SkyMap
#include <skymap.h>
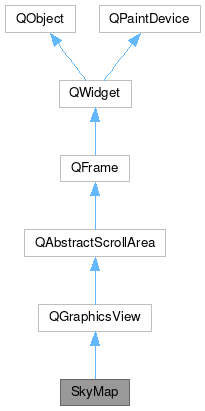
Public Types | |
enum | Cursor { NoCursor , Cross , Circle } |
enum | Projection { Lambert , AzimuthalEquidistant , Orthographic , Equirectangular , Stereographic , Gnomonic , UnknownProjection } |
![]() | |
enum | CacheModeFlag |
enum | DragMode |
enum | OptimizationFlag |
enum | ViewportAnchor |
enum | ViewportUpdateMode |
![]() | |
enum | SizeAdjustPolicy |
![]() | |
enum | Shadow |
enum | Shape |
enum | StyleMask |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
Signals | |
void | destinationChanged () |
void | mosaicCenterChanged (dms dRA, dms dDE) |
void | mousePointChanged (SkyPoint *) |
void | objectChanged (SkyObject *) |
void | objectClicked (SkyObject *) |
void | positionChanged (SkyPoint *) |
void | positionClicked (SkyPoint *) |
void | removeSkyObject (SkyObject *object) |
void | updateQueued () |
void | zoomChanged () |
Detailed Description
This is the canvas on which the sky is painted.
It's the main widget for KStars. Contains SkyPoint members for the map's Focus (current central position), Destination (requested central position), FocusPoint (next queued position to be focused), MousePoint (position of mouse cursor), and ClickedPoint (position of last mouse click). Also contains the InfoBoxes for on-screen data display.
SkyMap handles most user interaction events (both mouse and keyboard).
Canvas widget for displaying the sky bitmap; also handles user interaction events.
- Version
- 1.0
Member Enumeration Documentation
◆ Cursor
◆ Projection
Constructor & Destructor Documentation
◆ SkyMap()
|
protected |
Constructor.
Read stored settings from KConfig object (focus position, zoom factor, sky color, etc.). Run initPopupMenus().
Definition at line 176 of file skymap.cpp.
◆ ~SkyMap()
|
override |
Destructor (empty)
Definition at line 279 of file skymap.cpp.
Member Function Documentation
◆ clickedObject()
|
inline |
Retrieve the object nearest to a mouse click event.
If the user clicks on the sky map, a pointer to the nearest SkyObject is stored in the private member ClickedObject. This function returns the ClickedObject pointer, or nullptr if there is no CLickedObject. @return a pointer to the object nearest to a user mouse click.
◆ clickedPoint()
|
inline |
Retrieve the ClickedPoint position.
When the user clicks on a point in the sky map, the sky coordinates of the mouse cursor are stored in the private member ClickedPoint. This function retrieves a pointer to ClickedPoint. @return a pointer to ClickedPoint, the sky coordinates where the user clicked.
◆ Create()
|
static |
Definition at line 164 of file skymap.cpp.
◆ destination()
|
inline |
◆ destinationChanged
|
signal |
Emitted by setDestination(), and connected to slewFocus().
Whenever the Destination point is changed, slewFocus() will iteratively step the Focus toward Destination until it is reached.
◆ drawObjectLabels()
Proxy method for SkyMapDrawAbstract::drawObjectLabels()
◆ event()
|
overrideprotectedvirtual |
Reimplemented from QGraphicsView.
Definition at line 413 of file skymapevents.cpp.
◆ exportSkyImage() [1/2]
|
inline |
Proxy method for SkyMapDrawAbstract::exportSkyImage()
◆ exportSkyImage() [2/2]
|
inline |
◆ focus()
|
inline |
◆ focusObject()
|
inline |
Retrieve the object which is centered in the sky map.
If the user centers the sky map on an object (by double-clicking or using the Find Object dialog), a pointer to the "focused" object is stored in the private member FocusObject. This function returns a pointer to the FocusObject, or nullptr if there is not FocusObject. @return a pointer to the object at the center of the sky map.
◆ focusPoint()
|
inline |
retrieve the FocusPoint position.
The FocusPoint stores the position on the sky that is to be focused next. This is not exactly the same as the Destination point, because when the Destination is set, it will begin slewing immediately. @return a pointer to the sky point which is to be focused next.
◆ forceUpdate
|
slot |
Recalculates the positions of objects in the sky, and then repaints the sky map.
If the positions don't need to be recalculated, use update() instead of forceUpdate(). This saves a lot of CPU time.
- Parameters
-
now if true, paintEvent() is run immediately. Otherwise, it is added to the event queue
Definition at line 1177 of file skymap.cpp.
◆ forceUpdateNow
|
inlineslot |
Convenience function; simply calls forceUpdate(true).
- See also
- forceUpdate()
◆ fov()
float SkyMap::fov | ( | ) |
- Returns
- the angular field of view of the sky map, in degrees.
- Note
- it must use either the height or the width of the window to calculate the FOV angle. It chooses whichever is larger.
Definition at line 1197 of file skymap.cpp.
◆ getCenterPoint()
SkyPoint SkyMap::getCenterPoint | ( | ) |
Definition at line 836 of file skymap.cpp.
◆ getSkyMapDrawAbstract()
|
inline |
◆ Instance()
|
static |
Definition at line 171 of file skymap.cpp.
◆ IsFocused()
◆ isInFovCaptureMode()
◆ isInObjectPointingMode()
◆ isObjectLabeled()
bool SkyMap::isObjectLabeled | ( | SkyObject * | o | ) |
- Returns
- true if the object currently has a user label attached.
- Note
- this function only checks for a label explicitly added to the object with the right-click popup menu; other kinds of labels are not detected by this function.
- Parameters
-
o pointer to the sky object to be tested for a User label.
Definition at line 831 of file skymap.cpp.
◆ IsSlewing()
◆ isSlewing()
bool SkyMap::isSlewing | ( | ) | const |
Definition at line 1364 of file skymap.cpp.
◆ keyPressEvent()
|
overrideprotectedvirtual |
Process keystrokes:
- arrow keys Slew the map
- +/- keys Zoom in and out
- Space Toggle between Horizontal and Equatorial coordinate systems
- 0-9 Go to a major Solar System body (0=Sun; 1-9 are the major planets, except 3=Moon)
- [ Place starting point for measuring an angular distance
- ] End point for Angular Distance; display measurement.
- Escape Cancel Angular measurement
- ,/< Step backward one time step
- ./> Step forward one time step
Reimplemented from QGraphicsView.
Definition at line 48 of file skymapevents.cpp.
◆ keyReleaseEvent()
|
overrideprotectedvirtual |
When keyRelease is triggered, just set the "slewing" flag to false, and update the display (to draw objects that are hidden when slewing==true).
Reimplemented from QGraphicsView.
Definition at line 477 of file skymapevents.cpp.
◆ mosaicCenterChanged
Emitter when mosaic center is dragged in the sky map.
◆ mouseDoubleClickEvent()
|
overrideprotectedvirtual |
Center SkyMap at double-clicked location
Reimplemented from QGraphicsView.
Definition at line 859 of file skymapevents.cpp.
◆ mouseMoveEvent()
|
overrideprotectedvirtual |
This function does several different things depending on the state of the program:
- If Angle-measurement mode is active, update the end-ruler point to the mouse cursor, and continue this function.
- If we are defining a ZoomBox, update the ZoomBox rectangle, redraw the screen, and return.
- If dragging the mouse in the map, update focus such that RA, Dec under the mouse cursor remains constant.
- If just moving the mouse, simply update the curso coordinates in the status bar.
Reimplemented from QGraphicsView.
Definition at line 503 of file skymapevents.cpp.
◆ mousePoint()
|
inline |
◆ mousePointChanged
|
signal |
Emitted when position under mouse changed.
◆ mousePressEvent()
|
overrideprotectedvirtual |
Determine RA, Dec coordinates of clicked location.
Find the SkyObject which is nearest to the clicked location.
If left-clicked: Set set mouseButtonDown==true, slewing==true; display nearest object name in status bar. If right-clicked: display popup menu appropriate for nearest object.
Reimplemented from QGraphicsView.
Definition at line 760 of file skymapevents.cpp.
◆ mouseReleaseEvent()
|
overrideprotectedvirtual |
set mouseButtonDown==false, slewing==false
Reimplemented from QGraphicsView.
Definition at line 697 of file skymapevents.cpp.
◆ objectChanged
|
signal |
Emitted when current object changed.
◆ objectClicked
|
signal |
Emitted when a position is clicked.
◆ positionChanged
|
signal |
Emitted when pointing changed.
(At least should)
◆ positionClicked
|
signal |
Emitted when a position is clicked.
◆ projector()
◆ removeSkyObject
|
signal |
Emitted when a sky object is removed from the database.
◆ resizeEvent()
|
overrideprotectedvirtual |
If the skymap will be resized, the sky must be new computed.
So this function calls explicitly new computing of the skymap.
Reimplemented from QGraphicsView.
Definition at line 32 of file skymapevents.cpp.
◆ setClickedObject()
void SkyMap::setClickedObject | ( | SkyObject * | o | ) |
Set the ClickedObject pointer to the argument.
- Parameters
-
o pointer to the SkyObject to be assigned as the ClickedObject
Definition at line 366 of file skymap.cpp.
◆ setClickedPoint()
Set the ClickedPoint to the skypoint given as an argument.
- Parameters
-
f pointer to the new ClickedPoint.
Definition at line 1012 of file skymap.cpp.
◆ setDestination() [1/2]
sets the destination point of the skymap, using ra/dec coordinates.
@note This function behaves essentially like the above function. It differs only in the data types of its arguments. @param ra the new right ascension @param dec the new declination
Definition at line 989 of file skymap.cpp.
◆ setDestination() [2/2]
sets the destination point of the sky map.
- Note
- setDestination() emits the destinationChanged() SIGNAL, which triggers the SLOT function SkyMap::slewFocus(). This function iteratively steps the Focus point toward Destination, repainting the sky at each step (if Options::useAnimatedSlewing()==true).
- Parameters
-
f a pointer to the SkyPoint the map should slew to
Definition at line 984 of file skymap.cpp.
◆ setDestinationAltAz()
sets the destination point of the sky map, using its alt/az coordinates.
- Parameters
-
alt the new altitude az the new azimuth altIsRefracted set to true if the altitude supplied is apparent
Definition at line 996 of file skymap.cpp.
◆ setFocus() [1/2]
sets the focus point of the skymap, using ra/dec coordinates
@note This function behaves essentially like the above function. It differs only in the data types of its arguments. @param ra the new right ascension @param dec the new declination
Definition at line 963 of file skymap.cpp.
◆ setFocus() [2/2]
void SkyMap::setFocus | ( | SkyPoint * | f | ) |
sets the central focus point of the sky map.
- Parameters
-
f a pointer to the SkyPoint the map should be centered on
Definition at line 958 of file skymap.cpp.
◆ setFocusAltAz()
sets the focus point of the sky map, using its alt/az coordinates
- Parameters
-
alt the new altitude (actual, without refraction correction) az the new azimuth
Definition at line 972 of file skymap.cpp.
◆ setFocusObject()
void SkyMap::setFocusObject | ( | SkyObject * | o | ) |
Set the FocusObject pointer to the argument.
- Parameters
-
o pointer to the SkyObject to be assigned as the FocusObject
Definition at line 371 of file skymap.cpp.
◆ setFocusPoint()
|
inline |
◆ setFovCaptureMode()
◆ setLegend()
◆ setMouseCursorShape()
void SkyMap::setMouseCursorShape | ( | Cursor | type | ) |
Sets the shape of the default mouse cursor.
Definition at line 1309 of file skymap.cpp.
◆ setObjectPointingMode()
◆ setPreviewLegend()
◆ setupProjector()
void SkyMap::setupProjector | ( | ) |
Call to set up the projector before a draw cycle.
Definition at line 1246 of file skymap.cpp.
◆ setZoomFactor()
void SkyMap::setZoomFactor | ( | double | factor | ) |
◆ showFocusCoords()
void SkyMap::showFocusCoords | ( | ) |
Update object name and coordinates in the Focus InfoBox.
Definition at line 327 of file skymap.cpp.
◆ slewFocus
|
slot |
Step the Focus point toward the Destination point.
Do this iteratively, redrawing the Sky Map after each step, until the Focus point is within 1 step of the Destination point. For the final step, snap directly to Destination, and redraw the map.
Definition at line 1037 of file skymap.cpp.
◆ slotAddFlag
|
slot |
Open Flag Manager window with clickedObject() RA and Dec entered.
Definition at line 759 of file skymap.cpp.
◆ slotAddObjectLabel
|
slot |
Add ClickedObject to KStarsData::ObjLabelList, which stores pointers to SkyObjects which have User Labels attached.
Definition at line 872 of file skymap.cpp.
◆ slotAddPlanetTrail
|
slot |
Add a Planet Trail to ClickedObject.
- Note
- Trails are added simply by calling KSPlanetBase::addToTrail() to add the first point. as long as the trail is not empty, new points will be automatically appended to it.
- if ClickedObject is not a Solar System body, this function does nothing.
- See also
- KSPlanetBase::addToTrail()
Definition at line 888 of file skymap.cpp.
◆ slotBeginAngularDistance
|
slot |
Enables the angular distance measuring mode.
It saves the first position of the ruler in a SkyPoint. It makes difference between having clicked on the skymap and not having done so
- Note
- This method is draw-backend independent.
Definition at line 611 of file skymap.cpp.
◆ slotBeginStarHop
|
slot |
Definition at line 616 of file skymap.cpp.
◆ slotCancelLegendPreviewMode
|
slot |
Definition at line 920 of file skymap.cpp.
◆ slotCancelRulerMode
|
slot |
Disables the angular distance measuring mode.
Nothing is printed in the status bar
Definition at line 753 of file skymap.cpp.
◆ slotCaptureFov
|
slot |
Definition at line 936 of file skymap.cpp.
◆ slotCenter
|
slot |
Center the display at the point ClickedPoint.
The essential part of the function is to simply set the Destination point, which will emit the destinationChanged() SIGNAL, which triggers the slewFocus() SLOT. Additionally, this function performs some bookkeeping tasks, such updating whether we are tracking the new object/position, adding a Planet Trail if required, etc.
- See also
- destinationChanged()
- slewFocus()
Definition at line 380 of file skymap.cpp.
◆ slotClockSlewing
|
slot |
Checks whether the timestep exceeds a threshold value.
If so, sets ClockSlewing=true and sets the SimClock to ManualMode.
Definition at line 944 of file skymap.cpp.
◆ slotCopyCoordinates
|
slot |
slotCopyCoordinates Copies J2000 and JNow equatorial coordinates to the clipboard in addition to horizontal coords.
Definition at line 504 of file skymap.cpp.
◆ slotCopyTLE
|
slot |
slotCopyTLE Copy satellite TLE to clipboard.
Definition at line 546 of file skymap.cpp.
◆ slotDeleteFlag
|
slot |
Delete selected flag.
- Parameters
-
flagIdx index of flag to be deleted.
Definition at line 798 of file skymap.cpp.
◆ slotDetail
|
slot |
Popup menu function: Show the Detailed Information window for ClickedObject.
Definition at line 898 of file skymap.cpp.
◆ slotDisplayFadingText
Render a fading text label on the screen to flash information.
Definition at line 1377 of file skymap.cpp.
◆ slotDSS
|
slot |
Popup menu function: Display 1st-Generation DSS image with the Image Viewer.
- Note
- the URL is generated using the coordinates of ClickedPoint.
Definition at line 472 of file skymap.cpp.
◆ slotEditFlag
|
slot |
Open Flag Manager window with selected flag focused and ready to edit.
- Parameters
-
flagIdx index of flag to be edited.
Definition at line 789 of file skymap.cpp.
◆ slotEndRulerMode
|
slot |
Computes the angular distance, prints the result in the status bar and disables the angular distance measuring mode If the user has clicked on the map the status bar shows the name of the clicked object plus the angular distance.
If the user did not clicked on the map, just pressed ], only the angular distance is printed
- Note
- This method is draw-backend independent.
Definition at line 647 of file skymap.cpp.
◆ slotEyepieceView
|
slot |
Render eyepiece view.
Definition at line 607 of file skymap.cpp.
◆ slotFinishFovCaptureMode
|
slot |
Definition at line 927 of file skymap.cpp.
◆ slotImage
|
slot |
Popup menu function: Show image of ClickedObject (only available for some objects).
Definition at line 812 of file skymap.cpp.
◆ slotInfo
|
slot |
Popup menu function: Show webpage about ClickedObject (only available for some objects).
Definition at line 822 of file skymap.cpp.
◆ slotObjectSelected
|
slot |
Object pointing for Printing Wizard done.
Definition at line 911 of file skymap.cpp.
◆ slotRemoveCustomObject
|
slot |
Remove custom object from internet search in the local catalog.
Definition at line 852 of file skymap.cpp.
◆ slotRemoveObjectLabel
|
slot |
Remove ClickedObject from KStarsData::ObjLabelList, which stores pointers to SkyObjects which have User Labels attached.
Definition at line 846 of file skymap.cpp.
◆ slotRemovePlanetTrail
|
slot |
Remove the PlanetTrail from ClickedObject.
- Note
- The Trail is removed by simply calling KSPlanetBase::clearTrail(). As long as the trail is empty, no new points will be automatically appended.
- See also
- KSPlanetBase::clearTrail()
Definition at line 878 of file skymap.cpp.
◆ slotSDSS
|
slot |
Popup menu function: Display Sloan Digital Sky Survey image with the Image Viewer.
- Note
- the URL is generated using the coordinates of ClickedPoint.
Definition at line 563 of file skymap.cpp.
◆ slotSetSkyRotation
|
slot |
Sets the base sky rotation (before correction) to the given angle.
Definition at line 1222 of file skymap.cpp.
◆ slotStartXplanetViewer
|
slot |
Run Xplanet Viewer to display images of the planets.
Definition at line 1369 of file skymap.cpp.
◆ slotToggleFocusBox
|
slot |
Toggle visibility of focus infobox.
Definition at line 263 of file skymap.cpp.
◆ slotToggleGeoBox
|
slot |
Toggle visibility of geo infobox.
Definition at line 258 of file skymap.cpp.
◆ slotToggleInfoboxes
|
slot |
Toggle visibility of all infoboxes.
Definition at line 273 of file skymap.cpp.
◆ slotToggleTimeBox
|
slot |
Toggle visibility of time infobox.
Definition at line 268 of file skymap.cpp.
◆ slotUpdateSky
|
slot |
Update the focus point and call forceUpdate()
- Parameters
-
now is passed on to forceUpdate()
Definition at line 458 of file skymap.cpp.
◆ slotZoomDefault
|
slot |
Set default zoom.
Definition at line 1162 of file skymap.cpp.
◆ slotZoomIn
|
slot |
Zoom in one step.
Definition at line 1152 of file skymap.cpp.
◆ slotZoomOut
|
slot |
Zoom out one step.
Definition at line 1157 of file skymap.cpp.
◆ stopTracking()
void SkyMap::stopTracking | ( | ) |
Definition at line 404 of file skymapevents.cpp.
◆ updateAngleRuler()
void SkyMap::updateAngleRuler | ( | ) |
update the geometry of the angle ruler.
Definition at line 1357 of file skymap.cpp.
◆ updateFocus()
void SkyMap::updateFocus | ( | ) |
Update the focus position according to current options.
Definition at line 1017 of file skymap.cpp.
◆ updateInfoBoxes()
void SkyMap::updateInfoBoxes | ( | ) |
Update info boxes coordinates.
Definition at line 335 of file skymap.cpp.
◆ wheelEvent()
|
overrideprotectedvirtual |
Zoom in and out with the mouse wheel.
Reimplemented from QGraphicsView.
Definition at line 689 of file skymapevents.cpp.
◆ zoomChanged
|
signal |
Emitted when zoom level is changed.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Tue Mar 26 2024 11:19:05 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.