SkyPoint
#include <skypoint.h>
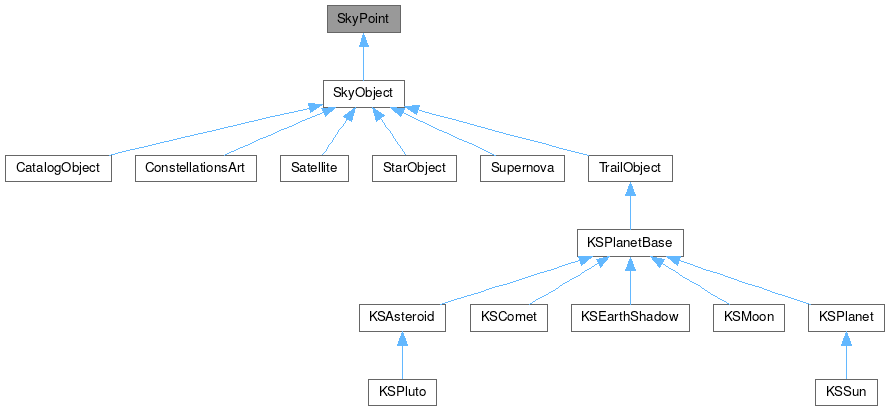
Public Member Functions | |
SkyPoint () | |
SkyPoint (const CachingDms &r, const CachingDms &d) | |
SkyPoint (const dms &r, const dms &d) | |
SkyPoint (double r, double d) | |
void | aberrate (const KSNumbers *num, bool reverse=false) |
void | addEterms (void) |
double | airmass () const |
const dms & | alt () const |
dms | altRefracted () const |
dms | angularDistanceTo (const SkyPoint *sp, double *const positionAngle=nullptr) const |
void | apparentCoord (long double jd0, long double jdf) |
const dms & | az () const |
void | B1950ToJ2000 (void) |
bool | bendlight () |
SkyPoint | catalogueCoord (long double jdf) |
bool | checkBendLight () |
bool | checkCircumpolar (const dms *gLat) const |
const CachingDms & | dec () const |
const CachingDms & | dec0 () const |
SkyPoint | deprecess (const KSNumbers *num, long double epoch=J2000) |
void | Equatorial1950ToGalactic (dms &galLong, dms &galLat) |
void | EquatorialToHorizontal (const CachingDms *LST, const CachingDms *lat) |
void | EquatorialToHorizontal (const dms *LST, const dms *lat) |
SkyPoint | Eterms (void) |
void | findEcliptic (const CachingDms *Obliquity, dms &EcLong, dms &EcLat) |
void | GalacticToEquatorial1950 (const dms *galLong, const dms *galLat) |
long double | getLastPrecessJD () const |
void | HorizontalToEquatorial (const dms *LST, const dms *lat) |
bool | isValid () const |
void | J2000ToB1950 (void) |
double | maxAlt (const dms &lat) const |
double | minAlt (const dms &lat) const |
SkyPoint | moveAway (const SkyPoint &from, double dist) const |
void | nutate (const KSNumbers *num, const bool reverse=false) |
bool | operator== (SkyPoint &p) const |
dms | parallacticAngle (const CachingDms &LST, const CachingDms &lat) |
void | precessFromAnyEpoch (long double jd0, long double jdf) |
const CachingDms & | ra () const |
const CachingDms & | ra0 () const |
void | set (const dms &r, const dms &d) |
void | setAlt (dms alt) |
void | setAlt (double alt) |
void | setAltRefracted (dms alt_apparent) |
void | setAltRefracted (double alt_apparent) |
void | setAz (dms az) |
void | setAz (double az) |
void | setDec (const CachingDms &d) |
void | setDec (dms d) |
void | setDec (double d) |
void | setDec0 (const CachingDms &d) |
void | setDec0 (dms d) |
void | setDec0 (double d) |
void | setFromEcliptic (const CachingDms *Obliquity, const dms &EcLong, const dms &EcLat) |
void | setRA (const CachingDms &r) |
void | setRA (dms &r) |
void | setRA (double r) |
void | setRA0 (CachingDms r) |
void | setRA0 (dms r) |
void | setRA0 (double r) |
void | subtractEterms (void) |
virtual void | updateCoords (const KSNumbers *num, bool includePlanets=true, const CachingDms *lat=nullptr, const CachingDms *LST=nullptr, bool forceRecompute=false) |
virtual void | updateCoordsNow (const KSNumbers *num) |
double | vGeocentric (double vhelio, long double jd) |
double | vGeoToVHelio (double vgeo, long double jd) |
double | vHeliocentric (double vlsr, long double jd) |
double | vHelioToVlsr (double vhelio, long double jd) |
double | vREarth (long double jd0) |
double | vRSite (double vsite[3]) |
double | vRSun (long double jd) |
double | vTopocentric (double vgeo, double vsite[3]) |
double | vTopoToVGeo (double vtopo, double vsite[3]) |
Static Public Member Functions | |
static dms | findAltitude (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | refract (const dms alt, bool conditional=true) |
static double | refract (const double alt, bool conditional=true) |
static double | refractionCorr (double alt) |
static SkyPoint | timeTransformed (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | unrefract (const dms alt, bool conditional=true) |
static double | unrefract (const double alt, bool conditional=true) |
Static Public Attributes | |
static const double | altCrit = -1.0 |
static bool | implementationIsLibnova = false |
Protected Member Functions | |
void | precess (const KSNumbers *num) |
Protected Attributes | |
long double | lastPrecessJD { 0 } |
Detailed Description
The sky coordinates of a point in the sky.
The coordinates are stored in both Equatorial (Right Ascension, Declination) and Horizontal (Azimuth, Altitude) coordinate systems. Provides set/get functions for each coordinate angle, and functions to convert between the Equatorial and Horizon coordinate systems.
Because the coordinate values change slowly over time (due to precession, nutation), the "catalog coordinates" are stored (RA0, Dec0), which were the true coordinates on Jan 1, 2000. The true coordinates (RA, Dec) at any other epoch can be found from the catalog coordinates using updateCoords().
Stores dms coordinates for a point in the sky. for converting between coordinate systems.
- Version
- 1.0
Definition at line 44 of file skypoint.h.
Constructor & Destructor Documentation
◆ SkyPoint() [1/4]
Default constructor: Sets RA, Dec and RA0, Dec0 according to arguments.
Does not set Altitude or Azimuth.
- Parameters
-
r Right Ascension d Declination
Definition at line 54 of file skypoint.h.
◆ SkyPoint() [2/4]
|
inline |
Definition at line 56 of file skypoint.h.
◆ SkyPoint() [3/4]
|
inlineexplicit |
Alternate constructor using double arguments, for convenience.
It behaves essentially like the default constructor.
- Parameters
-
r Right Ascension, expressed as a double d Declination, expressed as a double
- Note
- This also sets RA0 and Dec0
Definition at line 67 of file skypoint.h.
◆ SkyPoint() [4/4]
SkyPoint::SkyPoint | ( | ) |
Default constructor.
Sets nonsense values for RA, Dec etc
Definition at line 53 of file skypoint.cpp.
Member Function Documentation
◆ aberrate()
void SkyPoint::aberrate | ( | const KSNumbers * | num, |
bool | reverse = false ) |
Determine the effects of aberration for this SkyPoint.
- Parameters
-
num pointer to KSNumbers object containing current values of time-dependent variables. reverse bool, if true the aberration is removed.
Definition at line 454 of file skypoint.cpp.
◆ addEterms()
void SkyPoint::addEterms | ( | void | ) |
Coordinates in the FK4 catalog include the effect of aberration due to the ellipticity of the orbit of the Earth.
Coordinates in the FK5 catalog do not include these terms. In order to convert from B1950 (FK4) to actual mean places one has to use this function.
Definition at line 883 of file skypoint.cpp.
◆ airmass()
|
inline |
- Returns
- the airmass of the point. Convenience method.
- Note
- Question: is it better to use alt or refracted alt? Minor difference, probably doesn't matter.
Definition at line 303 of file skypoint.h.
◆ alt()
|
inline |
- Returns
- a pointer to the current Altitude.
Definition at line 281 of file skypoint.h.
◆ altRefracted()
dms SkyPoint::altRefracted | ( | ) | const |
- Returns
- refracted altitude. This function uses Options::useRefraction to determine whether refraction correction should be applied
Definition at line 1050 of file skypoint.cpp.
◆ angularDistanceTo()
dms SkyPoint::angularDistanceTo | ( | const SkyPoint * | sp, |
double *const | positionAngle = nullptr ) const |
Computes the angular distance between two SkyObjects.
The algorithm to compute this distance is: cos(distance) = sin(d1)*sin(d2) + cos(d1)*cos(d2)*cos(a1-a2) where a1,d1 are the coordinates of the first object and a2,d2 are the coordinates of the second object. However this algorithm is not accurate when the angular separation is small. Meeus provides a different algorithm in page 111 which we implement here.
- Parameters
-
sp SkyPoint to which distance is to be calculated positionAngle if a non-null pointer is passed, the position angle [E of N] in degrees from this SkyPoint to sp is computed and stored in the passed variable.
- Returns
- dms angle representing angular separation.
Definition at line 899 of file skypoint.cpp.
◆ apparentCoord()
void SkyPoint::apparentCoord | ( | long double | jd0, |
long double | jdf ) |
Computes the apparent coordinates for this SkyPoint for any epoch, accounting for the effects of precession, nutation, and aberration.
Similar to updateCoords(), but the starting epoch need not be J2000, and the target epoch need not be the present time.
- Parameters
-
jd0 Julian Day which identifies the original epoch jdf Julian Day which identifies the final epoch
Definition at line 700 of file skypoint.cpp.
◆ az()
|
inline |
- Returns
- a pointer to the current Azimuth.
Definition at line 275 of file skypoint.h.
◆ B1950ToJ2000()
void SkyPoint::B1950ToJ2000 | ( | void | ) |
Exact precession from Besselian epoch 1950 to epoch J2000.
The coordinates referred to the first epoch are in the FK4 catalog, while the latter are in the Fk5 one.
Reference: Smith, C. A.; Kaplan, G. H.; Hughes, J. A.; Seidelmann, P. K.; Yallop, B. D.; Hohenkerk, C. Y. Astronomical Journal, vol. 97, Jan. 1989, p. 265-279
This transformation requires 4 steps:
- Correct E-terms
- Precess from B1950 to 1984, January 1st, 0h, using Newcomb expressions
- Add zero point correction in right ascension for 1984
- Precess from 1984, January 1st, 0h to J2000
Definition at line 773 of file skypoint.cpp.
◆ bendlight()
bool SkyPoint::bendlight | ( | ) |
Correct for the effect of "bending" of light around the sun for positions near the sun.
General Relativity tells us that a photon with an impact parameter b is deflected through an angle 1.75" (Rs / b) where Rs is the solar radius.
- Returns
- : true if the light was bent, false otherwise
Definition at line 425 of file skypoint.cpp.
◆ catalogueCoord()
SkyPoint SkyPoint::catalogueCoord | ( | long double | jdf | ) |
Computes the J2000.0 catalogue coordinates for this SkyPoint using the epoch removing aberration, nutation and precession Catalogue coordinates are in Ra0, Dec0 as well as Ra, Dec and lastPrecessJD is set to J2000.0.
- Fixme
- We do not undo nutation and aberration
catalogueCoord converts observed to J2000 using epoch jdf
- Parameters
-
jdf Julian Day which identifies the current epoch
- Returns
- SpyPoint containing J2000 coordinates
Definition at line 710 of file skypoint.cpp.
◆ checkBendLight()
bool SkyPoint::checkBendLight | ( | ) |
Check if this sky point is close enough to the sun for gravitational lensing to be significant.
Definition at line 399 of file skypoint.cpp.
◆ checkCircumpolar()
bool SkyPoint::checkCircumpolar | ( | const dms * | gLat | ) | const |
Check if this point is circumpolar at the given geographic latitude.
Definition at line 1045 of file skypoint.cpp.
◆ dec()
|
inline |
- Returns
- a pointer to the current Declination.
Definition at line 269 of file skypoint.h.
◆ dec0()
|
inline |
- Returns
- a pointer to the catalog Declination.
Definition at line 257 of file skypoint.h.
◆ deprecess()
Obtain a Skypoint with RA0 and Dec0 set from the RA, Dec of this skypoint.
Also set the RA0, Dec0 of this SkyPoint if not set already and the target epoch is J2000.
Definition at line 257 of file skypoint.cpp.
◆ Equatorial1950ToGalactic()
Computes galactic coordinates from equatorial coordinates referred to epoch 1950.
RA and Dec are, therefore assumed to be B1950 coordinates.
Definition at line 735 of file skypoint.cpp.
◆ EquatorialToHorizontal() [1/2]
void SkyPoint::EquatorialToHorizontal | ( | const CachingDms * | LST, |
const CachingDms * | lat ) |
Determine the (Altitude, Azimuth) coordinates of the SkyPoint from its (RA, Dec) coordinates, given the local sidereal time and the observer's latitude.
- Parameters
-
LST pointer to the local sidereal time lat pointer to the geographic latitude
Definition at line 77 of file skypoint.cpp.
◆ EquatorialToHorizontal() [2/2]
Definition at line 70 of file skypoint.cpp.
◆ Eterms()
SkyPoint SkyPoint::Eterms | ( | void | ) |
Determine the E-terms of aberration In the past, the mean places of stars published in catalogs included the contribution to the aberration due to the ellipticity of the orbit of the Earth.
These terms, known as E-terms were almost constant, and in the newer catalogs (FK5) are not included. Therefore to convert from FK4 to FK5 one has to compute these E-terms.
Definition at line 868 of file skypoint.cpp.
◆ findAltitude()
|
static |
Compute the altitude of a given skypoint hour hours from the given date/time.
- Parameters
-
p SkyPoint whose altitude is to be computed (const pointer, the method works on a clone) dt Date/time that corresponds to 0 hour geo GeoLocation object specifying the location hour double specifying offset in hours from dt for which altitude is to be found
- Returns
- a dms containing (unrefracted?) altitude of the object at dt + hour hours at the given location
- Note
- This method is used in multiple places across KStars
Definition at line 1111 of file skypoint.cpp.
◆ findEcliptic()
void SkyPoint::findEcliptic | ( | const CachingDms * | Obliquity, |
dms & | EcLong, | ||
dms & | EcLat ) |
Determine the Ecliptic coordinates of the SkyPoint, given the Julian Date.
The ecliptic coordinates are returned as reference arguments (since they are not stored internally)
Definition at line 187 of file skypoint.cpp.
◆ GalacticToEquatorial1950()
Computes equatorial coordinates referred to 1950 from galactic ones referred to epoch B1950.
RA and Dec are, therefore assumed to be B1950 coordinates.
Definition at line 754 of file skypoint.cpp.
◆ getLastPrecessJD()
|
inline |
- Returns
- the JD for the precessed coordinates
Definition at line 294 of file skypoint.h.
◆ HorizontalToEquatorial()
Determine the (RA, Dec) coordinates of the SkyPoint from its (Altitude, Azimuth) coordinates, given the local sidereal time and the observer's latitude.
- Parameters
-
LST pointer to the local sidereal time lat pointer to the geographic latitude
Definition at line 143 of file skypoint.cpp.
◆ isValid()
|
inline |
isValid Check if the RA and DE fall within expected range
- Returns
- True if valid, false otherwise.
Definition at line 312 of file skypoint.h.
◆ J2000ToB1950()
void SkyPoint::J2000ToB1950 | ( | void | ) |
Exact precession from epoch J2000 Besselian epoch 1950.
The coordinates referred to the first epoch are in the FK4 catalog, while the latter are in the Fk5 one.
Reference: Smith, C. A.; Kaplan, G. H.; Hughes, J. A.; Seidelmann, P. K.; Yallop, B. D.; Hohenkerk, C. Y. Astronomical Journal, vol. 97, Jan. 1989, p. 265-279
This transformation requires 4 steps:
- Precess from J2000 to 1984, January 1st, 0h
- Add zero point correction in right ascension for 1984
- Precess from 1984, January 1st, 0h, to B1950 using Newcomb expressions
- Correct E-terms
Definition at line 819 of file skypoint.cpp.
◆ maxAlt()
double SkyPoint::maxAlt | ( | const dms & | lat | ) | const |
Return the object's altitude at the upper culmination for the given latitude.
- Returns
- the maximum altitude in degrees
Definition at line 1136 of file skypoint.cpp.
◆ minAlt()
double SkyPoint::minAlt | ( | const dms & | lat | ) | const |
Return the object's altitude at the lower culmination for the given latitude.
- Returns
- the minimum altitude in degrees
Definition at line 1144 of file skypoint.cpp.
◆ moveAway()
Find the SkyPoint obtained by moving distance dist (arcseconds) away from the givenSkyPoint.
- Parameters
-
dist Distance to move through in arcseconds from The SkyPoint to move away from
Definition at line 371 of file skypoint.cpp.
◆ nutate()
void SkyPoint::nutate | ( | const KSNumbers * | num, |
const bool | reverse = false ) |
Apply the effects of nutation to this SkyPoint.
- Parameters
-
num pointer to KSNumbers object containing current values of time-dependent variables. reverse bool, if true the nutation is removed
Definition at line 275 of file skypoint.cpp.
◆ operator==()
|
inline |
- Returns
- returns true if current epoch RA / Dec match
Definition at line 551 of file skypoint.h.
◆ parallacticAngle()
dms SkyPoint::parallacticAngle | ( | const CachingDms & | LST, |
const CachingDms & | lat ) |
Return the Parallactic Angle.
The parallactic angle is the angle between "up" and "north". See Jean Meeus' "Astronomical Algorithms" second edition, Chapter 14 for more details (especially Fig 4 on Pg 99). The angle returned in this case, between a vector of increasing altitude and a vector of increasing declination, is measured in the clockwise sense as seen on the sky.
- Parameters
-
LST Local Sidereal Time lat Latitude
- Note
- EquatorialToHorizontal() need not be called before invoking this, but it is wise to call updateCoords() to ensure ra() and dec() refer to the right epoch.
- Returns
- the parallactic angle in the clockwise sense
Definition at line 1152 of file skypoint.cpp.
◆ precess()
|
protected |
Precess this SkyPoint's catalog coordinates to the epoch described by the given KSNumbers object.
- Parameters
-
num pointer to a KSNumbers object describing the target epoch.
Definition at line 223 of file skypoint.cpp.
◆ precessFromAnyEpoch()
void SkyPoint::precessFromAnyEpoch | ( | long double | jd0, |
long double | jdf ) |
General case of precession.
It precess from an original epoch to a final epoch. In this case RA0, and Dec0 from SkyPoint object represent the coordinates for the original epoch and not for J2000, as usual.
- Parameters
-
jd0 Julian Day which identifies the original epoch jdf Julian Day which identifies the final epoch
Definition at line 625 of file skypoint.cpp.
◆ ra()
|
inline |
- Returns
- a pointer to the current Right Ascension.
Definition at line 263 of file skypoint.h.
◆ ra0()
|
inline |
- Returns
- a pointer to the catalog Right Ascension.
Definition at line 251 of file skypoint.h.
◆ refract() [1/2]
Apply refraction correction to altitude.
Overloaded method using dms provided for convenience
- See also
- SkyPoint::refract( const double alt )
Definition at line 686 of file skypoint.h.
◆ refract() [2/2]
|
static |
Apply refraction correction to altitude, depending on conditional.
- Parameters
-
alt altitude to be corrected, in degrees conditional an optional boolean to decide whether to apply the correction or not
- Note
- If conditional is false, this method returns its argument unmodified. This is a convenience feature as it is often needed to gate these corrections.
- Returns
- altitude after refraction correction (if applicable), in degrees
Definition at line 1070 of file skypoint.cpp.
◆ refractionCorr()
|
static |
Calculate refraction correction.
Parameter and return value are in degrees
Definition at line 1065 of file skypoint.cpp.
◆ set()
Sets RA, Dec and RA0, Dec0 according to arguments.
Does not set Altitude or Azimuth.
- Parameters
-
r Right Ascension d Declination
- Note
- This function also sets RA0 and Dec0 to the same values, so call at your own peril!
- FIXME: This method must be removed, or an epoch argument must be added.
Definition at line 63 of file skypoint.cpp.
◆ setAlt() [1/2]
|
inline |
◆ setAlt() [2/2]
|
inline |
Overloaded member function, provided for convenience.
It behaves essentially like the above function.
- Parameters
-
alt Altitude, expressed as a double.
Definition at line 220 of file skypoint.h.
◆ setAltRefracted() [1/2]
void SkyPoint::setAltRefracted | ( | dms | alt_apparent | ) |
Sets the apparent altitude, checking whether refraction corrections are enabled.
- Parameters
-
alt_apparent Apparent altitude (subject to Options::useRefraction())
Definition at line 1055 of file skypoint.cpp.
◆ setAltRefracted() [2/2]
void SkyPoint::setAltRefracted | ( | double | alt_apparent | ) |
Overloaded member function, provided for convenience.
It behaves essentially like the above function.
- Parameters
-
alt_apparent Apparent altitude (subject to Options::useRefraction())
Definition at line 1060 of file skypoint.cpp.
◆ setAz() [1/2]
|
inline |
◆ setAz() [2/2]
|
inline |
Overloaded member function, provided for convenience.
It behaves essentially like the above function.
- Parameters
-
az Azimuth, expressed as a double.
Definition at line 241 of file skypoint.h.
◆ setDec() [1/3]
|
inline |
Definition at line 173 of file skypoint.h.
◆ setDec() [2/3]
|
inline |
Sets Dec, the current Declination.
- Parameters
-
d Declination.
Definition at line 169 of file skypoint.h.
◆ setDec() [3/3]
|
inline |
Overloaded member function, provided for convenience.
It behaves essentially like the above function.
- Parameters
-
d Declination, expressed as a double.
Definition at line 184 of file skypoint.h.
◆ setDec0() [1/3]
|
inline |
Definition at line 123 of file skypoint.h.
◆ setDec0() [2/3]
|
inline |
Sets Dec0, the catalog Declination.
- Parameters
-
d catalog Declination.
Definition at line 119 of file skypoint.h.
◆ setDec0() [3/3]
|
inline |
Overloaded member function, provided for convenience.
It behaves essentially like the above function.
- Parameters
-
d Declination, expressed as a double.
Definition at line 134 of file skypoint.h.
◆ setFromEcliptic()
void SkyPoint::setFromEcliptic | ( | const CachingDms * | Obliquity, |
const dms & | EcLong, | ||
const dms & | EcLat ) |
Set the current (RA, Dec) coordinates of the SkyPoint, given pointers to its Ecliptic (Long, Lat) coordinates, and to the current obliquity angle (the angle between the equator and ecliptic).
Definition at line 201 of file skypoint.cpp.
◆ setRA() [1/3]
|
inline |
Definition at line 148 of file skypoint.h.
◆ setRA() [2/3]
|
inline |
Sets RA, the current Right Ascension.
- Parameters
-
r Right Ascension.
Definition at line 144 of file skypoint.h.
◆ setRA() [3/3]
|
inline |
Overloaded member function, provided for convenience.
It behaves essentially like the above function.
- Parameters
-
r Right Ascension, expressed as a double.
Definition at line 159 of file skypoint.h.
◆ setRA0() [1/3]
|
inline |
Definition at line 98 of file skypoint.h.
◆ setRA0() [2/3]
|
inline |
Sets RA0, the catalog Right Ascension.
- Parameters
-
r catalog Right Ascension.
Definition at line 94 of file skypoint.h.
◆ setRA0() [3/3]
|
inline |
Overloaded member function, provided for convenience.
It behaves essentially like the above function.
- Parameters
-
r Right Ascension, expressed as a double.
Definition at line 109 of file skypoint.h.
◆ subtractEterms()
void SkyPoint::subtractEterms | ( | void | ) |
Coordinates in the FK4 catalog include the effect of aberration due to the ellipticity of the orbit of the Earth.
Coordinates in the FK5 catalog do not include these terms. In order to convert from FK5 coordinates to B1950 (FK4) one has to use this function.
Definition at line 891 of file skypoint.cpp.
◆ timeTransformed()
|
static |
returns a time-transformed SkyPoint.
See SkyPoint::findAltitude() for details
- Todo
- Fix this documentation.
Definition at line 1121 of file skypoint.cpp.
◆ unrefract() [1/2]
Remove refraction correction.
Overloaded method using dms provided for convenience
- See also
- SkyPoint::unrefract( const double alt )
Definition at line 696 of file skypoint.h.
◆ unrefract() [2/2]
|
static |
Remove refraction correction, depending on conditional.
- Parameters
-
alt altitude from which refraction correction must be removed, in degrees conditional an optional boolean to decide whether to undo the correction or not
- Returns
- altitude without refraction correction, in degrees
- Note
- If conditional is false, this method returns its argument unmodified. This is a convenience feature as it is often needed to gate these corrections.
Definition at line 1091 of file skypoint.cpp.
◆ updateCoords()
|
virtual |
Determine the current coordinates (RA, Dec) from the catalog coordinates (RA0, Dec0), accounting for both precession and nutation.
- Parameters
-
num pointer to KSNumbers object containing current values of time-dependent variables. includePlanets does nothing in this implementation (see KSPlanetBase::updateCoords()). lat does nothing in this implementation (see KSPlanetBase::updateCoords()). LST does nothing in this implementation (see KSPlanetBase::updateCoords()). forceRecompute reapplies precession, nutation and aberration even if the time passed since the last computation is not significant.
Reimplemented in KSEarthShadow, KSPlanetBase, and StarObject.
Definition at line 582 of file skypoint.cpp.
◆ updateCoordsNow()
|
inlinevirtual |
updateCoordsNow Shortcut for updateCoords( const KSNumbers *num, false, nullptr, nullptr, true)
- Parameters
-
num pointer to KSNumbers object containing current values of time-dependent variables.
Definition at line 391 of file skypoint.h.
◆ vGeocentric()
double SkyPoint::vGeocentric | ( | double | vhelio, |
long double | jd ) |
Computes the radial velocity of a source referred to the center of the earth from the radial velocity referred to the solar system barycenter.
- Parameters
-
vhelio radial velocity of the source referred to the barycenter of the solar system in km/s jd Epoch expressed as julian day to which the source coordinates refer to.
- Returns
- Radial velocity of the source referred to the center of the Earth in km/s
Definition at line 1015 of file skypoint.cpp.
◆ vGeoToVHelio()
double SkyPoint::vGeoToVHelio | ( | double | vgeo, |
long double | jd ) |
Computes the radial velocity of a source referred to the solar system barycenter from the velocity referred to the center of the earth.
- Parameters
-
vgeo radial velocity of the source referred to the center of the Earth [km/s] jd Epoch expressed as julian day to which the source coordinates refer to.
- Returns
- Radial velocity of the source referred to the solar system barycenter in km/s
Definition at line 1020 of file skypoint.cpp.
◆ vHeliocentric()
double SkyPoint::vHeliocentric | ( | double | vlsr, |
long double | jd ) |
Computes the radial velocity of a source referred to the solar system barycenter from the radial velocity referred to the Local Standard of Rest, aka known as VLSR.
To compute it we need the coordinates of the source the VLSR and the epoch for the source coordinates.
- Parameters
-
vlsr radial velocity of the source referred to the LSR in km/s jd Epoch expressed as julian day to which the source coordinates refer to.
- Returns
- Radial velocity of the source referred to the barycenter of the solar system in km/s
Definition at line 972 of file skypoint.cpp.
◆ vHelioToVlsr()
double SkyPoint::vHelioToVlsr | ( | double | vhelio, |
long double | jd ) |
Computes the radial velocity of a source referred to the Local Standard of Rest, also known as VLSR from the radial velocity referred to the solar system barycenter.
- Parameters
-
vhelio radial velocity of the source referred to the LSR in km/s jd Epoch expressed as julian day to which the source coordinates refer to.
- Returns
- Radial velocity of the source referred to the barycenter of the solar system in km/s
Definition at line 977 of file skypoint.cpp.
◆ vREarth()
double SkyPoint::vREarth | ( | long double | jd0 | ) |
Computes the velocity of any object projected on the direction of the source.
- Parameters
-
jd0 Julian day for which we compute the direction of the source
- Returns
- velocity of the Earth projected on the direction of the source kms-1
Definition at line 982 of file skypoint.cpp.
◆ vRSite()
double SkyPoint::vRSite | ( | double | vsite[3] | ) |
Computes the velocity of any object (observer's site) projected on the direction of the source.
- Parameters
-
vsite velocity of that object in cartesian coordinates
- Returns
- velocity of the object projected on the direction of the source kms-1
Definition at line 1025 of file skypoint.cpp.
◆ vRSun()
double SkyPoint::vRSun | ( | long double | jd | ) |
Computes the velocity of the Sun projected on the direction of the source.
- Parameters
-
jd Epoch expressed as julian day to which the source coordinates refer to.
- Returns
- Radial velocity of the source referred to the barycenter of the solar system in km/s
Definition at line 934 of file skypoint.cpp.
◆ vTopocentric()
double SkyPoint::vTopocentric | ( | double | vgeo, |
double | vsite[3] ) |
Computes the radial velocity of a source referred to the observer site on the surface of the earth from the geocentric velocity and the velocity of the site referred to the center of the Earth.
- Parameters
-
vgeo radial velocity of the source referred to the center of the earth in km/s vsite Velocity at which the observer moves referred to the center of the earth.
- Returns
- Radial velocity of the source referred to the observer's site in km/s
Definition at line 1040 of file skypoint.cpp.
◆ vTopoToVGeo()
double SkyPoint::vTopoToVGeo | ( | double | vtopo, |
double | vsite[3] ) |
Computes the radial velocity of a source referred to the center of the Earth from the radial velocity referred to an observer site on the surface of the earth.
- Parameters
-
vtopo radial velocity of the source referred to the observer's site in km/s vsite Velocity at which the observer moves referred to the center of the earth.
- Returns
- Radial velocity of the source referred the center of the earth in km/s
Definition at line 1035 of file skypoint.cpp.
Member Data Documentation
◆ altCrit
|
static |
Critical height for atmospheric refraction corrections.
Below this, the height formula produces meaningless results and the correction value is just interpolated.
Definition at line 727 of file skypoint.h.
◆ implementationIsLibnova
|
static |
Definition at line 768 of file skypoint.h.
◆ lastPrecessJD
|
protected |
Definition at line 795 of file skypoint.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 20 2024 11:53:02 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.