KStarsData
#include <kstarsdata.h>
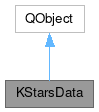
Signals | |
void | clearCache () |
void | geoChanged () |
void | progressText (const QString &text) |
void | skyUpdate (bool) |
Public Slots | |
std::pair< bool, QString > | addToUserData (const QString &name, const SkyObjectUserdata::LinkData &data) |
std::pair< bool, QString > | deleteUserData (const QString &name, const unsigned int index, SkyObjectUserdata::Type type) |
std::pair< bool, QString > | editUserData (const QString &name, const unsigned int index, const SkyObjectUserdata::LinkData &data) |
const SkyObjectUserdata::Data & | getUserData (const QString &name) |
void | setTimeDirection (float scale) |
void | slotConsoleMessage (QString s) |
void | updateTime (GeoLocation *geo, const bool automaticDSTchange=true) |
std::pair< bool, QString > | updateUserLog (const QString &name, const QString &newLog) |
Protected Member Functions | |
KStarsData () | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
Detailed Description
KStarsData is the backbone of KStars.
It contains all the data used by KStars, including the SkyMapComposite that contains all items in the skymap (stars, deep-sky objects, planets, constellations, etc). Other kinds of data are stored here as well: the geographic locations, the timezone rules, etc.
- Version
- 1.0
Definition at line 71 of file kstarsdata.h.
Constructor & Destructor Documentation
◆ KStarsData()
|
protected |
Constructor.
Definition at line 105 of file kstarsdata.cpp.
◆ ~KStarsData()
|
override |
Member Function Documentation
◆ add_color_scheme()
Register a color scheme with filename
and name
.
Definition at line 205 of file kstarsdata.h.
◆ addToUserData
|
slot |
Adds a link data
to the user data for the object with name
, both in memory and on disk.
- Returns
- {success, error_message}
Definition at line 1531 of file kstarsdata.cpp.
◆ addTransientFOV()
|
inline |
addTransientFOV Adds a new FOV to the list.
- Parameters
-
newFOV pointer to FOV object.
Definition at line 323 of file kstarsdata.h.
◆ avdTree()
|
inline |
Return ADV Tree.
Definition at line 347 of file kstarsdata.h.
◆ changeDateTime()
void KStarsData::changeDateTime | ( | const KStarsDateTime & | newDate | ) |
Change the current simulation date/time to the KStarsDateTime argument.
Specified DateTime is always universal time.
- Parameters
-
newDate the DateTime to set.
Definition at line 327 of file kstarsdata.cpp.
◆ clearCache
|
signal |
If data changed, emit clearCache signal.
◆ clearTransientFOVs()
|
inline |
Definition at line 327 of file kstarsdata.h.
◆ clock()
|
inline |
- Returns
- pointer to the simulation Clock object
Definition at line 218 of file kstarsdata.h.
◆ color_schemes()
- Returns
- a map of color scheme names and filenames
Definition at line 212 of file kstarsdata.h.
◆ colorScheme()
|
inline |
- Returns
- pointer to the ColorScheme object
Definition at line 172 of file kstarsdata.h.
◆ colorSchemeFileName() [1/2]
|
inline |
- Returns
- file name of current color scheme
Definition at line 178 of file kstarsdata.h.
◆ colorSchemeFileName() [2/2]
- Returns
- file name of the color scheme with the name
name
Definition at line 181 of file kstarsdata.h.
◆ colorSchemeName() [1/2]
|
inline |
- Returns
- file name of the current color scheme
Definition at line 187 of file kstarsdata.h.
◆ colorSchemeName() [2/2]
- Returns
- the name of the color scheme with the name
name
Definition at line 193 of file kstarsdata.h.
◆ Create()
|
static |
Definition at line 89 of file kstarsdata.cpp.
◆ deleteUserData
|
slot |
Remove data of type
from the user data at index
for the object with name
, both in memory and on disk.
- Returns
- {success, error_message}
Definition at line 1668 of file kstarsdata.cpp.
◆ editUserData
|
slot |
Replace data
in the user data at index
for the object with name
, both in memory and on disk.
- Returns
- {success, error_message}
Definition at line 1646 of file kstarsdata.cpp.
◆ executeScript()
Execute a script.
This function actually duplicates the DCOP functionality for those cases when invoking DCOP is not practical (i.e., when preparing a sky image in command-line dump mode).
- Parameters
-
name the filename of the script to "execute". map pointer to the SkyMap object.
- Returns
- true if the script was successfully parsed.
Definition at line 912 of file kstarsdata.cpp.
◆ executeSession()
Execute * KStarsData::executeSession | ( | ) |
Definition at line 1512 of file kstarsdata.cpp.
◆ geo()
|
inline |
- Returns
- pointer to the GeoLocation object
Definition at line 230 of file kstarsdata.h.
◆ geoChanged
|
signal |
Emitted when geo location changed.
◆ getAvailableFOVs()
- Returns
- the list of available FOVs
Definition at line 314 of file kstarsdata.h.
◆ getGeoList()
|
inline |
- Returns
- list of all geographic locations
Definition at line 236 of file kstarsdata.h.
◆ getRulebook()
|
inline |
Return map for daylight saving rules.
Definition at line 262 of file kstarsdata.h.
◆ getTransientFOVs()
- Returns
- the list of transient FOVs
Definition at line 335 of file kstarsdata.h.
◆ getUserData
|
slot |
Get a reference to the user data of an object with the name name
.
Definition at line 1755 of file kstarsdata.cpp.
◆ getVisibleFOVs()
- Returns
- the list of visible FOVs
Definition at line 306 of file kstarsdata.h.
◆ hasColorScheme()
- Returns
- if the color scheme with the name or filename
scheme
is loaded
Definition at line 199 of file kstarsdata.h.
◆ imageExporter()
ImageExporter * KStarsData::imageExporter | ( | ) |
Definition at line 1521 of file kstarsdata.cpp.
◆ incUpdateID()
unsigned int KStarsData::incUpdateID | ( | ) |
Definition at line 305 of file kstarsdata.cpp.
◆ initialize()
bool KStarsData::initialize | ( | ) |
Initialize KStarsData while running splash screen.
- Returns
- true on success.
This code to add Height column to table city in mycitydb.sqlite is a transitional measure to support a meaningful geographic elevation.
Definition at line 131 of file kstarsdata.cpp.
◆ Instance()
|
inlinestatic |
Definition at line 91 of file kstarsdata.h.
◆ isTimeRunningForward()
|
inline |
Returns true if time is running forward else false.
Used by KStars to prevent double calculations of daylight saving change time.
Definition at line 119 of file kstarsdata.h.
◆ locationNamed()
GeoLocation * KStarsData::locationNamed | ( | const QString & | city, |
const QString & | province = QString(), | ||
const QString & | country = QString() ) |
Definition at line 364 of file kstarsdata.cpp.
◆ logObject()
|
inline |
Return log object.
Definition at line 341 of file kstarsdata.h.
◆ lst()
|
inline |
- Returns
- pointer to the local sidereal time: a dms object
Definition at line 224 of file kstarsdata.h.
◆ lt()
|
inline |
- Returns
- pointer to the current simulation local time
Definition at line 151 of file kstarsdata.h.
◆ nearestLocation()
GeoLocation * KStarsData::nearestLocation | ( | double | longitude, |
double | latitude ) |
nearestLocation Return nearest location to the given longitude and latitude coordinates
- Parameters
-
longitude Longitude (-180 to +180) latitude Latitude (-90 to +90)
- Returns
- nearest geographical location to the parameters above.
Definition at line 377 of file kstarsdata.cpp.
◆ objectNamed()
Find object by name.
- Returns
- pointer to the localization (KLocale) object
- Parameters
-
name Object name to find
- Returns
- pointer to SkyObject matching this name
Definition at line 429 of file kstarsdata.cpp.
◆ observingList()
|
inline |
Definition at line 352 of file kstarsdata.h.
◆ progressText
Signal that specifies the text that should be drawn in the KStarsSplash window.
◆ setFullTimeUpdate()
void KStarsData::setFullTimeUpdate | ( | ) |
The Sky is updated more frequently than the moon, which is updated more frequently than the planets.
The date of the last update for each category is recorded so we know when we need to do it again (see KStars::updateTime()). Initializing these to -1000000.0 ensures they will be updated immediately on the first call to KStars::updateTime().
Definition at line 313 of file kstarsdata.cpp.
◆ setLocation()
void KStarsData::setLocation | ( | const GeoLocation & | l | ) |
Set the GeoLocation according to the argument.
- Parameters
-
l reference to the new GeoLocation
Definition at line 403 of file kstarsdata.cpp.
◆ setLocationFromOptions()
void KStarsData::setLocationFromOptions | ( | ) |
Set the GeoLocation according to the values stored in the configuration file.
Definition at line 396 of file kstarsdata.cpp.
◆ setNextDSTChange()
|
inline |
Set the NextDSTChange member.
Need this accessor because I could not make KStars::privatedata a friend class for some reason...:/
Definition at line 110 of file kstarsdata.h.
◆ setSnapNextFocus()
|
inline |
Disable or re-enable the slewing animation for the next Focus change.
- Note
- If the user has turned off all animated slewing, setSnapNextFocus(false) will NOT enable animation on the next slew. A false argument would only be used if you have previously called setSnapNextFocus(true), but then decided you didn't want that after all. In other words, it's extremely unlikely you'd ever want to use setSnapNextFocus(false).
- Parameters
-
b when true (the default), the next Focus change will omit the slewing animation.
Definition at line 283 of file kstarsdata.h.
◆ setTimeDirection
|
slot |
Sets the direction of time and stores it in bool TimeRunForwards.
If scale >= 0 time is running forward else time runs backward. We need this to calculate just one daylight saving change time (previous or next DST change).
Definition at line 359 of file kstarsdata.cpp.
◆ skyComposite()
|
inline |
- Returns
- pointer to SkyComposite
Definition at line 166 of file kstarsdata.h.
◆ skyUpdate
|
signal |
Should be used to refresh skymap.
◆ slotConsoleMessage
|
inlineslot |
send a message to the console
Definition at line 393 of file kstarsdata.h.
◆ snapNextFocus()
|
inline |
- Returns
- whether the next Focus change will omit the slewing animation.
Definition at line 268 of file kstarsdata.h.
◆ syncFOV()
void KStarsData::syncFOV | ( | ) |
Synchronize list of visible FOVs and list of selected FOVs in Options.
Definition at line 1487 of file kstarsdata.cpp.
◆ syncLST()
void KStarsData::syncLST | ( | ) |
Sync the LST with the simulation clock.
Definition at line 322 of file kstarsdata.cpp.
◆ syncUpdateIDs()
void KStarsData::syncUpdateIDs | ( | ) |
Definition at line 296 of file kstarsdata.cpp.
◆ updateID()
|
inline |
Definition at line 364 of file kstarsdata.h.
◆ updateNum()
|
inline |
Definition at line 372 of file kstarsdata.h.
◆ updateNumID()
|
inline |
Definition at line 368 of file kstarsdata.h.
◆ updateTime
|
slot |
Update the Simulation Clock.
Update positions of Planets. Update Alt/Az coordinates of objects. Update precession. emit the skyUpdate() signal so that SkyMap / whatever draws the sky can update itself
This is ugly. It will change! (JH:)hey, it's much less ugly now...can we lose the comment yet? :p
Definition at line 234 of file kstarsdata.cpp.
◆ updateUserLog
|
slot |
Update the user log of the object with the name
to contain newLog
(find and replace).
- Returns
- {success, error_message}
Definition at line 1691 of file kstarsdata.cpp.
◆ userdb()
|
inline |
- Returns
- pointer to the KSUserDB object
Definition at line 215 of file kstarsdata.h.
◆ ut()
|
inline |
- Returns
- reference to the current simulation universal time
Definition at line 157 of file kstarsdata.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Tue Mar 26 2024 11:19:04 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.