FlagComponent
#include <flagcomponent.h>
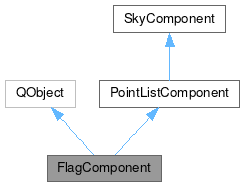
Public Member Functions | |
FlagComponent (SkyComposite *) | |
void | add (const SkyPoint &flagPoint, QString epoch, QString image, QString label, QColor labelColor) |
void | draw (SkyPainter *skyp) override |
QString | epoch (int index) |
QPair< double, double > | epochCoords (int index) |
QList< int > | getFlagsNearPix (SkyPoint *point, int pixelRadius) |
QStringList | getNames () |
QImage | image (int index) |
QList< QImage > | imageList () |
QImage | imageList (int index) |
QString | imageName (int index) |
QString | label (int index) |
QColor | labelColor (int index) |
void | loadFromFile () |
void | remove (int index) |
void | saveToFile () |
bool | selected () override |
int | size () |
void | update (KSNumbers *num=nullptr) override |
void | updateFlag (int index, const SkyPoint &flagPoint, QString epoch, QString image, QString label, QColor labelColor) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
PointListComponent (SkyComposite *parent) | |
QList< std::shared_ptr< SkyPoint > > & | pointList () |
void | update (KSNumbers *num=nullptr) override |
![]() | |
SkyComponent (SkyComposite *parent=nullptr) | |
virtual void | drawTrails (SkyPainter *skyp) |
virtual void | emitProgressText (const QString &message) |
virtual SkyObject * | findByName (const QString &name, bool exact=true) |
QHash< int, QVector< QPair< QString, const SkyObject * > > > & | objectLists () |
QVector< QPair< QString, const SkyObject * > > & | objectLists (int type) |
QHash< int, QStringList > & | objectNames () |
QStringList & | objectNames (int type) |
virtual SkyObject * | objectNearest (SkyPoint *p, double &maxrad) |
virtual void | objectsInArea (QList< SkyObject * > &list, const SkyRegion ®ion) |
SkyComposite * | parent () |
void | removeFromLists (const SkyObject *obj) |
void | removeFromNames (const SkyObject *obj) |
virtual void | updateMoons (KSNumbers *) |
virtual void | updateSolarSystemBodies (KSNumbers *) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Represents a flag on the sky map.
Each flag is composed by a SkyPoint where coordinates are stored, an epoch and a label. This class also stores flag images and associates each flag with an image. When FlagComponent is created, it seeks all file names beginning with "_flag" in the user directory and consider them as flag images.
The file flags.dat stores coordinates, epoch, image name and label of each flags and is read to init FlagComponent
- Version
- 1.1
Definition at line 33 of file flagcomponent.h.
Constructor & Destructor Documentation
◆ FlagComponent()
|
explicit |
Constructor.
Definition at line 28 of file flagcomponent.cpp.
Member Function Documentation
◆ add()
void FlagComponent::add | ( | const SkyPoint & | flagPoint, |
QString | epoch, | ||
QString | image, | ||
QString | label, | ||
QColor | labelColor ) |
Add a flag.
- Parameters
-
flagPoint Sky point in epoch coordinates epoch Moment for which celestial coordinates are specified image Image name label Label of the flag labelColor Color of the label
Definition at line 149 of file flagcomponent.cpp.
◆ draw()
|
overridevirtual |
Draw the object on the SkyMap skyp
a pointer to the SkyPainter to use.
Implements SkyComponent.
Definition at line 52 of file flagcomponent.cpp.
◆ epoch()
QString FlagComponent::epoch | ( | int | index | ) |
Get epoch.
- Returns
- the epoch as a string
- Parameters
-
index Index of the flag
Definition at line 236 of file flagcomponent.cpp.
◆ epochCoords()
QPair< double, double > FlagComponent::epochCoords | ( | int | index | ) |
epochCoords return coordinates recorded in original epoch
- Parameters
-
index index of the flag
- Returns
- pair of RA/DEC in original epoch
Definition at line 358 of file flagcomponent.cpp.
◆ getFlagsNearPix()
Get list of flag indexes near specified SkyPoint with radius specified in pixels.
- Parameters
-
point central SkyPoint. pixelRadius radius in pixels.
Definition at line 301 of file flagcomponent.cpp.
◆ getNames()
QStringList FlagComponent::getNames | ( | ) |
Return image names.
- Returns
- the list of all image names
Definition at line 226 of file flagcomponent.cpp.
◆ image()
QImage FlagComponent::image | ( | int | index | ) |
Get image.
- Returns
- the image associated with the flag
- Parameters
-
index Index of the flag
Definition at line 266 of file flagcomponent.cpp.
◆ imageList() [1/2]
◆ imageList() [2/2]
QImage FlagComponent::imageList | ( | int | index | ) |
Get image.
- Parameters
-
index Index of the image in m_Images
- Returns
- an image from m_Images
Definition at line 333 of file flagcomponent.cpp.
◆ imageName()
QString FlagComponent::imageName | ( | int | index | ) |
Get image name.
- Returns
- the name of the image associated with the flag
- Parameters
-
index Index of the flag
Definition at line 281 of file flagcomponent.cpp.
◆ label()
QString FlagComponent::label | ( | int | index | ) |
Get label.
- Returns
- the label as a string
- Parameters
-
index Index of the flag
Definition at line 246 of file flagcomponent.cpp.
◆ labelColor()
QColor FlagComponent::labelColor | ( | int | index | ) |
Get label color.
- Returns
- the label color
- Parameters
-
index Index of the flag
Definition at line 256 of file flagcomponent.cpp.
◆ loadFromFile()
void FlagComponent::loadFromFile | ( | ) |
Load flags from flags.dat file.
Definition at line 71 of file flagcomponent.cpp.
◆ remove()
void FlagComponent::remove | ( | int | index | ) |
Remove a flag.
- Parameters
-
index Index of the flag to be remove.
Definition at line 175 of file flagcomponent.cpp.
◆ saveToFile()
void FlagComponent::saveToFile | ( | ) |
Save flags to flags.dat file.
Definition at line 133 of file flagcomponent.cpp.
◆ selected()
|
overridevirtual |
- Returns
- true if component is to be drawn on the map.
Reimplemented from SkyComponent.
Definition at line 66 of file flagcomponent.cpp.
◆ size()
int FlagComponent::size | ( | ) |
Return the numbers of flags.
- Returns
- the size of m_PointList
Definition at line 231 of file flagcomponent.cpp.
◆ update()
|
overridevirtual |
Update the sky position(s) of this component.
This function usually just updates the Horizontal (Azimuth/Altitude) coordinates of its member object(s). However, the precession and nutation must also be recomputed periodically. num
Pointer to the KSNumbers object
- See also
- SingleComponent::update()
- ListComponent::update()
- ConstellationBoundaryComponent::update()
Reimplemented from SkyComponent.
Definition at line 369 of file flagcomponent.cpp.
◆ updateFlag()
void FlagComponent::updateFlag | ( | int | index, |
const SkyPoint & | flagPoint, | ||
QString | epoch, | ||
QString | image, | ||
QString | label, | ||
QColor | labelColor ) |
Update a flag.
- Parameters
-
index index of the flag to be updated. flagPoint point of the flag. epoch new flag epoch. image new flag image. label new flag label. labelColor new flag label color.
Definition at line 196 of file flagcomponent.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 20 2024 11:53:01 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.