SkyPainter
#include <skypainter.h>
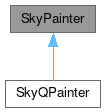
Public Member Functions | |
virtual void | begin ()=0 |
virtual bool | drawAsteroid (KSAsteroid *ast)=0 |
virtual bool | drawCatalogObject (const CatalogObject &obj)=0 |
virtual bool | drawComet (KSComet *com)=0 |
virtual bool | drawConstellationArtImage (ConstellationsArt *obj)=0 |
virtual bool | drawEarthShadow (KSEarthShadow *shadow)=0 |
virtual void | drawFlags ()=0 |
virtual bool | drawHips (bool useCache=false)=0 |
virtual void | drawHorizon (bool filled, SkyPoint *labelPoint=nullptr, bool *drawLabel=nullptr)=0 |
virtual bool | drawImageOverlay (const QList< ImageOverlay > *imageOverlays, bool useCache=false)=0 |
virtual void | drawObservingList (const QList< SkyObject * > &obs)=0 |
virtual bool | drawPlanet (KSPlanetBase *planet)=0 |
virtual bool | drawPointSource (const SkyPoint *loc, float mag, char sp='A')=0 |
virtual bool | drawSatellite (Satellite *sat)=0 |
virtual void | drawSkyBackground ()=0 |
virtual void | drawSkyLine (SkyPoint *a, SkyPoint *b)=0 |
virtual void | drawSkyPolygon (LineList *list, bool forceClip=true)=0 |
virtual void | drawSkyPolyline (LineList *list, SkipHashList *skipList=nullptr, LineListLabel *label=nullptr)=0 |
virtual bool | drawSupernova (Supernova *sup)=0 |
virtual bool | drawTerrain (bool useCache=false)=0 |
virtual void | end ()=0 |
virtual void | setBrush (const QBrush &brush)=0 |
virtual void | setPen (const QPen &pen)=0 |
void | setSizeMagLimit (float sizeMagLim) |
float | starWidth (float mag) const |
Detailed Description
Draws things on the sky, without regard to backend.
This class serves as an interface to draw objects onto the sky without worrying about whether we are using a QPainter or OpenGL.
Definition at line 39 of file skypainter.h.
Constructor & Destructor Documentation
◆ SkyPainter()
SkyPainter::SkyPainter | ( | ) |
Definition at line 21 of file skypainter.cpp.
Member Function Documentation
◆ begin()
|
pure virtual |
Begin painting.
- Note
- this function must be called before painting anything.
- See also
- end()
Implemented in SkyQPainter.
◆ drawAsteroid()
|
pure virtual |
Draw an asteroid in the sky.
- Parameters
-
ast asteroid to draw
- Returns
- true if a asteroid was drawn
Implemented in SkyQPainter.
◆ drawCatalogObject()
|
pure virtual |
Draw a deep sky object (loaded from the new implementation)
- Parameters
-
obj the object to draw drawImage if true, try to draw the image of the object
- Returns
- true if it was drawn
Implemented in SkyQPainter.
◆ drawComet()
|
pure virtual |
Draw a comet in the sky.
- Parameters
-
com comet to draw
- Returns
- true if a comet was drawn
Implemented in SkyQPainter.
◆ drawConstellationArtImage()
|
pure virtual |
Draw a ConstellationsArt object.
- Parameters
-
obj the object to draw
- Returns
- true if it was drawn
Implemented in SkyQPainter.
◆ drawEarthShadow()
|
pure virtual |
Draw the earths shadow on the moon (red-ish)
- Parameters
-
shadow the shadow to draw
- Returns
- true if it was drawn
Implemented in SkyQPainter.
◆ drawFlags()
|
pure virtual |
Draw flags.
Implemented in SkyQPainter.
◆ drawHips()
|
pure virtual |
drawMosaicPanel Draws mosaic panel in planning or operation mode.
- Returns
- true if it was drawn
drawHips Draw HIPS all sky catalog
- Parameters
-
useCache if True, try to re-use last generated image instead of rendering a new image.
- Returns
- true if it was drawn
Implemented in SkyQPainter.
◆ drawImageOverlay()
|
pure virtual |
drawImageOverlay Draws a user-supplied image onto the skymap
- Parameters
-
useCache if True, try to re-use last generated image instead of rendering a new image.
- Returns
- true if it was drawn
Implemented in SkyQPainter.
◆ drawObservingList()
Draw the symbols for the observing list.
- Parameters
-
obs the observing list
Implemented in SkyQPainter.
◆ drawPlanet()
|
pure virtual |
Draw a planet.
- Parameters
-
planet the planet to draw
- Returns
- true if it was drawn
Implemented in SkyQPainter.
◆ drawPointSource()
|
pure virtual |
Draw a point source (e.g., a star).
- Parameters
-
loc the location of the source in the sky mag the magnitude of the source sp the spectral class of the source
- Returns
- true if a source was drawn
Implemented in SkyQPainter.
◆ drawSatellite()
|
pure virtual |
Draw a satellite.
Implemented in SkyQPainter.
◆ drawSkyBackground()
|
pure virtual |
Draw the sky background.
Implemented in SkyQPainter.
◆ drawSkyLine()
Draw a line between points in the sky.
- Parameters
-
a the first point b the second point
- Note
- this function will skip lines not on screen and clip lines that are only partially visible.
Implemented in SkyQPainter.
◆ drawSkyPolygon()
|
pure virtual |
Draw a polygon in the sky.
- Parameters
-
list a list of points in the sky forceClip If true (default), it enforces clipping of the polygon, otherwise, it draws the complete polygen without running any boundary checks.
- See also
- drawSkyPolyline()
Implemented in SkyQPainter.
◆ drawSkyPolyline()
|
pure virtual |
Draw a polyline in the sky.
- Parameters
-
list a list of points in the sky skipList a SkipList object used to control skipping line segments label a pointer to the label for this line
- Note
- it's more efficient to use this than repeated calls to drawSkyLine(), because it avoids an extra points->size() -2 projections.
Implemented in SkyQPainter.
◆ drawSupernova()
|
pure virtual |
Draw a Supernova.
Implemented in SkyQPainter.
◆ drawTerrain()
|
pure virtual |
drawTerrain Draw the Terrain
- Parameters
-
useCache if True, try to re-use last generated image instead of rendering a new image.
- Returns
- true if it was drawn
Implemented in SkyQPainter.
◆ end()
|
pure virtual |
End and finalize painting.
- Note
- this function must be called after painting anything.
- it is not guaranteed that anything will actually be drawn until end() is called.
- See also
- begin();
Implemented in SkyQPainter.
◆ setBrush()
|
pure virtual |
Set the brush of the painter.
Implemented in SkyQPainter.
◆ setPen()
|
pure virtual |
Set the pen of the painter.
Implemented in SkyQPainter.
◆ setSizeMagLimit()
void SkyPainter::setSizeMagLimit | ( | float | sizeMagLim | ) |
Definition at line 25 of file skypainter.cpp.
◆ starWidth()
float SkyPainter::starWidth | ( | float | mag | ) | const |
Get the width of a star of magnitude mag.
Definition at line 30 of file skypainter.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 21 2025 11:54:30 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.