SkyMapComposite
#include <skymapcomposite.h>
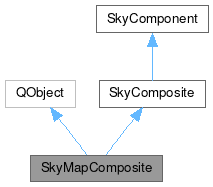
Signals | |
void | progressText (const QString &message) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
SkyMapComposite is the root object in the object hierarchy of the sky map.
All requests to update, init, draw etc. will be done with this class. The requests will be delegated to it's children. The object hierarchy will created by adding new objects via addComponent().
- Version
- 0.1
Definition at line 63 of file skymapcomposite.h.
Constructor & Destructor Documentation
◆ SkyMapComposite()
|
explicit |
Constructor parent
pointer to the parent SkyComponent.
Definition at line 58 of file skymapcomposite.cpp.
Member Function Documentation
◆ addNameLabel()
bool SkyMapComposite::addNameLabel | ( | SkyObject * | o | ) |
Definition at line 520 of file skymapcomposite.cpp.
◆ artificialHorizon()
ArtificialHorizonComponent * SkyMapComposite::artificialHorizon | ( | ) |
Definition at line 831 of file skymapcomposite.cpp.
◆ asteroids()
Definition at line 723 of file skymapcomposite.cpp.
◆ catalogsComponent()
|
inline |
Definition at line 225 of file skymapcomposite.h.
◆ comets()
Definition at line 728 of file skymapcomposite.cpp.
◆ constellationArt()
|
inline |
Definition at line 210 of file skymapcomposite.h.
◆ constellationBoundary()
|
inline |
Definition at line 179 of file skymapcomposite.h.
◆ constellationLines()
|
inline |
Definition at line 183 of file skymapcomposite.h.
◆ constellationNames()
Definition at line 712 of file skymapcomposite.cpp.
◆ constellationNamesComponent()
|
inline |
Definition at line 220 of file skymapcomposite.h.
◆ currentCulture()
QString SkyMapComposite::currentCulture | ( | ) |
Definition at line 810 of file skymapcomposite.cpp.
◆ draw()
|
overridevirtual |
Delegate draw requests to all sub components psky
Reference to the QPainter on which to paint.
Reimplemented from SkyComposite.
Definition at line 244 of file skymapcomposite.cpp.
◆ earth()
KSPlanet * SkyMapComposite::earth | ( | ) |
Definition at line 790 of file skymapcomposite.cpp.
◆ ecliptic()
|
inline |
Definition at line 188 of file skymapcomposite.h.
◆ emitProgressText()
|
overridevirtual |
Emit signal about progress.
Reimplemented from SkyComponent.
Definition at line 702 of file skymapcomposite.cpp.
◆ equator()
|
inline |
Definition at line 192 of file skymapcomposite.h.
◆ equatorialCoordGrid()
|
inline |
Definition at line 197 of file skymapcomposite.h.
◆ findByName()
Search the children of this SkyMapComposite for a SkyObject whose name matches the argument.
The objects' primary, secondary and long-form names will all be checked for a match.
- Note
- Overloaded from SkyComposite. In this version, we search the most likely object classes first to be more efficient.
name
the name to be matchedexact
If true, it will return an exact match (default), otherwise it can return a partial match.
- Returns
- a pointer to the SkyObject whose name matches the argument, or a nullptr pointer if no match was found.
Reimplemented from SkyComposite.
Definition at line 563 of file skymapcomposite.cpp.
◆ findObjectsInArea()
QList< SkyObject * > SkyMapComposite::findObjectsInArea | ( | const SkyPoint & | p1, |
const SkyPoint & | p2 ) |
- Returns
- the list of objects in the region defined by skypoints
- Parameters
-
p1 first sky point (top-left vertex of rectangular region) p2 second sky point (bottom-right vertex of rectangular region)
Definition at line 549 of file skymapcomposite.cpp.
◆ findStarByGenetiveName()
Definition at line 591 of file skymapcomposite.cpp.
◆ flags()
FlagComponent * SkyMapComposite::flags | ( | ) |
Definition at line 815 of file skymapcomposite.cpp.
◆ getCultureName()
QString SkyMapComposite::getCultureName | ( | int | index | ) |
Definition at line 800 of file skymapcomposite.cpp.
◆ getCultureNames()
QStringList SkyMapComposite::getCultureNames | ( | ) |
Definition at line 795 of file skymapcomposite.cpp.
◆ getSkyObjectsList()
const QList< SkyObject * > * SkyMapComposite::getSkyObjectsList | ( | SkyObject::TYPE | t | ) |
Definition at line 758 of file skymapcomposite.cpp.
◆ getStarHopRouteList()
|
inline |
Definition at line 268 of file skymapcomposite.h.
◆ horizon()
|
inline |
Getters for SkyComponents.
Definition at line 174 of file skymapcomposite.h.
◆ horizontalCoordGrid()
|
inline |
Definition at line 201 of file skymapcomposite.h.
◆ imageOverlay()
ImageOverlayComponent * SkyMapComposite::imageOverlay | ( | ) |
Definition at line 836 of file skymapcomposite.cpp.
◆ isLocalCNames()
bool SkyMapComposite::isLocalCNames | ( | ) |
Definition at line 697 of file skymapcomposite.cpp.
◆ labelObjects()
Definition at line 245 of file skymapcomposite.h.
◆ localMeridianComponent()
|
inline |
Definition at line 205 of file skymapcomposite.h.
◆ milkyWay()
|
inline |
Definition at line 230 of file skymapcomposite.h.
◆ objectNearest()
- Returns
- the object nearest a given point in the sky.
- Parameters
-
p The point to find an object near maxrad The maximum search radius, in Degrees
- Note
- the angular separation to the matched object is returned through the maxrad variable.
Reimplemented from SkyComposite.
Definition at line 418 of file skymapcomposite.cpp.
◆ planet()
KSPlanetBase * SkyMapComposite::planet | ( | int | n | ) |
Definition at line 596 of file skymapcomposite.cpp.
◆ planets()
Definition at line 738 of file skymapcomposite.cpp.
◆ reloadCLines()
void SkyMapComposite::reloadCLines | ( | ) |
Definition at line 621 of file skymapcomposite.cpp.
◆ reloadCNames()
void SkyMapComposite::reloadCNames | ( | ) |
Definition at line 634 of file skymapcomposite.cpp.
◆ reloadConstellationArt()
void SkyMapComposite::reloadConstellationArt | ( | ) |
Definition at line 650 of file skymapcomposite.cpp.
◆ reloadDeepSky()
void SkyMapComposite::reloadDeepSky | ( | ) |
Definition at line 663 of file skymapcomposite.cpp.
◆ removeNameLabel()
bool SkyMapComposite::removeNameLabel | ( | SkyObject * | o | ) |
Definition at line 528 of file skymapcomposite.cpp.
◆ satellites()
SatellitesComponent * SkyMapComposite::satellites | ( | ) |
Definition at line 821 of file skymapcomposite.cpp.
◆ setCurrentCulture()
void SkyMapComposite::setCurrentCulture | ( | QString | culture | ) |
Definition at line 805 of file skymapcomposite.cpp.
◆ solarSystemComposite()
|
inline |
Definition at line 215 of file skymapcomposite.h.
◆ starNearest()
- Returns
- the star nearest a given point in the sky.
- Parameters
-
p The point to find a star near maxrad The maximum search radius, in Degrees
- Note
- the angular separation to the matched star is returned through the maxrad variable.
Definition at line 502 of file skymapcomposite.cpp.
◆ stars()
Definition at line 718 of file skymapcomposite.cpp.
◆ supernovae()
Definition at line 733 of file skymapcomposite.cpp.
◆ supernovaeComponent()
SupernovaeComponent * SkyMapComposite::supernovaeComponent | ( | ) |
Definition at line 826 of file skymapcomposite.cpp.
◆ update()
|
overridevirtual |
Delegate update-position requests to all sub components.
This function usually just updates the Horizontal (Azimuth/Altitude) coordinates. However, the precession and nutation must also be recomputed periodically. Requests to do so are sent through the doPrecess parameter. num
Pointer to the KSNumbers object
- See also
- updatePlanets()
- updateMoons()
- Note
- By default, the num parameter is nullptr, indicating that Precession/Nutation computation should be skipped; this computation is only occasionally required.
Reimplemented from SkyComposite.
Definition at line 186 of file skymapcomposite.cpp.
◆ updateMoons()
|
overridevirtual |
Delegate moon position updates to the SolarSystemComposite.
Planet positions change over time, so they need to be recomputed periodically, but not on every call to update(). This function will recompute the positions of the Earth's Moon and Jupiter's four Galilean moons. These objects are done separately from the other solar system bodies, because their positions change more rapidly, and so updateMoons() must be called more often than updatePlanets(). num
Pointer to the KSNumbers object
- See also
- update()
- updatePlanets()
- SolarSystemComposite::updateMoons()
Reimplemented from SkyComponent.
Definition at line 233 of file skymapcomposite.cpp.
◆ updateSolarSystemBodies()
|
overridevirtual |
Delegate planet position updates to the SolarSystemComposite.
Planet positions change over time, so they need to be recomputed periodically, but not on every call to update(). This function will recompute the positions of all solar system bodies except the Earth's Moon, Jupiter's Moons AND Saturn Moons (because these objects' positions change on a much more rapid timescale). num
Pointer to the KSNumbers object
- See also
- update()
- updateMoons()
- SolarSystemComposite::updatePlanets()
Reimplemented from SkyComponent.
Definition at line 228 of file skymapcomposite.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.