
static SkyPoint | timeTransformed (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | unrefract (const dms alt, bool conditional=true) |
static double | unrefract (const double alt, bool conditional=true) |
Static Public Attributes | |
static QVector< QColor > | planetColor |
![]() | |
static const int | MaxTrail = 400 |
![]() | |
static const UID | invalidUID = ~0 |
static const UID | UID_DEEPSKY = 2 |
static const UID | UID_GALAXY = 1 |
static const UID | UID_SOLARSYS = 3 |
static const UID | UID_STAR = 0 |
![]() | |
static const double | altCrit = -1.0 |
static bool | implementationIsLibnova = false |
Protected Member Functions | |
virtual double | findAngularSize () |
virtual bool | findGeocentricPosition (const KSNumbers *num, const KSPlanetBase *Earth=nullptr)=0 |
virtual void | findMagnitude (const KSNumbers *num)=0 |
void | findPA (const KSNumbers *num) |
virtual void | findPhase () |
UID | solarsysUID (UID type) const |
![]() | |
void | setMag (float m) |
void | setName (const QString &name) |
void | setName2 (const QString &name2=QString()) |
![]() | |
void | precess (const KSNumbers *num) |
Protected Attributes | |
EclipticPosition | ep |
EclipticPosition | helEcPos |
QImage | m_image |
double | Phase {NaN::d} |
double | Rearth {NaN::d} |
![]() | |
QList< QString > | m_TrailLabels |
QList< SkyPoint > | Trail |
![]() | |
bool | has_been_updated = true |
QString | LongName |
QString | Name |
QString | Name2 |
![]() | |
double | lastPrecessJD { 0 } |
Static Protected Attributes | |
static const UID | UID_SOL_ASTEROID = 1 |
static const UID | UID_SOL_BIGOBJ = 0 |
static const UID | UID_SOL_COMET = 2 |
![]() | |
static QSet< TrailObject * > | trailObjects |
Detailed Description
A subclass of TrailObject that provides additional information needed for most solar system objects.
This is a base class for KSSun, KSMoon, KSPlanet, KSAsteroid and KSComet. Those classes cover all solar system objects except planetary moons, which are derived directly from TrailObject
Provides necessary information about objects in the solar system.
- Version
- 1.0
Definition at line 49 of file ksplanetbase.h.
Member Enumeration Documentation
◆ Planets
enum KSPlanetBase::Planets |
Definition at line 69 of file ksplanetbase.h.
Constructor & Destructor Documentation
◆ KSPlanetBase()
|
explicit |
Constructor.
Calls SkyObject constructor with type=2 (planet), coordinates=0.0, mag=0.0, primary name s, and all other QStrings empty.
- Parameters
-
s Name of planet image_file filename of the planet's image c color of the symbol to use for this planet pSize the planet's physical size, in km
Definition at line 37 of file ksplanetbase.cpp.
◆ ~KSPlanetBase()
|
overridedefault |
Destructor (empty)
Member Function Documentation
◆ angSize()
|
inline |
- Returns
- the Planet's angular size, in arcminutes
Definition at line 184 of file ksplanetbase.h.
◆ color()
|
inline |
- Returns
- the color for the planet symbol
Definition at line 203 of file ksplanetbase.h.
◆ createPlanet()
|
static |
Definition at line 53 of file ksplanetbase.cpp.
◆ ecLat()
|
inline |
- Returns
- pointer to Ecliptic Latitude coordinate
Definition at line 94 of file ksplanetbase.h.
◆ EclipticToEquatorial()
void KSPlanetBase::EclipticToEquatorial | ( | const CachingDms * | Obliquity | ) |
Convert Ecliptic longitude/latitude to Right Ascension/Declination.
Definition at line 81 of file ksplanetbase.cpp.
◆ ecLong()
|
inline |
- Returns
- pointer to Ecliptic Longitude coordinate
Definition at line 91 of file ksplanetbase.h.
◆ EquatorialToEcliptic()
void KSPlanetBase::EquatorialToEcliptic | ( | const CachingDms * | Obliquity | ) |
Convert Right Ascension/Declination to Ecliptic longitude/latitude.
Definition at line 76 of file ksplanetbase.cpp.
◆ findAngularSize()
|
inlineprotectedvirtual |
Reimplemented in KSEarthShadow.
Definition at line 252 of file ksplanetbase.h.
◆ findGeocentricPosition()
|
protectedpure virtual |
find the object's current geocentric equatorial coordinates (RA and Dec) This function is pure virtual; it must be overloaded by subclasses.
This function is private; it is called by the public function findPosition() which also includes the figure-of-the-earth correction, localizeCoords().
- Parameters
-
num pointer to current KSNumbers object Earth pointer to planet Earth (needed to calculate geocentric coords)
- Returns
- true if position was successfully calculated.
Implemented in KSAsteroid, KSComet, KSEarthShadow, KSMoon, KSPlanet, KSPluto, and KSSun.
◆ findMagnitude()
|
protectedpure virtual |
Computes the visual magnitude for the major planets.
- Parameters
-
num pointer to a ksnumbers object. Needed for the saturn rings contribution to saturn's magnitude.
Implemented in KSEarthShadow.
◆ findPA()
|
protected |
Determine the position angle of the planet for a given date (used internally by findPosition() )
Definition at line 240 of file ksplanetbase.cpp.
◆ findPhase()
|
protectedvirtual |
Determine the phase of the planet.
Reimplemented in KSEarthShadow, and KSMoon.
Definition at line 282 of file ksplanetbase.cpp.
◆ findPosition()
void KSPlanetBase::findPosition | ( | const KSNumbers * | num, |
const CachingDms * | lat = nullptr, | ||
const CachingDms * | LST = nullptr, | ||
const KSPlanetBase * | Earth = nullptr ) |
Find position, including correction for Figure-of-the-Earth.
- Parameters
-
num KSNumbers pointer for the target date/time lat pointer to the geographic latitude; if nullptr, we skip localizeCoords() LST pointer to the local sidereal time; if nullptr, we skip localizeCoords() Earth pointer to the Earth (not used for the Moon)
Definition at line 109 of file ksplanetbase.cpp.
◆ helEcLat()
|
inline |
- Returns
- pointer to Ecliptic Heliocentric Latitude coordinate
Definition at line 112 of file ksplanetbase.h.
◆ helEcLong()
|
inline |
- Returns
- pointer to Ecliptic Heliocentric Longitude coordinate
Definition at line 109 of file ksplanetbase.h.
◆ image()
|
inline |
- Returns
- pointer to this planet's texture
Definition at line 127 of file ksplanetbase.h.
◆ init()
void KSPlanetBase::init | ( | const QString & | s, |
const QString & | image_file, | ||
const QColor & | c, | ||
double | pSize ) |
Definition at line 43 of file ksplanetbase.cpp.
◆ isMajorPlanet()
bool KSPlanetBase::isMajorPlanet | ( | ) | const |
- Returns
- true if the KSPlanet is one of the eight major planets
Definition at line 144 of file ksplanetbase.cpp.
◆ labelOffset()
|
overridevirtual |
- Returns
- the pixel distance for offseting the object's name label
Reimplemented from SkyObject.
Definition at line 262 of file ksplanetbase.cpp.
◆ loadData()
|
pure virtual |
Implemented in KSAsteroid, KSComet, KSMoon, KSPlanet, and KSSun.
◆ pa()
|
inlineoverridevirtual |
- Returns
- the Planet's position angle.
Reimplemented from SkyObject.
Definition at line 175 of file ksplanetbase.h.
◆ phase()
|
inline |
- Returns
- the phase angle of this planet
Definition at line 200 of file ksplanetbase.h.
◆ physicalSize()
|
inline |
- Returns
- the Planet's physical size, in km
Definition at line 192 of file ksplanetbase.h.
◆ rearth()
|
inline |
- Returns
- distance from Earth, in Astronomical Units (1 AU is Earth-Sun distance)
Definition at line 139 of file ksplanetbase.h.
◆ rsun()
|
inline |
- Returns
- distance from Sun, in Astronomical Units (1 AU is Earth-Sun distance)
Definition at line 130 of file ksplanetbase.h.
◆ setAngularSize()
|
inline |
set the planet's angular size, in km.
- Parameters
-
size the planet's size, in km
Definition at line 189 of file ksplanetbase.h.
◆ setColor()
|
inline |
Set the color for the planet symbol.
Definition at line 206 of file ksplanetbase.h.
◆ setEcLat()
|
inline |
Set Ecliptic Geocentric Latitude according to argument.
- Parameters
-
elat Ecliptic Latitude
Definition at line 106 of file ksplanetbase.h.
◆ setEcLong()
|
inline |
Set Ecliptic Geocentric Longitude according to argument.
- Parameters
-
elong Ecliptic Longitude
Definition at line 100 of file ksplanetbase.h.
◆ setPA()
|
inline |
Set the Planet's position angle.
- Parameters
-
p the new position angle
Definition at line 181 of file ksplanetbase.h.
◆ setPhysicalSize()
|
inline |
set the planet's physical size, in km.
- Parameters
-
size the planet's size, in km
Definition at line 197 of file ksplanetbase.h.
◆ setRearth() [1/2]
void KSPlanetBase::setRearth | ( | const KSPlanetBase * | Earth | ) |
compute and set the distance from Earth, in AU.
- Parameters
-
Earth pointer to the Earth from which to calculate the distance.
Definition at line 197 of file ksplanetbase.cpp.
◆ setRearth() [2/2]
|
inline |
Set the distance from Earth, in AU.
- Parameters
-
r the new earth-distance in AU
Definition at line 145 of file ksplanetbase.h.
◆ setRsun()
|
inline |
Set the solar distance in AU.
- Parameters
-
r the new solar distance in AU
Definition at line 136 of file ksplanetbase.h.
◆ solarsysUID()
Compute high 32-bits of UID.
Definition at line 223 of file ksplanetbase.h.
◆ updateCoords()
|
overridevirtual |
Update position of the planet (reimplemented from SkyPoint)
- Parameters
-
num current KSNumbers object includePlanets this function does nothing if includePlanets=false lat pointer to the geographic latitude; if nullptr, we skip localizeCoords() LST pointer to the local sidereal time; if nullptr, we skip localizeCoords() forceRecompute defines whether the data should be recomputed forcefully
Reimplemented from SkyPoint.
Definition at line 86 of file ksplanetbase.cpp.
Member Data Documentation
◆ ep
|
protected |
Definition at line 254 of file ksplanetbase.h.
◆ helEcPos
|
protected |
Definition at line 258 of file ksplanetbase.h.
◆ m_image
|
protected |
Definition at line 261 of file ksplanetbase.h.
◆ Phase
|
protected |
Definition at line 260 of file ksplanetbase.h.
◆ planetColor
◆ Rearth
|
protected |
Definition at line 259 of file ksplanetbase.h.
◆ UID_SOL_ASTEROID
|
staticprotected |
Asteroids.
Definition at line 218 of file ksplanetbase.h.
◆ UID_SOL_BIGOBJ
|
staticprotected |
◆ UID_SOL_COMET
|
staticprotected |
Comets.
Definition at line 220 of file ksplanetbase.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:59:53 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.