CachingDms
#include <cachingdms.h>
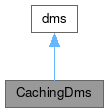
Public Member Functions | |
CachingDms () | |
CachingDms (const dms &angle) | |
CachingDms (const double &x) | |
CachingDms (const int &d, const int &m=0, const int &s=0, const int &ms=0) | |
CachingDms (const QString &s, bool isDeg=true) | |
double | cos () const |
CachingDms | operator- () |
void | setD (const double &x) override |
void | setD (const int &d, const int &m, const int &s, const int &ms=0) override |
bool | setFromString (const QString &s, bool isDeg=true) override |
void | setH (const double &x) override |
void | setH (const int &h, const int &m, const int &s, const int &ms=0) override |
void | setRadians (const double &a) override |
void | setUsing_acos (const double &cosine) |
void | setUsing_asin (const double &sine) |
void | setUsing_atan2 (const double &y, const double &x) |
double | sin () const |
void | SinCos (double &s, double &c) const |
![]() | |
dms () | |
dms (const double &x) | |
dms (const int &d, const int &m=0, const int &s=0, const int &ms=0) | |
dms (const QString &s, bool isDeg=true) | |
virtual | ~dms ()=default |
int | arcmin () const |
int | arcsec () const |
double | cos () const |
int | degree () const |
const double & | Degrees () const |
const dms | deltaAngle (dms angle) const |
int | hour () const |
double | Hours () const |
double | HoursHa () const |
int | marcsec () const |
int | minute () const |
int | msecond () const |
dms | operator- () |
double | radians () const |
const dms | reduce () const |
void | reduceToRange (enum dms::AngleRanges range) |
int | second () const |
double | sin () const |
void | SinCos (double &s, double &c) const |
double | tan () const |
const QString | toDMSString (const bool forceSign=false, const bool machineReadable=false, const bool highPrecision=false) const |
const QString | toHMSString (const bool machineReadable=false, const bool highPrecision=false) const |
Static Public Member Functions | |
static CachingDms | fromString (const QString &s, bool deg) |
![]() | |
static dms | fromString (const QString &s, bool deg) |
static double | reduce (const double D) |
Additional Inherited Members | |
![]() | |
enum | AngleRanges { ZERO_TO_2PI , MINUSPI_TO_PI } |
![]() | |
static constexpr double | DegToRad = { M_PI / 180.0 } |
static constexpr double | PI = { M_PI } |
![]() | |
double | D |
Detailed Description
a dms subclass that caches its sine and cosine values every time the angle is changed.
- Note
- This is to be used for those angles where sin/cos is repeatedly computed.
Definition at line 18 of file cachingdms.h.
Constructor & Destructor Documentation
◆ CachingDms() [1/5]
|
inline |
Default Constructor.
Definition at line 24 of file cachingdms.h.
◆ CachingDms() [2/5]
|
explicit |
Degree angle constructor.
- Parameters
-
x is the angle in degrees
Definition at line 24 of file cachingdms.cpp.
◆ CachingDms() [3/5]
|
explicit |
QString constructor.
Definition at line 42 of file cachingdms.cpp.
◆ CachingDms() [4/5]
|
explicit |
DMS representation constructor.
Definition at line 52 of file cachingdms.cpp.
◆ CachingDms() [5/5]
CachingDms::CachingDms | ( | const dms & | angle | ) |
Casting constructor.
Definition at line 133 of file cachingdms.cpp.
Member Function Documentation
◆ cos()
|
inline |
Get the cosine of this angle.
- Note
- Re-implements dms::cos()
- This just uses the cached value assuming that it is good
Definition at line 204 of file cachingdms.h.
◆ fromString()
|
static |
Construct an angle from the given string.
- Note
- Re-implements dms::fromString()
Definition at line 121 of file cachingdms.cpp.
◆ operator-()
CachingDms CachingDms::operator- | ( | ) |
operator -
- Note
- In addition to negating the angle, we negate the sine value
Definition at line 128 of file cachingdms.cpp.
◆ setD() [1/2]
|
inlineoverridevirtual |
Sets the angle in degrees supplied as a double.
- Note
- Re-implements dms::setD() with sine/cosine caching
Reimplemented from dms.
Definition at line 59 of file cachingdms.h.
◆ setD() [2/2]
|
inlineoverridevirtual |
◆ setFromString()
|
inlineoverridevirtual |
Sets the angle from string.
- Note
- Re-implements dms::setFromString()
Reimplemented from dms.
Definition at line 120 of file cachingdms.h.
◆ setH() [1/2]
|
inlineoverridevirtual |
Sets the angle in hours, supplied as a double.
- Note
- Re-implements dms::setH() with sine/cosine caching
- While this and other methods internally call setD, we want to avoid unnecessary vtable lookups. We'd rather have inline than virtual when speed matters in general.
Reimplemented from dms.
Definition at line 91 of file cachingdms.h.
◆ setH() [2/2]
|
inlineoverridevirtual |
Sets the angle in HMS form.
- Note
- Re-implements dms::setH() with sine/cosine caching
Reimplemented from dms.
Definition at line 107 of file cachingdms.h.
◆ setRadians()
|
inlineoverridevirtual |
◆ setUsing_acos()
void CachingDms::setUsing_acos | ( | const double & | cosine | ) |
Sets the angle using acos()
- Parameters
-
cosine Cosine of the angle
- Note
- The advantage is that we can cache the cosine value supplied
- The limited range of acos must be borne in mind
Definition at line 107 of file cachingdms.cpp.
◆ setUsing_asin()
void CachingDms::setUsing_asin | ( | const double & | sine | ) |
Sets the angle using asin()
- Parameters
-
sine Sine of the angle
- Note
- The advantage is that we can cache the sine value supplied
- The limited range of asin must be borne in mind
Definition at line 93 of file cachingdms.cpp.
◆ setUsing_atan2()
void CachingDms::setUsing_atan2 | ( | const double & | y, |
const double & | x ) |
Sets the angle using atan2()
- Note
- The advantage is that we can calculate sin/cos faster because we know the tangent
Definition at line 62 of file cachingdms.cpp.
◆ sin()
|
inline |
Get the sine of this angle.
- Note
- Re-implements dms::sin()
- This just uses the cached value assuming that it is good
Definition at line 190 of file cachingdms.h.
◆ SinCos()
|
inline |
Get the sine and cosine together.
- Note
- Re-implements dms::SinCos()
- This just uses the cached values assuming that they are good
Definition at line 175 of file cachingdms.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.