KStarsDateTime
#include <kstarsdatetime.h>
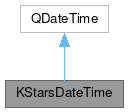
Public Types | |
enum | EpochType { JULIAN , BESSELIAN } |
![]() | |
enum | YearRange |
Public Member Functions | |
KStarsDateTime () | |
KStarsDateTime (const QDate &_d, const QTime &_t, Qt::TimeSpec timeSpec=Qt::UTC) | |
KStarsDateTime (const QDateTime &qdt) | |
KStarsDateTime (long double djd) | |
KStarsDateTime | addDays (int nd) const |
KStarsDateTime | addSecs (double s) const |
long double | djd () const |
double | epoch () const |
dms | gst () const |
QTime | GSTtoUT (dms GST) const |
bool | operator!= (const KStarsDateTime &d) const |
bool | operator< (const KStarsDateTime &d) const |
bool | operator<= (const KStarsDateTime &d) const |
bool | operator== (const KStarsDateTime &d) const |
bool | operator> (const KStarsDateTime &d) const |
bool | operator>= (const KStarsDateTime &d) const |
void | setDate (const QDate &d) |
void | setDJD (long double jd) |
bool | setFromEpoch (const QString &e) |
void | setFromEpoch (double e) |
bool | setFromEpoch (double e, EpochType type) |
void | setTime (const QTime &t) |
KStarsDateTime (const KStarsDateTime &kdt) | |
KStarsDateTime & | operator= (const KStarsDateTime &kdt) noexcept |
![]() | |
QDateTime (const QDateTime &other) | |
QDateTime (QDate date, QTime time) | |
QDateTime (QDate date, QTime time, const QTimeZone &timeZone) | |
QDateTime (QDate date, QTime time, Qt::TimeSpec spec, int offsetSeconds) | |
QDateTime (QDateTime &&other) | |
QDateTime | addDays (qint64 ndays) const const |
QDateTime | addDuration (std::chrono::milliseconds msecs) const const |
QDateTime | addMonths (int nmonths) const const |
QDateTime | addMSecs (qint64 msecs) const const |
QDateTime | addSecs (qint64 s) const const |
QDateTime | addYears (int nyears) const const |
QDate | date () const const |
qint64 | daysTo (const QDateTime &other) const const |
bool | isDaylightTime () const const |
bool | isNull () const const |
bool | isValid () const const |
qint64 | msecsTo (const QDateTime &other) const const |
int | offsetFromUtc () const const |
bool | operator!= (const QDateTime &lhs, const QDateTime &rhs) |
QDateTime | operator+ (const QDateTime &dateTime, std::chrono::milliseconds duration) |
QDateTime | operator+ (std::chrono::milliseconds duration, const QDateTime &dateTime) |
QDateTime & | operator+= (std::chrono::milliseconds duration) |
QDateTime | operator- (const QDateTime &dateTime, std::chrono::milliseconds duration) |
std::chrono::milliseconds | operator- (const QDateTime &lhs, const QDateTime &rhs) |
QDateTime & | operator-= (std::chrono::milliseconds duration) |
bool | operator< (const QDateTime &lhs, const QDateTime &rhs) |
QDataStream & | operator<< (QDataStream &out, const QDateTime &dateTime) |
bool | operator<= (const QDateTime &lhs, const QDateTime &rhs) |
QDateTime & | operator= (const QDateTime &other) |
bool | operator== (const QDateTime &lhs, const QDateTime &rhs) |
bool | operator> (const QDateTime &lhs, const QDateTime &rhs) |
bool | operator>= (const QDateTime &lhs, const QDateTime &rhs) |
QDataStream & | operator>> (QDataStream &in, QDateTime &dateTime) |
qint64 | secsTo (const QDateTime &other) const const |
void | setDate (QDate date) |
void | setMSecsSinceEpoch (qint64 msecs) |
void | setOffsetFromUtc (int offsetSeconds) |
void | setSecsSinceEpoch (qint64 secs) |
void | setTime (QTime time) |
void | setTimeSpec (Qt::TimeSpec spec) |
void | setTimeZone (const QTimeZone &toZone) |
void | swap (QDateTime &other) |
QTime | time () const const |
QTimeZone | timeRepresentation () const const |
Qt::TimeSpec | timeSpec () const const |
QTimeZone | timeZone () const const |
QString | timeZoneAbbreviation () const const |
CFDateRef | toCFDate () const const |
QDateTime | toLocalTime () const const |
qint64 | toMSecsSinceEpoch () const const |
NSDate * | toNSDate () const const |
QDateTime | toOffsetFromUtc (int offsetSeconds) const const |
qint64 | toSecsSinceEpoch () const const |
std::chrono::sys_time< std::chrono::milliseconds > | toStdSysMilliseconds () const const |
std::chrono::sys_seconds | toStdSysSeconds () const const |
QString | toString (const QString &format, QCalendar cal) const const |
QString | toString (QStringView format, QCalendar cal) const const |
QString | toString (Qt::DateFormat format) const const |
QDateTime | toTimeSpec (Qt::TimeSpec spec) const const |
QDateTime | toTimeZone (const QTimeZone &timeZone) const const |
QDateTime | toUTC () const const |
Static Public Member Functions | |
static KStarsDateTime | currentDateTime () |
static KStarsDateTime | currentDateTimeUtc () |
static long double | epochToJd (double epoch, EpochType type=JULIAN) |
static KStarsDateTime | fromString (const QString &s) |
static double | jdToEpoch (long double jd, EpochType type=JULIAN) |
static double | stringToEpoch (const QString &eName, bool &ok) |
![]() | |
QDateTime | currentDateTime () |
QDateTime | currentDateTime (const QTimeZone &zone) |
QDateTime | currentDateTimeUtc () |
qint64 | currentMSecsSinceEpoch () |
qint64 | currentSecsSinceEpoch () |
QDateTime | fromCFDate (CFDateRef date) |
QDateTime | fromMSecsSinceEpoch (qint64 msecs) |
QDateTime | fromMSecsSinceEpoch (qint64 msecs, const QTimeZone &timeZone) |
QDateTime | fromMSecsSinceEpoch (qint64 msecs, Qt::TimeSpec spec, int offsetSeconds) |
QDateTime | fromNSDate (const NSDate *date) |
QDateTime | fromSecsSinceEpoch (qint64 secs) |
QDateTime | fromSecsSinceEpoch (qint64 secs, const QTimeZone &timeZone) |
QDateTime | fromSecsSinceEpoch (qint64 secs, Qt::TimeSpec spec, int offsetSeconds) |
QDateTime | fromStdLocalTime (const std::chrono::local_time< std::chrono::milliseconds > &time) |
QDateTime | fromStdTimePoint (const std::chrono::local_time< std::chrono::milliseconds > &time) |
QDateTime | fromStdTimePoint (const std::chrono::time_point< Clock, Duration > &time) |
QDateTime | fromStdZonedTime (const std::chrono::zoned_time< std::chrono::milliseconds, const std::chrono::time_zone * > &time) |
QDateTime | fromString (const QString &string, const QString &format, QCalendar cal) |
QDateTime | fromString (const QString &string, QStringView format, QCalendar cal) |
QDateTime | fromString (const QString &string, Qt::DateFormat format) |
QDateTime | fromString (QStringView string, QStringView format, QCalendar cal) |
QDateTime | fromString (QStringView string, Qt::DateFormat format) |
Static Public Attributes | |
static constexpr const double | B1900 = 2415020.31352 |
static constexpr const double | JD_PER_BYEAR = 365.242198781 |
Additional Inherited Members | |
![]() | |
First | |
Last | |
Detailed Description
Extension of QDateTime for KStars KStarsDateTime can represent the date/time as a Julian Day, using a long double, in which the fractional portion encodes the time of day to a precision of a less than a second.
Also adds Greenwich Sidereal Time and "epoch", which is just the date expressed as a floating point number representing the year, with the fractional part representing the date and time (with poor time resolution; typically the Epoch is only taken to the hundredths place, which is a few days).
- Note
- Local time and Local sideral time are not handled here. Because they depend on the geographic location, they are part of the GeoLocation class.
- The default timespec is UTC unless the passed value has different timespec value.
- See also
- GeoLocation::GSTtoLST()
- GeoLocation::UTtoLT()
- Version
- 1.1
Definition at line 35 of file kstarsdatetime.h.
Member Enumeration Documentation
◆ EpochType
description options
- Note
- After 1976, the IAU standard for epochs is Julian Years.
Enumerator | |
---|---|
JULIAN | Julian epoch (see http://scienceworld.wolfram.com/astronomy/JulianEpoch.html) |
BESSELIAN | Besselian epoch (see http://scienceworld.wolfram.com/astronomy/BesselianEpoch.html) |
Definition at line 185 of file kstarsdatetime.h.
Constructor & Destructor Documentation
◆ KStarsDateTime() [1/5]
KStarsDateTime::KStarsDateTime | ( | ) |
Default constructor Creates a date/time at J2000 (noon on Jan 1, 200)
- Note
- This sets the timespec to UTC.
Definition at line 16 of file kstarsdatetime.cpp.
◆ KStarsDateTime() [2/5]
|
explicit |
Constructor Creates a date/time at the specified Julian Day.
jd
The Julian Day
- Note
- This sets the timespec to UTC.
Definition at line 66 of file kstarsdatetime.cpp.
◆ KStarsDateTime() [3/5]
KStarsDateTime::KStarsDateTime | ( | const KStarsDateTime & | kdt | ) |
Copy constructor kdt
The KStarsDateTime object to copy.
- Note
- The timespec is copied from kdt.
Definition at line 22 of file kstarsdatetime.cpp.
◆ KStarsDateTime() [4/5]
|
explicit |
Copy constructor qdt
The QDateTime object to copy.
- Note
- The timespec is copied from qdt.
Definition at line 46 of file kstarsdatetime.cpp.
◆ KStarsDateTime() [5/5]
KStarsDateTime::KStarsDateTime | ( | const QDate & | _d, |
const QTime & | _t, | ||
Qt::TimeSpec | timeSpec = Qt::UTC ) |
Constructor Create a KStarsDateTimne based on the specified Date and Time.
_d
The QDate to assign _t
The QTime to assign timespec
The desired timespec, UTC by default.
Definition at line 57 of file kstarsdatetime.cpp.
Member Function Documentation
◆ addDays()
|
inline |
Modify the Date/Time by adding a number of days.
nd
the number of days to add. The number can be negative.
Definition at line 110 of file kstarsdatetime.h.
◆ addSecs()
KStarsDateTime KStarsDateTime::addSecs | ( | double | s | ) | const |
- Returns
- a KStarsDateTime that is the given number of seconds later than this KStarsDateTime.
s
the number of seconds to add. The number can be negative.
Definition at line 153 of file kstarsdatetime.cpp.
◆ currentDateTime()
|
static |
- Returns
- the date and time according to the CPU clock
Definition at line 73 of file kstarsdatetime.cpp.
◆ currentDateTimeUtc()
|
static |
- Returns
- the UTC date and time according to the CPU clock
Definition at line 82 of file kstarsdatetime.cpp.
◆ djd()
|
inline |
- Returns
- the julian day as a long double, including the time as the fractional portion.
Definition at line 163 of file kstarsdatetime.h.
◆ epoch()
|
inline |
This is (approximately) the year expressed as a floating-point value.
- Returns
- the (Julian) epoch value of the Date/Time.
- See also
- setFromEpoch()
- Note
- The definition of Julian Epoch used here comes from http://scienceworld.wolfram.com/astronomy/JulianEpoch.html
Definition at line 197 of file kstarsdatetime.h.
◆ epochToJd()
Takes in an epoch and returns a Julian Date.
- Returns
- the Julian Date (date with fraction)
- Parameters
-
epoch A floating-point year value specifying the Epoch type JULIAN or BESSELIAN depending on what convention the epoch is specified in
Definition at line 256 of file kstarsdatetime.cpp.
◆ fromString()
|
static |
- Returns
- a KStarsDateTime object parsed from the given string.
- Note
- This function is format-agnostic; it will try several formats when parsing the string.
- Parameters
-
s the string expressing the date/time to be parsed.
Definition at line 91 of file kstarsdatetime.cpp.
◆ gst()
dms KStarsDateTime::gst | ( | ) | const |
- Returns
- The Greenwich Sidereal Time The Greenwich sidereal time is the Right Ascension coordinate that is currently transiting the Prime Meridian at the Royal Observatory in Greenwich, UK (longitude=0.0)
Definition at line 167 of file kstarsdatetime.cpp.
◆ GSTtoUT()
Convert a given Greenwich Sidereal Time to Universal Time (=Greenwich Mean Time).
GST
the Greenwich Sidereal Time to convert to Universal Time.
Definition at line 206 of file kstarsdatetime.cpp.
◆ jdToEpoch()
|
static |
Takes in a Julian Date and returns the corresponding epoch year in the given system.
- Returns
- the epoch as a floating-point year value
- Parameters
-
jd Julian date type Epoch system (KStarsDateTime::JULIAN or KStarsDateTime::BESSELIAN)
Definition at line 269 of file kstarsdatetime.cpp.
◆ operator!=()
|
inline |
Definition at line 121 of file kstarsdatetime.h.
◆ operator<()
|
inline |
Definition at line 125 of file kstarsdatetime.h.
◆ operator<=()
|
inline |
Definition at line 129 of file kstarsdatetime.h.
◆ operator=()
|
noexcept |
Definition at line 27 of file kstarsdatetime.cpp.
◆ operator==()
|
inline |
Definition at line 117 of file kstarsdatetime.h.
◆ operator>()
|
inline |
Definition at line 133 of file kstarsdatetime.h.
◆ operator>=()
|
inline |
Definition at line 137 of file kstarsdatetime.h.
◆ setDate()
void KStarsDateTime::setDate | ( | const QDate & | d | ) |
Assign the Date according to a QDate object.
d
the QDate to assign
Definition at line 144 of file kstarsdatetime.cpp.
◆ setDJD()
void KStarsDateTime::setDJD | ( | long double | jd | ) |
Assign the static_cast<long double> Julian Day value, which includes the time of day encoded in the fractional portion.
jd
the Julian Day value to assign.
Definition at line 118 of file kstarsdatetime.cpp.
◆ setFromEpoch() [1/3]
bool KStarsDateTime::setFromEpoch | ( | const QString & | e | ) |
Set the Date/Time from an epoch value, represented as a string.
e
the epoch value
- Returns
- true if date set successfully
- See also
- epoch()
Definition at line 245 of file kstarsdatetime.cpp.
◆ setFromEpoch() [2/3]
void KStarsDateTime::setFromEpoch | ( | double | e | ) |
Set the Date/Time from an epoch value, represented as a double.
e
the epoch value
- Note
- This method assumes that the epoch 1950.0 is Besselian, otherwise assumes that the epoch is a Julian epoch. This is provided for backward compatibility, and because custom catalogs may still use 1950.0 to mean B1950.0 despite the IAU standard for epochs being Julian.
- See also
- epoch()
Definition at line 228 of file kstarsdatetime.cpp.
◆ setFromEpoch() [3/3]
bool KStarsDateTime::setFromEpoch | ( | double | e, |
EpochType | type ) |
Set the Date/Time from an epoch value, represented as a double.
e
the epoch value
- See also
- epoch()
Definition at line 236 of file kstarsdatetime.cpp.
◆ setTime()
void KStarsDateTime::setTime | ( | const QTime & | t | ) |
Assign the Time according to a QTime object.
t
the QTime to assign
- Note
- timespec is NOT changed even if the passed QTime has a different timespec than current.
Definition at line 161 of file kstarsdatetime.cpp.
◆ stringToEpoch()
|
static |
Takes in a string and returns a Julian epoch.
Definition at line 288 of file kstarsdatetime.cpp.
Member Data Documentation
◆ B1900
|
staticconstexpr |
The following values were obtained from Eric Weisstein's world of science: http://scienceworld.wolfram.com/astronomy/BesselianEpoch.html.
Definition at line 250 of file kstarsdatetime.h.
◆ JD_PER_BYEAR
|
staticconstexpr |
Definition at line 251 of file kstarsdatetime.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.