dms
#include <dms.h>
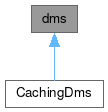
Public Types | |
enum | AngleRanges { ZERO_TO_2PI , MINUSPI_TO_PI } |
Public Member Functions | |
dms () | |
dms (const double &x) | |
dms (const int &d, const int &m=0, const int &s=0, const int &ms=0) | |
dms (const QString &s, bool isDeg=true) | |
virtual | ~dms ()=default |
int | arcmin () const |
int | arcsec () const |
double | cos () const |
int | degree () const |
const double & | Degrees () const |
const dms | deltaAngle (dms angle) const |
int | hour () const |
double | Hours () const |
double | HoursHa () const |
int | marcsec () const |
int | minute () const |
int | msecond () const |
dms | operator- () |
double | radians () const |
const dms | reduce () const |
void | reduceToRange (enum dms::AngleRanges range) |
int | second () const |
virtual void | setD (const double &x) |
virtual void | setD (const int &d, const int &m, const int &s, const int &ms=0) |
virtual bool | setFromString (const QString &s, bool isDeg=true) |
virtual void | setH (const double &x) |
virtual void | setH (const int &h, const int &m, const int &s, const int &ms=0) |
virtual void | setRadians (const double &Rad) |
double | sin () const |
void | SinCos (double &s, double &c) const |
double | tan () const |
const QString | toDMSString (const bool forceSign=false, const bool machineReadable=false, const bool highPrecision=false) const |
const QString | toHMSString (const bool machineReadable=false, const bool highPrecision=false) const |
Static Public Member Functions | |
static dms | fromString (const QString &s, bool deg) |
static double | reduce (const double D) |
Static Public Attributes | |
static constexpr double | DegToRad = { M_PI / 180.0 } |
static constexpr double | PI = { M_PI } |
Protected Attributes | |
double | D |
Detailed Description
An angle, stored as degrees, but expressible in many ways.
- Version
- 1.0
dms encapsulates an angle. The angle is stored as a double, equal to the value of the angle in degrees. Methods are available for setting/getting the angle as a floating-point measured in Degrees or Hours, or as integer triplets (degrees, arcminutes, arcseconds or hours, minutes, seconds). There is also a method to set the angle according to a radian value, and to return the angle expressed in radians. Finally, a SinCos() method computes the sin and cosine of the angle.
Member Enumeration Documentation
◆ AngleRanges
enum dms::AngleRanges |
Constructor & Destructor Documentation
◆ dms() [1/4]
◆ ~dms()
|
virtualdefault |
Empty virtual destructor.
◆ dms() [2/4]
|
inlineexplicit |
Set the floating-point value of the angle according to the four integer arguments.
- Parameters
-
d degree portion of angle (int). Defaults to zero. m arcminute portion of angle (int). Defaults to zero. s arcsecond portion of angle (int). Defaults to zero. ms arcsecond portion of angle (int). Defaults to zero.
◆ dms() [3/4]
|
inlineexplicit |
◆ dms() [4/4]
|
inlineexplicit |
Construct an angle from a string representation.
Attempt to create the angle according to the string argument. If the string cannot be parsed as an angle value, the angle is set to zero.
- Warning
- There is not an unambiguous notification that it failed to parse the string, since the string could have been a valid representation of zero degrees. If this is a concern, use the setFromString() function directly instead.
- Parameters
-
s the string to parse as a dms value. isDeg if true, value is in degrees; if false, value is in hours.
- See also
- setFromString()
Member Function Documentation
◆ arcmin()
int dms::arcmin | ( | void | ) | const |
◆ arcsec()
int dms::arcsec | ( | void | ) | const |
◆ cos()
|
inline |
◆ degree()
|
inline |
◆ Degrees()
|
inline |
◆ deltaAngle()
deltaAngle Return the shortest difference (path) between this angle and the supplied angle.
The range is normalized to [-180,+180].
- Parameters
-
angle Angle to subtract from current angle.
- Returns
- Normalized angle in the range [-180,+180] which is positive going counter-clockwise.
◆ fromString()
Static function to create a DMS object from a QString.
There are several ways to specify the angle:
- Integer numbers ( 5 or -33 )
- Floating-point numbers ( 5.0 or -33.0 )
- colon-delimited integers ( 5:0:0 or -33:0:0 )
- colon-delimited with float seconds ( 5:0:0.0 or -33:0:0.0 )
- colon-delimited with float minutes ( 5:0.0 or -33:0.0 )
- space-delimited ( 5 0 0; -33 0 0 ) or ( 5 0.0 or -33 0.0 )
- space-delimited, with unit labels ( 5h 0m 0s or -33d 0m 0s )
- Parameters
-
s the string to be parsed as an angle value deg if true, s is expressed in degrees; if false, s is expressed in hours
- Returns
- a dms object whose value is parsed from the string argument
◆ hour()
|
inline |
◆ Hours()
|
inline |
◆ HoursHa()
|
inline |
◆ marcsec()
int dms::marcsec | ( | void | ) | const |
◆ minute()
int dms::minute | ( | void | ) | const |
◆ msecond()
int dms::msecond | ( | void | ) | const |
◆ operator-()
◆ radians()
|
inline |
◆ reduce() [1/2]
const dms dms::reduce | ( | void | ) | const |
◆ reduce() [2/2]
|
static |
◆ reduceToRange()
void dms::reduceToRange | ( | enum dms::AngleRanges | range | ) |
◆ second()
int dms::second | ( | void | ) | const |
◆ setD() [1/2]
|
inlinevirtual |
Sets floating-point value of angle, in degrees.
- Parameters
-
x new angle (double)
Reimplemented in CachingDms.
◆ setD() [2/2]
|
virtual |
Sets floating-point value of angle, in degrees.
This is an overloaded member function; it behaves essentially like the above function. The floating-point value of the angle (D) is determined from the following formulae:
- Parameters
-
d integer degrees portion of angle m integer arcminutes portion of angle s integer arcseconds portion of angle ms integer arcseconds portion of angle
Reimplemented in CachingDms.
◆ setFromString()
|
virtual |
Attempt to parse the string argument as a dms value, and set the dms object accordingly.
- Parameters
-
s the string to be parsed as a dms value. The string can be an int or floating-point value, or a triplet of values (d/h, m, s) separated by spaces or colons. isDeg if true, the value is in degrees. Otherwise, it is in hours.
- Returns
- true if sting was parsed successfully. Otherwise, set the dms value to 0.0 and return false.
Reimplemented in CachingDms.
◆ setH() [1/2]
|
inlinevirtual |
Sets floating-point value of angle, in hours.
Converts argument from hours to degrees, then sets floating-point value of angle, in degrees.
- Parameters
-
x new angle, in hours (double)
- See also
- setD()
Reimplemented in CachingDms.
◆ setH() [2/2]
|
virtual |
Sets floating-point value of angle, in hours.
Converts argument values from hours to degrees, then sets floating-point value of angle, in degrees. This is an overloaded member function, provided for convenience. It behaves essentially like the above function.
- Parameters
-
h integer hours portion of angle m integer minutes portion of angle s integer seconds portion of angle ms integer milliseconds portion of angle
- See also
- setD()
Reimplemented in CachingDms.
◆ setRadians()
|
inlinevirtual |
Set angle according to the argument, in radians.
This function converts the argument to degrees, then sets the angle with setD().
- Parameters
-
Rad an angle in radians
Reimplemented in CachingDms.
◆ sin()
|
inline |
◆ SinCos()
|
inline |
◆ tan()
|
inline |
◆ toDMSString()
const QString dms::toDMSString | ( | const bool | forceSign = false, |
const bool | machineReadable = false, | ||
const bool | highPrecision = false ) const |
- Returns
- a nicely-formatted string representation of the angle in degrees, arcminutes, and arcseconds.
- Parameters
-
forceSign if true
then adds '+' or '-' to the stringmachineReadable uses a colon separator and produces +/-dd:mm:ss format instead highPrecision adds milliseconds, if false
the seconds will be shown as an integer
◆ toHMSString()
const QString dms::toHMSString | ( | const bool | machineReadable = false, |
const bool | highPrecision = false ) const |
Member Data Documentation
◆ D
◆ DegToRad
|
staticconstexpr |
◆ PI
|
staticconstexpr |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.