DeepStarComponent
#include <deepstarcomponent.h>
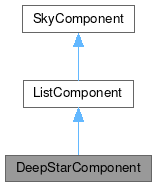
Public Member Functions | |
DeepStarComponent (SkyComposite *parent, QString fileName, float trigMag, bool staticstars=false) | |
void | draw (SkyPainter *skyp) override |
float | faintMagnitude () const |
bool | fileOpen () const |
StarObject * | findByHDIndex (int HDnum) |
BinFileHelper * | getStarReader () |
bool | hasStaticStars () const |
bool | loadStaticStars () |
SkyObject * | objectNearest (SkyPoint *p, double &maxrad) override |
bool | openDataFile () |
bool | selected () override |
bool | starsInAperture (QList< StarObject * > &list, const SkyPoint ¢er, float radius, float maglim=-29) |
void | update (KSNumbers *num) override |
bool | verifySBLIntegrity () |
![]() | |
ListComponent (SkyComposite *parent) | |
void | appendListObject (SkyObject *object) |
void | clear () |
SkyObject * | findByName (const QString &name, bool exact=true) override |
const QList< SkyObject * > & | objectList () const |
SkyObject * | objectNearest (SkyPoint *p, double &maxrad) override |
void | update (KSNumbers *num=nullptr) override |
![]() | |
SkyComponent (SkyComposite *parent=nullptr) | |
virtual void | drawTrails (SkyPainter *skyp) |
virtual void | emitProgressText (const QString &message) |
QHash< int, QVector< QPair< QString, const SkyObject * > > > & | objectLists () |
QVector< QPair< QString, const SkyObject * > > & | objectLists (int type) |
QHash< int, QStringList > & | objectNames () |
QStringList & | objectNames (int type) |
virtual void | objectsInArea (QList< SkyObject * > &list, const SkyRegion ®ion) |
SkyComposite * | parent () |
void | removeFromLists (const SkyObject *obj) |
void | removeFromNames (const SkyObject *obj) |
virtual void | updateMoons (KSNumbers *) |
virtual void | updateSolarSystemBodies (KSNumbers *) |
Static Public Member Functions | |
static void | byteSwap (DeepStarData *stardata) |
static void | byteSwap (StarData *stardata) |
Static Public Attributes | |
static StarBlockFactory | m_StarBlockFactory |
Additional Inherited Members | |
![]() | |
QHash< QString, SkyObject * > | m_ObjectHash |
QList< SkyObject * > | m_ObjectList |
Detailed Description
Stores and manages unnamed stars, most of which are dynamically loaded into memory.
- Note
- Much of the code here is copied from class StarComponent authored by Thomas Kabelmann
- Version
- 0.1
Definition at line 31 of file deepstarcomponent.h.
Constructor & Destructor Documentation
◆ DeepStarComponent()
DeepStarComponent::DeepStarComponent | ( | SkyComposite * | parent, |
QString | fileName, | ||
float | trigMag, | ||
bool | staticstars = false ) |
Definition at line 32 of file deepstarcomponent.cpp.
◆ ~DeepStarComponent()
|
override |
Definition at line 43 of file deepstarcomponent.cpp.
Member Function Documentation
◆ byteSwap() [1/2]
|
static |
Definition at line 601 of file deepstarcomponent.cpp.
◆ byteSwap() [2/2]
|
static |
Definition at line 611 of file deepstarcomponent.cpp.
◆ draw()
|
overridevirtual |
Draw the object on the SkyMap skyp
a pointer to the SkyPainter to use.
Implements SkyComponent.
Definition at line 231 of file deepstarcomponent.cpp.
◆ faintMagnitude()
|
inline |
- Returns
- return the estimated faint magnitude limit of this DeepStarComponent
Definition at line 62 of file deepstarcomponent.h.
◆ fileOpen()
|
inline |
Definition at line 75 of file deepstarcomponent.h.
◆ findByHDIndex()
StarObject * DeepStarComponent::findByHDIndex | ( | int | HDnum | ) |
- Parameters
-
HDnum Henry-Draper catalog number of the desired star
- Returns
- A star matching the given Henry-Draper catalog number
Definition at line 482 of file deepstarcomponent.cpp.
◆ getStarReader()
|
inline |
Definition at line 77 of file deepstarcomponent.h.
◆ hasStaticStars()
|
inline |
- Returns
- true if this DeepStarComponent has static stars (that are not dynamically loaded)
Definition at line 57 of file deepstarcomponent.h.
◆ loadStaticStars()
bool DeepStarComponent::loadStaticStars | ( | ) |
Definition at line 50 of file deepstarcomponent.cpp.
◆ objectNearest()
- Returns
- Nearest star within maxrad of SkyPoint p, or nullptr if not found
Reimplemented from SkyComponent.
Definition at line 494 of file deepstarcomponent.cpp.
◆ openDataFile()
bool DeepStarComponent::openDataFile | ( | ) |
Definition at line 425 of file deepstarcomponent.cpp.
◆ selected()
|
overridevirtual |
- Returns
- true if component is to be drawn on the map.
Reimplemented from SkyComponent.
Definition at line 209 of file deepstarcomponent.cpp.
◆ starsInAperture()
bool DeepStarComponent::starsInAperture | ( | QList< StarObject * > & | list, |
const SkyPoint & | center, | ||
float | radius, | ||
float | maglim = -29 ) |
Add to the given list, the stars from this component, that lie within the specified circular aperture, and that are brighter than the limiting magnitude specified.
center
The center point of the aperture radius
The radius around the center point that defines the aperture maglim
Optional parameter indicating the limiting magnitude. If magnitude limit is numerically < -28, the limiting magnitude is assumed to be the limiting magnitude of the catalog (i.e. no magnitude limit) list
The list to operate on
- Returns
- false if the limiting magnitude is brighter than the trigger magnitude of the DeepStarComponent
Definition at line 552 of file deepstarcomponent.cpp.
◆ update()
|
overridevirtual |
Update the sky position(s) of this component.
This function usually just updates the Horizontal (Azimuth/Altitude) coordinates of its member object(s). However, the precession and nutation must also be recomputed periodically. num
Pointer to the KSNumbers object
- See also
- SingleComponent::update()
- ListComponent::update()
- ConstellationBoundaryComponent::update()
Reimplemented from SkyComponent.
Definition at line 226 of file deepstarcomponent.cpp.
◆ verifySBLIntegrity()
bool DeepStarComponent::verifySBLIntegrity | ( | ) |
Definition at line 623 of file deepstarcomponent.cpp.
Member Data Documentation
◆ m_StarBlockFactory
|
static |
Definition at line 102 of file deepstarcomponent.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.