SkyQPainter
#include <skyqpainter.h>
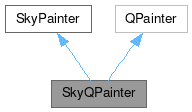
Public Member Functions | |
SkyQPainter (QPaintDevice *pd) | |
SkyQPainter (QPaintDevice *pd, const QSize &canvasSize) | |
SkyQPainter (QWidget *widget, QPaintDevice *pd=nullptr) | |
void | begin () override |
bool | drawAsteroid (KSAsteroid *ast) override |
bool | drawCatalogObject (const CatalogObject &obj) override |
void | drawCatalogObjectImage (const QPointF &pos, const CatalogObject &obj, float positionAngle) |
bool | drawComet (KSComet *com) override |
bool | drawConstellationArtImage (ConstellationsArt *obj) override |
virtual void | drawDeepSkySymbol (const QPointF &pos, int type, float size, float e, float positionAngle) |
bool | drawEarthShadow (KSEarthShadow *shadow) override |
void | drawFlags () override |
bool | drawHips (bool useCache=false) override |
void | drawHorizon (bool filled, SkyPoint *labelPoint=nullptr, bool *drawLabel=nullptr) override |
bool | drawImageOverlay (const QList< ImageOverlay > *imageOverlays, bool useCache=false) override |
void | drawObservingList (const QList< SkyObject * > &obs) override |
bool | drawPlanet (KSPlanetBase *planet) override |
virtual void | drawPointSource (const QPointF &pos, float size, char sp='A') |
bool | drawPointSource (const SkyPoint *loc, float mag, char sp='A') override |
bool | drawSatellite (Satellite *sat) override |
void | drawSkyBackground () override |
void | drawSkyLine (SkyPoint *a, SkyPoint *b) override |
void | drawSkyPolygon (LineList *list, bool forceClip=true) override |
void | drawSkyPolyline (LineList *list, SkipHashList *skipList=nullptr, LineListLabel *label=nullptr) override |
bool | drawSupernova (Supernova *sup) override |
bool | drawTerrain (bool useCache=false) override |
void | end () override |
bool | getVectorStars () const |
void | setBrush (const QBrush &brush) override |
void | setPaintDevice (QPaintDevice *pd) |
void | setPen (const QPen &pen) override |
void | setSize (int width, int height) |
void | setVectorStars (bool vectorStars) |
![]() | |
void | setSizeMagLimit (float sizeMagLim) |
float | starWidth (float mag) const |
![]() | |
QPainter (QPaintDevice *device) | |
const QBrush & | background () const const |
Qt::BGMode | backgroundMode () const const |
bool | begin (QPaintDevice *device) |
void | beginNativePainting () |
QRect | boundingRect (const QRect &rectangle, int flags, const QString &text) |
QRectF | boundingRect (const QRectF &rectangle, const QString &text, const QTextOption &option) |
QRectF | boundingRect (const QRectF &rectangle, int flags, const QString &text) |
QRect | boundingRect (int x, int y, int w, int h, int flags, const QString &text) |
const QBrush & | brush () const const |
QPoint | brushOrigin () const const |
QRectF | clipBoundingRect () const const |
QPainterPath | clipPath () const const |
QRegion | clipRegion () const const |
QTransform | combinedTransform () const const |
CompositionMode | compositionMode () const const |
QPaintDevice * | device () const const |
const QTransform & | deviceTransform () const const |
void | drawArc (const QRect &rectangle, int startAngle, int spanAngle) |
void | drawArc (const QRectF &rectangle, int startAngle, int spanAngle) |
void | drawArc (int x, int y, int width, int height, int startAngle, int spanAngle) |
void | drawChord (const QRect &rectangle, int startAngle, int spanAngle) |
void | drawChord (const QRectF &rectangle, int startAngle, int spanAngle) |
void | drawChord (int x, int y, int width, int height, int startAngle, int spanAngle) |
void | drawConvexPolygon (const QPoint *points, int pointCount) |
void | drawConvexPolygon (const QPointF *points, int pointCount) |
void | drawConvexPolygon (const QPolygon &polygon) |
void | drawConvexPolygon (const QPolygonF &polygon) |
void | drawEllipse (const QPoint ¢er, int rx, int ry) |
void | drawEllipse (const QPointF ¢er, qreal rx, qreal ry) |
void | drawEllipse (const QRect &rectangle) |
void | drawEllipse (const QRectF &rectangle) |
void | drawEllipse (int x, int y, int width, int height) |
void | drawGlyphRun (const QPointF &position, const QGlyphRun &glyphs) |
void | drawImage (const QPoint &point, const QImage &image) |
void | drawImage (const QPoint &point, const QImage &image, const QRect &source, Qt::ImageConversionFlags flags) |
void | drawImage (const QPointF &point, const QImage &image) |
void | drawImage (const QPointF &point, const QImage &image, const QRectF &source, Qt::ImageConversionFlags flags) |
void | drawImage (const QRect &rectangle, const QImage &image) |
void | drawImage (const QRect &target, const QImage &image, const QRect &source, Qt::ImageConversionFlags flags) |
void | drawImage (const QRectF &rectangle, const QImage &image) |
void | drawImage (const QRectF &target, const QImage &image, const QRectF &source, Qt::ImageConversionFlags flags) |
void | drawImage (int x, int y, const QImage &image, int sx, int sy, int sw, int sh, Qt::ImageConversionFlags flags) |
void | drawLine (const QLine &line) |
void | drawLine (const QLineF &line) |
void | drawLine (const QPoint &p1, const QPoint &p2) |
void | drawLine (const QPointF &p1, const QPointF &p2) |
void | drawLine (int x1, int y1, int x2, int y2) |
void | drawLines (const QLine *lines, int lineCount) |
void | drawLines (const QLineF *lines, int lineCount) |
void | drawLines (const QList< QLine > &lines) |
void | drawLines (const QList< QLineF > &lines) |
void | drawLines (const QList< QPoint > &pointPairs) |
void | drawLines (const QList< QPointF > &pointPairs) |
void | drawLines (const QPoint *pointPairs, int lineCount) |
void | drawLines (const QPointF *pointPairs, int lineCount) |
void | drawPath (const QPainterPath &path) |
void | drawPicture (const QPoint &point, const QPicture &picture) |
void | drawPicture (const QPointF &point, const QPicture &picture) |
void | drawPicture (int x, int y, const QPicture &picture) |
void | drawPie (const QRect &rectangle, int startAngle, int spanAngle) |
void | drawPie (const QRectF &rectangle, int startAngle, int spanAngle) |
void | drawPie (int x, int y, int width, int height, int startAngle, int spanAngle) |
void | drawPixmap (const QPoint &point, const QPixmap &pixmap) |
void | drawPixmap (const QPoint &point, const QPixmap &pixmap, const QRect &source) |
void | drawPixmap (const QPointF &point, const QPixmap &pixmap) |
void | drawPixmap (const QPointF &point, const QPixmap &pixmap, const QRectF &source) |
void | drawPixmap (const QRect &rectangle, const QPixmap &pixmap) |
void | drawPixmap (const QRect &target, const QPixmap &pixmap, const QRect &source) |
void | drawPixmap (const QRectF &target, const QPixmap &pixmap, const QRectF &source) |
void | drawPixmap (int x, int y, const QPixmap &pixmap) |
void | drawPixmap (int x, int y, const QPixmap &pixmap, int sx, int sy, int sw, int sh) |
void | drawPixmap (int x, int y, int w, int h, const QPixmap &pixmap, int sx, int sy, int sw, int sh) |
void | drawPixmap (int x, int y, int width, int height, const QPixmap &pixmap) |
void | drawPixmapFragments (const PixmapFragment *fragments, int fragmentCount, const QPixmap &pixmap, PixmapFragmentHints hints) |
void | drawPoint (const QPoint &position) |
void | drawPoint (const QPointF &position) |
void | drawPoint (int x, int y) |
void | drawPoints (const QPoint *points, int pointCount) |
void | drawPoints (const QPointF *points, int pointCount) |
void | drawPoints (const QPolygon &points) |
void | drawPoints (const QPolygonF &points) |
void | drawPolygon (const QPoint *points, int pointCount, Qt::FillRule fillRule) |
void | drawPolygon (const QPointF *points, int pointCount, Qt::FillRule fillRule) |
void | drawPolygon (const QPolygon &points, Qt::FillRule fillRule) |
void | drawPolygon (const QPolygonF &points, Qt::FillRule fillRule) |
void | drawPolyline (const QPoint *points, int pointCount) |
void | drawPolyline (const QPointF *points, int pointCount) |
void | drawPolyline (const QPolygon &points) |
void | drawPolyline (const QPolygonF &points) |
void | drawRect (const QRect &rectangle) |
void | drawRect (const QRectF &rectangle) |
void | drawRect (int x, int y, int width, int height) |
void | drawRects (const QList< QRect > &rectangles) |
void | drawRects (const QList< QRectF > &rectangles) |
void | drawRects (const QRect *rectangles, int rectCount) |
void | drawRects (const QRectF *rectangles, int rectCount) |
void | drawRoundedRect (const QRect &rect, qreal xRadius, qreal yRadius, Qt::SizeMode mode) |
void | drawRoundedRect (const QRectF &rect, qreal xRadius, qreal yRadius, Qt::SizeMode mode) |
void | drawRoundedRect (int x, int y, int w, int h, qreal xRadius, qreal yRadius, Qt::SizeMode mode) |
void | drawStaticText (const QPoint &topLeftPosition, const QStaticText &staticText) |
void | drawStaticText (const QPointF &topLeftPosition, const QStaticText &staticText) |
void | drawStaticText (int left, int top, const QStaticText &staticText) |
void | drawText (const QPoint &position, const QString &text) |
void | drawText (const QPointF &position, const QString &text) |
void | drawText (const QRect &rectangle, int flags, const QString &text, QRect *boundingRect) |
void | drawText (const QRectF &rectangle, const QString &text, const QTextOption &option) |
void | drawText (const QRectF &rectangle, int flags, const QString &text, QRectF *boundingRect) |
void | drawText (int x, int y, const QString &text) |
void | drawText (int x, int y, int width, int height, int flags, const QString &text, QRect *boundingRect) |
void | drawTiledPixmap (const QRect &rectangle, const QPixmap &pixmap, const QPoint &position) |
void | drawTiledPixmap (const QRectF &rectangle, const QPixmap &pixmap, const QPointF &position) |
void | drawTiledPixmap (int x, int y, int width, int height, const QPixmap &pixmap, int sx, int sy) |
bool | end () |
void | endNativePainting () |
void | eraseRect (const QRect &rectangle) |
void | eraseRect (const QRectF &rectangle) |
void | eraseRect (int x, int y, int width, int height) |
void | fillPath (const QPainterPath &path, const QBrush &brush) |
void | fillRect (const QRect &rectangle, const QBrush &brush) |
void | fillRect (const QRect &rectangle, const QColor &color) |
void | fillRect (const QRect &rectangle, QGradient::Preset preset) |
void | fillRect (const QRect &rectangle, Qt::BrushStyle style) |
void | fillRect (const QRect &rectangle, Qt::GlobalColor color) |
void | fillRect (const QRectF &rectangle, const QBrush &brush) |
void | fillRect (const QRectF &rectangle, const QColor &color) |
void | fillRect (const QRectF &rectangle, QGradient::Preset preset) |
void | fillRect (const QRectF &rectangle, Qt::BrushStyle style) |
void | fillRect (const QRectF &rectangle, Qt::GlobalColor color) |
void | fillRect (int x, int y, int width, int height, const QBrush &brush) |
void | fillRect (int x, int y, int width, int height, const QColor &color) |
void | fillRect (int x, int y, int width, int height, QGradient::Preset preset) |
void | fillRect (int x, int y, int width, int height, Qt::BrushStyle style) |
void | fillRect (int x, int y, int width, int height, Qt::GlobalColor color) |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
bool | hasClipping () const const |
bool | isActive () const const |
Qt::LayoutDirection | layoutDirection () const const |
qreal | opacity () const const |
QPaintEngine * | paintEngine () const const |
const QPen & | pen () const const |
RenderHints | renderHints () const const |
void | resetTransform () |
void | restore () |
void | rotate (qreal angle) |
void | save () |
void | scale (qreal sx, qreal sy) |
void | setBackground (const QBrush &brush) |
void | setBackgroundMode (Qt::BGMode mode) |
void | setBrush (const QBrush &brush) |
void | setBrush (Qt::BrushStyle style) |
void | setBrushOrigin (const QPoint &position) |
void | setBrushOrigin (const QPointF &position) |
void | setBrushOrigin (int x, int y) |
void | setClipPath (const QPainterPath &path, Qt::ClipOperation operation) |
void | setClipping (bool enable) |
void | setClipRect (const QRect &rectangle, Qt::ClipOperation operation) |
void | setClipRect (const QRectF &rectangle, Qt::ClipOperation operation) |
void | setClipRect (int x, int y, int width, int height, Qt::ClipOperation operation) |
void | setClipRegion (const QRegion ®ion, Qt::ClipOperation operation) |
void | setCompositionMode (CompositionMode mode) |
void | setFont (const QFont &font) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setOpacity (qreal opacity) |
void | setPen (const QColor &color) |
void | setPen (const QPen &pen) |
void | setPen (Qt::PenStyle style) |
void | setRenderHint (RenderHint hint, bool on) |
void | setRenderHints (RenderHints hints, bool on) |
void | setTransform (const QTransform &transform, bool combine) |
void | setViewport (const QRect &rectangle) |
void | setViewport (int x, int y, int width, int height) |
void | setViewTransformEnabled (bool enable) |
void | setWindow (const QRect &rectangle) |
void | setWindow (int x, int y, int width, int height) |
void | setWorldMatrixEnabled (bool enable) |
void | setWorldTransform (const QTransform &matrix, bool combine) |
void | shear (qreal sh, qreal sv) |
void | strokePath (const QPainterPath &path, const QPen &pen) |
bool | testRenderHint (RenderHint hint) const const |
const QTransform & | transform () const const |
void | translate (const QPoint &offset) |
void | translate (const QPointF &offset) |
void | translate (qreal dx, qreal dy) |
QRect | viewport () const const |
bool | viewTransformEnabled () const const |
QRect | window () const const |
bool | worldMatrixEnabled () const const |
const QTransform & | worldTransform () const const |
Static Public Member Functions | |
static void | initStarImages () |
static void | releaseImageCache () |
Detailed Description
The QPainter-based painting backend.
This class implements the SkyPainter interface using a QPainter. For documentation,
- See also
- SkyPainter.
Definition at line 30 of file skyqpainter.h.
Constructor & Destructor Documentation
◆ SkyQPainter() [1/3]
SkyQPainter::SkyQPainter | ( | QPaintDevice * | pd, |
const QSize & | canvasSize ) |
Creates a SkyQPainter with the given QPaintDevice and uses the dimensions of the paint device as canvas dimensions.
- Parameters
-
pd the painting device. Cannot be 0 canvasSize the size of the canvas
Definition at line 120 of file skyqpainter.cpp.
◆ SkyQPainter() [2/3]
|
explicit |
Creates a SkyQPainter with the given QPaintDevice and given canvas size.
- Parameters
-
pd the painting device. Cannot be 0
Definition at line 112 of file skyqpainter.cpp.
◆ SkyQPainter() [3/3]
|
explicit |
Creates a SkyQPainter given a QWidget and an optional QPaintDevice.
- Parameters
-
widget the QWidget that provides the canvas size, and also the paint device unless pd
is specifiedpd the painting device. If 0, then widget
will be used.
Definition at line 128 of file skyqpainter.cpp.
◆ ~SkyQPainter()
|
override |
Definition at line 137 of file skyqpainter.cpp.
Member Function Documentation
◆ begin()
|
overridevirtual |
Begin painting.
- Note
- this function must be called before painting anything.
- See also
- end()
Implements SkyPainter.
Definition at line 148 of file skyqpainter.cpp.
◆ drawAsteroid()
|
overridevirtual |
Draw an asteroid in the sky.
- Parameters
-
ast asteroid to draw
- Returns
- true if a asteroid was drawn
Implements SkyPainter.
Definition at line 692 of file skyqpainter.cpp.
◆ drawCatalogObject()
|
overridevirtual |
Draw a deep sky object (loaded from the new implementation)
- Parameters
-
obj the object to draw drawImage if true, try to draw the image of the object
- Returns
- true if it was drawn
Implements SkyPainter.
Definition at line 967 of file skyqpainter.cpp.
◆ drawCatalogObjectImage()
void SkyQPainter::drawCatalogObjectImage | ( | const QPointF & | pos, |
const CatalogObject & | obj, | ||
float | positionAngle ) |
Definition at line 948 of file skyqpainter.cpp.
◆ drawComet()
|
overridevirtual |
Draw a comet in the sky.
- Parameters
-
com comet to draw
- Returns
- true if a comet was drawn
Implements SkyPainter.
Definition at line 624 of file skyqpainter.cpp.
◆ drawConstellationArtImage()
|
overridevirtual |
Draw a ConstellationsArt object.
- Parameters
-
obj the object to draw
- Returns
- true if it was drawn
Implements SkyPainter.
Definition at line 772 of file skyqpainter.cpp.
◆ drawDeepSkySymbol()
|
virtual |
Definition at line 1003 of file skyqpainter.cpp.
◆ drawEarthShadow()
|
overridevirtual |
Draw the earths shadow on the moon (red-ish)
- Parameters
-
shadow the shadow to draw
- Returns
- true if it was drawn
Implements SkyPainter.
Definition at line 598 of file skyqpainter.cpp.
◆ drawFlags()
|
overridevirtual |
◆ drawHips()
|
overridevirtual |
drawMosaicPanel Draws mosaic panel in planning or operation mode.
- Returns
- true if it was drawn
drawHips Draw HIPS all sky catalog
- Parameters
-
useCache if True, try to re-use last generated image instead of rendering a new image.
- Returns
- true if it was drawn
Implements SkyPainter.
Definition at line 839 of file skyqpainter.cpp.
◆ drawHorizon()
|
overridevirtual |
Implements SkyPainter.
Definition at line 1292 of file skyqpainter.cpp.
◆ drawImageOverlay()
|
overridevirtual |
drawImageOverlay Draws a user-supplied image onto the skymap
- Parameters
-
useCache if True, try to re-use last generated image instead of rendering a new image.
- Returns
- true if it was drawn
Implements SkyPainter.
Definition at line 875 of file skyqpainter.cpp.
◆ drawObservingList()
Draw the symbols for the observing list.
- Parameters
-
obs the observing list
Implements SkyPainter.
Definition at line 1242 of file skyqpainter.cpp.
◆ drawPlanet()
|
overridevirtual |
Draw a planet.
- Parameters
-
planet the planet to draw
- Returns
- true if it was drawn
Implements SkyPainter.
Definition at line 508 of file skyqpainter.cpp.
◆ drawPointSource() [1/2]
|
virtual |
This function exists so that we can draw other objects (e.g., planets) as point sources.
Definition at line 736 of file skyqpainter.cpp.
◆ drawPointSource() [2/2]
|
overridevirtual |
Draw a point source (e.g., a star).
- Parameters
-
loc the location of the source in the sky mag the magnitude of the source sp the spectral class of the source
- Returns
- true if a source was drawn
Implements SkyPainter.
Definition at line 716 of file skyqpainter.cpp.
◆ drawSatellite()
|
overridevirtual |
◆ drawSkyBackground()
|
overridevirtual |
◆ drawSkyLine()
Draw a line between points in the sky.
- Parameters
-
a the first point b the second point
- Note
- this function will skip lines not on screen and clip lines that are only partially visible.
Implements SkyPainter.
Definition at line 370 of file skyqpainter.cpp.
◆ drawSkyPolygon()
|
overridevirtual |
Draw a polygon in the sky.
- Parameters
-
list a list of points in the sky forceClip If true (default), it enforces clipping of the polygon, otherwise, it draws the complete polygen without running any boundary checks.
- See also
- drawSkyPolyline()
Implements SkyPainter.
Definition at line 450 of file skyqpainter.cpp.
◆ drawSkyPolyline()
|
overridevirtual |
Draw a polyline in the sky.
- Parameters
-
list a list of points in the sky skipList a SkipList object used to control skipping line segments label a pointer to the label for this line
- Note
- it's more efficient to use this than repeated calls to drawSkyLine(), because it avoids an extra points->size() -2 projections.
Implements SkyPainter.
Definition at line 396 of file skyqpainter.cpp.
◆ drawSupernova()
|
overridevirtual |
◆ drawTerrain()
|
overridevirtual |
drawTerrain Draw the Terrain
- Parameters
-
useCache if True, try to re-use last generated image instead of rendering a new image.
- Returns
- true if it was drawn
Implements SkyPainter.
Definition at line 859 of file skyqpainter.cpp.
◆ end()
|
overridevirtual |
End and finalize painting.
- Note
- this function must be called after painting anything.
- it is not guaranteed that anything will actually be drawn until end() is called.
- See also
- begin();
Implements SkyPainter.
Definition at line 157 of file skyqpainter.cpp.
◆ getVectorStars()
|
inline |
Definition at line 73 of file skyqpainter.h.
◆ initStarImages()
|
static |
Recalculates the star pixmaps.
Definition at line 263 of file skyqpainter.cpp.
◆ releaseImageCache()
|
static |
Release the image cache.
Definition at line 96 of file skyqpainter.cpp.
◆ setBrush()
|
overridevirtual |
Set the brush of the painter.
Implements SkyPainter.
Definition at line 258 of file skyqpainter.cpp.
◆ setPaintDevice()
|
inline |
Definition at line 58 of file skyqpainter.h.
◆ setPen()
|
overridevirtual |
◆ setSize()
void SkyQPainter::setSize | ( | int | width, |
int | height ) |
Definition at line 142 of file skyqpainter.cpp.
◆ setVectorStars()
|
inline |
- Parameters
-
vectorStars Draw stars as vector graphics whenever possible.
- Note
- Drawing stars as vectors is slower, but is better when saving .svg files. Set to true only when you are drawing on a canvas where speed doesn't matter. Definitely not when drawing on the SkyMap.
Definition at line 69 of file skyqpainter.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 4 2024 16:38:45 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.