Projector
#include <projector.h>
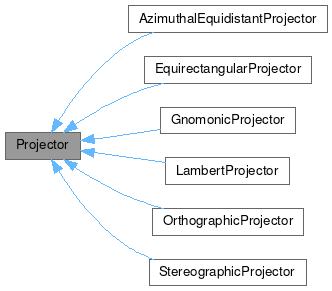
Public Types | |
enum | Projection { Lambert , AzimuthalEquidistant , Orthographic , Equirectangular , Stereographic , Gnomonic , UnknownProjection } |
Public Member Functions | |
Projector (const ViewParams &p) | |
bool | checkVisibility (const SkyPoint *p) const |
QPointF | clipLine (SkyPoint *p1, SkyPoint *p2) const |
Eigen::Vector2f | clipLineVec (SkyPoint *p1, SkyPoint *p2) const |
virtual QPolygonF | clipPoly () const |
double | findNorthPA (const SkyPoint *o, float x, float y) const |
double | findPA (const SkyObject *o, float x, float y) const |
double | findZenithPA (const SkyPoint *o, float x, float y) const |
double | fov () const |
virtual SkyPoint | fromScreen (const QPointF &p, dms *LST, const dms *lat, bool onlyAltAz=false) const |
virtual QVector< Eigen::Vector2f > | groundPoly (SkyPoint *labelpoint=nullptr, bool *drawLabel=nullptr) const |
bool | onScreen (const Eigen::Vector2f &p) const |
bool | onScreen (const QPointF &p) const |
void | setViewParams (const ViewParams &p) |
QPointF | toScreen (const SkyPoint *o, bool oRefract=true, bool *onVisibleHemisphere=nullptr) const |
virtual Eigen::Vector2f | toScreenVec (const SkyPoint *o, bool oRefract=true, bool *onVisibleHemisphere=nullptr) const |
virtual Q_INVOKABLE Projection | type () const =0 |
virtual bool | unusablePoint (const QPointF &p) const |
virtual void | updateClipPoly () |
ViewParams | viewParams () const |
Protected Member Functions | |
virtual double | cosMaxFieldAngle () const |
Eigen::Vector2f | derst (double x, double y) const |
virtual double | projectionK (double x) const |
virtual double | projectionL (double x) const |
virtual double | radius () const |
Eigen::Vector2f | rst (double x, double y) const |
Static Protected Member Functions | |
static SkyPoint | pointAt (double az) |
Protected Attributes | |
QPolygonF | m_clipPolygon |
double | m_cosY0 { 0 } |
KStarsData * | m_data { nullptr } |
double | m_fov { 0 } |
double | m_sinY0 { 0 } |
ViewParams | m_vp |
Detailed Description
The Projector class is the primary class that serves as an interface to handle projections.
Definition at line 57 of file projector.h.
Member Enumeration Documentation
◆ Projection
enum Projector::Projection |
Definition at line 77 of file projector.h.
Constructor & Destructor Documentation
◆ Projector()
|
explicit |
Constructor.
- Parameters
-
p the ViewParams for this projection
Definition at line 38 of file projector.cpp.
Member Function Documentation
◆ checkVisibility()
bool Projector::checkVisibility | ( | const SkyPoint * | p | ) | const |
Determine if the skypoint p is likely to be visible in the display window.
checkVisibility() is an optimization function. It determines whether an object appears within the bounds of the skymap window, and therefore should be drawn. The idea is to save time by skipping objects which are off-screen, so it is absolutely essential that checkVisibility() is significantly faster than the computations required to draw the object to the screen.
If the ground is to be filled, the function first checks whether the point is below the horizon, because they will be covered by the ground anyways. Importantly, it does not call the expensive EquatorialToHorizontal function. This means that the horizontal coordinates MUST BE CORRECT! The vast majority of points are already synchronized, so recomputing the horizontal coordinates is a waste.
The function then checks the difference between the Declination/Altitude coordinate of the Focus position, and that of the point p. If the absolute value of this difference is larger than fov, then the function returns false. For most configurations of the sky map window, this simple check is enough to exclude a large number of objects.
Next, it determines if one of the poles of the current Coordinate System (Equatorial or Horizontal) is currently inside the sky map window. This is stored in the member variable 'bool SkyMap::isPoleVisible, and is set by the function SkyMap::setMapGeometry(), which is called by SkyMap::paintEvent(). If a Pole is visible, then it will return true immediately. The idea is that when a pole is on-screen it is computationally expensive to determine whether a particular position is on-screen or not: for many valid Dec/Alt values, all values of RA/Az will indeed be onscreen, but for other valid Dec/Alt values, only most RA/Az values are onscreen. It is cheaper to simply accept all "horizontal" RA/Az values, since we have already determined that they are on-screen in the "vertical" Dec/Alt coordinate.
Finally, if no Pole is onscreen, it checks the difference between the Focus position's RA/Az coordinate and that of the point p. If the absolute value of this difference is larger than XMax, the function returns false. Otherwise, it returns true.
- Parameters
-
p pointer to the skypoint to be checked.
- Returns
- true if the point p was found to be inside the Sky map window.
- See also
- SkyMap::setMapGeometry()
- SkyMap::fov()
- Note
- If you are creating skypoints using equatorial coordinates, then YOU MUST CALL EQUATORIALTOHORIZONTAL BEFORE THIS FUNCTION!
To avoid calculating refraction, we just use the unrefracted altitude and add a 2-degree 'safety factor'
Definition at line 183 of file projector.cpp.
◆ clipLine()
ASSUMES *p1 did not clip but *p2 did.
Returns the QPointF on the line between *p1 and *p2 that just clips.
Definition at line 108 of file projector.cpp.
◆ clipLineVec()
ASSUMES *p1 did not clip but *p2 did.
Returns the Eigen::Vector2f on the line between *p1 and *p2 that just clips.
Definition at line 113 of file projector.cpp.
◆ clipPoly()
|
virtual |
- Returns
- the clipping polygen covering the visible sky area. Anything outside this polygon is clipped by QPainter.
Definition at line 435 of file projector.cpp.
◆ cosMaxFieldAngle()
|
inlineprotectedvirtual |
This function returns the cosine of the maximum field angle, i.e., the maximum angular distance from the focus for which a point should be projected.
Default is 0, i.e., 90 degrees.
Reimplemented in GnomonicProjector, and StereographicProjector.
Definition at line 299 of file projector.h.
◆ derst()
|
inlineprotected |
Transform screen (x, y) to projector (x, y) accounting for scale, rotation.
Transforms the Cartesian position on the screen to the Cartesian position accepted by the projector algorithm by applying th escale factor, rotation and shift from SkyMap origin
rst stands for rotate-scale-translate
- See also
- rst
Definition at line 336 of file projector.h.
◆ findNorthPA()
double Projector::findNorthPA | ( | const SkyPoint * | o, |
float | x, | ||
float | y ) const |
Determine the on-screen position angle of a SkyPont with recept with NCP.
This is the object's sky position angle (w.r.t. North). of "North" at the position of the object (w.r.t. the screen Y-axis). The latter is determined by constructing a test point with the same RA but a slightly increased Dec as the object, and calculating the angle w.r.t. the Y-axis of the line connecting the object to its test point.
- Note
- This method assumes that o->pa() returns a clockwise sense angle @fixme We seem to use different conventions across KStars
Definition at line 236 of file projector.cpp.
◆ findPA()
double Projector::findPA | ( | const SkyObject * | o, |
float | x, | ||
float | y ) const |
Determine the on-screen position angle of a SkyObject.
This is the sum of the object's sky position angle (w.r.t. North), and the position angle of "North" at the position of the object (w.r.t. the screen Y-axis). The latter is determined by constructing a test point with the same RA but a slightly increased Dec as the object, and calculating the angle w.r.t. the Y-axis of the line connecting the object to its test point.
Definition at line 271 of file projector.cpp.
◆ findZenithPA()
double Projector::findZenithPA | ( | const SkyPoint * | o, |
float | x, | ||
float | y ) const |
Determine the on-screen angle of a SkyPoint with respect to Zenith.
- Note
- Similar to
- See also
- findNorthPA
- Note
- It is assumed that EquatorialToHorizontal has been called on
o
@description This is determined by constructing a test point with the same Azimuth but a slightly increased Altitude, and calculating the angle w.r.t. the Y-axis of the line connecting the object to its test point.
Definition at line 276 of file projector.cpp.
◆ fov()
double Projector::fov | ( | ) | const |
Return the FOV of this projection.
Definition at line 88 of file projector.cpp.
◆ fromScreen()
|
virtual |
Determine RA, Dec coordinates of the pixel at (dx, dy), which are the screen pixel coordinate offsets from the center of the Sky pixmap.
- Parameters
-
p the screen pixel position to convert LST pointer to the local sidereal time, as a dms object. lat pointer to the current geographic laitude, as a dms object onlyAltAz the returned SkyPoint's RA & DEC are not computed, only Alt/Az.
N.B. We don't cache these sin/cos values in the inverse projection because it causes 'shaking' when moving the sky.
Reimplemented in EquirectangularProjector.
Definition at line 460 of file projector.cpp.
◆ groundPoly()
|
virtual |
Get the ground polygon.
- Parameters
-
labelpoint This point will be set to something suitable for attaching a label drawLabel this tells whether to draw a label.
- Returns
- the ground polygon
Reimplemented in EquirectangularProjector.
Definition at line 313 of file projector.cpp.
◆ onScreen() [1/2]
bool Projector::onScreen | ( | const Eigen::Vector2f & | p | ) | const |
Definition at line 103 of file projector.cpp.
◆ onScreen() [2/2]
bool Projector::onScreen | ( | const QPointF & | p | ) | const |
Check whether the projected point is on-screen.
Definition at line 98 of file projector.cpp.
◆ pointAt()
|
staticprotected |
Helper function for drawing ground.
- Returns
- the point with Alt = 0, az =
az
Definition at line 29 of file projector.cpp.
◆ projectionK()
|
inlineprotectedvirtual |
This function handles some of the projection-specific code.
- See also
- toScreen()
Reimplemented in AzimuthalEquidistantProjector, GnomonicProjector, LambertProjector, OrthographicProjector, and StereographicProjector.
Definition at line 280 of file projector.h.
◆ projectionL()
|
inlineprotectedvirtual |
This function handles some of the projection-specific code.
- See also
- toScreen()
Reimplemented in AzimuthalEquidistantProjector, GnomonicProjector, LambertProjector, OrthographicProjector, and StereographicProjector.
Definition at line 289 of file projector.h.
◆ radius()
|
inlineprotectedvirtual |
Get the radius of this projection's sky circle.
- Returns
- the radius in radians
Reimplemented in AzimuthalEquidistantProjector, EquirectangularProjector, GnomonicProjector, LambertProjector, OrthographicProjector, and StereographicProjector.
Definition at line 271 of file projector.h.
◆ rst()
|
inlineprotected |
Transform proj (x, y) to screen (x, y) accounting for scale and rotation.
Transforms the Cartesian position given by the projector algorithm into the screen coordinate by applying the scale factor, rotation and shift from SkyMap origin
rst stands for rotate-scale-translate
Definition at line 314 of file projector.h.
◆ setViewParams()
void Projector::setViewParams | ( | const ViewParams & | p | ) |
Update cached values for projector.
Precompute cached values
Definition at line 46 of file projector.cpp.
◆ toScreen()
QPointF Projector::toScreen | ( | const SkyPoint * | o, |
bool | oRefract = true, | ||
bool * | onVisibleHemisphere = nullptr ) const |
This is exactly the same as toScreenVec but it returns a QPointF.
It just calls toScreenVec and converts the result.
- See also
- toScreenVec()
Definition at line 93 of file projector.cpp.
◆ toScreenVec()
|
virtual |
Given the coordinates of the SkyPoint argument, determine the pixel coordinates in the SkyMap.
Since most of the projections used by KStars are very similar, if this function were to be reimplemented in each projection subclass we would end up changing maybe 5 or 6 lines out of 150. Instead, we have a default implementation that uses the projectionK and projectionL functions to take care of the differences between e.g. Orthographic and Stereographic. There is also the cosMaxFieldAngle function, which is used for testing whether a point is on the visible part of the projection, and the radius function which gives the radius of the projection in screen coordinates.
While this seems ugly, it is less ugly than duplicating 150 loc to change 5.
- Returns
- Eigen::Vector2f containing screen pixel x, y coordinates of SkyPoint.
- Parameters
-
o pointer to the SkyPoint for which to calculate x, y coordinates. oRefract true = use Options::useRefraction() value. false = do not use refraction. This argument is only needed for the Horizon, which should never be refracted. onVisibleHemisphere pointer to a bool to indicate whether the point is on the visible part of the Celestial Sphere.
Reimplemented in EquirectangularProjector.
Definition at line 517 of file projector.cpp.
◆ type()
|
pure virtual |
Return the type of this projection.
Implemented in AzimuthalEquidistantProjector, EquirectangularProjector, GnomonicProjector, LambertProjector, OrthographicProjector, and StereographicProjector.
◆ unusablePoint()
|
virtual |
Check if the current point on screen is a valid point on the sky.
This is needed to avoid a crash of the program if the user clicks on a point outside the sky (the corners of the sky map at the lowest zoom level are the invalid points).
- Parameters
-
p the screen pixel position
Reimplemented in EquirectangularProjector.
Definition at line 440 of file projector.cpp.
◆ updateClipPoly()
|
virtual |
updateClipPoly calculate the clipping polygen given the current FOV.
Reimplemented in EquirectangularProjector.
Definition at line 417 of file projector.cpp.
◆ viewParams()
|
inline |
Definition at line 72 of file projector.h.
Member Data Documentation
◆ m_clipPolygon
|
protected |
Definition at line 359 of file projector.h.
◆ m_cosY0
|
protected |
Definition at line 357 of file projector.h.
◆ m_data
|
protected |
Definition at line 354 of file projector.h.
◆ m_fov
|
protected |
Definition at line 358 of file projector.h.
◆ m_sinY0
|
protected |
Definition at line 356 of file projector.h.
◆ m_vp
|
protected |
Definition at line 355 of file projector.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:59:53 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.