KSPlanet
#include <ksplanet.h>
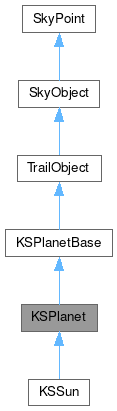
Classes | |
class | OrbitData |
class | OrbitDataColl |
class | OrbitDataManager |
Public Member Functions | |
KSPlanet (const QString &s="unnamed", const QString &image_file=QString(), const QColor &c=Qt::white, double pSize=0) | |
KSPlanet (int n) | |
virtual void | calcEcliptic (double jm, EclipticPosition &ret) const |
KSPlanet * | clone () const override |
SkyObject::UID | getUID () const override |
bool | loadData () override |
QString | untranslatedName () const |
![]() | |
KSPlanetBase (const QString &s=i18n("unnamed"), const QString &image_file=QString(), const QColor &c=Qt::white, double pSize=0) | |
~KSPlanetBase () override=default | |
double | angSize () const |
QColor & | color () |
const dms & | ecLat () const |
void | EclipticToEquatorial (const CachingDms *Obliquity) |
const dms & | ecLong () const |
void | EquatorialToEcliptic (const CachingDms *Obliquity) |
void | findPosition (const KSNumbers *num, const CachingDms *lat=nullptr, const CachingDms *LST=nullptr, const KSPlanetBase *Earth=nullptr) |
const dms & | helEcLat () const |
const dms & | helEcLong () const |
const QImage & | image () const |
void | init (const QString &s, const QString &image_file, const QColor &c, double pSize) |
bool | isMajorPlanet () const |
double | labelOffset () const override |
double | pa () const override |
dms | phase () |
double | physicalSize () const |
double | rearth () const |
double | rsun () const |
void | setAngularSize (double size) |
void | setColor (const QColor &c) |
void | setEcLat (dms elat) |
void | setEcLong (dms elong) |
void | setPA (double p) |
void | setPhysicalSize (double size) |
void | setRearth (const KSPlanetBase *Earth) |
void | setRearth (double r) |
void | setRsun (double r) |
void | updateCoords (const KSNumbers *num, bool includePlanets=true, const CachingDms *lat=nullptr, const CachingDms *LST=nullptr, bool forceRecompute=false) override |
![]() | |
TrailObject (int t, double r, double d, float m=0.0, const QString &n=QString()) | |
TrailObject (int t=TYPE_UNKNOWN, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString()) | |
void | addToTrail (const QString &label=QString()) |
void | clearTrail () |
void | clipTrail () |
TrailObject * | clone () const override |
void | drawTrail (SkyPainter *skyp) const |
bool | hasTrail () const |
void | initPopupMenu (KSPopupMenu *pmenu) override |
const QList< SkyPoint > & | trail () const |
void | updateTrail (dms *LST, const dms *lat) |
![]() | |
SkyObject (int t, double r, double d, float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
SkyObject (int t=TYPE_UNKNOWN, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
virtual | ~SkyObject () override=default |
bool | hashBeenUpdated () |
bool | hasLongName () const |
bool | hasName () const |
bool | hasName2 () const |
bool | isSolarSystem () const |
virtual QString | labelString () const |
virtual QString | longname (void) const |
float | mag () const |
QString | messageFromTitle (const QString &imageTitle) const |
virtual QString | name (void) const |
QString | name2 (void) const |
SkyPoint | recomputeCoords (const KStarsDateTime &dt, const GeoLocation *geo=nullptr) const |
SkyPoint | recomputeHorizontalCoords (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | riseSetTime (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
dms | riseSetTimeAz (const KStarsDateTime &dt, const GeoLocation *geo, bool rst) const |
QTime | riseSetTimeUT (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
void | setLongName (const QString &longname=QString()) |
void | setName (const QString &name) |
void | setName2 (const QString &name2=QString()) |
void | setType (int t) |
void | showPopupMenu (KSPopupMenu *pmenu, const QPoint &pos) |
dms | transitAltitude (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTime (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTimeUT (const KStarsDateTime &dt, const GeoLocation *geo) const |
QString | translatedLongName () const |
QString | translatedName () const |
QString | translatedName2 () const |
int | type (void) const |
QString | typeName () const |
![]() | |
SkyPoint () | |
SkyPoint (const CachingDms &r, const CachingDms &d) | |
SkyPoint (const dms &r, const dms &d) | |
SkyPoint (double r, double d) | |
void | aberrate (const KSNumbers *num, bool reverse=false) |
void | addEterms (void) |
double | airmass () const |
const dms & | alt () const |
dms | altRefracted () const |
dms | angularDistanceTo (const SkyPoint *sp, double *const positionAngle=nullptr) const |
void | apparentCoord (long double jd0, long double jdf) |
const dms & | az () const |
void | B1950ToJ2000 (void) |
bool | bendlight () |
SkyPoint | catalogueCoord (long double jdf) |
bool | checkBendLight () |
bool | checkCircumpolar (const dms *gLat) const |
const CachingDms & | dec () const |
const CachingDms & | dec0 () const |
SkyPoint | deprecess (const KSNumbers *num, long double epoch=J2000) |
void | Equatorial1950ToGalactic (dms &galLong, dms &galLat) |
void | EquatorialToHorizontal (const CachingDms *LST, const CachingDms *lat) |
void | EquatorialToHorizontal (const dms *LST, const dms *lat) |
SkyPoint | Eterms (void) |
void | findEcliptic (const CachingDms *Obliquity, dms &EcLong, dms &EcLat) |
void | GalacticToEquatorial1950 (const dms *galLong, const dms *galLat) |
long double | getLastPrecessJD () const |
void | HorizontalToEquatorial (const dms *LST, const dms *lat) |
bool | isValid () const |
void | J2000ToB1950 (void) |
double | maxAlt (const dms &lat) const |
double | minAlt (const dms &lat) const |
SkyPoint | moveAway (const SkyPoint &from, double dist) const |
void | nutate (const KSNumbers *num, const bool reverse=false) |
bool | operator== (SkyPoint &p) const |
dms | parallacticAngle (const CachingDms &LST, const CachingDms &lat) |
void | precessFromAnyEpoch (long double jd0, long double jdf) |
const CachingDms & | ra () const |
const CachingDms & | ra0 () const |
void | set (const dms &r, const dms &d) |
void | setAlt (dms alt) |
void | setAlt (double alt) |
void | setAltRefracted (dms alt_apparent) |
void | setAltRefracted (double alt_apparent) |
void | setAz (dms az) |
void | setAz (double az) |
void | setDec (const CachingDms &d) |
void | setDec (dms d) |
void | setDec (double d) |
void | setDec0 (const CachingDms &d) |
void | setDec0 (dms d) |
void | setDec0 (double d) |
void | setFromEcliptic (const CachingDms *Obliquity, const dms &EcLong, const dms &EcLat) |
void | setRA (const CachingDms &r) |
void | setRA (dms &r) |
void | setRA (double r) |
void | setRA0 (CachingDms r) |
void | setRA0 (dms r) |
void | setRA0 (double r) |
void | subtractEterms (void) |
virtual void | updateCoordsNow (const KSNumbers *num) |
double | vGeocentric (double vhelio, long double jd) |
double | vGeoToVHelio (double vgeo, long double jd) |
double | vHeliocentric (double vlsr, long double jd) |
double | vHelioToVlsr (double vhelio, long double jd) |
double | vREarth (long double jd0) |
double | vRSite (double vsite[3]) |
double | vRSun (long double jd) |
double | vTopocentric (double vgeo, double vsite[3]) |
double | vTopoToVGeo (double vtopo, double vsite[3]) |
Protected Types | |
typedef QVector< OrbitData > | OBArray[6] |
Protected Member Functions | |
bool | findGeocentricPosition (const KSNumbers *num, const KSPlanetBase *Earth=nullptr) override |
![]() | |
virtual double | findAngularSize () |
void | findPA (const KSNumbers *num) |
virtual void | findPhase () |
UID | solarsysUID (UID type) const |
![]() | |
void | setMag (float m) |
![]() | |
void | precess (const KSNumbers *num) |
Protected Attributes | |
bool | data_loaded { false } |
![]() | |
EclipticPosition | ep |
EclipticPosition | helEcPos |
QImage | m_image |
double | Phase {NaN::d} |
double | Rearth {NaN::d} |
![]() | |
QList< QString > | m_TrailLabels |
QList< SkyPoint > | Trail |
![]() | |
bool | has_been_updated = true |
QString | LongName |
QString | Name |
QString | Name2 |
![]() | |
long double | lastPrecessJD { 0 } |
Static Protected Attributes | |
static OrbitDataManager | odm |
![]() | |
static const UID | UID_SOL_ASTEROID = 1 |
static const UID | UID_SOL_BIGOBJ = 0 |
static const UID | UID_SOL_COMET = 2 |
![]() | |
static QSet< TrailObject * > | trailObjects |
Additional Inherited Members | |
![]() | |
enum | Planets { MERCURY = 0 , VENUS = 1 , MARS = 2 , JUPITER = 3 , SATURN = 4 , URANUS = 5 , NEPTUNE = 6 , SUN = 7 , MOON = 8 , EARTH_SHADOW = 9 , UNKNOWN_PLANET } |
![]() | |
enum | TYPE { STAR = 0 , CATALOG_STAR = 1 , PLANET = 2 , OPEN_CLUSTER = 3 , GLOBULAR_CLUSTER = 4 , GASEOUS_NEBULA = 5 , PLANETARY_NEBULA = 6 , SUPERNOVA_REMNANT = 7 , GALAXY = 8 , COMET = 9 , ASTEROID = 10 , CONSTELLATION = 11 , MOON = 12 , ASTERISM = 13 , GALAXY_CLUSTER = 14 , DARK_NEBULA = 15 , QUASAR = 16 , MULT_STAR = 17 , RADIO_SOURCE = 18 , SATELLITE = 19 , SUPERNOVA = 20 , NUMBER_OF_KNOWN_TYPES = 21 , TYPE_UNKNOWN = 255 } |
typedef qint64 | UID |
![]() | |
static KSPlanetBase * | createPlanet (int n) |
![]() | |
static void | clearTrailsExcept (SkyObject *o) |
![]() | |
static QString | typeName (const int t) |
static QString | typeShortName (const int t) |
![]() | |
static dms | findAltitude (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | refract (const dms alt, bool conditional=true) |
static double | refract (const double alt, bool conditional=true) |
static double | refractionCorr (double alt) |
static SkyPoint | timeTransformed (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | unrefract (const dms alt, bool conditional=true) |
static double | unrefract (const double alt, bool conditional=true) |
![]() | |
static QVector< QColor > | planetColor |
![]() | |
static const int | MaxTrail = 400 |
![]() | |
static const UID | invalidUID = ~0 |
static const UID | UID_DEEPSKY = 2 |
static const UID | UID_GALAXY = 1 |
static const UID | UID_SOLARSYS = 3 |
static const UID | UID_STAR = 0 |
![]() | |
static const double | altCrit = -1.0 |
static bool | implementationIsLibnova = false |
Detailed Description
A subclass of KSPlanetBase for seven of the major planets in the solar system (Earth and Pluto have their own specialized classes derived from KSPlanetBase).
- Note
- The Sun is subclassed from KSPlanet.
KSPlanet contains internal classes to manage the computations of a planet's position. The position is computed as a series of sinusoidal sums, similar to a Fourier transform. See "Astronomical Algorithms" by Jean Meeus or the file README.planetmath for details.
Provides necessary information about objects in the solar system.
- Version
- 1.0
Definition at line 32 of file ksplanet.h.
Member Typedef Documentation
◆ OBArray
Definition at line 110 of file ksplanet.h.
Constructor & Destructor Documentation
◆ KSPlanet() [1/2]
|
explicit |
Constructor.
- Parameters
-
s Name of planet image_file filename of the planet's image c the color for the planet pSize physical diameter of the planet, in km
Definition at line 114 of file ksplanet.cpp.
◆ KSPlanet() [2/2]
|
explicit |
Simplified constructor.
- Parameters
-
n identifier of the planet to be created
- See also
- PLANET enum
Definition at line 119 of file ksplanet.cpp.
Member Function Documentation
◆ calcEcliptic()
|
virtual |
Calculate the ecliptic longitude and latitude of the planet for the given date (expressed in Julian Millenia since J2000).
A reference to the ecliptic coordinates is returned as the second object.
- Parameters
-
jm Julian Millenia (=jd/1000) ret The ecliptic coordinates are returned by reference through this argument.
Definition at line 192 of file ksplanet.cpp.
◆ clone()
|
overridevirtual |
Create copy of object.
This method is virtual copy constructor. It allows for safe copying of objects. In other words, KSPlanet object stored in SkyObject pointer will be copied as KSPlanet.
Each subclass of SkyObject MUST implement clone method. There is no checking to ensure this, though.
- Returns
- pointer to newly allocated object. Caller takes full responsibility for deallocating it.
Reimplemented from SkyObject.
Reimplemented in KSSun.
Definition at line 150 of file ksplanet.cpp.
◆ findGeocentricPosition()
|
overrideprotectedvirtual |
Calculate the geocentric RA, Dec coordinates of the Planet.
- Note
- reimplemented from KSPlanetBase
- Parameters
-
num pointer to object with time-dependent values for the desired date Earth pointer to the planet Earth (needed to calculate geocentric coords)
- Returns
- true if position was successfully calculated.
Implements KSPlanetBase.
Reimplemented in KSSun.
Definition at line 265 of file ksplanet.cpp.
◆ getUID()
|
overridevirtual |
◆ loadData()
|
overridevirtual |
Preload the data used by findPosition.
Implements KSPlanetBase.
Reimplemented in KSSun.
Definition at line 186 of file ksplanet.cpp.
◆ untranslatedName()
QString KSPlanet::untranslatedName | ( | ) | const |
return the untranslated name This is a dirty way to solve a lot of localization-related trouble for the KDE 4.2 release TODO: Change the whole architecture for names later
Definition at line 157 of file ksplanet.cpp.
Member Data Documentation
◆ data_loaded
|
protected |
Definition at line 173 of file ksplanet.h.
◆ odm
|
staticprotected |
Definition at line 174 of file ksplanet.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:47:16 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.