Supernova
#include <supernova.h>
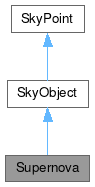
Public Member Functions | |
Supernova (const QString &sName, dms ra, dms dec, const QString &type=QString(), const QString &hostGalaxy=QString(), const QString &date=QString(), float sRedShift=0.0, float sMag=99.9, const QDateTime &discoveryDate=QDateTime::currentDateTime()) | |
Supernova * | clone () const override |
float | getAgeDays () |
QString | getDate () const |
QString | getHostGalaxy () const |
float | getRedShift () const |
QString | getType () const |
void | initPopupMenu (KSPopupMenu *) override |
QString | url () |
![]() | |
SkyObject (int t, double r, double d, float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
SkyObject (int t=TYPE_UNKNOWN, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
virtual | ~SkyObject () override=default |
virtual UID | getUID () const |
bool | hashBeenUpdated () |
bool | hasLongName () const |
bool | hasName () const |
bool | hasName2 () const |
bool | isSolarSystem () const |
virtual double | labelOffset () const |
virtual QString | labelString () const |
virtual QString | longname (void) const |
float | mag () const |
QString | messageFromTitle (const QString &imageTitle) const |
virtual QString | name (void) const |
QString | name2 (void) const |
virtual double | pa () const |
SkyPoint | recomputeCoords (const KStarsDateTime &dt, const GeoLocation *geo=nullptr) const |
SkyPoint | recomputeHorizontalCoords (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | riseSetTime (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
dms | riseSetTimeAz (const KStarsDateTime &dt, const GeoLocation *geo, bool rst) const |
QTime | riseSetTimeUT (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
void | setLongName (const QString &longname=QString()) |
void | setName (const QString &name) |
void | setName2 (const QString &name2=QString()) |
void | setType (int t) |
void | showPopupMenu (KSPopupMenu *pmenu, const QPoint &pos) |
dms | transitAltitude (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTime (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTimeUT (const KStarsDateTime &dt, const GeoLocation *geo) const |
QString | translatedLongName () const |
QString | translatedName () const |
QString | translatedName2 () const |
int | type (void) const |
QString | typeName () const |
![]() | |
SkyPoint () | |
SkyPoint (const CachingDms &r, const CachingDms &d) | |
SkyPoint (const dms &r, const dms &d) | |
SkyPoint (double r, double d) | |
void | aberrate (const KSNumbers *num, bool reverse=false) |
void | addEterms (void) |
double | airmass () const |
const dms & | alt () const |
dms | altRefracted () const |
dms | angularDistanceTo (const SkyPoint *sp, double *const positionAngle=nullptr) const |
void | apparentCoord (long double jd0, long double jdf) |
const dms & | az () const |
void | B1950ToJ2000 (void) |
bool | bendlight () |
SkyPoint | catalogueCoord (long double jdf) |
bool | checkBendLight () |
bool | checkCircumpolar (const dms *gLat) const |
const CachingDms & | dec () const |
const CachingDms & | dec0 () const |
SkyPoint | deprecess (const KSNumbers *num, long double epoch=J2000) |
void | Equatorial1950ToGalactic (dms &galLong, dms &galLat) |
void | EquatorialToHorizontal (const CachingDms *LST, const CachingDms *lat) |
void | EquatorialToHorizontal (const dms *LST, const dms *lat) |
SkyPoint | Eterms (void) |
void | findEcliptic (const CachingDms *Obliquity, dms &EcLong, dms &EcLat) |
void | GalacticToEquatorial1950 (const dms *galLong, const dms *galLat) |
long double | getLastPrecessJD () const |
void | HorizontalToEquatorial (const dms *LST, const dms *lat) |
void | HorizontalToEquatorialICRS (const dms *LST, const dms *lat, const long double jdf) |
void | HorizontalToEquatorialNow () |
bool | isValid () const |
void | J2000ToB1950 (void) |
double | maxAlt (const dms &lat) const |
double | minAlt (const dms &lat) const |
SkyPoint | moveAway (const SkyPoint &from, double dist) const |
void | nutate (const KSNumbers *num, const bool reverse=false) |
bool | operator== (SkyPoint &p) const |
dms | parallacticAngle (const CachingDms &LST, const CachingDms &lat) |
void | precessFromAnyEpoch (long double jd0, long double jdf) |
const CachingDms & | ra () const |
const CachingDms & | ra0 () const |
void | set (const dms &r, const dms &d) |
void | setAlt (dms alt) |
void | setAlt (double alt) |
void | setAltRefracted (dms alt_apparent) |
void | setAltRefracted (double alt_apparent) |
void | setAz (dms az) |
void | setAz (double az) |
void | setDec (const CachingDms &d) |
void | setDec (dms d) |
void | setDec (double d) |
void | setDec0 (const CachingDms &d) |
void | setDec0 (dms d) |
void | setDec0 (double d) |
void | setFromEcliptic (const CachingDms *Obliquity, const dms &EcLong, const dms &EcLat) |
void | setRA (const CachingDms &r) |
void | setRA (dms &r) |
void | setRA (double r) |
void | setRA0 (CachingDms r) |
void | setRA0 (dms r) |
void | setRA0 (double r) |
void | subtractEterms (void) |
virtual void | updateCoords (const KSNumbers *num, bool includePlanets=true, const CachingDms *lat=nullptr, const CachingDms *LST=nullptr, bool forceRecompute=false) |
virtual void | updateCoordsNow (const KSNumbers *num) |
double | vGeocentric (double vhelio, long double jd) |
double | vGeoToVHelio (double vgeo, long double jd) |
double | vHeliocentric (double vlsr, long double jd) |
double | vHelioToVlsr (double vhelio, long double jd) |
double | vREarth (long double jd0) |
double | vRSite (double vsite[3]) |
double | vRSun (long double jd) |
double | vTopocentric (double vgeo, double vsite[3]) |
double | vTopoToVGeo (double vtopo, double vsite[3]) |
Additional Inherited Members | |
![]() | |
enum | TYPE { STAR = 0 , CATALOG_STAR = 1 , PLANET = 2 , OPEN_CLUSTER = 3 , GLOBULAR_CLUSTER = 4 , GASEOUS_NEBULA = 5 , PLANETARY_NEBULA = 6 , SUPERNOVA_REMNANT = 7 , GALAXY = 8 , COMET = 9 , ASTEROID = 10 , CONSTELLATION = 11 , MOON = 12 , ASTERISM = 13 , GALAXY_CLUSTER = 14 , DARK_NEBULA = 15 , QUASAR = 16 , MULT_STAR = 17 , RADIO_SOURCE = 18 , SATELLITE = 19 , SUPERNOVA = 20 , NUMBER_OF_KNOWN_TYPES = 21 , TYPE_UNKNOWN = 255 } |
typedef qint64 | UID |
![]() | |
static QString | typeName (const int t) |
static QString | typeShortName (const int t) |
![]() | |
static dms | findAltitude (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | refract (const dms alt, bool conditional=true) |
static double | refract (const double alt, bool conditional=true) |
static double | refractionCorr (double alt) |
static SkyPoint | timeTransformed (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | unrefract (const dms alt, bool conditional=true) |
static double | unrefract (const double alt, bool conditional=true) |
![]() | |
static const UID | invalidUID = ~0 |
static const UID | UID_DEEPSKY = 2 |
static const UID | UID_GALAXY = 1 |
static const UID | UID_SOLARSYS = 3 |
static const UID | UID_STAR = 0 |
![]() | |
static const double | altCrit = -1.0 |
static bool | implementationIsLibnova = false |
![]() | |
void | setMag (float m) |
![]() | |
void | precess (const KSNumbers *num) |
![]() | |
bool | has_been_updated = true |
QString | LongName |
QString | Name |
QString | Name2 |
![]() | |
long double | lastPrecessJD { 0 } |
Detailed Description
Represents the supernova object.
It is a subclass of the SkyObject class. This class has the information for different supernovae.
N.B. This was modified to use the Open Supernova Project
- Note
- The Data File Contains the following parameters
- sName Designation
- RA Right Ascension
- Dec Declination
- type Supernova Type
- hostGalaxy Host Galaxy for the supernova
- date Discovery date yyyy/mm/dd
- sRedShift Redshift
- sMag Maximum Apparent magnitude
Definition at line 33 of file supernova.h.
Constructor & Destructor Documentation
◆ Supernova()
|
explicit |
Definition at line 16 of file supernova.cpp.
Member Function Documentation
◆ clone()
|
overridevirtual |
- Returns
- a clone of this object
- Note
- See SkyObject::clone()
Reimplemented from SkyObject.
Definition at line 24 of file supernova.cpp.
◆ getAgeDays()
|
inline |
Definition at line 59 of file supernova.h.
◆ getDate()
|
inline |
- Returns
- the date the supernova was observed
Definition at line 54 of file supernova.h.
◆ getHostGalaxy()
|
inline |
- Returns
- the host galaxy of the supernova
Definition at line 51 of file supernova.h.
◆ getRedShift()
|
inline |
- Returns
- the date the supernova was observed
Definition at line 57 of file supernova.h.
◆ getType()
|
inline |
- Returns
- the type of the supernova
Definition at line 48 of file supernova.h.
◆ initPopupMenu()
|
overridevirtual |
Initialize the popup menut.
This function should call correct initialization function in KSPopupMenu. By overloading the function, we don't have to check the object type when we need the menu.
Reimplemented from SkyObject.
Definition at line 30 of file supernova.cpp.
◆ url()
QString Supernova::url | ( | ) |
Definition at line 39 of file supernova.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:41 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.