StarComponent
#include <starcomponent.h>
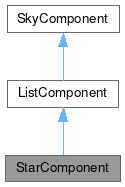
Public Member Functions | |
void | appendListObject (SkyObject *object) |
void | draw (SkyPainter *skyp) override |
void | drawLabels () |
StarObject * | findByHDIndex (int HDnum) |
virtual SkyObject * | findStarByGenetiveName (const QString name) |
SkyObject * | objectNearest (SkyPoint *p, double &maxrad) override |
void | objectsInArea (QList< SkyObject * > &list, const SkyRegion ®ion) override |
bool | selected () override |
void | starsInAperture (QList< StarObject * > &list, const SkyPoint ¢er, float radius, float maglim=-29) |
void | update (KSNumbers *num) override |
![]() | |
ListComponent (SkyComposite *parent) | |
void | appendListObject (SkyObject *object) |
void | clear () |
SkyObject * | findByName (const QString &name, bool exact=true) override |
const QList< SkyObject * > & | objectList () const |
![]() | |
SkyComponent (SkyComposite *parent=nullptr) | |
virtual void | drawTrails (SkyPainter *skyp) |
virtual void | emitProgressText (const QString &message) |
QHash< int, QVector< QPair< QString, const SkyObject * > > > & | objectLists () |
QVector< QPair< QString, const SkyObject * > > & | objectLists (int type) |
QHash< int, QStringList > & | objectNames () |
QStringList & | objectNames (int type) |
SkyComposite * | parent () |
void | removeFromLists (const SkyObject *obj) |
void | removeFromNames (const SkyObject *obj) |
virtual void | updateMoons (KSNumbers *) |
virtual void | updateSolarSystemBodies (KSNumbers *) |
Static Public Member Functions | |
static void | byteSwap (StarData *stardata) |
static StarComponent * | Create (SkyComposite *) |
static StarComponent * | Instance () |
static float | zoomMagnitudeLimit () |
Protected Member Functions | |
StarComponent (SkyComposite *) | |
Additional Inherited Members | |
![]() | |
QHash< QString, SkyObject * > | m_ObjectHash |
QList< SkyObject * > | m_ObjectList |
Detailed Description
Represents the stars on the sky map.
For optimization reasons the stars are not separate objects and are stored in a list.
The StarComponent class manages all stars drawn in KStars. While it handles all stars having names using its own member methods, it shunts the responsibility of unnamed stars to the class 'DeepStarComponent', objects of which it maintains.
- Version
- 1.0
Definition at line 47 of file starcomponent.h.
Constructor & Destructor Documentation
◆ StarComponent()
|
protected |
Definition at line 48 of file starcomponent.cpp.
◆ ~StarComponent()
|
override |
Definition at line 80 of file starcomponent.cpp.
Member Function Documentation
◆ appendListObject()
void StarComponent::appendListObject | ( | SkyObject * | object | ) |
Append a star to the Object List.
(including genetive name)
Overrides ListComponent::appendListObject() to include genetive names of stars as well.
Definition at line 579 of file starcomponent.cpp.
◆ byteSwap()
|
static |
Definition at line 757 of file starcomponent.cpp.
◆ Create()
|
static |
Create an instance of StarComponent.
Definition at line 92 of file starcomponent.cpp.
◆ draw()
|
overridevirtual |
Draw the object on the SkyMap skyp
a pointer to the SkyPainter to use.
Implements SkyComponent.
Definition at line 251 of file starcomponent.cpp.
◆ drawLabels()
void StarComponent::drawLabels | ( | ) |
draw all the labels in the prioritized LabelLists and then clear the LabelLists.
Definition at line 372 of file starcomponent.cpp.
◆ findByHDIndex()
StarObject * StarComponent::findByHDIndex | ( | int | HDnum | ) |
Find stars by HD catalog index.
- Parameters
-
HDnum HD Catalog Number of the star to find
- Returns
- If the star is a static star, a pointer to the star will be returned If it is a dynamic star, a fake copy will be created that survives till the next findByHDIndex() call. If no match was found, returns nullptr.
Definition at line 614 of file starcomponent.cpp.
◆ findStarByGenetiveName()
Definition at line 589 of file starcomponent.cpp.
◆ Instance()
|
inlinestatic |
- Returns
- the instance of StarComponent if already created, nullptr otherwise
Definition at line 64 of file starcomponent.h.
◆ objectNearest()
Find the SkyObject nearest the given SkyPoint.
Look for a SkyObject that is nearer to point p than maxrad. If one is found, then maxrad is reset to the separation of the new nearest object. p
pointer to the SkyPoint to search around maxrad
reference to current search radius in degrees
- Returns
- a pointer to the nearest SkyObject
- Note
- This function simply returns a nullptr pointer; it is reimplemented in various sub-classes.
Reimplemented from ListComponent.
Definition at line 671 of file starcomponent.cpp.
◆ objectsInArea()
|
overridevirtual |
Searches the region(s) and appends the SkyObjects found to the list of sky objects.
Look for a SkyObject that is in one of the regions If found, then append to the list of sky objects list
list of SkyObject to which matching list has to be appended to region
defines the regions in which the search for SkyObject should be done within
Reimplemented from SkyComponent.
Definition at line 602 of file starcomponent.cpp.
◆ selected()
|
overridevirtual |
- Returns
- true if component is to be drawn on the map.
Reimplemented from SkyComponent.
Definition at line 99 of file starcomponent.cpp.
◆ starsInAperture()
void StarComponent::starsInAperture | ( | QList< StarObject * > & | list, |
const SkyPoint & | center, | ||
float | radius, | ||
float | maglim = -29 ) |
Add to the given list, the stars from this component, that lie within the specified circular aperture, and that are brighter than the limiting magnitude specified.
center
The center point of the aperture radius
The radius around the center point that defines the aperture maglim
Optional parameter indicating the limiting magnitude. If magnitude limit is numerically < -28, the limiting magnitude is assumed to be the limiting magnitude of the catalog (i.e. no magnitude limit) list
The list to operate on
Definition at line 721 of file starcomponent.cpp.
◆ update()
|
overridevirtual |
Update the sky positions of this component.
This function usually just updates the Horizontal (Azimuth/Altitude) coordinates of the objects in this component. If the KSNumbers* argument is not nullptr, this function also recomputes precession and nutation for the date in KSNumbers. num
Pointer to the KSNumbers object
- Note
- By default, the num parameter is nullptr, indicating that Precession/Nutation computation should be skipped; this computation is only occasionally required.
Reimplemented from ListComponent.
Definition at line 133 of file starcomponent.cpp.
◆ zoomMagnitudeLimit()
|
static |
Definition at line 218 of file starcomponent.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:41 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.