StarObject
#include <starobject.h>
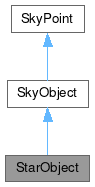
Public Member Functions | |
StarObject (const StarObject &o) | |
StarObject (dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &sptype="--", double pmra=0.0, double pmdec=0.0, double par=0.0, bool mult=false, bool var=false, int hd=0) | |
StarObject (double r, double d, float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &sptype="--", double pmra=0.0, double pmdec=0.0, double par=0.0, bool mult=false, bool var=false, int hd=0) | |
~StarObject () override=default | |
StarObject * | clone () const override |
QString | constell (void) const |
double | distance () const |
float | getBMag () const |
float | getBVIndex () const |
int | getHDIndex () const |
bool | getIndexCoords (const double julianMillenia, CachingDms &ra, CachingDms &dec) const |
bool | getIndexCoords (const double julianMillenia, double *ra, double *dec) const |
bool | getIndexCoords (const KSNumbers *num, CachingDms &ra, CachingDms &dec) const |
bool | getIndexCoords (const KSNumbers *num, double *ra, double *dec) const |
UID | getUID () const override |
float | getVMag () const |
QString | gname (bool useGreekChars=true) const |
QString | greekLetter (bool useGreekChars=true) const |
bool | hasLatinName () const |
bool | hasName () const |
void | init (const DeepStarData *stardata) |
void | init (const StarData *stardata) |
void | initPopupMenu (KSPopupMenu *pmenu) override |
bool | isMultiple () const |
bool | isVariable () const |
void | JITupdate () |
double | labelOffset () const override |
QString | labelString () const override |
QString | longname (void) const override |
QString | name (void) const override |
QString | nameLabel (bool drawName, bool drawMag) const |
double | parallax () const |
double | pmDec () const |
double | pmMagnitude () const |
double | pmMagnitudeSquared () const |
double | pmRA () const |
void | setMultiple (bool m) |
void | setNames (const QString &name, const QString &name2) |
void | setParallax (double plx) |
void | setProperMotion (double pmra, double pmdec) |
void | setVariable (bool v) |
char | spchar () const |
QString | sptype (void) const |
void | updateCoords (const KSNumbers *num, bool includePlanets=true, const CachingDms *lat=nullptr, const CachingDms *LST=nullptr, bool forceRecompute=false) override |
![]() | |
SkyObject (int t, double r, double d, float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
SkyObject (int t=TYPE_UNKNOWN, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
virtual | ~SkyObject () override=default |
bool | hashBeenUpdated () |
bool | hasLongName () const |
bool | hasName () const |
bool | hasName2 () const |
bool | isSolarSystem () const |
float | mag () const |
QString | messageFromTitle (const QString &imageTitle) const |
QString | name2 (void) const |
virtual double | pa () const |
SkyPoint | recomputeCoords (const KStarsDateTime &dt, const GeoLocation *geo=nullptr) const |
SkyPoint | recomputeHorizontalCoords (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | riseSetTime (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
dms | riseSetTimeAz (const KStarsDateTime &dt, const GeoLocation *geo, bool rst) const |
QTime | riseSetTimeUT (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
void | setLongName (const QString &longname=QString()) |
void | setName (const QString &name) |
void | setName2 (const QString &name2=QString()) |
void | setType (int t) |
void | showPopupMenu (KSPopupMenu *pmenu, const QPoint &pos) |
dms | transitAltitude (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTime (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTimeUT (const KStarsDateTime &dt, const GeoLocation *geo) const |
QString | translatedLongName () const |
QString | translatedName () const |
QString | translatedName2 () const |
int | type (void) const |
QString | typeName () const |
![]() | |
SkyPoint () | |
SkyPoint (const CachingDms &r, const CachingDms &d) | |
SkyPoint (const dms &r, const dms &d) | |
SkyPoint (double r, double d) | |
void | aberrate (const KSNumbers *num, bool reverse=false) |
void | addEterms (void) |
double | airmass () const |
const dms & | alt () const |
dms | altRefracted () const |
dms | angularDistanceTo (const SkyPoint *sp, double *const positionAngle=nullptr) const |
void | apparentCoord (long double jd0, long double jdf) |
const dms & | az () const |
void | B1950ToJ2000 (void) |
bool | bendlight () |
SkyPoint | catalogueCoord (long double jdf) |
bool | checkBendLight () |
bool | checkCircumpolar (const dms *gLat) const |
const CachingDms & | dec () const |
const CachingDms & | dec0 () const |
SkyPoint | deprecess (const KSNumbers *num, long double epoch=J2000) |
void | Equatorial1950ToGalactic (dms &galLong, dms &galLat) |
void | EquatorialToHorizontal (const CachingDms *LST, const CachingDms *lat) |
void | EquatorialToHorizontal (const dms *LST, const dms *lat) |
SkyPoint | Eterms (void) |
void | findEcliptic (const CachingDms *Obliquity, dms &EcLong, dms &EcLat) |
void | GalacticToEquatorial1950 (const dms *galLong, const dms *galLat) |
long double | getLastPrecessJD () const |
void | HorizontalToEquatorial (const dms *LST, const dms *lat) |
void | HorizontalToEquatorialICRS (const dms *LST, const dms *lat, const long double jdf) |
void | HorizontalToEquatorialNow () |
bool | isValid () const |
void | J2000ToB1950 (void) |
double | maxAlt (const dms &lat) const |
double | minAlt (const dms &lat) const |
SkyPoint | moveAway (const SkyPoint &from, double dist) const |
void | nutate (const KSNumbers *num, const bool reverse=false) |
bool | operator== (SkyPoint &p) const |
dms | parallacticAngle (const CachingDms &LST, const CachingDms &lat) |
void | precessFromAnyEpoch (long double jd0, long double jdf) |
const CachingDms & | ra () const |
const CachingDms & | ra0 () const |
void | set (const dms &r, const dms &d) |
void | setAlt (dms alt) |
void | setAlt (double alt) |
void | setAltRefracted (dms alt_apparent) |
void | setAltRefracted (double alt_apparent) |
void | setAz (dms az) |
void | setAz (double az) |
void | setDec (const CachingDms &d) |
void | setDec (dms d) |
void | setDec (double d) |
void | setDec0 (const CachingDms &d) |
void | setDec0 (dms d) |
void | setDec0 (double d) |
void | setFromEcliptic (const CachingDms *Obliquity, const dms &EcLong, const dms &EcLat) |
void | setRA (const CachingDms &r) |
void | setRA (dms &r) |
void | setRA (double r) |
void | setRA0 (CachingDms r) |
void | setRA0 (dms r) |
void | setRA0 (double r) |
void | subtractEterms (void) |
virtual void | updateCoordsNow (const KSNumbers *num) |
double | vGeocentric (double vhelio, long double jd) |
double | vGeoToVHelio (double vgeo, long double jd) |
double | vHeliocentric (double vlsr, long double jd) |
double | vHelioToVlsr (double vhelio, long double jd) |
double | vREarth (long double jd0) |
double | vRSite (double vsite[3]) |
double | vRSun (long double jd) |
double | vTopocentric (double vgeo, double vsite[3]) |
double | vTopoToVGeo (double vtopo, double vsite[3]) |
Static Public Member Functions | |
static double | reindexInterval (double pm) |
![]() | |
static QString | typeName (const int t) |
static QString | typeShortName (const int t) |
![]() | |
static dms | findAltitude (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | refract (const dms alt, bool conditional=true) |
static double | refract (const double alt, bool conditional=true) |
static double | refractionCorr (double alt) |
static SkyPoint | timeTransformed (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | unrefract (const dms alt, bool conditional=true) |
static double | unrefract (const double alt, bool conditional=true) |
Public Attributes | |
quint64 | updateID { 0 } |
quint64 | updateNumID { 0 } |
Additional Inherited Members | |
![]() | |
enum | TYPE { STAR = 0 , CATALOG_STAR = 1 , PLANET = 2 , OPEN_CLUSTER = 3 , GLOBULAR_CLUSTER = 4 , GASEOUS_NEBULA = 5 , PLANETARY_NEBULA = 6 , SUPERNOVA_REMNANT = 7 , GALAXY = 8 , COMET = 9 , ASTEROID = 10 , CONSTELLATION = 11 , MOON = 12 , ASTERISM = 13 , GALAXY_CLUSTER = 14 , DARK_NEBULA = 15 , QUASAR = 16 , MULT_STAR = 17 , RADIO_SOURCE = 18 , SATELLITE = 19 , SUPERNOVA = 20 , NUMBER_OF_KNOWN_TYPES = 21 , TYPE_UNKNOWN = 255 } |
typedef qint64 | UID |
![]() | |
static const UID | invalidUID = ~0 |
static const UID | UID_DEEPSKY = 2 |
static const UID | UID_GALAXY = 1 |
static const UID | UID_SOLARSYS = 3 |
static const UID | UID_STAR = 0 |
![]() | |
static const double | altCrit = -1.0 |
static bool | implementationIsLibnova = false |
![]() | |
void | setMag (float m) |
![]() | |
void | precess (const KSNumbers *num) |
![]() | |
bool | has_been_updated = true |
QString | LongName |
QString | Name |
QString | Name2 |
![]() | |
long double | lastPrecessJD { 0 } |
Detailed Description
This is a subclass of SkyObject.
It adds the Spectral type, and flags for variability and multiplicity. For stars, the primary name (n) is the latin name (e.g., "Betelgeuse"). The secondary name (n2) is the genetive name (e.g., "alpha Orionis").
subclass of SkyObject specialized for stars.
- Version
- 1.0
Definition at line 32 of file starobject.h.
Constructor & Destructor Documentation
◆ StarObject() [1/3]
|
explicit |
Constructor.
Sets sky coordinates, magnitude, latin name, genetive name, and spectral type.
- Parameters
-
r Right Ascension d Declination m magnitude n common name n2 genetive name sptype Spectral Type pmra Proper motion in RA direction [mas/yr] pmdec Proper motion in Dec direction [mas/yr] par Parallax angle [mas] mult Multiplicity flag (false=dingle star; true=multiple star) var Variability flag (true if star is a known periodic variable) hd Henry Draper Number
Definition at line 57 of file starobject.cpp.
◆ StarObject() [2/3]
StarObject::StarObject | ( | double | r, |
double | d, | ||
float | m = 0.0, | ||
const QString & | n = QString(), | ||
const QString & | n2 = QString(), | ||
const QString & | sptype = "--", | ||
double | pmra = 0.0, | ||
double | pmdec = 0.0, | ||
double | par = 0.0, | ||
bool | mult = false, | ||
bool | var = false, | ||
int | hd = 0 ) |
Constructor.
Sets sky coordinates, magnitude, latin name, genetive name, and spectral type. Differs from above function only in data type of RA and Dec.
- Parameters
-
r Right Ascension d Declination m magnitude n common name n2 genetive name sptype Spectral Type pmra Proper motion in RA direction [mas/yr] pmdec Proper motion in Dec direction [mas/yr] par Parallax angle [mas] mult Multiplicity flag (false=dingle star; true=multiple star) var Variability flag (true if star is a known periodic variable) hd Henry Draper Number
Definition at line 87 of file starobject.cpp.
◆ StarObject() [3/3]
StarObject::StarObject | ( | const StarObject & | o | ) |
Copy constructor.
Definition at line 117 of file starobject.cpp.
◆ ~StarObject()
|
overridedefault |
Destructor.
(Empty)
Member Function Documentation
◆ clone()
|
overridevirtual |
Create copy of object.
This method is virtual copy constructor. It allows for safe copying of objects. In other words, KSPlanet object stored in SkyObject pointer will be copied as KSPlanet.
Each subclass of SkyObject MUST implement clone method. There is no checking to ensure this, though.
- Returns
- pointer to newly allocated object. Caller takes full responsibility for deallocating it.
Reimplemented from SkyObject.
Definition at line 126 of file starobject.cpp.
◆ constell()
QString StarObject::constell | ( | void | ) | const |
- Returns
- the genitive form of the star's constellation.
Definition at line 614 of file starobject.cpp.
◆ distance()
|
inline |
- Returns
- the star's distance from the Sun in parsecs, as computed from the parallax.
Definition at line 242 of file starobject.h.
◆ getBMag()
|
inline |
- Returns
- the blue magnitude of the star
Definition at line 281 of file starobject.h.
◆ getBVIndex()
|
inline |
- Returns
- the B - V color index of the star, or a nonsense number larger than 30 if it's not well defined
Definition at line 287 of file starobject.h.
◆ getHDIndex()
|
inline |
- Returns
- the star's HD index
Definition at line 254 of file starobject.h.
◆ getIndexCoords() [1/4]
bool StarObject::getIndexCoords | ( | const double | julianMillenia, |
CachingDms & | ra, | ||
CachingDms & | dec ) const |
Fills ra and dec with the coordinates of the star with the proper motion correction but without precision and its friends.
It is used in StarComponent to re-index all the stars.
- Note
- In the Hipparcos catalog, from which most of the proper motion data in KStars is obtained, the RA correction already has the cos(delta) factor incorporated into it. See https://heasarc.gsfc.nasa.gov/W3Browse/all/hipparcos.html
- Returns
- true if we changed the coordinates, false otherwise NOTE: ra and dec both in degrees.
Definition at line 290 of file starobject.cpp.
◆ getIndexCoords() [2/4]
bool StarObject::getIndexCoords | ( | const double | julianMillenia, |
double * | ra, | ||
double * | dec ) const |
Definition at line 409 of file starobject.cpp.
◆ getIndexCoords() [3/4]
|
inline |
Definition at line 188 of file starobject.h.
◆ getIndexCoords() [4/4]
|
inline |
Definition at line 191 of file starobject.h.
◆ getUID()
|
overridevirtual |
◆ getVMag()
|
inline |
- Returns
- the Visual magnitude of the star
Definition at line 278 of file starobject.h.
◆ gname()
QString StarObject::gname | ( | bool | useGreekChars = true | ) | const |
Returns the genetive name of the star.
- Returns
- genetive name of the star
Definition at line 559 of file starobject.cpp.
◆ greekLetter()
QString StarObject::greekLetter | ( | bool | useGreekChars = true | ) | const |
Returns the greek letter portion of the star's genetive name.
Returns empty string if star has no genetive name defined.
- Returns
- greek letter portion of genetive name
Definition at line 567 of file starobject.cpp.
◆ hasLatinName()
|
inline |
- Returns
- true if the star has a latin name ("star" or HD... doesn't count)
Definition at line 123 of file starobject.h.
◆ hasName()
|
inline |
- Returns
- true if the star has a name ("star" doesn't count)
Definition at line 120 of file starobject.h.
◆ init() [1/2]
void StarObject::init | ( | const DeepStarData * | stardata | ) |
Initializes a StarObject to given data.
- Parameters
-
stardata Pointer to deepStarData object containing the available data
- Returns
- Nothing
Definition at line 184 of file starobject.cpp.
◆ init() [2/2]
void StarObject::init | ( | const StarData * | stardata | ) |
Initializes a StarObject to given data.
This is almost like the StarObject constructor itself, but it avoids setting up name, gname etc for unnamed stars. If called instead of the constructor, this method will be much faster for unnamed stars
- Parameters
-
stardata Pointer to starData object containing required data (except name and gname)
- Returns
- Nothing
Definition at line 133 of file starobject.cpp.
◆ initPopupMenu()
|
overridevirtual |
Initialize the popup menut.
This function should call correct initialization function in KSPopupMenu. By overloading the function, we don't have to check the object type when we need the menu.
Reimplemented from SkyObject.
Definition at line 249 of file starobject.cpp.
◆ isMultiple()
|
inline |
- Returns
- whether the star is a binary or multiple starobject
Definition at line 251 of file starobject.h.
◆ isVariable()
|
inline |
- Returns
- whether the star is a binary or multiple starobject
Definition at line 264 of file starobject.h.
◆ JITupdate()
void StarObject::JITupdate | ( | ) |
added for JIT updates from both StarComponent and ConstellationLines
Definition at line 526 of file starobject.cpp.
◆ labelOffset()
|
overridevirtual |
- Returns
- the pixel distance for offseting the star's name label This takes the zoom level and the star's brightness into account.
Reimplemented from SkyObject.
Definition at line 656 of file starobject.cpp.
◆ labelString()
|
overridevirtual |
- Returns
- the string used to label the object on the map In the default implementation, this just returns translatedName() Overridden by StarObject.
Reimplemented from SkyObject.
Definition at line 651 of file starobject.cpp.
◆ longname()
|
inlineoverridevirtual |
If star is unnamed return "star" otherwise return the longname.
Reimplemented from SkyObject.
Definition at line 133 of file starobject.h.
◆ name()
|
inlineoverridevirtual |
If star is unnamed return "star" otherwise return the name.
Reimplemented from SkyObject.
Definition at line 130 of file starobject.h.
◆ nameLabel()
QString StarObject::nameLabel | ( | bool | drawName, |
bool | drawMag ) const |
returns the name, the magnitude or both.
Definition at line 626 of file starobject.cpp.
◆ parallax()
|
inline |
- Returns
- the star's parallax angle, in milliarcsec
Definition at line 239 of file starobject.h.
◆ pmDec()
|
inline |
- Returns
- the Dec component of the star's proper motion, in mas/yr
Definition at line 233 of file starobject.h.
◆ pmMagnitude()
|
inline |
returns the magnitude of the proper motion correction in milliarcsec/year
Definition at line 199 of file starobject.h.
◆ pmMagnitudeSquared()
|
inline |
returns the square of the magnitude of the proper motion correction in (milliarcsec/year)^2
- Note
- In the Hipparcos catalog, from which most of the proper motion data in KStars is obtained, the RA correction already has the cos(delta) factor incorporated into it. See https://heasarc.gsfc.nasa.gov/W3Browse/all/hipparcos.html
- This method is faster when the square root need not be taken
Definition at line 212 of file starobject.h.
◆ pmRA()
|
inline |
- Returns
- the RA component of the star's proper motion, in mas/yr (multiplied by cos(dec))
Definition at line 230 of file starobject.h.
◆ reindexInterval()
|
static |
returns the reindex interval (in centuries!) for the given magnitude of proper motion (in milliarcsec/year).
ASSUMING a 25 arc-minute margin for proper motion.
Definition at line 46 of file starobject.cpp.
◆ setMultiple()
|
inline |
set the star's multiplicity flag (i.e., is it a binary or multiple star?)
- Parameters
-
m true if binary/multiple star system
Definition at line 248 of file starobject.h.
◆ setNames()
Sets the name, genetive name, and long name.
- Parameters
-
name Common name name2 Genetive name
Definition at line 231 of file starobject.cpp.
◆ setParallax()
|
inline |
set the star's parallax angle, in milliarcsec
Definition at line 236 of file starobject.h.
◆ setProperMotion()
|
inline |
Set the Ra and Dec components of the star's proper motion, in milliarcsec/year.
Note that the RA component should already have been multiplied by cos(dec).
- Parameters
-
pmra the new RA proper motion pmdec the new Dec proper motion
Definition at line 223 of file starobject.h.
◆ setVariable()
|
inline |
set the star's variability flag
- Parameters
-
v true if star is variable
Definition at line 261 of file starobject.h.
◆ spchar()
char StarObject::spchar | ( | ) | const |
Returns just the first character of the spectral type string.
Definition at line 554 of file starobject.cpp.
◆ sptype()
QString StarObject::sptype | ( | void | ) | const |
Returns entire spectral type string.
- Returns
- Spectral Type string
Definition at line 549 of file starobject.cpp.
◆ updateCoords()
|
overridevirtual |
Determine the current coordinates (RA, Dec) from the catalog coordinates (RA0, Dec0), accounting for both precession and nutation.
- Parameters
-
num pointer to KSNumbers object containing current values of time-dependent variables. includePlanets does nothing in this implementation (see KSPlanetBase::updateCoords()). lat does nothing in this implementation (see KSPlanetBase::updateCoords()). LST does nothing in this implementation (see KSPlanetBase::updateCoords()). forceRecompute defines whether the data should be recomputed forcefully.
Reimplemented from SkyPoint.
Definition at line 258 of file starobject.cpp.
Member Data Documentation
◆ updateID
quint64 StarObject::updateID { 0 } |
Definition at line 291 of file starobject.h.
◆ updateNumID
quint64 StarObject::updateNumID { 0 } |
Definition at line 292 of file starobject.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:41 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.